C++ streams are used to perform input and output operations in a standardized way, allowing programmers to read data from input devices and write data to output devices.
Here's a simple example of using input and output streams in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Output to console
int number;
std::cin >> number; // Input from user
std::cout << "You entered: " << number << std::endl; // Output the entered number
return 0;
}
What is a Stream in C++?
In C++, a stream is an abstraction that represents a flow of data. It allows you to perform input and output operations efficiently using a consistent interface, regardless of the source or destination of the data. At its core, a stream can be thought of as a sequence of bytes that can be read from or written to.
Understanding Streams
Streams facilitate interaction with various data sources, ranging from console inputs to files, and they abstract the underlying complexities. A stream provides a layer that normalizes how data is handled, making it easier for programmers to read and write data without worrying about the specifics of the medium.
Types of C++ Streams
C++ streams can be categorized into several types:
-
Input Streams: These are used to read data from a source, such as the console or a file. The most common input stream object is `cin`, which reads input from standard input (keyboard).
-
Output Streams: These allow you to send data to a destination, like the console or a file. The most commonly used output stream is `cout`, which sends output to standard output (screen).
-
String Streams: C++ also offers `stringstream`, which allows you to treat strings as input or output streams, enabling you to manipulate string data as if it were a stream.
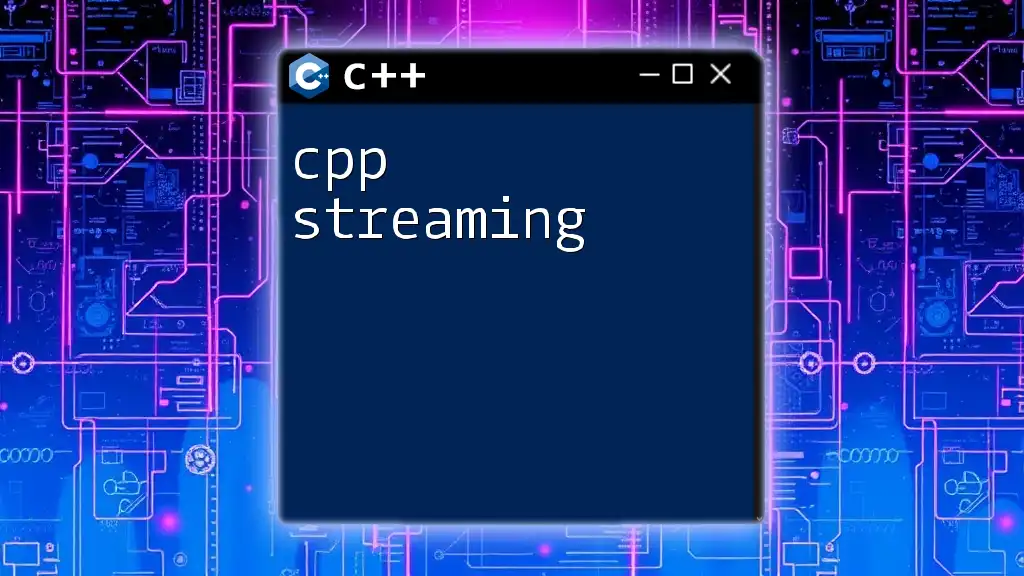
C++ Iostream: A Closer Look
Introduction to the iostream Library
C++ incorporates the `iostream` library, which defines objects and functions to perform input and output operations. You include this library in your program with:
#include <iostream>
This inclusion provides access to several standard objects used for I/O operations.
Commonly Used I/O Objects
- std::cin: This object represents the standard input stream. It's used to receive input from the user. Typically, you would use it like this:
int age;
std::cout << "Enter your age: ";
std::cin >> age;
- std::cout: The standard output stream sends data to the console. Here’s how it works:
std::cout << "Hello, World!" << std::endl;
- std::cerr: It is used for error messages and outputs unbuffered data, which makes it suitable for logging errors. Here is an example:
std::cerr << "Error: Cannot open file." << std::endl;
- std::clog: Contrary to `cerr`, `clog` is used for logging messages and is buffered. This means that it is suitable for general logging as it may improve performance:
std::clog << "Log: Process started." << std::endl;
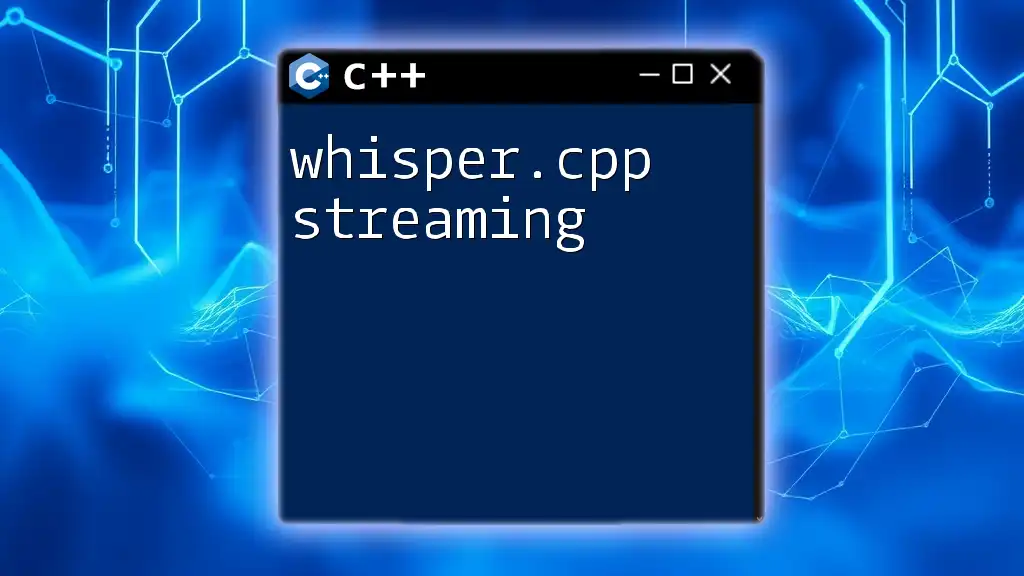
How to Use C++ Streams Effectively
Basic Input/Output Operations
Using streams in C++ typically involves reading data from an input stream and writing data to an output stream. This interaction is straightforward:
std::string name;
std::cout << "Enter your name: ";
std::cin >> name;
std::cout << "Welcome, " << name << "!" << std::endl;
Formatted Input/Output
C++ provides manipulators, which are special functions that adjust the stream's behavior. Common manipulators include:
- std::endl: Used to end a line and flush the output buffer.
- std::setw: Sets the width of the next output field.
Example of using manipulators:
std::cout << std::setw(10) << "Name" << std::setw(5) << "Age" << std::endl;
std::cout << std::setw(10) << name << std::setw(5) << age << std::endl;
Error Handling in C++ Streams
Stream operations can fail for various reasons (e.g., end of file, reading an invalid input). Checking the stream's state is crucial. You can check if an input operation was successful by using:
if (std::cin.fail()) {
std::cerr << "Input error!" << std::endl;
}
Common stream state flags include:
- eof: End of file reached.
- fail: A logical error occurred on input/output operation.
- bad: A read/write error on the stream.
Always ensure to validate the results of your stream operations to prevent unexpected behavior.
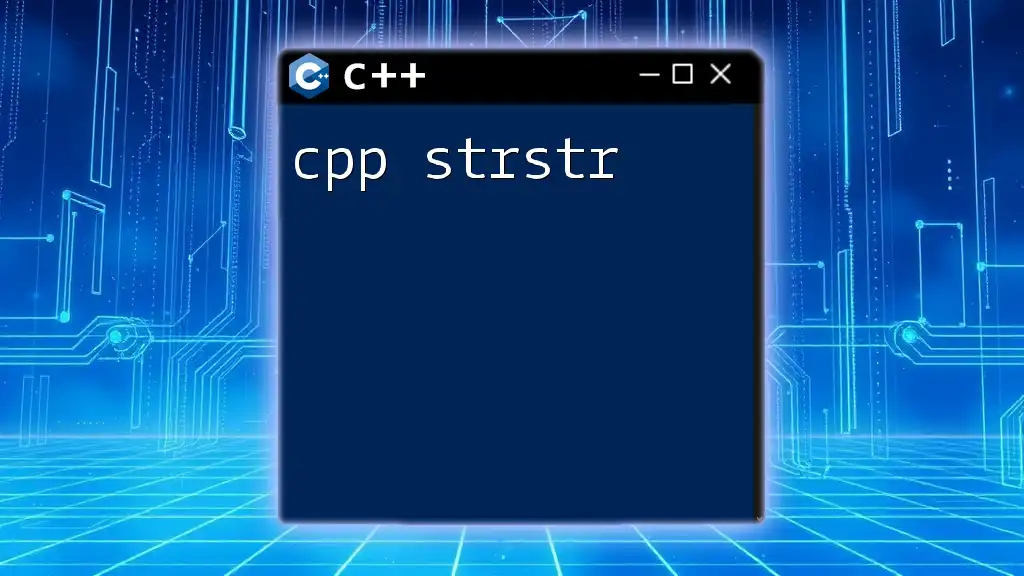
Advanced Topics in C++ Streams
File I/O Streams
File handling in C++ is accomplished using the `fstream`, `ifstream`, and `ofstream` classes.
- Reading from Files: To read data from a file, you would typically use `ifstream`:
std::ifstream inFile("example.txt");
std::string line;
if (inFile.is_open()) {
while (getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
- Writing to Files: With `ofstream`, you can write data to files easily:
std::ofstream outFile("output.txt");
outFile << "Hello, file!" << std::endl;
outFile.close();
String Streams in C++
`stringstream` provides a unique way to handle strings as streams, allowing for convenient string manipulation. Here’s an example of how you can use it:
std::stringstream ss;
ss << "Hello, " << name << "!";
std::string greeting = ss.str();
std::cout << greeting << std::endl;
This allows you to construct complex strings dynamically, very similar to how one would format output.
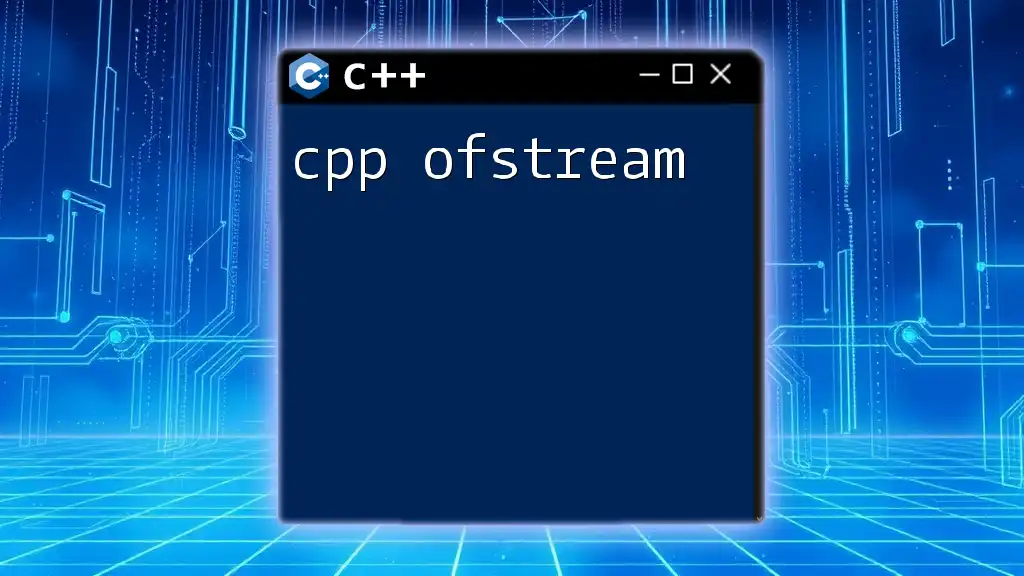
Best Practices for Using C++ Streams
Do's and Don'ts with C++ Streams
- Do always validate your inputs and outputs to ensure stability.
- Do not forget to explicitly close file streams after operations to prevent resource leaks.
- Do prefer `std::cerr` for error messages to ensure they are outputted promptly.
Performance Considerations
While streams provide a convenient interface for I/O, frequent stream operations can become a bottleneck. To mitigate performance issues, consider:
- Buffering output: Use `std::ios::sync_with_stdio(false)` to improve performance for extensive I/O operations.
- Minimizing interactions with the stream: Batch read/write operations where possible.
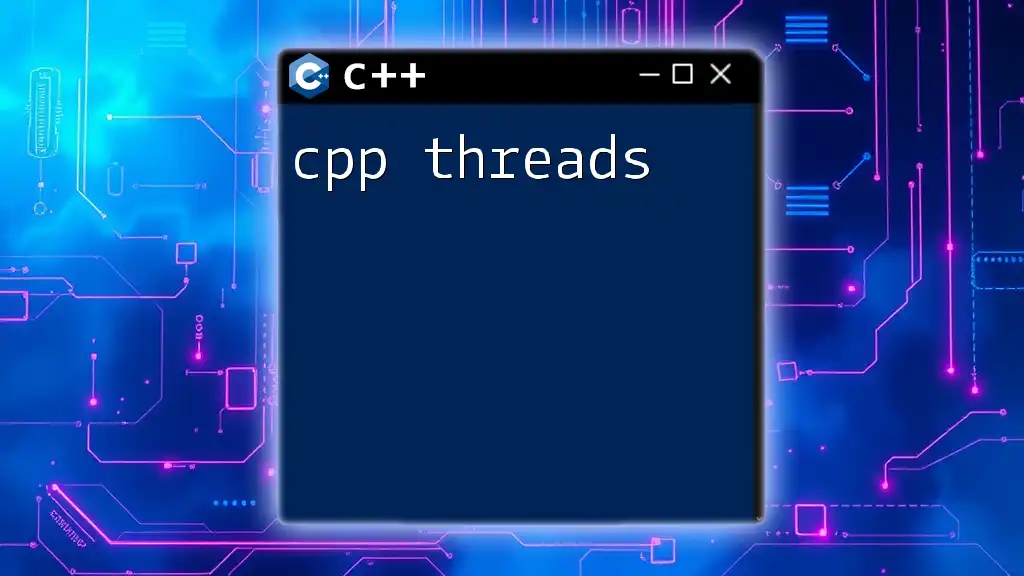
Conclusion
Understanding and effectively utilizing C++ streams is fundamental to mastering input and output operations in your programs. By grasping the principles of streams and synchronized I/O, you unlock the ability to handle data flow flexibly and efficiently. Whether you are dealing with user input, file handling, or string manipulation, mastering C++ streams sets a solid foundation for robust program design.
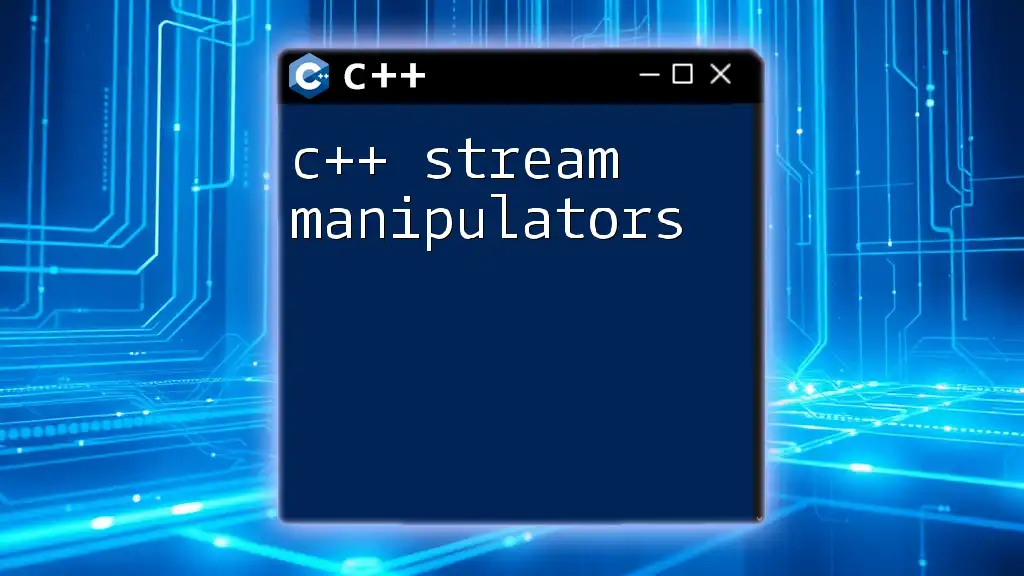
Further Reading and Resources
For those eager to delve deeper into the world of C++ streams, consider referring to the official documentation for detailed guidelines and advanced features. There are numerous tutorials and online courses available that can enhance your understanding and proficiency with C++ streams.