"CPP student jobs involve practical positions where students can apply their knowledge of C++ programming through internships or entry-level tasks, allowing them to gain hands-on experience in the tech industry."
Here’s a simple example of a C++ command that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ and Its Importance
What is C++?
C++ is a high-level programming language developed in the early 1980s, created by Bjarne Stroustrup at Bell Labs. It is an extension of the C programming language and incorporates features such as object-oriented programming (OOP), generic programming, and low-level memory manipulation. This versatility allows C++ to be widely used across various domains, ranging from system software to game development.
Key features of C++ include:
- Object-Oriented Programming: C++ promotes the use of classes and objects, making it easier to organize and manage complex code.
- Efficiency and Speed: Due to its low-level capabilities, C++ is known for its performance, which is crucial for system applications and resource-intensive programming tasks.
- Standard Template Library (STL): It provides a rich set of algorithms and data structures that streamline development processes.
Why C++ is Valuable in the Job Market
The demand for C++ developers in the tech world is immense, owing to its widespread applicability.
Industries heavily reliant on C++ include:
- Gaming: Many game engines, such as Unreal Engine, are built on C++ due to its performance and control over system resources.
- Finance: High-frequency trading systems and quantitative analysis tools often utilize C++ for faster computation.
- Embedded Systems: C++ is used in developing applications for devices that have limited processing capabilities, such as IoT devices.
Future trends indicate that as technology evolves, the need for C++ expertise will continue to grow, especially in areas such as artificial intelligence and machine learning.
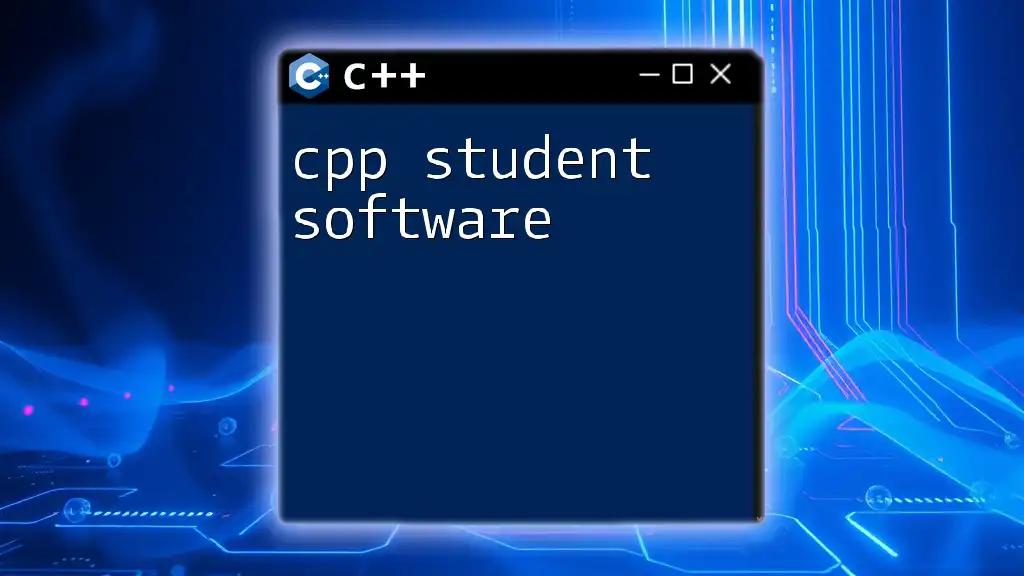
Types of Student Jobs in C++
Internships
Internships are often the first step into the professional world, providing valuable experience and insights into working environments. They offer a chance to apply classroom knowledge to real-world challenges.
To find C++ internship opportunities, students should:
- Utilize platforms such as LinkedIn and Glassdoor.
- Attend college career fairs and networking events.
- Reach out to local startups or tech companies directly.
During an internship, students can expect to work on a variety of tasks, which may include developing software components, debugging code, and collaborating with teams on projects. For instance, an intern might create a simple inventory management system using C++.
Part-time Positions
Part-time programming jobs are another fantastic option for students looking to build their C++ skills while balancing other commitments. These roles typically allow employees to work flexible hours, making them ideal for busy students.
Finding part-time C++ jobs can be achieved through job boards like Indeed or specialized tech job sites. Responsibilities may vary widely, but they often involve writing code, participating in code reviews, and testing features.
Freelancing
Freelancing provides a dynamic way to work as a C++ developer. It allows students to pick projects that pique their interest while working independently.
To get started in freelancing:
- Join platforms like Upwork or Freelancer, where students can post their skills and bid on C++ projects.
- Build a presence by contributing to open-source projects on GitHub or similar platforms.
Pros of freelancing include the opportunity to work on diverse projects and flexible schedules, while cons may involve inconsistent income and the need for self-promotion.
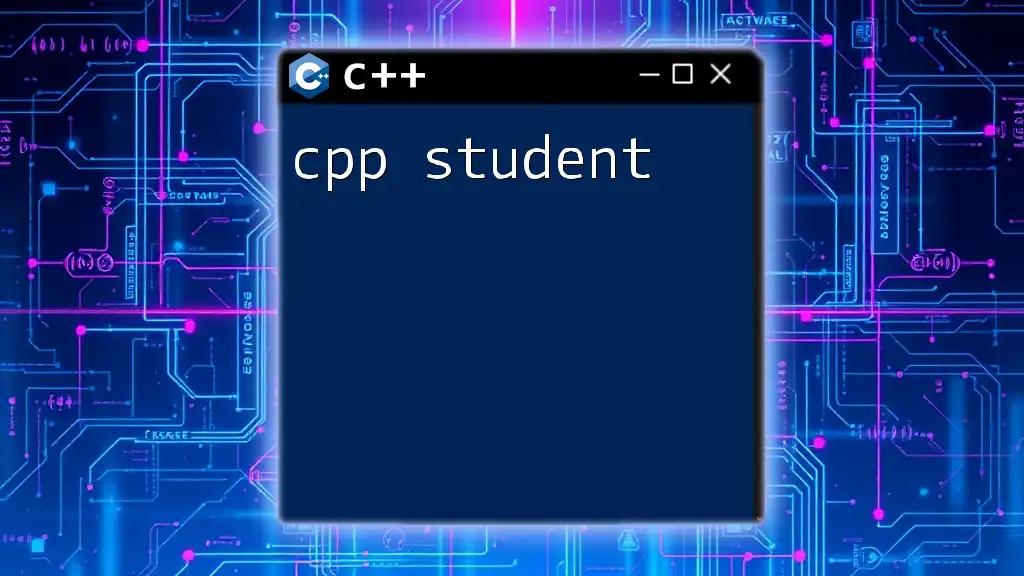
Skills Required for C++ Student Jobs
Core C++ Skills
Proficiency in C++ is crucial for landing a job. Students should be comfortable with both basic and advanced concepts.
For example, understanding polymorphism is essential in designing flexible and reusable code. Here’s a straightforward implementation:
#include <iostream>
using namespace std;
class Animal {
public:
virtual void sound() { cout << "Some sound\n"; }
};
class Dog : public Animal {
public:
void sound() override { cout << "Bark\n"; }
};
void animalSound(Animal *a) {
a->sound();
}
int main() {
Animal *a = new Dog();
animalSound(a);
delete a;
return 0;
}
In the example above, we see how a base class `Animal` can define a function that is overridden by a derived class `Dog`. This encapsulates specific behaviors while maintaining a consistent interface.
Other Necessary Skills
In addition to core C++ knowledge, students should also develop a set of complementary skills:
- Version Control: Mastery of tools like Git is essential for collaborating and managing code effectively.
- Problem-Solving Skills: Strong analytical reasoning will help in tackling real-world problems efficiently.
- Algorithms and Data Structures: A solid understanding of algorithms (like sorting and searching) and data structures (such as arrays, lists, and graphs) is necessary for optimizing code and tackling technical interviews.
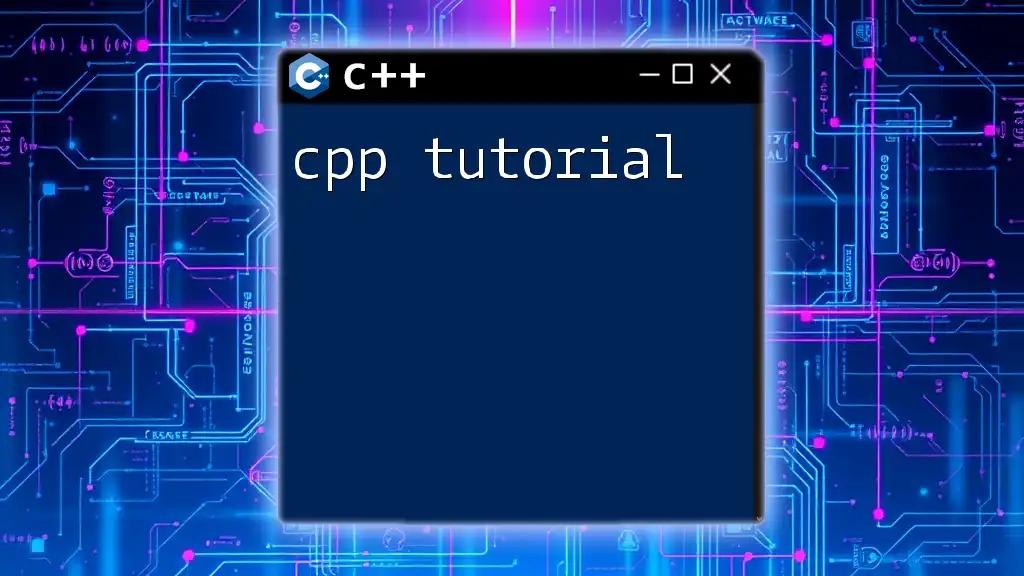
Building a Strong Resume and Portfolio
Crafting Your Resume
When applying for C++ jobs, a well-structured resume is critical. Include elements such as:
- Your education (degree, major, and relevant coursework)
- C++ projects (both academic and personal experiences)
- Any internships or relevant work experience
Tailor your resume to highlight skills and experiences relevant to C++ development, ensuring keywords are included that match the job description.
Creating a Portfolio
A portfolio demonstrates your practical skills and showcases your projects. It should feature:
- Code snippets and explanations of your projects.
- Links to your repositories on GitHub or other code hosting platforms.
- Descriptions of how you solved coding challenges or built applications.
The portfolio serves as a compelling visual representation of your capabilities and engages potential employers.
Personal Branding
Having a strong personal brand can set you apart. Create an online presence through platforms like LinkedIn, where you can:
- Share articles about C++ development and trends.
- Connect with industry professionals.
- Highlight your completed projects.
Networking is invaluable in tech fields. By participating in forums, attending meetups, and engaging in coding communities, you will build relationships that could lead to job opportunities.

Interview Preparation
Common C++ Interview Questions
Familiarize yourself with common technical questions that may arise in interviews for C++ roles. Anticipate queries that test your understanding of algorithms, data structures, or C++ features. Common questions may include:
- Explain the principle of encapsulation in C++.
- How does inheritance work, and why is it useful?
Behavioral Interview Preparation
In addition to technical skills, employers often assess candidates based on behavioral attributes. Prepare for behavioral interviews by anticipating questions such as:
- "Describe a time when you faced a significant challenge in a project." Using the STAR method (Situation, Task, Action, Result), structure your answers to demonstrate your problem-solving abilities and teamwork experience.
Mock Interviews
Engaging in mock interviews is an effective way to prepare. Practice with peers or mentors who can provide constructive feedback. This experience builds confidence and sharpens your ability to think on your feet during actual interviews.

Conclusion
In summary, pursuing C++ student jobs offers a tremendous opportunity to deepen your programming knowledge and gain invaluable real-world experience. Embracing internships, part-time roles, or freelance projects can help you build confidence and professional connections. Remember that every opportunity is a step towards your career goal, so embrace the learning journey. Join our workshops and courses to enhance your C++ skills further and stand out in this competitive job market!