A C++ tree is a data structure that consists of nodes connected by edges, commonly used to represent hierarchical relationships, where each node can have multiple children but only one parent.
Here’s a simple implementation of a binary tree in C++:
#include <iostream>
struct Node {
int data;
Node* left;
Node* right;
Node(int value) : data(value), left(nullptr), right(nullptr) {}
};
class BinaryTree {
public:
Node* root;
BinaryTree() : root(nullptr) {}
void insert(int value) {
root = insertRec(root, value);
}
Node* insertRec(Node* node, int value) {
if (node == nullptr) {
return new Node(value);
}
if (value < node->data) {
node->left = insertRec(node->left, value);
} else {
node->right = insertRec(node->right, value);
}
return node;
}
};
int main() {
BinaryTree tree;
tree.insert(5);
tree.insert(3);
tree.insert(7);
// Additional operations can be added here
return 0;
}
Understanding Tree Fundamentals
Basic Terminology
To fully grasp the concept of a cpp tree, it's essential to familiarize yourself with some fundamental terms:
- Node: A basic unit of a tree, where each node contains data and can link to other nodes.
- Edge: The connection between two nodes in a tree.
- Root: The topmost node of a tree, serving as the starting point for operations.
- Leaves: Nodes with no children, often found at the lowest level of the tree.
- Height: The height of a tree is defined as the number of edges on the longest path from the root to a leaf.
Types of Trees
C++ supports various tree structures, each serving its unique purpose.
Binary Trees
A binary tree is a tree where each node has at most two children, often referred to as the left and right children. The properties of binary trees are crucial for other structures, such as binary search trees.
Binary Search Trees (BST)
A binary search tree is a type of binary tree where nodes are arranged such that the left child's value is less than the parent's, and the right child's value is greater. This property allows for efficient searching and sorting.
Example of insertion and searching in a BST:
Node* insert(Node* root, int value) {
if (root == nullptr) {
return new Node(value);
}
if (value < root->data) {
root->left = insert(root->left, value);
} else {
root->right = insert(root->right, value);
}
return root;
}
When searching for a value:
bool search(Node* root, int value) {
if (root == nullptr) {
return false;
}
if (root->data == value) {
return true;
}
return value < root->data ? search(root->left, value) : search(root->right, value);
}
Balanced Trees
Balanced trees are designed to maintain minimal height, ensuring that the tree remains balanced during insertions and deletions. Popular types include:
- AVL Trees: Automatically balances itself through rotations after operations.
- Red-Black Trees: A self-balancing binary search tree that guarantees a height of log(n).
N-ary Trees
An N-ary tree is a tree in which each node can have N children, making it suitable for hierarchical data representation. These trees are useful in applications like parsing expressions or representing file systems.
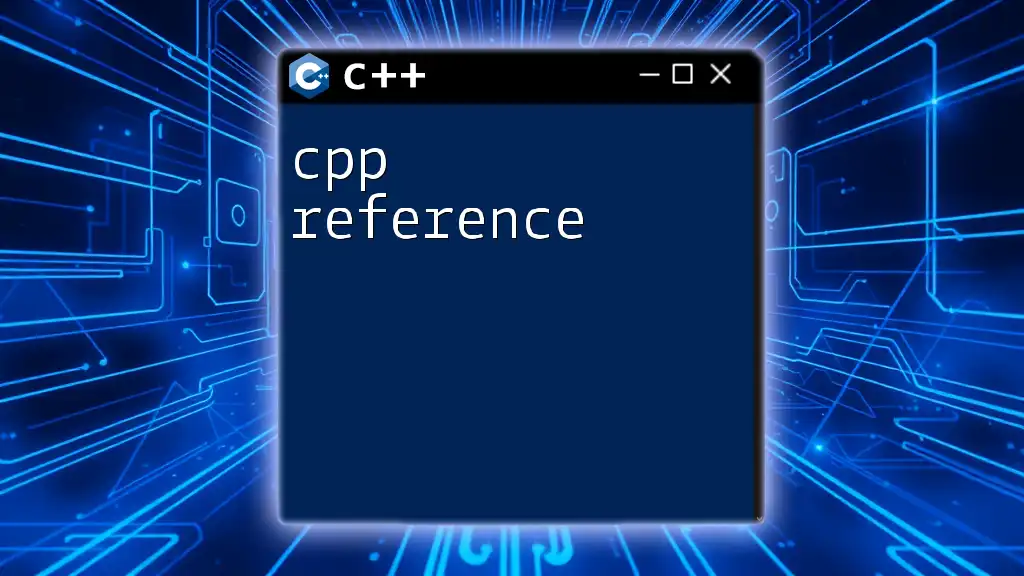
Implementing Trees in C++
Creating a Node Structure
To implement a tree in C++, you need to define a node structure that can hold values and pointers to children.
struct Node {
int data;
Node* left;
Node* right;
Node(int value) : data(value), left(nullptr), right(nullptr) {}
};
This structure provides a framework for creating nodes that compose the tree.
Basic Tree Operations
Understanding how to perform basic operations like insertion and searching is vital for working with cpp trees.
Insertion
When inserting elements, we maintain the binary search property as shown in the earlier example.
Searching
Searching is straightforward and relies on the properties of the BST.
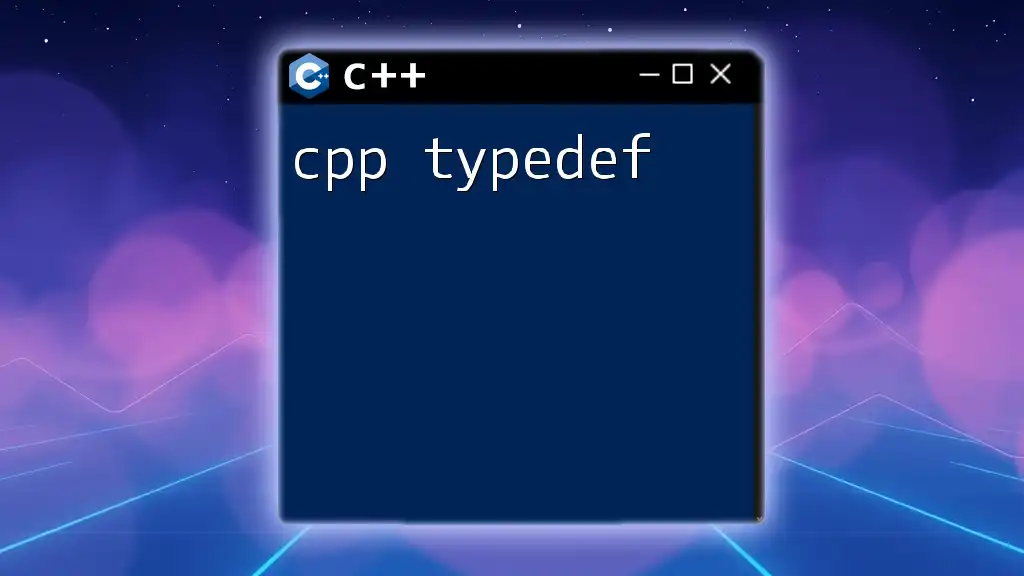
Traversing Trees
Tree traversal is a fundamental concept that allows you to visit each node in a tree systematically. There are three primary methods for traversal:
In-order Traversal
In-order traversal visits nodes in the left subtree, then the current node, and finally the right subtree. This method yields nodes in ascending order for binary search trees.
void inOrder(Node* root) {
if (root) {
inOrder(root->left);
std::cout << root->data << " ";
inOrder(root->right);
}
}
Pre-order Traversal
Pre-order traversal visits the current node before its children. This method is beneficial for creating a copy of the tree.
Post-order Traversal
Post-order traversal visits the current node after its children, making it ideal for deleting trees or calculating their size.
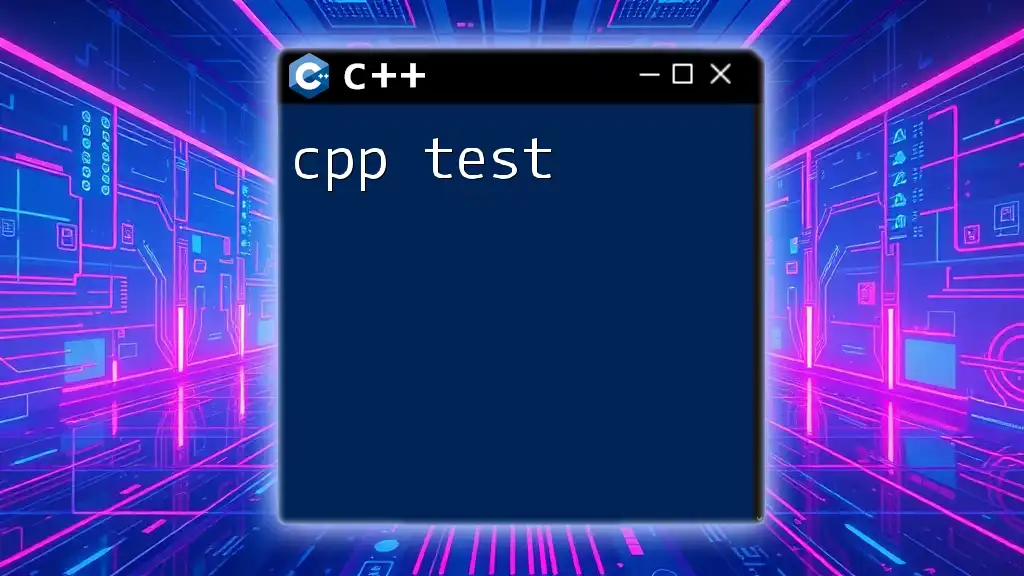
Applications of Trees
Trees have a wide range of applications across computer science and programming:
- Expression Trees: Used in compilers to represent expressions efficiently.
- Decision Trees: Commonly utilized in machine learning for classification problems.
- File Systems: Hierarchically structure files and directories, enabling quick access and manipulation.
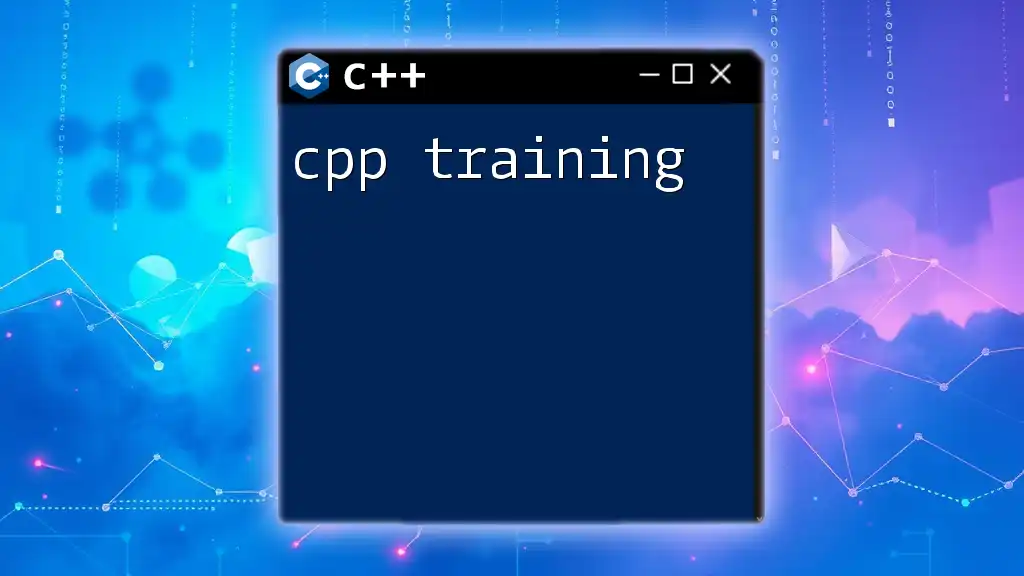
Advanced Tree Concepts
Balanced and Self-balancing Trees
Maintaining a balanced tree is crucial for performance. Self-balancing trees automatically adjust their heights during operations, ensuring operations remain efficient even as data increases.
Tree Algorithms
Mastering popular algorithms can further enhance your understanding and practical ability with cpp trees.
Lowest Common Ancestor (LCA)
Finding the Lowest Common Ancestor is a significant concept in tree algorithms, useful in various applications, such as comparing paths in hierarchies.
Tree Diameter
The diameter of a tree is the longest path between two nodes. Algorithms to calculate this often leverage depth-first search techniques for their efficiency.
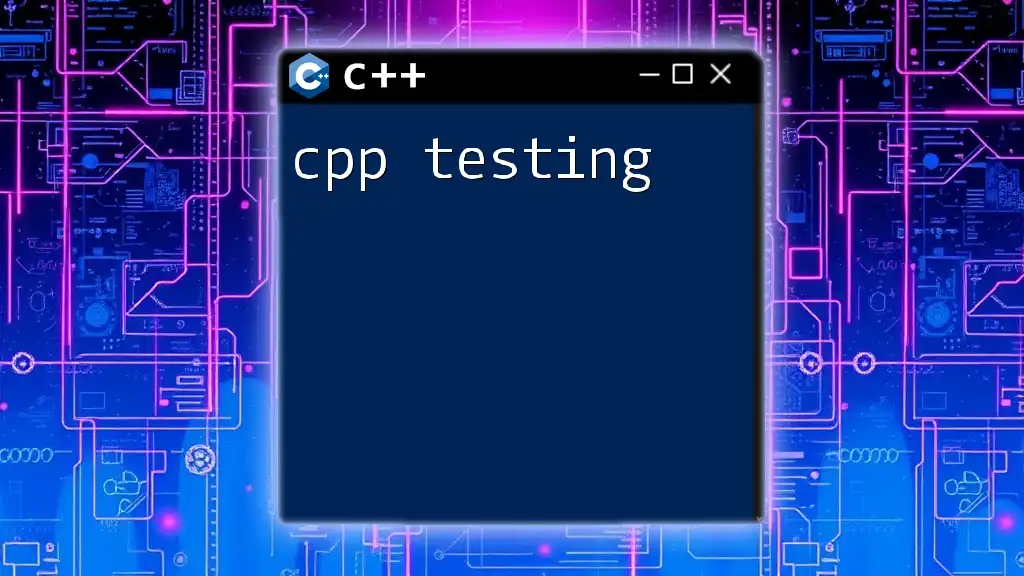
Conclusion
With this comprehensive guide to cpp trees, you should now have a solid foundation in both the theoretical aspects and practical implementation of tree data structures in C++. Understanding these concepts will greatly enhance your programming toolkit, allowing for more efficient data management and algorithm development.
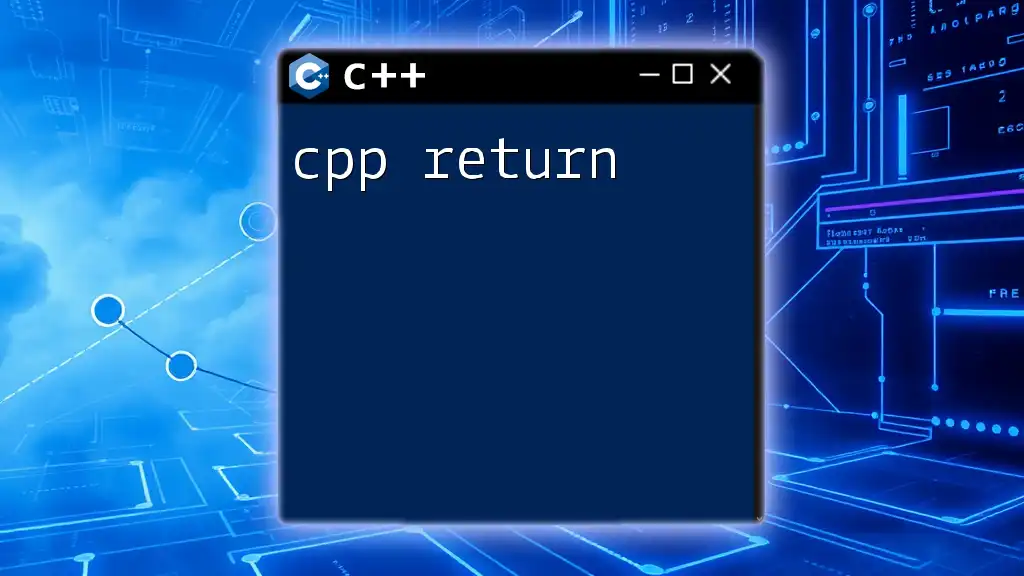
Additional Resources
To continue your journey into the world of trees in C++, consider exploring books, online courses, and official documentation that dive deeper into advanced topics and complex tree structures. This knowledge will empower you to tackle more challenging programming scenarios and solidify your skills in C++.