In C++, building 9 can refer to compiling and executing a simple program that demonstrates the use of loops, which can be achieved with the following code snippet:
#include <iostream>
int main() {
for (int i = 1; i <= 9; i++) {
std::cout << "Building " << i << std::endl;
}
return 0;
}
Understanding the C++ Build Environment
Essential Components of C++ Building
Compiler
The compiler plays a crucial role in the cpp building process. It is responsible for translating your high-level C++ code into machine code that the computer can understand. Some of the most popular compilers include GCC (GNU Compiler Collection), Clang, and MSVC (Microsoft Visual C++). Each compiler has its own set of features and optimizations, so choose one that aligns with your development goals.
Build Tools
CPP building often involves various tools that help streamline the build process. Make, CMake, and Ninja are some of the well-known build tools. These tools handle tasks like dependency management, compiling files, and generating makefiles, which results in faster and more efficient builds.
Linker
A linker serves as the bridge that combines multiple object files into a single executable. Understanding how the linker works is essential for cpp building, as it connects various modules and resolves external references. This process culminates in a final executable that can be run on the target machine.
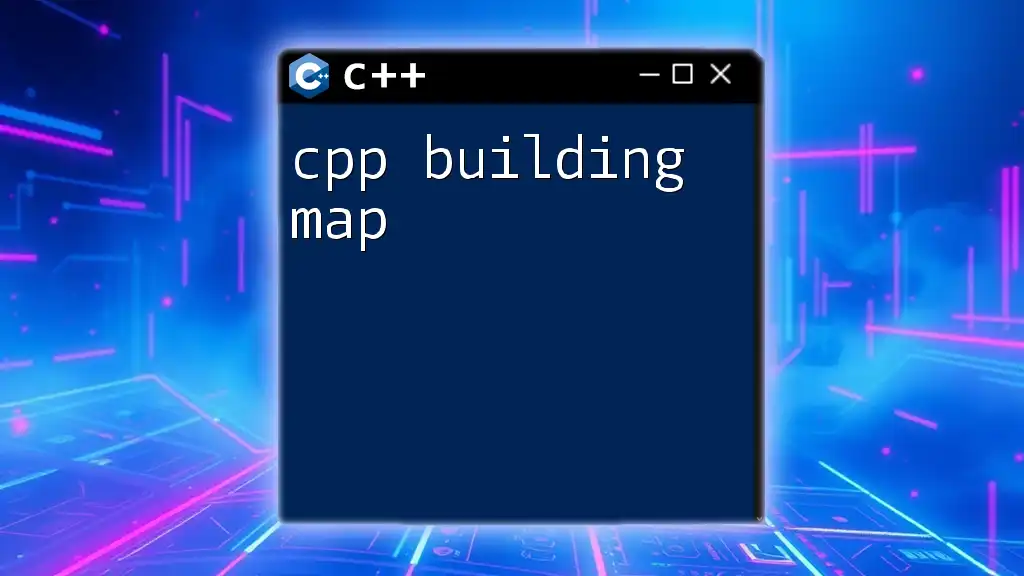
The Build Process in C++
Stages of the Build Process
Preprocessing
During the preprocessing stage, the compiler processes directives that begin with `#`. For example, the directive `#include <iostream>` tells the compiler to include the standard input-output stream library. This stage helps prepare the code for the subsequent compilation phase.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Compilation
After preprocessing, the compilation step converts the preprocessed code into object code. This is where syntax errors will surface. A successful compilation generates an object file (e.g., `.o` or `.obj`) which contains machine code that is not yet executable.
g++ -c myfile.cpp
The `-c` flag tells the compiler to compile the source file into an object file without linking.
Linking
In the linking phase, the object files are connected to form a complete executable. This is where the linker shines, merging various modules and resolving any external symbols.
g++ -o myprogram myfile.o
In this command, `-o` specifies the name of the output executable.
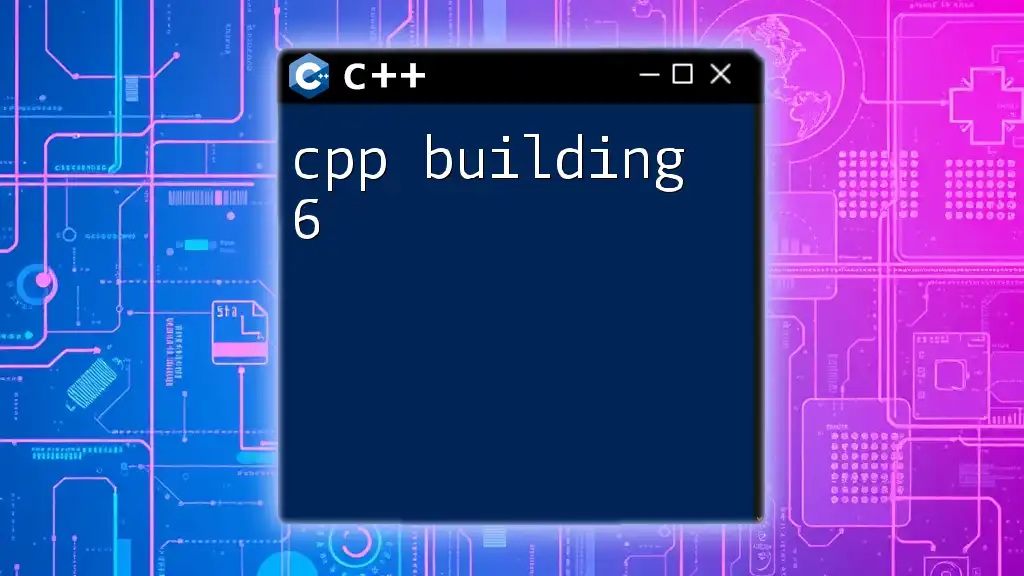
CMake: The Swiss Army Knife of C++ Builds
Why Choose CMake?
CMake stands out for its versatility and cross-platform capabilities. Unlike other make systems, CMake generates standard build files or project files for various platforms, allowing developers to write a single configuration that works across different systems.
Basic CMake Commands
Here are some essential CMake commands:
- `cmake_minimum_required(VERSION 3.10)` sets the minimum version of CMake required.
- `project(MyProject)` defines the name of the project.
- `add_executable(myprogram main.cpp)` declares the executable and the source files it uses.
A simple CMake configuration file (CMakeLists.txt) may look like this:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
add_executable(myprogram main.cpp)
Example Project Structure
A typical CMake project structure may resemble:
/MyProject
│
├── CMakeLists.txt
├── src
│ ├── main.cpp
│ └── other_file.cpp
└── include
└── my_header.h
In this structure, the `src` folder contains source files, while the `include` folder contains header files.
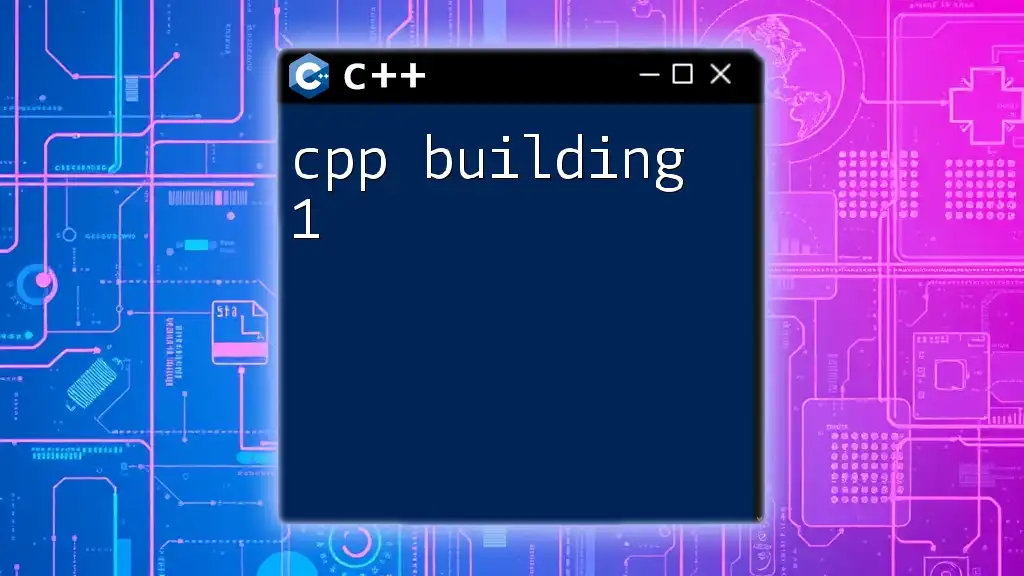
Building and Running a C++ Project
Using Command-Line Tools
Compiling with g++
For swift compilations, the `g++` compiler is one of the most convenient tools to use. A command to compile a simple C++ file could look like this:
g++ myfile.cpp -o myprogram
This command compiles `myfile.cpp` and creates an executable named `myprogram`.
Running the Executable
To run the executable, use the following command in the terminal:
./myprogram
You should see the output from your program, confirming that it ran successfully.
Automating Builds with Makefiles
Makefiles automate the build process significantly. A basic Makefile might look like this:
CC = g++
CFLAGS = -Wall -g
SOURCES = main.cpp
EXECUTABLE = myprogram
all: $(EXECUTABLE)
$(EXECUTABLE): $(SOURCES)
$(CC) $(CFLAGS) -o $@ $^
This Makefile specifies how to compile and link the program. The `all` target builds the executable.
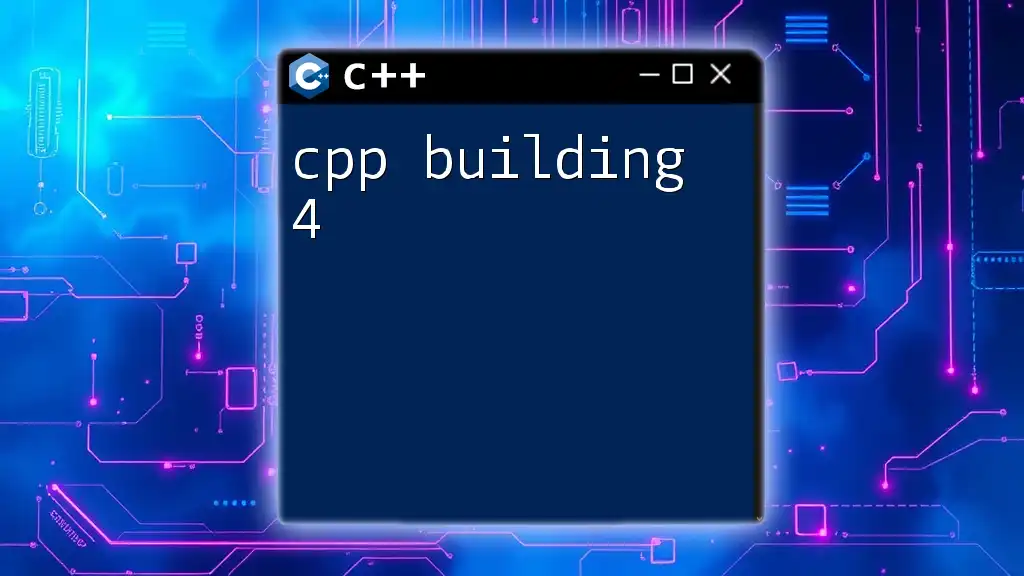
Debugging Build Issues
Common Build Errors in C++
Before diving into debugging, it's important to be aware of the common build errors that may arise:
Syntax Errors
Syntax errors occur when the code does not adhere to C++ rules. These can often be fixed by scrutinizing the code and checking for missing semicolons or mismatched parentheses.
Linker Errors
Linker errors are frequently due to missing implementations of functions or unresolved external symbols. Checking that all object files are properly linked can resolve these issues.
Runtime Errors
Runtime errors usually surface after a successful build. They can arise from issues like invalid memory access. Utilizing tools like GDB for debugging can help pinpoint these issues effectively.
Using Debugging Tools
Debugging tools such as GDB (GNU Debugger) and Valgrind are essential in identifying and fixing build-related issues. They allow you to step through code line by line, inspect variables, and identify precisely where problems arise.
To set up debugging with GDB and CMake, you can add the following line to your CMakeLists.txt to ensure debugging information is included:
set(CMAKE_BUILD_TYPE Debug)
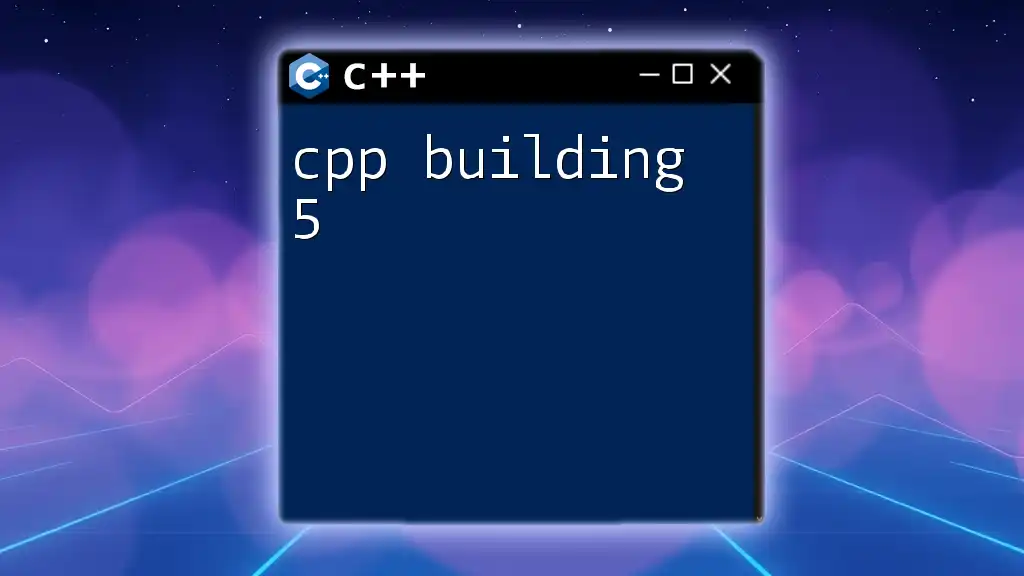
Best Practices for C++ Building
Organizing Your Code for Better Builds
To facilitate efficient building, modular design is essential. Structuring code into logical modules improves readability, maintainability, and enables easier debugging. Aim to separate concerns by utilizing folders for source files, headers, and resources.
Version Control Integration
Implementing version control systems like Git can enhance collaboration and maintain code history. Some git commands that are useful for managing your build workflow include:
- `git init` to initialize a new repository.
- `git add .` to stage all changes.
- `git commit -m "message"` to save your progress.
By incorporating version control, you can easily track changes and roll back to previous states if necessary.
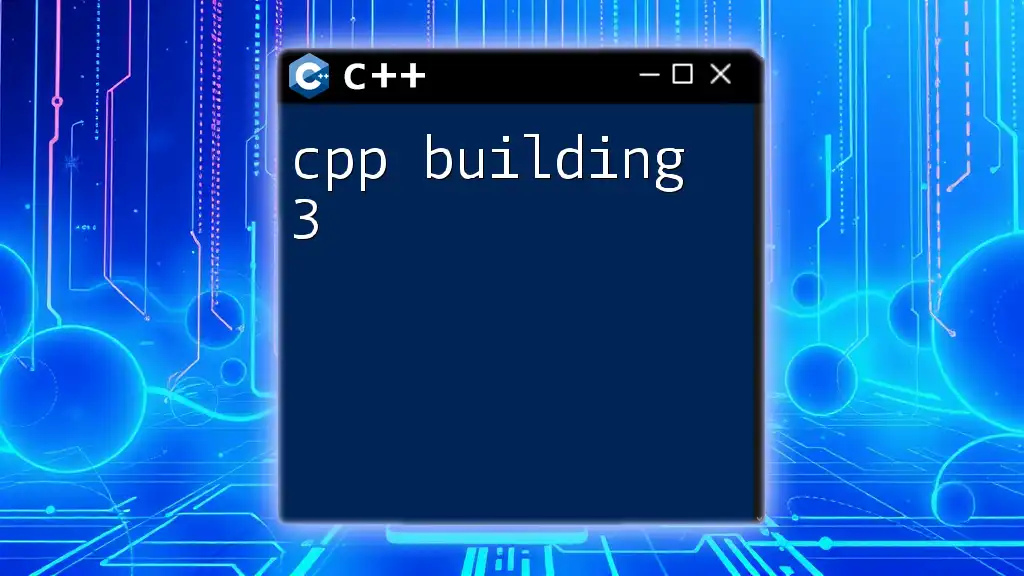
Conclusion
CPP Building 9 encompasses the entire process of compiling, linking, and executing C++ code effectively. With a comprehensive understanding of the build environment, tools like CMake, and best practices, you are well-equipped to develop robust C++ applications. Employ these strategies in your projects and foster a deeper understanding of how to streamline your cpp building workflow for optimal results.
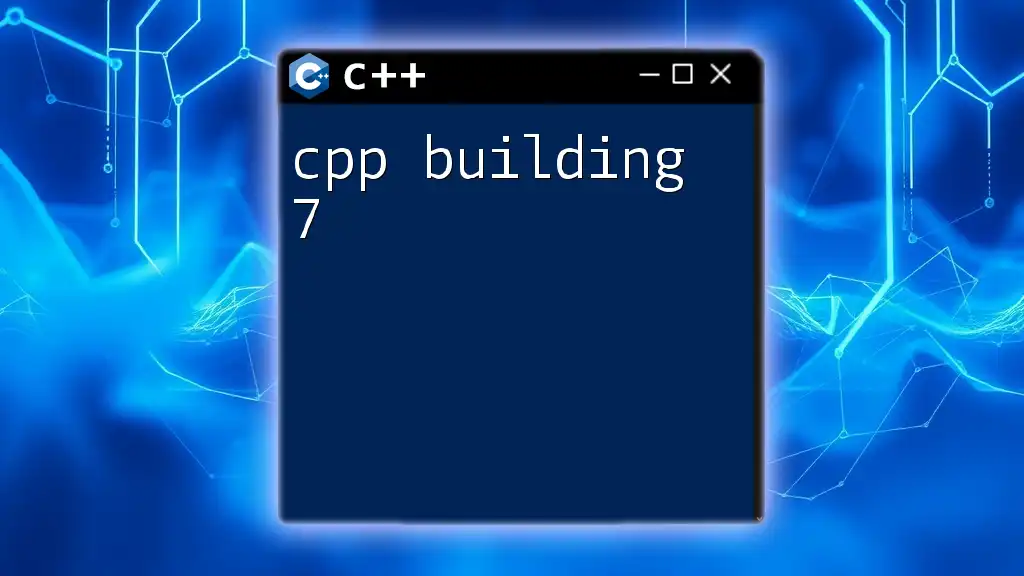
Additional Resources
To deepen your knowledge, consider exploring the following:
- Official Documentation: GCC, Clang, CMake documentation pages
- Books: "C++ Primer" and "Effective Modern C++"
- Online Courses: Platforms like Coursera and Udemy offer specialized C++ courses tailored to building and project management.
Armed with these insights, you're ready to embark on your cpp building journey!