The tilde (`~`) in C++ is used as a bitwise NOT operator and as a destructor in classes, flipping the bits of its operand or defining behavior when an object is destroyed, respectively.
Here’s a code snippet demonstrating both uses:
#include <iostream>
class Demo {
public:
Demo() { std::cout << "Constructor called" << std::endl; }
~Demo() { std::cout << "Destructor called" << std::endl; }
};
int main() {
Demo obj;
int number = 5; // 0101 in binary
int result = ~number; // Bitwise NOT operation
std::cout << "Bitwise NOT of " << number << " is " << result << std::endl; // Output will be -6
return 0;
}
What is the Tilde (~) in C++?
Definition of the Tilde Symbol
The tilde symbol (~) can be found in various programming languages, often used for different purposes. In C++, it primarily serves as a bitwise NOT operator and marks the beginning of a destructor. Understanding its meaning in these contexts is crucial for effective C++ programming.
Tilde Usage in C++
In C++, the tilde indicates two fundamental concepts:
- Bitwise NOT Operator: This operator is essential in performing bit manipulation.
- Destructor: The tilde signals the end of an object's lifecycle in object-oriented programming.
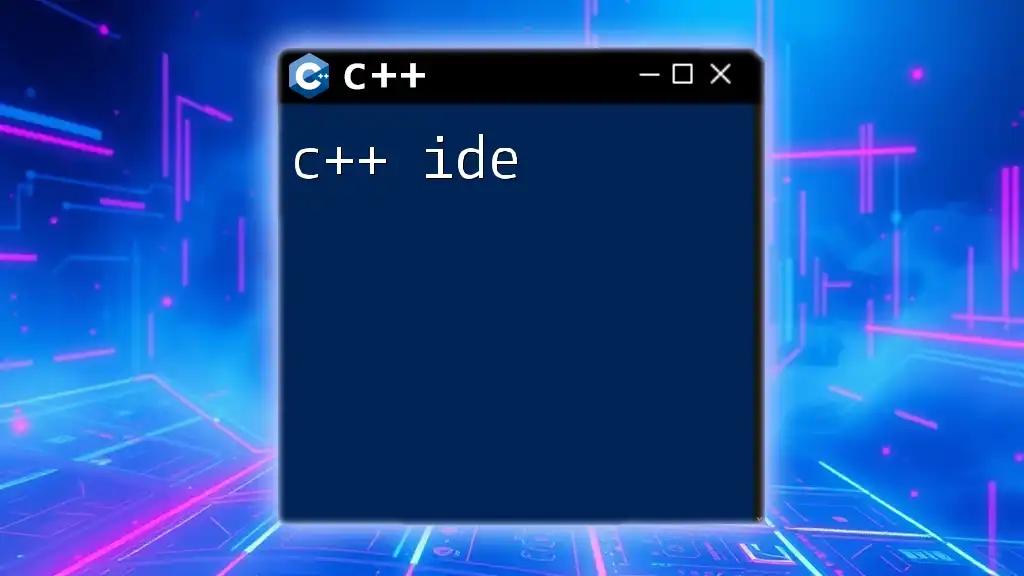
Tilde as the Bitwise NOT Operator
Understanding Bitwise Operations
Bitwise operations directly manipulate bits within data types. This kind of manipulation is critical in scenarios such as performance optimization, direct hardware interaction, and cryptography. The ability to work at the bit level unlocks powerful programming capabilities.
How the Bitwise NOT Operator Works
The tilde serves as the bitwise NOT operator, which flips each bit of an integer. For an integer `x`, the tilde operation is denoted as `~x`. Whenever this operation is performed, each 0 becomes 1 and each 1 becomes 0 in its binary representation.
Code Example: Using the Bitwise NOT Operator
#include <iostream>
int main() {
int x = 5; // Binary: 0000 0101
int result = ~x; // Binary: 1111 1010
std::cout << "The bitwise NOT of " << x << " is " << result << std::endl; // Outputs: -6
return 0;
}
In this example, the integer `5` is represented in binary as `0000 0101`. When the bitwise NOT operator is applied, each bit is flipped, resulting in `1111 1010`, which corresponds to -6 in two's complement representation. This behavior highlights how the bitwise NOT operator can interact with negative numbers.

Tilde as a Destructor in C++
Understanding Constructors and Destructors
In C++, objects require careful management of resources. This is where constructors and destructors play a critical role. A constructor initializes an object when it is created, while a destructor is crucial for cleaning up when the object is no longer needed, thus preventing resource leaks.
Syntax of a Destructor
A destructor is defined using the tilde symbol followed by the class name. It is executed automatically when an object's lifetime ends, ensuring that any resources allocated during the object's life are freed appropriately.
Code Example: Implementing a Destructor
#include <iostream>
class MyClass {
public:
MyClass() {
std::cout << "Constructor called" << std::endl;
}
~MyClass() { // Tilde indicates this is a destructor
std::cout << "Destructor called" << std::endl;
}
};
int main() {
MyClass obj; // Destructor will be called when obj goes out of scope
return 0;
}
In this code, we define a class `MyClass` with a constructor that outputs a message when an object is created. The destructor, indicated by the tilde, outputs a message when the object is destroyed. This occurs seamlessly when `obj` goes out of scope, illustrating how destructors automate resource management in C++.
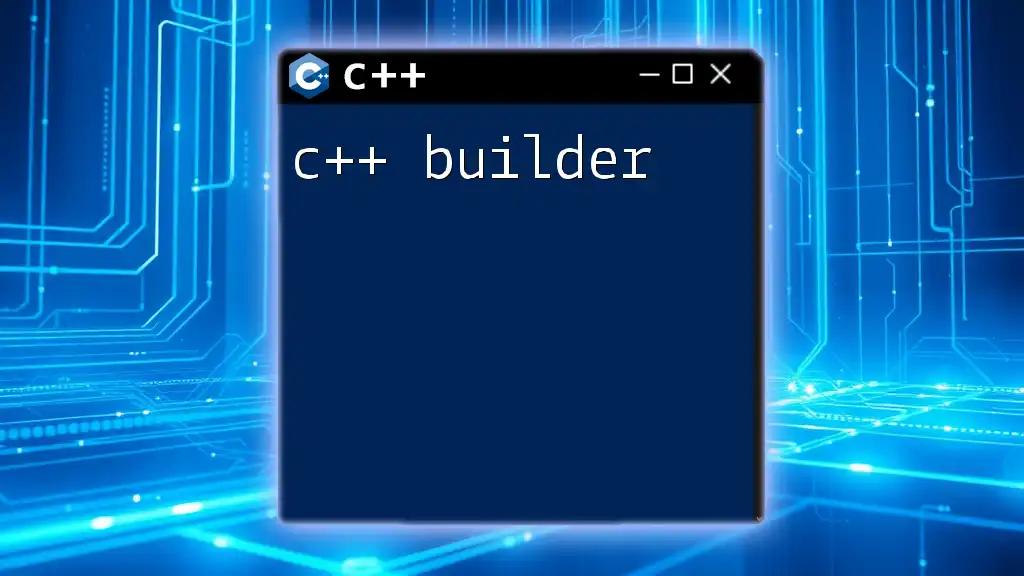
Additional Uses of the Tilde in C++
Tilde in Type Casting
In C++, although the tilde directly is not used for casting, it is essential to understand how it relates to memory manipulation. The tilde operator often appears in type-casting expressions where implicit type conversions are managed.
Tilde as a Unary Operator in Variadic Templates
In C++, variadic templates allow functions or classes to accept an arbitrary number of arguments. While the tilde doesn't directly operate here, understanding how to leverage templates can enhance your programming skills.
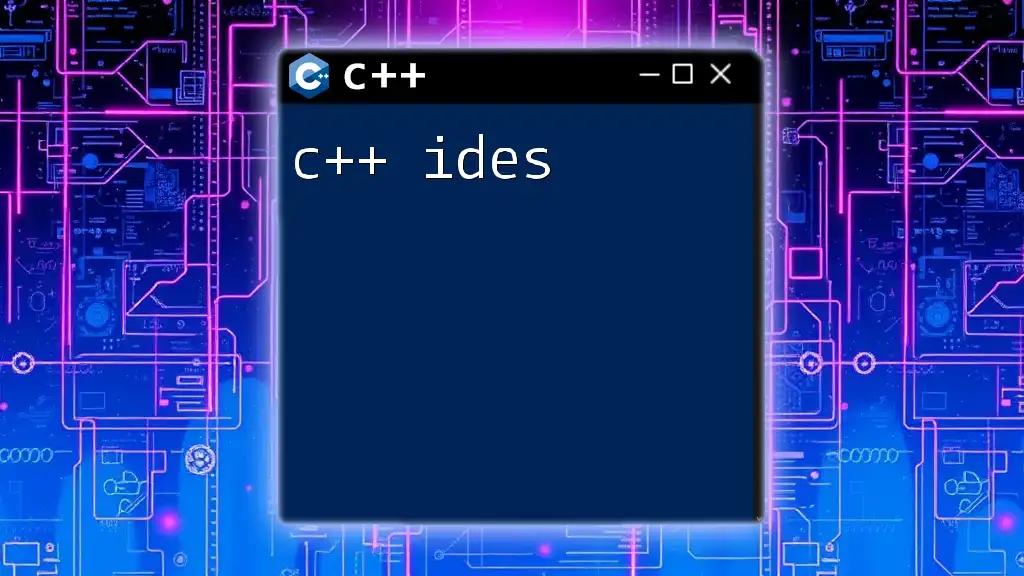
Tilde in Programming Best Practices
Understanding When to Use Tilde
When utilizing the tilde, it's essential to understand its context. Use it:
- For Bitwise NOT Operations: When you need to manipulate binary digits directly.
- For Declaring Destructors: To ensure proper cleanup of resources in an object-oriented context.
Common Mistakes to Avoid
Many beginners misunderstand the use of the tilde, especially with destructors. It's crucial to remember that destructors are called automatically; mismanagement can lead to undefined behavior. Always pair your constructors with destructors to manage resources efficiently.

Conclusion
The C++ tilde symbol serves several essential functions in the language, particularly as a bitwise NOT operator and as an indicator of destructors. Mastery of these concepts significantly enhances your ability to manipulate data and manage resources effectively in C++. As you continue to explore C++ programming, keep the versatility of the tilde in mind; it's a powerful tool in your coding arsenal.