C++ guidelines are a set of best practices designed to enhance code readability, maintainability, and performance, ensuring that programmers write efficient and effective C++ code.
Here's an example of a simple C++ guideline in action, demonstrating the use of proper naming conventions and clarity in variable declarations:
#include <iostream>
int main() {
int numberOfStudents = 30; // Use clear and descriptive variable names
std::cout << "Number of students: " << numberOfStudents << std::endl;
return 0;
}
Understanding C++ Guidelines
What Are C++ Guidelines?
C++ guidelines are a set of best practices designed to help developers write clean, maintainable, and efficient code. They emphasize the importance of consistency, readability, and the proper use of C++ features. By adhering to these guidelines, developers can produce software that is not only easier to understand but also more robust and less prone to errors.
C++ Core Guidelines
The C++ Core Guidelines provide a comprehensive framework aimed at promoting the use of modern C++ practices. These guidelines articulate how to leverage the strengths of C++, tackle common pitfalls, and ensure the safety and efficiency of your code. By integrating these guidelines into your workflow, you can significantly improve the overall quality of your software projects.
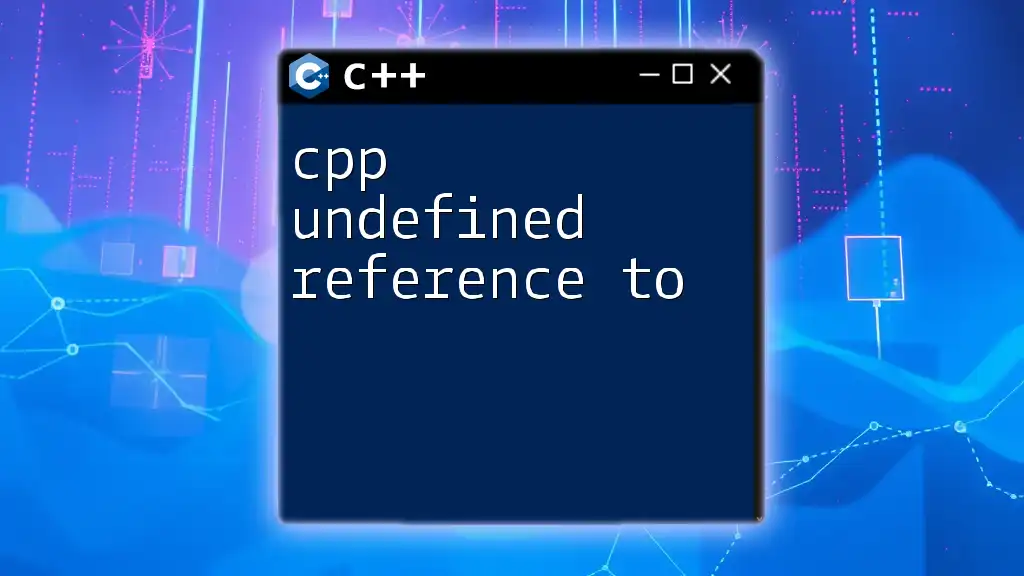
Key C++ Guidelines
Code Structure and Organization
Consistent Naming Conventions
Naming conventions play a pivotal role in ensuring that code is readable and understandable. Consistent naming helps developers quickly grasp the purpose of variables, functions, and classes. Choose a naming style and stick to it throughout your codebase, as this creates a coherent coding culture. Common styles include:
- camelCase: Often used for variable names and function names, e.g., `myVariableName`.
- snake_case: Common in C and Python, e.g., `my_variable_name`.
Example of naming conventions:
// Example of naming conventions
int myVariable; // camelCase
int my_variable; // snake_case
File Organization
Proper file organization aids in code navigation and project scalability. Structuring your files logically can save time in the long run. Consider separations such as:
- Header files (.h): Declare functions, classes, and other components.
- Source files (.cpp): Implement the functionalities declared in the header files.
- Resource directories: Organize assets such as images, stylesheets, and configuration files.
Strive for modularity by breaking down large files into smaller, focused components that can be reused across the project.
Best Practices for Using Language Features
Prefer Automatic Resource Management
Automatic resource management is a core principle in modern C++. Utilizing RAII (Resource Acquisition Is Initialization) allows resources to be tied to object lifetimes. When an object is created, it acquires resources, and when it goes out of scope, those resources are released automatically.
Example demonstrating RAII principles:
#include <memory>
void useResource() {
std::unique_ptr<int> resource = std::make_unique<int>(42);
// Resource automatically released when it goes out of scope
}
This eliminates the risk of memory leaks and simplifies resource management significantly.
Use Smart Pointers Over Raw Pointers
One of the key features introduced in C++11 is smart pointers, which help manage dynamic memory in a safer manner. Smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, provide automatic deallocation of resources, reducing the overhead of manual memory management.
std::shared_ptr<int> pointer1 = std::make_shared<int>(10);
std::unique_ptr<int> pointer2 = std::make_unique<int>(20);
Using smart pointers not only simplifies your code but also enhances safety by preventing memory leaks and dangling pointers.
Error Handling and Debugging
Proper Use of Exceptions
C++ provides a robust mechanism for error handling through exceptions. Exceptions should be used for exceptional circumstances - not for routine control flow. They enable clean separation of error handling logic from normal execution paths.
Example of correct exception handling practices:
try {
throw std::runtime_error("Error occurred");
} catch (const std::runtime_error& e) {
std::cerr << e.what() << std::endl;
}
Always ensure that exceptions are caught and handled gracefully to enhance the system's stability.
Logging vs. Output Statements
When debugging, using logging over standard output statements is crucial for maintaining clarity in code and improving performance. While output statements can clutter the code base and degrade runtime efficiency, logging frameworks provide structured and configurable ways to capture runtime events.
Consider utilizing libraries like Boost.Log or spdlog for efficient logging practices that can be easily integrated into your projects.
Code Review and Testing
Importance of Code Reviews
Code reviews are a critical part of the development process, promoting collaboration and improving code quality. Engaging multiple developers in the review process fosters a culture of knowledge sharing, encourages adherence to coding standards, and helps identify potential issues early on. Focus areas during reviews should include:
- Code correctness
- Code readability
- Appropriate use of C++ features
Encouraging an open mindset during reviews can significantly enhance team dynamics and drive quality improvements.
Unit Testing Practices
Unit testing ensures that individual components of your software perform as intended. By writing tests for your functions and classes, you can quickly identify bugs introduced during changes or additions to the codebase. Google Test is a popular framework for conducting unit tests in C++.
Example of a simple unit test using Google Test:
#include <gtest/gtest.h>
TEST(SampleTest, BasicAssertions) {
EXPECT_EQ(1, 1);
}
Incorporating unit tests into your workflow not only boosts confidence in your code changes but also serves as documentation for expected behavior.
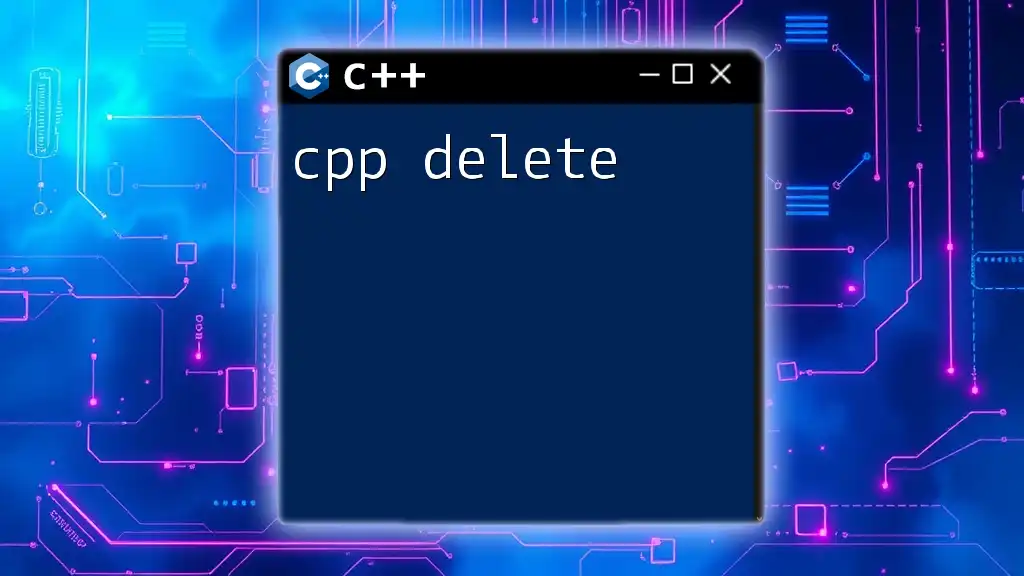
Advanced Guidelines
Performance Considerations
Optimization Techniques
While writing efficient code is important, optimization should be approached with caution. Premature optimization can lead to code that is harder to read and maintain. Instead, focus on writing clear and correct code initially, and use profiling tools to identify bottlenecks only when necessary.
Example demonstrating efficient use of STL algorithms:
// Prefer algorithms from the STL for optimization
std::vector<int> data;
std::sort(data.begin(), data.end());
Utilizing standard library algorithms ensures that you leverage optimized, tested code rather than re-implementing common algorithms.
C++11 and Beyond
Embracing New Features
C++11 introduced several new features that enhance productivity and code clarity. Features like auto, lambda expressions, and range-based for loops allow for more expressive code and reduce boilerplate.
Example using some of the new features:
#include <iostream>
#include <vector>
auto main() -> int {
auto vec = std::vector<int>{1, 2, 3, 4};
for (const auto& num : vec) {
std::cout << num << " ";
}
return 0;
}
By staying updated with the latest C++ standards, you can take advantage of advancements that simplify programming tasks and promote best practices.
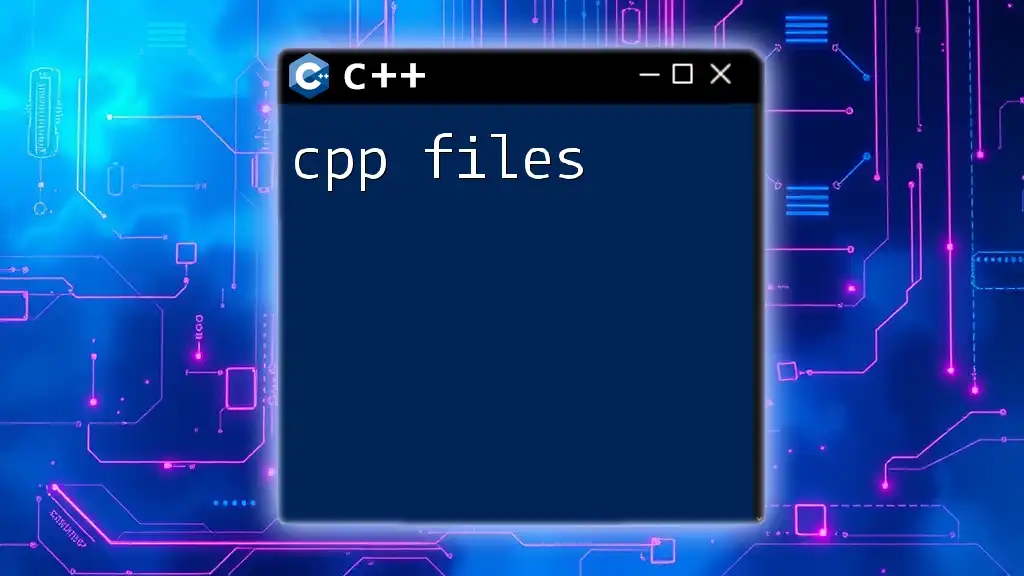
Building a Culture of Quality Code
Training and Mentorship
Fostering a culture of quality code is paramount in any development team. Ongoing training and mentorship programs help team members stay informed about best practices and evolving technologies. Encouraging participation in workshops, meetups, and online communities is vital to enhancing skills and knowledge.
Resources for Further Learning
To deepen your understanding of the principles discussed, consider exploring resources such as:
- "C++ Primer" by Stanley B. Lippman
- "Effective Modern C++" by Scott Meyers
- The official C++ Core Guidelines website for a wider array of standards and practices.
By frequently revisiting these resources, you can stay current with community best practices and continuously improve your craft.
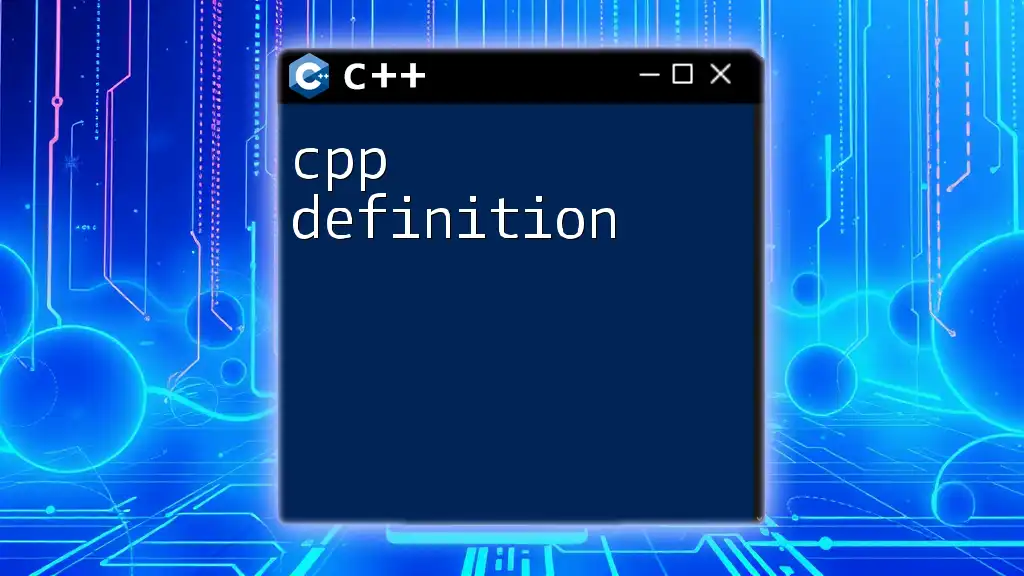
Conclusion
Embracing and implementing C++ guidelines is crucial in developing high-quality software. By prioritizing code readability, proper resource management, and systematic testing, you can significantly enhance code maintainability and efficiency. It’s time to integrate these cpp guidelines into your daily coding activities and contribute to a culture of quality within your development environment.