In C++, building refers to the process of compiling and linking your source code to produce an executable program, typically done using a command like `g++`.
g++ -o my_program my_program.cpp
Understanding C++ Building
What is C++ Building?
C++ building refers to the entire process of transforming human-readable C++ code into an executable machine code that your computer can understand and run. It involves several key stages, including compilation, linking, and finally, execution. Each stage plays a critical role in ensuring that your C++ programs perform as expected.
Why is Building Important?
Building your C++ code correctly is crucial for a number of reasons. First and foremost, optimized code leads to better performance and efficiency. Furthermore, a well-executed build process allows for easier debugging and a more streamlined development cycle. If there are issues within the code, recognizing and correcting them at the build stage saves a significant amount of time and effort later on.
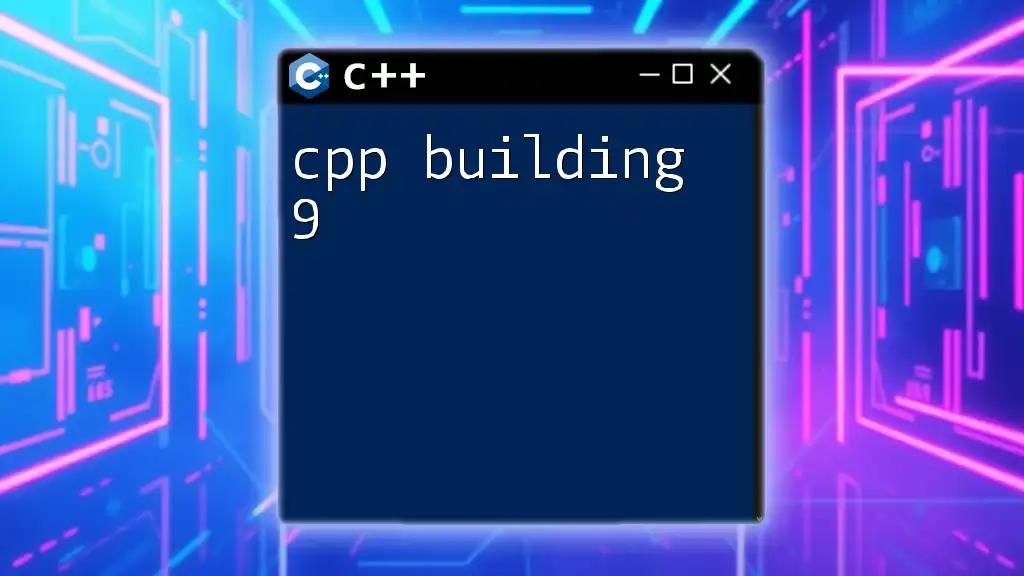
Tools for C++ Building
Compiler Options
Various compilers are available for C++, each with its own features and strengths. The three most notable compilers include:
- GCC (GNU Compiler Collection): A widely-used compiler known for its high performance and compatibility with various systems.
- Clang: Offers fast compilation and excellent diagnostic messages, making it a favorite among developers focused on code quality.
- MSVC (Microsoft Visual C++): Ideal for developers working in a Windows environment, featuring an integrated development environment (IDE).
Each compiler comes with unique flags and options that can enhance the build process and optimize results.
Build Systems
A build system automates the process of compiling and linking code files. This is particularly helpful in larger projects. Some common build systems include:
-
Make: A tool that automatically builds executable programs and libraries from source code. It uses a simple syntax for defining build instructions.
-
CMake: A more powerful cross-platform tool that generates build files for different platforms, making it ideal for more complex projects.
-
Ninja: A small, high-speed build system that focuses on speed and efficiency, often employed in large development environments.
These tools significantly streamline the process of C++ building, helping to eliminate human error and speed up project timelines.
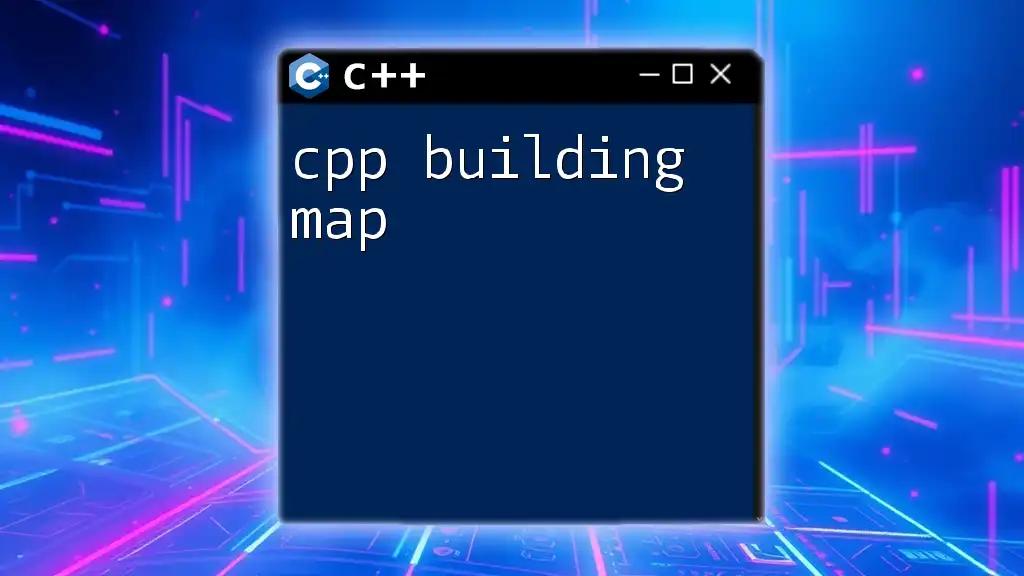
The C++ Build Process
Step 1: Writing the Code
Writing clean and efficient code is the foundational step in the building process. Following best practices ensures that your code is easier to read and maintain. Here’s an example of a basic C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program outputs "Hello, World!" to the console and serves as a simple introduction to the C++ syntax.
Step 2: Compiling the Code
What is Compilation?
Compilation is the process of translating the human-readable source code into machine code. During compilation, the preprocessor also processes directives and prepares the code for the next step.
Compilation Commands
To compile a C++ program, you’ll commonly use commands tailored to your specific compiler. Here’s how to compile a program using GCC and Clang:
Using GCC:
g++ main.cpp -o my_program
Using Clang:
clang++ main.cpp -o my_program
Understanding Compile Errors
As you compile your code, you may encounter errors. Common compile-time errors include:
- Syntax errors: Often due to typos or wrong command structures.
- Type errors: Occur when there is a type mismatch in variables.
- Missing headers: Result from not including necessary libraries or headers.
Addressing these errors promptly improves your code's reliability and prepares you for the linking stage.
Step 3: Linking the Code
What is Linking?
Linking is the process that combines various object files and libraries to create the final executable program. This is a crucial part of the build process, as it resolves dependencies and ensures that all components of your program work seamlessly together.
Static vs Dynamic Linking
There are two primary types of linking:
-
Static linking: Combines all necessary code into a single executable at compile time. Pros: Easier to distribute; Cons: Larger executable size.
-
Dynamic linking: Links libraries at run-time, resulting in smaller executable sizes. Pros: More efficient; Cons: Requires the presence of the library on the target machine.
Understanding when to use each method can significantly impact your program's performance and ease of distribution.
Linking Commands
You can link your compiled object files into an executable through commands like:
g++ -o my_program main.o other_file.o
This command merges the object files into a single executable named `my_program`.
Step 4: Running Your Program
After building your code, you can run your program using a simple command in the terminal:
./my_program
This step executes the program, allowing you to see the output or behavior of your code. Successful execution indicates that the building process was successful.
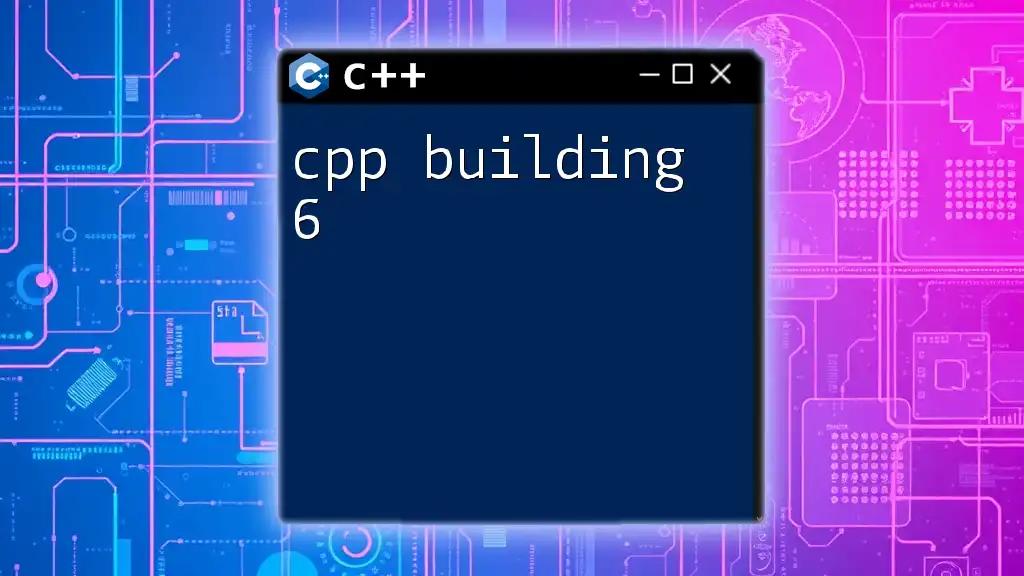
Advanced C++ Building Concepts
Optimization Techniques
Optimizing your code not only improves performance but can also reduce resource consumption. Here are some effective optimization techniques:
- Utilize compiler optimization flags such as `-O1`, `-O2`, and `-O3` to instruct the compiler to enhance code performance.
- Implement inline functions to reduce function call overhead.
- Use loop unrolling, which increases a program's execution speed by reducing the overhead of loop control.
Adopting these strategies in your build process can lead to significant improvements.
Debugging and Testing Your Build
Debugging is a crucial aspect of the C++ building process. Proper debugging tools and techniques can help identify issues before they escalate:
-
Use GDB (GNU Debugger) to step through your code and examine variables.
-
Employ Valgrind for memory debugging, ensuring that your program does not encounter memory leaks.
Example command for debugging with GDB:
gdb ./my_program
Cross-Platform Building
Cross-platform building ensures that your C++ code can run on various operating systems. This can be challenging due to differences in environment and dependencies. Tools like CMake allow you to write your build instructions once and generate files for multiple platforms, simplifying this process.
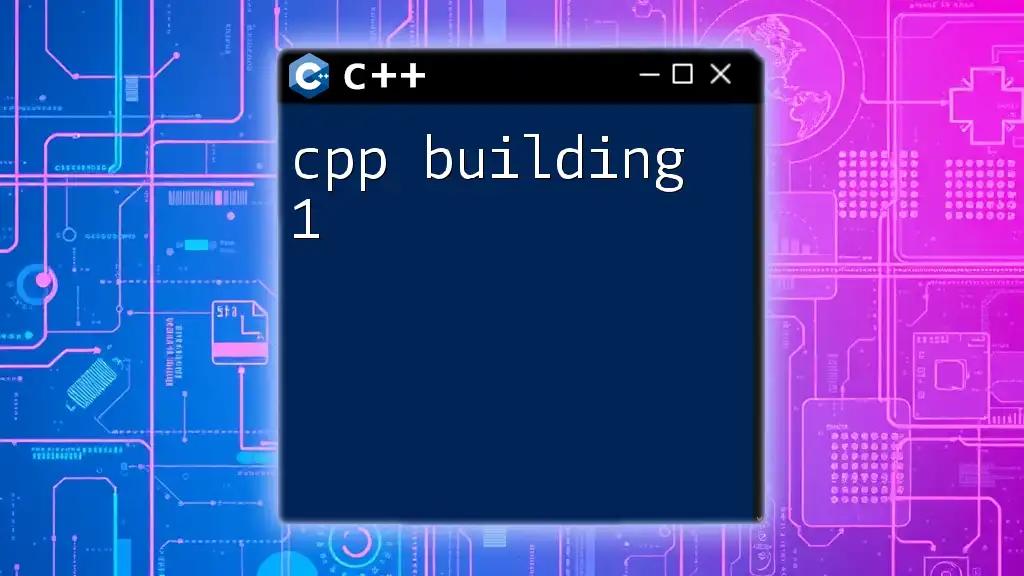
Best Practices for C++ Building
Following best practices is essential for efficient C++ building. Some key tips include:
- Use makefiles to automate your build process, keeping everything organized and manageable.
- Implement a version control system like Git to track changes and collaborate effectively.
Organizing your project directory with a clear structure also aids in keeping your build process efficient and predictable.
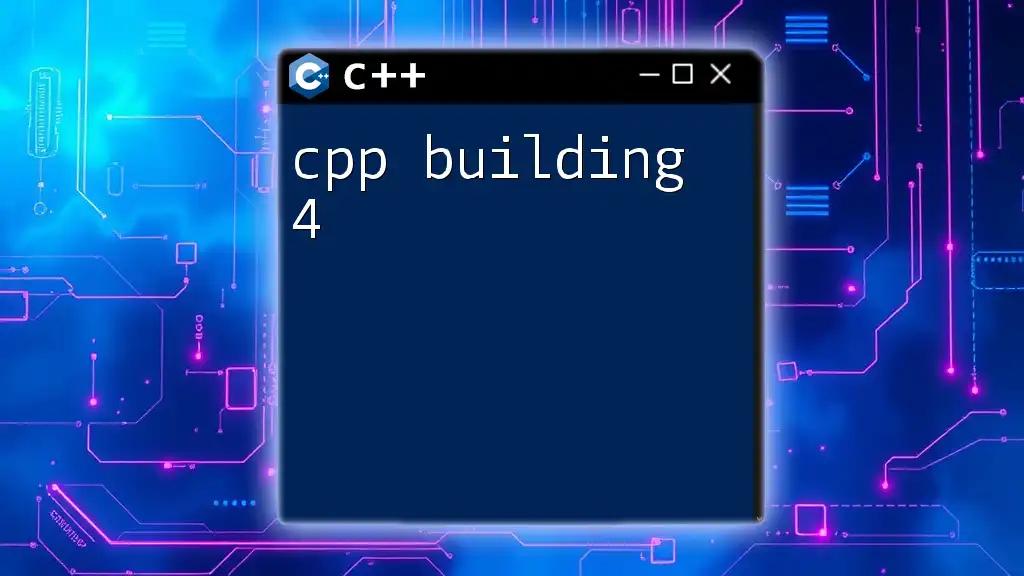
Conclusion
Mastering the process of C++ building is vital for any developer seeking to improve their skills. By understanding the components of building and implementing best practices, you can ensure your C++ projects are optimized, effective, and ready for deployment. Remember, the journey toward becoming proficient in C++ building is ongoing, and using the tools and techniques discussed will pave the way for your success.
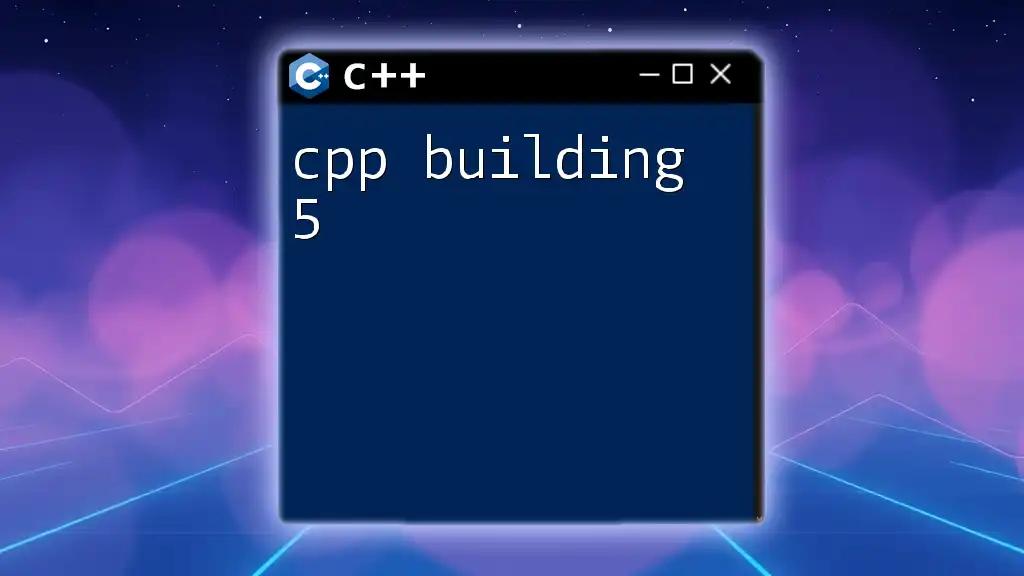
Additional Resources
To further enrich your knowledge of C++ building, consider exploring recommended books, online tutorials, and engaging with C++ communities where you can seek advice and collaborate with fellow developers.