The `typedef` keyword in C++ is used to create an alias for an existing data type, improving code readability and maintainability.
Here's a code snippet illustrating its use:
typedef unsigned long ulong;
ulong distance = 5000;
Understanding `typedef`
What is `typedef`?
`typedef`, short for "type definition," is a keyword in C++ used to create an alias for existing data types. This feature allows developers to define new names for existing types, making the code easier to read and understand. By using `typedef`, you can provide meaningful names for complex or frequently used types, enhancing the overall readability and maintainability of the code.
Why Use `typedef`?
The primary reasons for utilizing `typedef` include:
-
Improving code readability: With `typedef`, you can replace complex data types with simpler, more intuitive names. This clarity is especially useful in large codebases or when collaborating with a team.
-
Simplifying complex types: Types such as pointers, function pointers, and arrays can be cumbersome when expressed in their original form. `typedef` makes them easier to handle.
-
Creating meaningful names for types: Rather than using generic type names, `typedef` allows you to convey the purpose or context of the type, making the code more self-explanatory.
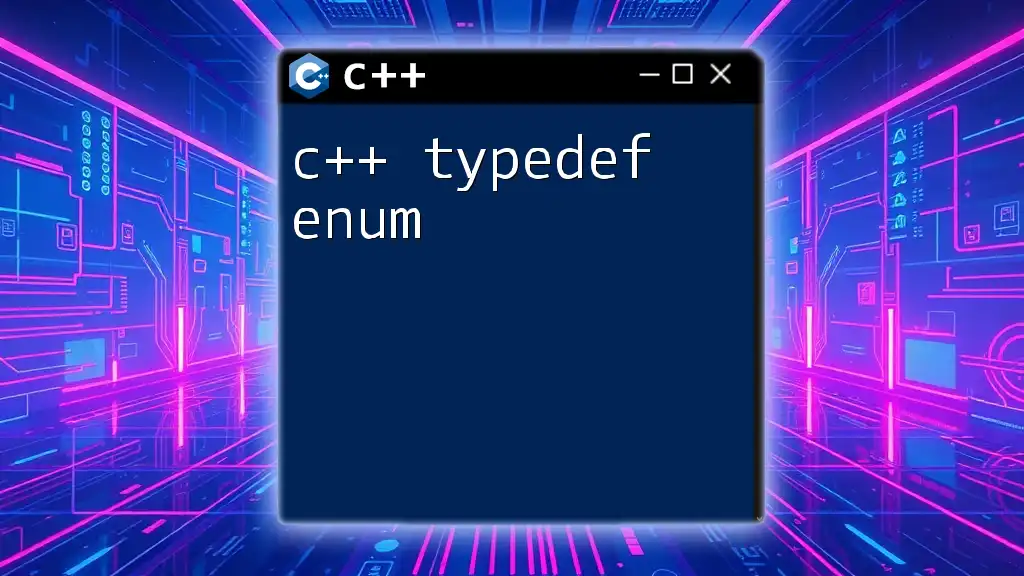
Basic Syntax of `typedef`
Syntax Explanation
The syntax for `typedef` is straightforward. It generally follows this format:
typedef existing_type new_type_name;
Here, `existing_type` is the type you want to create an alias for, and `new_type_name` is the name you will use in your code.
Example of Basic `typedef`
typedef unsigned long ulong;
In this example, we create an alias called `ulong` for the `unsigned long` type. This allows us to use `ulong` throughout our code, making it cleaner and more concise. For instance, we can declare variables as follows:
ulong value = 100000;
This approach highlights the utility of `typedef` in enhancing code readability.
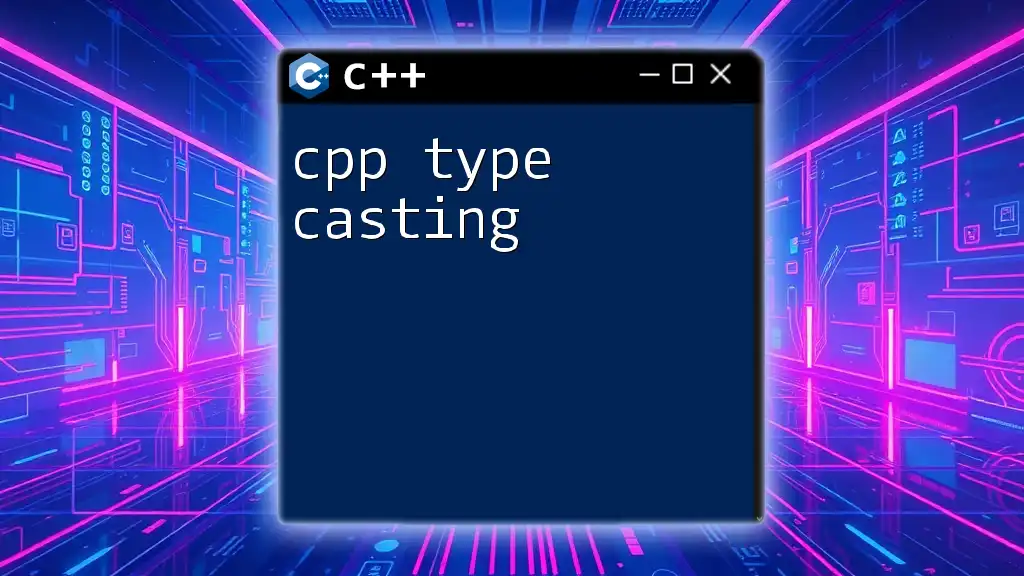
Using `typedef` with Structures
Creating Structs
Structs (short for structures) are user-defined data types in C++ that allow you to group related variables of different types under a single name. The ability to create your own data types is powerful, enabling better organization of related data.
Applying `typedef` to Structs
Consider the following example:
typedef struct {
int id;
char name[50];
} Employee;
In this code, we define a struct that represents an `Employee`, encapsulating an integer ID and a character array for the name. By using `typedef`, we can now use `Employee` as a type directly when declaring variables:
Employee emp1;
This eliminates the need for the `struct` keyword when instantiating new variables of this type and adds clarity to the code.
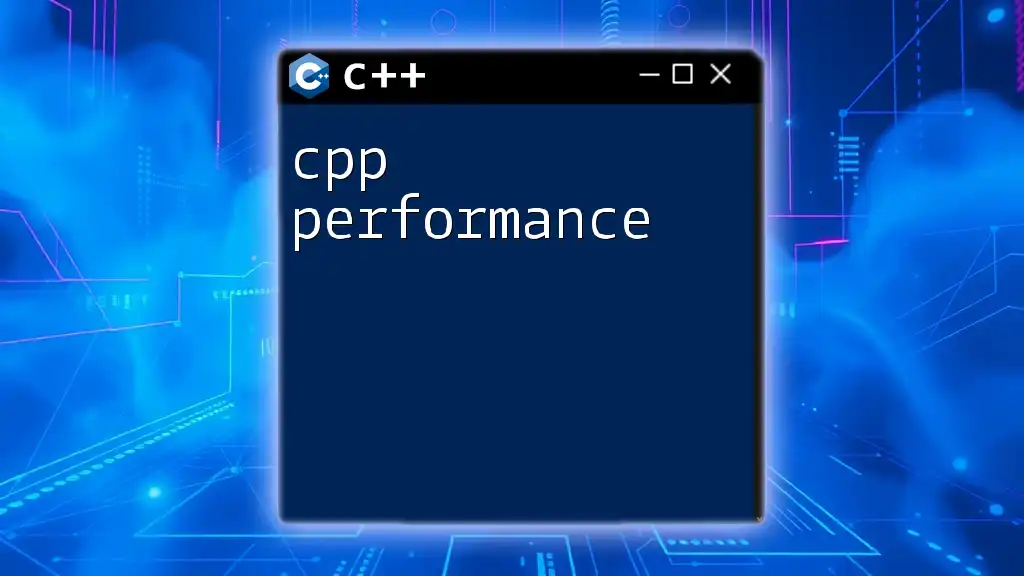
Using `typedef` with Classes
Overview of C++ Classes
While structs and classes are similar, classes offer additional features like encapsulation and inheritance. Choosing between them often depends on the context and required functionality.
Example of `typedef` with Classes
Here's how you can apply `typedef` to classes:
class Person {
public:
std::string name;
int age;
};
typedef Person Individual;
In this snippet, we define a `Person` class and create an alias called `Individual`. This allows you to write:
Individual john;
This improves code clarity and helps convey the purpose of the type being used.
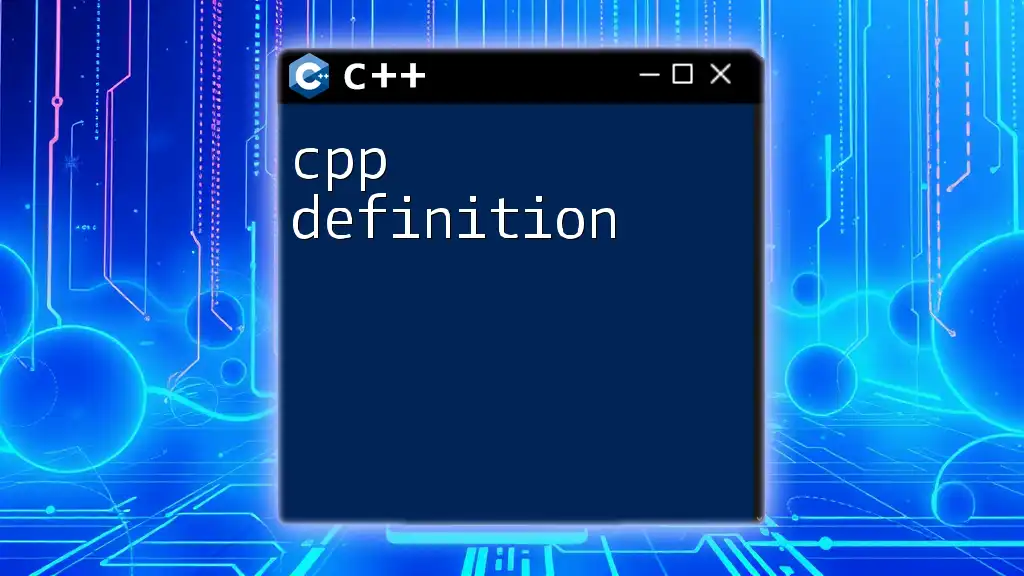
Advanced Uses of `typedef`
Complex Types
In C++, you might encounter complex data types like pointers and arrays. Using `typedef` can significantly simplify these expressions, making your code less cluttered.
Example with Pointers
Consider the following code:
typedef int* IntPtr;
Here, we create an alias `IntPtr` for a pointer to an integer. With this definition, you can declare pointers with the following clean syntax:
IntPtr ptr1, ptr2;
The clarity provided by using `IntPtr` outweighs long-hand pointer declarations, especially in situations involving multiple pointer variables.
Example with Function Pointers
Function pointers can be one of the most confusing aspects for beginners. Using `typedef`, you can define them more clearly:
typedef void (*FuncPtr)(int);
In this example, we create an alias `FuncPtr` for a pointer to a function that takes an integer as a parameter and returns nothing (void). This makes it easier to declare function pointers later in your code:
FuncPtr myFunctionPointer;
This approach significantly enhances clarity around what the pointer represents.
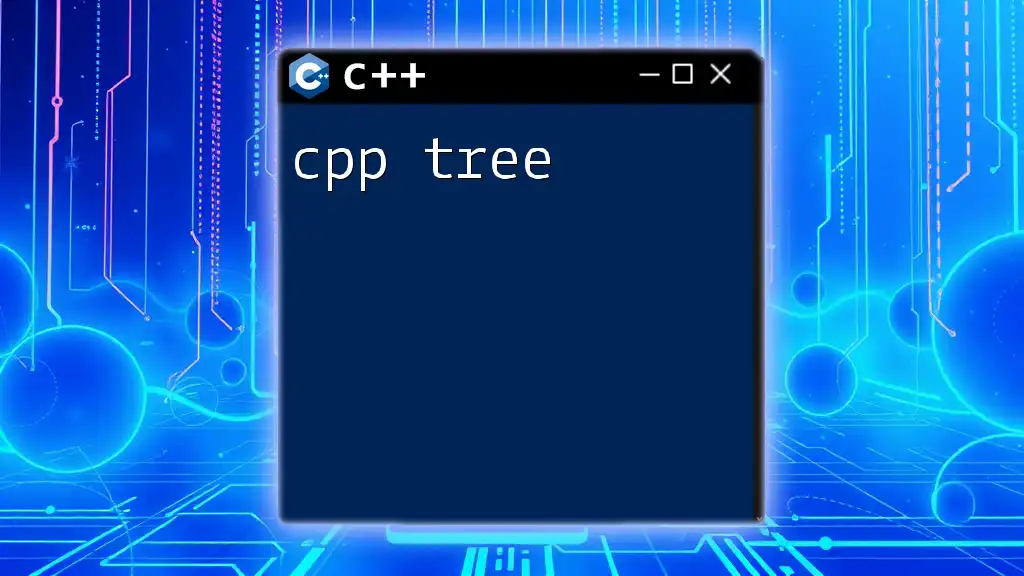
Differences Between `typedef` and `using`
Introduction to the `using` Keyword
The `using` keyword in C++ serves as an alternative to `typedef`. While both provide similar functionality—creating type aliases—`using` has syntax advantages and is generally preferred in modern C++.
Example of Using
Here's how you would use the `using` directive:
using ulong = unsigned long;
This syntax is straightforward and looks cleaner than the `typedef`. It is consistent and easy to read, making it a favored option for defining type aliases.
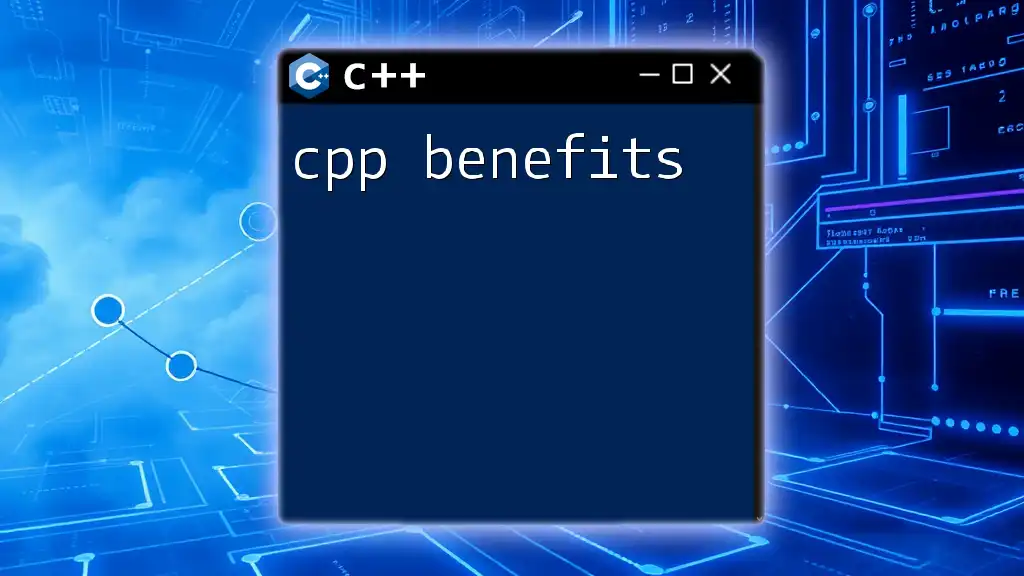
Best Practices for Using `typedef`
Naming Conventions
When creating type aliases, using meaningful names can significantly enhance code readability. Adhering to naming conventions like CamelCase or snake_case can help ensure consistency throughout your codebase.
Avoiding Common Pitfalls
Although `typedef` is powerful, it’s essential to avoid creating ambiguous or unclear type names. For example, creating a typedef like `Microwave m;` can lead to confusion, as it may not be clear whether `Microwave` refers to a type or a variable. Clear and descriptive names mitigate misunderstanding.
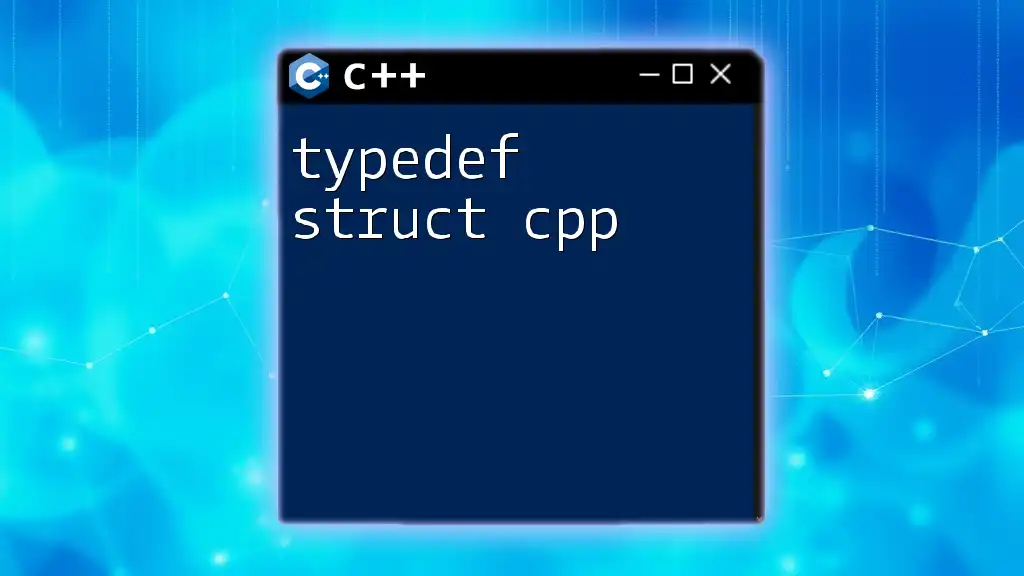
Practical Applications of `typedef`
Real-World Use Cases
In practice, `typedef` is frequently used in libraries and frameworks, especially when creating APIs that involve complex types or collections. For instance, in graphical programming, you may encounter type aliases for common structures like `Vector2` or `Color`.
Comparison of Code Snippets
typedef std::vector<std::pair<int, int>> PairVector;
versus
std::vector<std::pair<int, int>> vec;
The former enhances clarity by defining `PairVector`, making it obvious that `vec` contains a collection of pairs, while the latter can cause confusion at a glance.
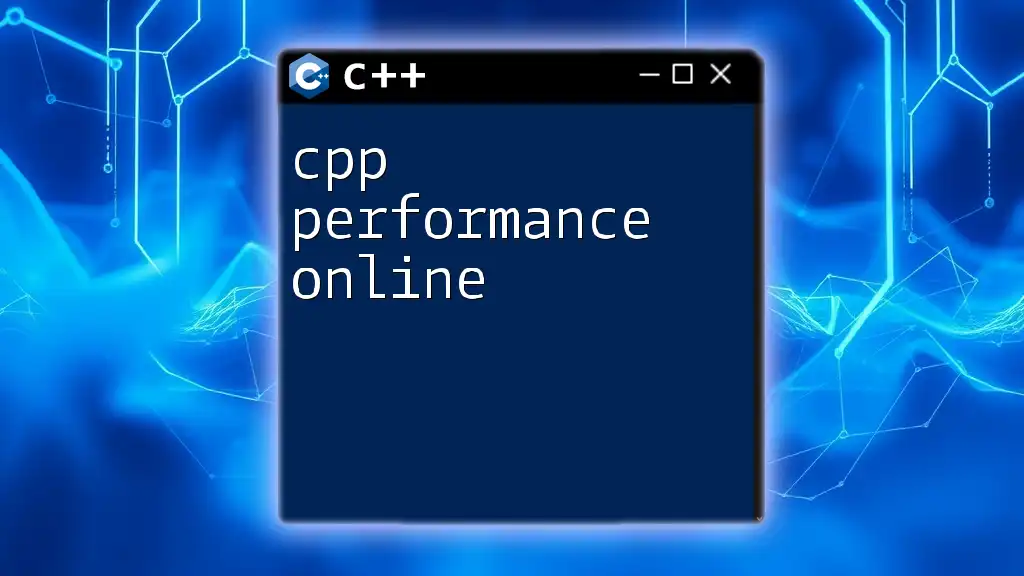
Conclusion
Summary of Key Takeaways
Throughout this guide, we explored the fundamental concepts of `cpp typedef`, including its purpose, syntax, and practical applications. We highlighted how `typedef` improves readability and simplifies complex types, enhancing overall code quality.
Encouragement to Practice
To truly grasp the utility of `typedef`, I encourage you to integrate it into your coding practice. Experiment with creating your own typedefs to simplify your projects and deepen your understanding of C++.
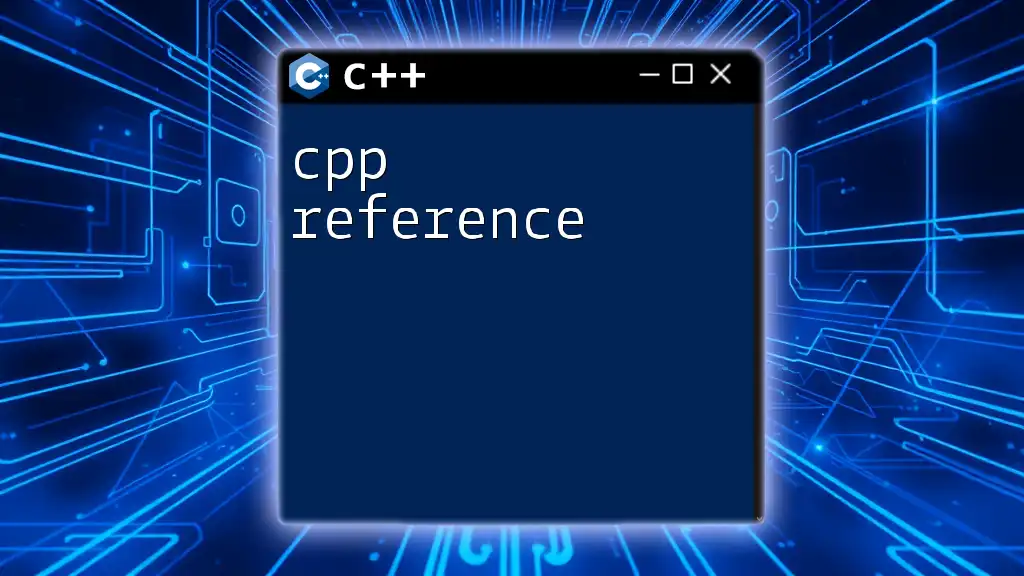
Additional Resources
Recommended Further Reading
For those looking to learn more about `typedef` and type aliases in C++, consider exploring comprehensive C++ programming books and online tutorials that cover advanced topics and best practices.
Tutorials and Practice Exercises
Engaging in exercises focusing on the creation of typedefs and their usage in various contexts can be beneficial in solidifying your skills and understanding of this important feature in C++.