C++ Builder is an integrated development environment (IDE) for rapid application development in C++ that simplifies the creation of Windows applications through visual design tools and powerful libraries.
Here's an example of a simple C++ program that creates a window using C++ Builder:
#include <vcl.h>
#pragma hdrstop
int WINAPI _tWinMain(HINSTANCE, HINSTANCE, LPTSTR, int) {
Application->Initialize();
Application->CreateForm(__classid(TForm1), &Form1);
Application->Run();
return 0;
}
What is C++ Builder?
C++ Builder is a powerful Integrated Development Environment (IDE) that enables developers to create native Windows applications using the C++ programming language. It provides a comprehensive suite of tools designed to streamline the development process and enhance productivity. With its visual form designer, component libraries, and extensive debugging capabilities, C++ Builder simplifies complex tasks, making it suitable for both novice and experienced developers.
Historical Background
C++ Builder was first introduced by Borland in the mid-1990s as a rapid application development (RAD) tool. Over the years, it has evolved significantly, integrating modern features while maintaining its core functionality. Today, it remains relevant for developers looking to leverage the efficiency of C++ in a robust development environment.
Target Audience
C++ Builder caters to a diverse range of developers, including:
- Software Engineers: Those looking for a comprehensive environment to develop complex applications.
- Game Developers: Utilizing its powerful libraries for game logic.
- Business Application Developers: Ideal for creating database-driven applications.
- Hobbyists and learners: Individuals wanting to grasp C++ without facing steep learning curves.

Key Features of C++ Builder
Integrated Development Environment (IDE)
The C++ Builder IDE boasts an intuitive UI that fosters easy navigation. Features include code editors with syntax highlighting, property inspectors for component management, and integrated debugging tools that enhance the programming experience.
Visual Component Library (VCL)
At the heart of C++ Builder lies the Visual Component Library (VCL). This library provides pre-built components for GUI design, allowing developers to drag and drop elements with ease. VCL is particularly advantageous for Windows development; however, developers have the option to use the FireMonkey framework for cross-platform applications.
Cross-platform Development
One of the most compelling features of C++ Builder is its ability to create applications that run seamlessly across multiple platforms, including Windows, macOS, and mobile devices. This capability significantly reduces development time, allowing for the efficient use of resources.

Getting Started with C++ Builder
Installation and Setup
To begin using C++ Builder, follow these steps:
- Download the Installer: Visit the official website to acquire the latest version of C++ Builder.
- Run the Installation: Follow the on-screen instructions to install the software.
- Configure Settings: Set up your development environment according to your preferences, including choosing the right components and tools.
System Requirements: Ensure your computer meets the minimum system requirements, including CPU, RAM, and disk space.
Creating Your First Project
When you launch C++ Builder, select 'New Project' from the menu. You can choose from various templates based on your requirements.
- Setting Project Options: Name your project and configure the essential settings such as Target Path and Output Directory.
- Understanding Project Structure: Familiarize yourself with the components of your project, including .cpp files for your code, .h files for declarations, and .dfm files for form design.
Understanding the IDE Layout
The C++ Builder IDE features several panels:
- Tool Palette: A library of components you can drag onto your forms.
- Form Designer: Where you visually construct your application’s UI.
- Code Editor: A space to write and edit your program's code.
- Object Inspector: Allows you to edit properties of selected components easily.
Through customizing these panels, you can enhance your workflow.

Building Applications: A Practical Approach
Creating a Simple GUI Application
To illustrate the development process, let’s create a simple to-do list application.
-
Design Your UI: Use the Form Designer to add components like `TListBox` and `TButton`.
-
Example Project Structure:
- ListBox where tasks will be displayed.
- EditBox for inputting new tasks.
- Button to add tasks to the ListBox.
Code Snippet for Adding a Task:
void __fastcall TForm1::AddButtonClick(TObject *Sender)
{
String task = TaskEdit->Text;
if (task.Length() > 0)
{
ListBox1->Items->Add(task);
TaskEdit->Clear();
}
}
Adding Functionality through C++ Code
To add functionality, connect UI elements to C++ code. The example above demonstrates a button click event that adds text entered in an edit box to a list box.
- Event Handling: An understanding of C++ syntax is crucial for linking UI actions to code.
Working with Databases
C++ Builder simplifies database interactions. You may utilize components like `TSQLQuery` for SQL-based operations.
Example: Connecting to a Database:
- Drag Database Components from the Tool Palette.
- Code Snippet for Database Connection:
void __fastcall TForm1::ConnectToDatabase()
{
SQLConnection1->Connected = true;
SQLQuery1->SQL->Text = "SELECT * FROM tasks";
SQLQuery1->Open();
}
This snippet connects to a database and fetches data into your application.
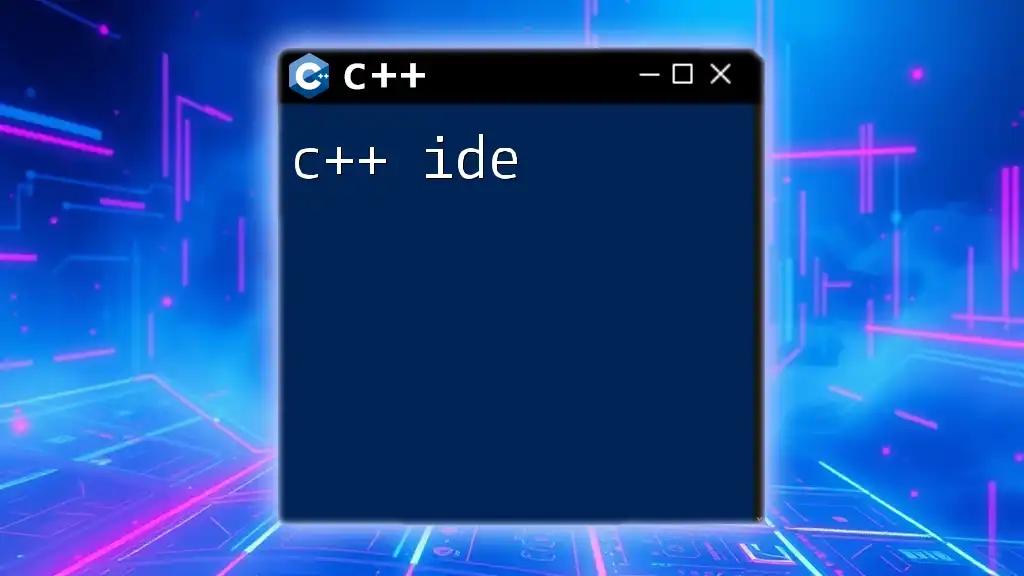
Advanced Features of C++ Builder
Multithreading Support
Modern applications often require multitasking capabilities. C++ Builder supports multithreading, allowing tasks to run concurrently without freezing the UI. Utilize `TThread` to create and manage threads efficiently.
Error Handling and Debugging Tools
C++ Builder provides robust debugging features, such as breakpoints and watch windows. Employ these tools to identify and resolve issues quickly. Implement try-catch blocks in your code to gracefully handle exceptions and errors.
Integrating with Third-Party Libraries
To enhance functionality, C++ Builder allows integration with external libraries. For instance, using a graphics library can significantly improve application visuals.
Example: Integrating a library could involve linking necessary headers and configuring project settings accordingly.
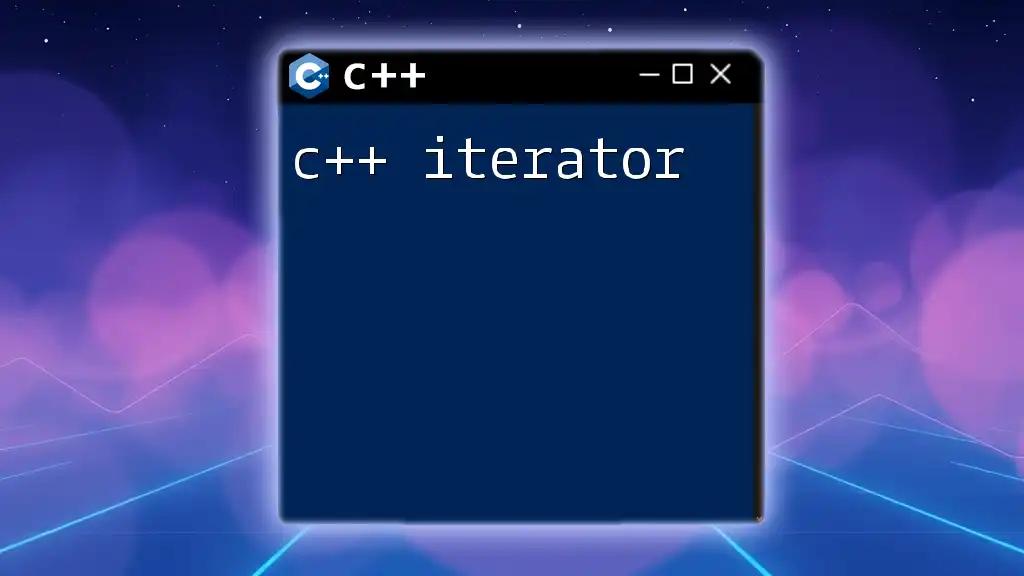
Deployment and Distribution
Preparing Your Application for Deployment
Before distributing your application, consider these essential steps:
- Testing: Thoroughly test your application on different machines.
- Building the Project: Generate the executable via the IDE.
Cross-Platform Deployment Options
With C++ Builder, deploying applications on platforms like iOS or Android is streamlined. Modify project settings and utilize specific tools designed for each platform while maintaining a single codebase.
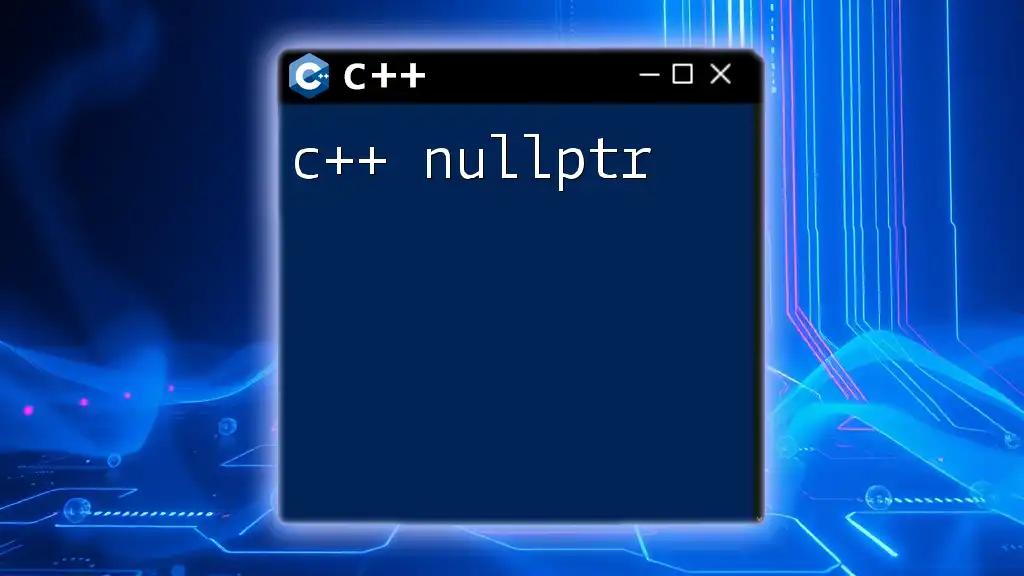
C++ Builder Best Practices
Coding Standards and Conventions
Maintaining clean, organized code is critical. Follow best practices such as:
- Consistent naming conventions.
- Proper commenting for clarity.
Performance Optimization Techniques
To ensure applications run smoothly, consider:
- Minimizing resource usage through efficient algorithms and data structures.
- Profiling your application to identify bottlenecks.
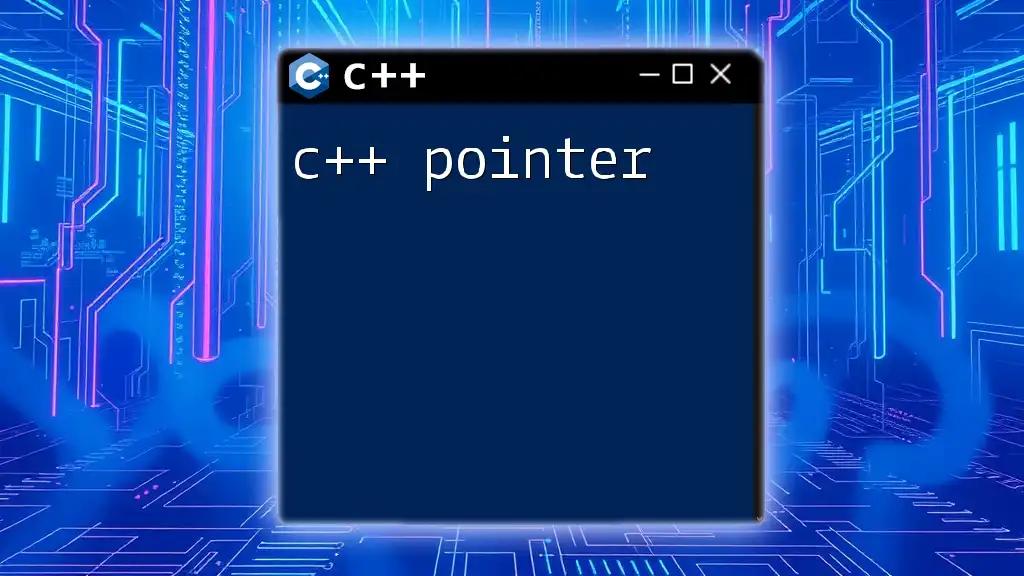
Community and Resources
Join the C++ Builder Community
Engaging with other developers provides invaluable support and knowledge. Participate in forums, attend local meetups, and join online communities to share experiences and ideas.
Learning Resources
For further education, explore:
- Books: Seek out comprehensive texts on C++ programming and C++ Builder.
- Online Courses: Numerous platforms offer courses ranging from beginner to advanced levels.
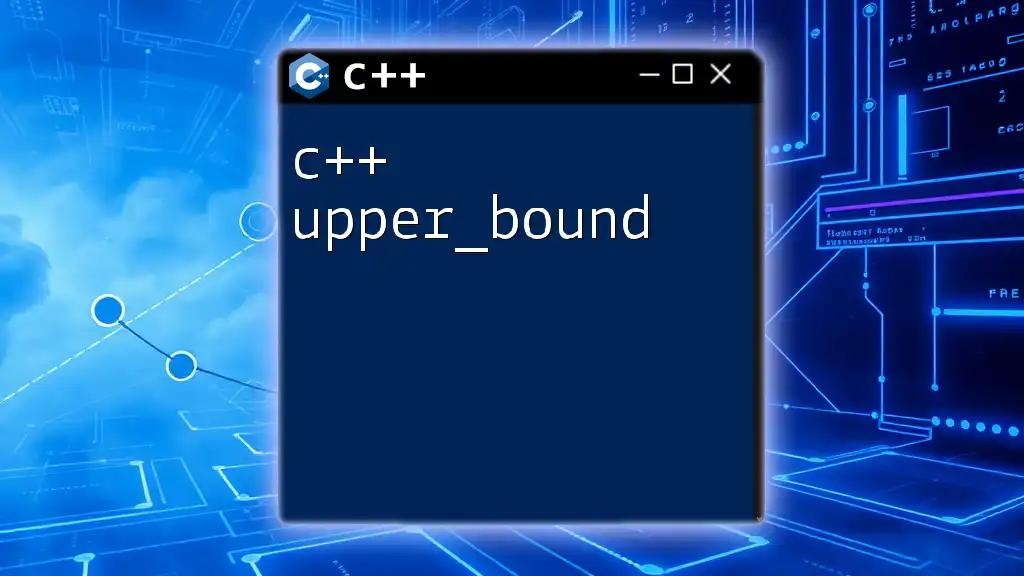
Conclusion
C++ Builder is a versatile tool that offers developers a rich environment to create native applications swiftly and efficiently. Whether you are building a simple app or complex software systems, mastering C++ Builder can significantly enhance your productivity and application quality.
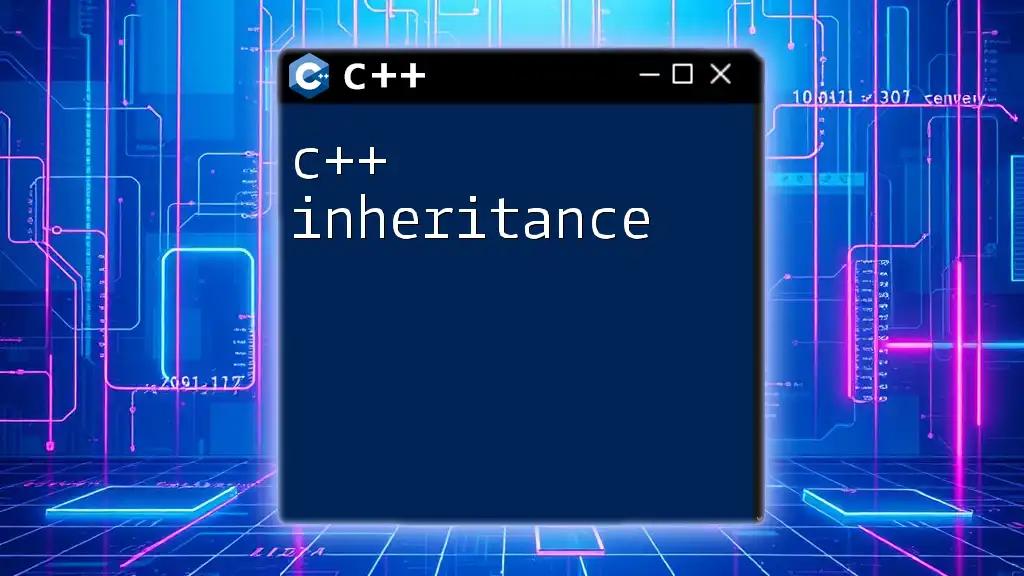
Call to Action
Get Started with C++ Builder Today! Dive into the world of application development by signing up for our courses, where you'll find hands-on lessons to kickstart your journey in building powerful software with C++ Builder. Explore initial resources and start your project today!