In C++, a struct can have default values for its member variables, allowing instances of the struct to initialize those members automatically when created. Here's an example:
struct Point {
int x = 0; // Default value for x
int y = 0; // Default value for y
};
Point p; // p.x and p.y will both be initialized to 0
Understanding C++ Structs
What is a C++ Struct?
A C++ struct is a user-defined data type that groups related variables under a single unit. It is similar to a class, but with default public access for its members. Structs are commonly used to represent a collection of related properties or attributes, making it easier to manage and handle these data points together.
Use Cases of Structs in C++
Structs are particularly useful in situations where you want to combine different data types. For example, in a game, you might use a struct to represent a player's attributes, such as health, score, and inventory items.
Basic Syntax of C++ Structs
The basic syntax for defining a struct in C++ looks like this:
struct Person {
std::string name;
int age;
};
This snippet defines a struct called `Person` that contains two members: `name` of type `std::string` and `age` of type `int`. Each instance of this struct can represent a unique person with associated name and age attributes.

C++ Struct Default Values
Introduction to Default Values
Default values in C++ structs allow you to set initial values for struct members when you create an instance of the struct. This can significantly enhance the usability of your structs and provide a clear starting point for your data.
How to Set Default Values in a C++ Struct
You can set default values directly in the member declarations. This is straightforward and makes your intent clear when someone reads the code:
struct Person {
std::string name = "Unknown";
int age = 0;
};
In the above example, any instance of `Person` will have `name` initialized to "Unknown" and `age` initialized to `0` unless specified otherwise during instantiation.
Using Constructors for Default Values
Constructors provide a more flexible way to set default values, especially when you want to initialize complex types or include logic. Here's how to define a constructor to set default values:
struct Person {
std::string name;
int age;
Person() : name("Unknown"), age(0) {}
};
In this example, whenever a `Person` object is created without providing explicit values, the constructor initializes `name` and `age` to "Unknown" and `0", respectively.

Benefits of Using Default Values in C++ Structs
Improved Code Readability
Setting default values can greatly enhance code readability. By providing sensible defaults, the intent of your code becomes clearer, allowing others (or even you, at a later time) to understand the expected values without needing to trace through the initialization code.
Consider the following comparison:
Without default values:
Person p1;
// p1.name and p1.age are uninitialized
With default values:
Person p2; // p2.name = "Unknown", p2.age = 0
In the second example, the state of `p2` is immediately clear.
Reduced Initialization Errors
Using default values helps to prevent uninitialized variable errors, a common source of bugs. If you fail to assign initial values, you might inadvertently work with undefined data, leading to unpredictable behavior.
For instance, the following code could lead to issues:
Person p1;
std::cout << p1.age; // Undefined behavior if age is uninitialized
In contrast, with default values, `p2.age` will always print `0`, providing a clear and safe starting point.

Common Pitfalls to Avoid with C++ Struct Default Values
Forgetting to Initialize
One common mistake is omitting default values entirely, which can lead to uninitialized data. This can cause unexpected behavior, making your program harder to debug.
Misusing Default Values
While default values can be powerful, using them inappropriately can cause confusion or mislead other programmers. It's essential to understand the context in which defaults make sense. For instance, different structs might not be compatible with the same default values, affecting program logic.
struct Vehicle {
std::string model = "Unknown Model"; // Could be misleading if not applicable
};
// When to provide explicit values becomes crucial
Vehicle v; // What does "Unknown Model" mean in this context?
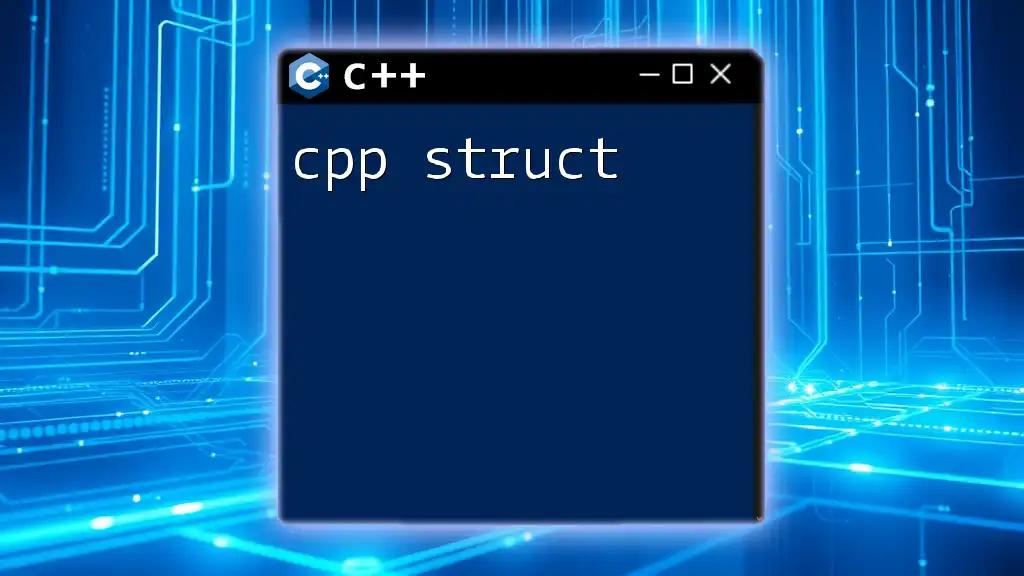
Best Practices for Using Default Values in C++ Structs
Keep It Simple
When designing structs, simplicity should be your guiding principle. By keeping your default values straightforward and intuitive, you help maintain clarity and usability.
Use Consistent Names and Types
Consistency in naming and typing across your structs promotes ease of understanding. For example:
struct Student {
std::string firstName = "John";
std::string lastName = "Doe";
int credits = 0;
};
Here, the uniform approach to naming types helps users grasp the struct’s purpose quickly.

Advanced Techniques
Default Values with Nested Structs
In more complex applications, you might encounter nested structs. Setting default values within nested structs can be done just like with regular structs:
struct Address {
std::string city = "Unknown City";
std::string state = "Unknown State";
};
struct Person {
std::string name;
int age;
Address address; // Nested struct
};
In this case, if you create a `Person` instance without explicitly detailing the `Address`, it automatically initializes with "Unknown City" and "Unknown State".
Combining Default Values with Inheritance
When dealing with inheritance, you can also utilize default values effectively. Here's an example with structs:
struct Employee : public Person {
std::string jobTitle = "Employee";
};
In this struct, any `Employee` instance will inherit the default values from `Person` while also initializing its own member `jobTitle`.
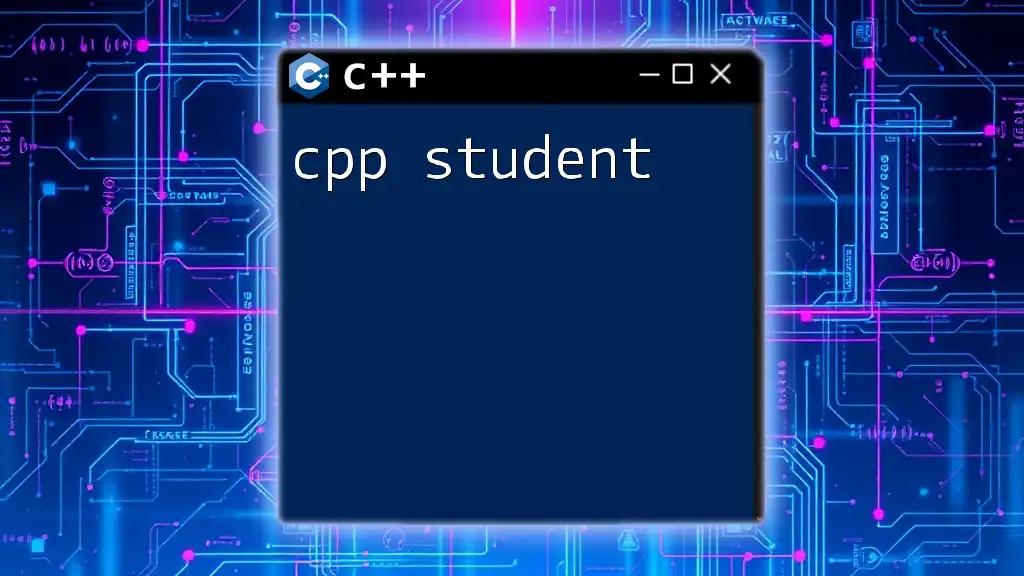
Conclusion
Incorporating cpp struct default values into your programming practice can lead to clearer, safer, and more maintainable code. By understanding how to effectively set and manage these defaults, along with being aware of common pitfalls, you can create robust data structures that facilitate easier developmental workflows.
Engage in practical experimentation with your own structs and see how default values impact your code. Practice not only enhances understanding— it also fosters an instinct for efficient programming.

Additional Resources
If you're eager to delve deeper, consider exploring recommended readings or joining C++ community platforms where developers share insights and best practices. Learning from others can provide valuable techniques and help further your understanding of this powerful programming language.