C++ Builder Free is a version of the popular C++ Builder integrated development environment that allows developers to create Windows applications quickly and easily using C++ commands.
Here’s a simple code snippet demonstrating how to create a basic Hello World application in C++ Builder:
#include <vcl.h>
#pragma hdrstop
#include <tchar.h>
int main()
{
ShowMessage("Hello, World!");
return 0;
}
What is C++Builder?
Definition and Background
C++Builder is a powerful integrated development environment (IDE) specifically designed for C++ programming, developed by Embarcadero Technologies. It excels in Rapid Application Development (RAD) by providing developers with the tools needed to create applications quickly and efficiently. With its robust features, C++Builder facilitates both desktop and mobile application development, making it a valuable asset in today’s software development landscape.
Key Features of C++Builder
C++Builder boasts a variety of features that enhance productivity and accelerate the development process:
- Visual Design Tools: C++Builder provides a rich set of visual components that allow you to construct user interfaces visually, speeding up the design process.
- VCL (Visual Component Library): This extensive library simplifies the creation of Windows applications with pre-built components, allowing developers to focus on functionality rather than boilerplate code.
- FireMonkey Framework: This cross-platform GUI framework extends the capabilities of C++Builder, enabling the development of applications that run on Windows, macOS, iOS, and Android.
- Integrated Debugging Tools: C++Builder offers powerful debugging capabilities, allowing developers to diagnose issues efficiently and improve code quality.
- Database Connectivity: With built-in database components and support for various database technologies, C++Builder facilitates the development of data-driven applications seamlessly.
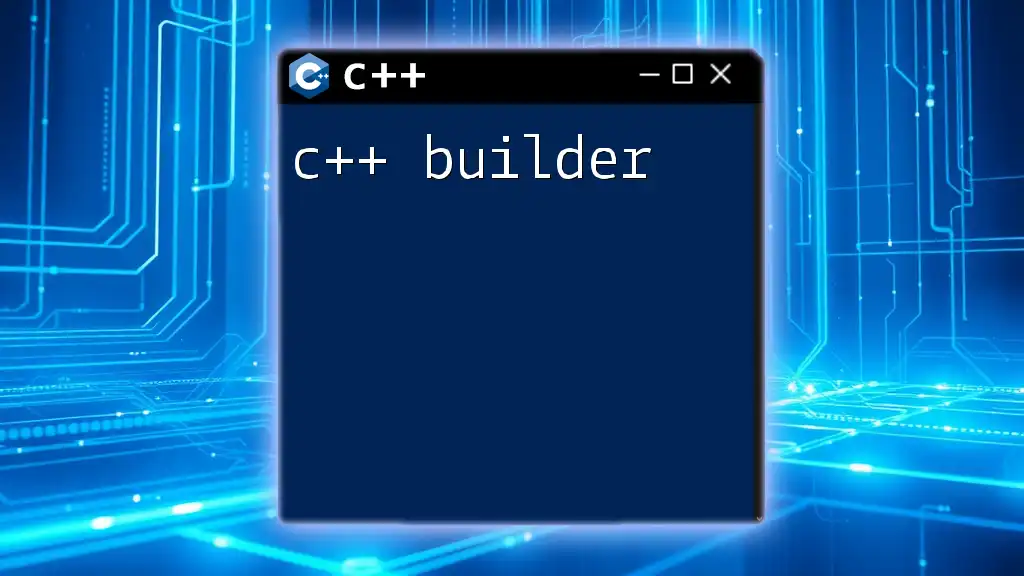
C++Builder Free: What You Need to Know
Overview of the Free Edition
C++Builder Free is a limited edition of C++Builder, aimed at beginners, hobbyists, and those looking to experiment without financial commitments. While it retains several powerful features, it lacks some advanced tools and capabilities found in the commercial versions.
Benefits of Using C++Builder Free
There are numerous advantages to choosing C++Builder Free:
- Cost-Effective Option: As a free offering, it provides an economical entry point for those who wish to learn or develop small-scale applications.
- Access to Core Features: Users gain access to many of the essential features of C++Builder that allow for efficient development workflows.
- Learning Opportunity: C++Builder Free is ideal for students and new developers looking to familiarize themselves with C++ programming and application design.
Limitations of C++Builder Free
However, potential users should be aware of its limitations:
- Restricted Features: Compared to the professional versions, the free edition may lack advanced functionalities, such as certain components and toolsets.
- Project Size Limits: C++Builder Free may impose restrictions on the size and complexity of projects that can be developed.
- Commercial Use Restrictions: It is important to understand that the free edition is typically limited to educational and non-commercial projects, so those looking to develop software for profit should consider an upgrade.
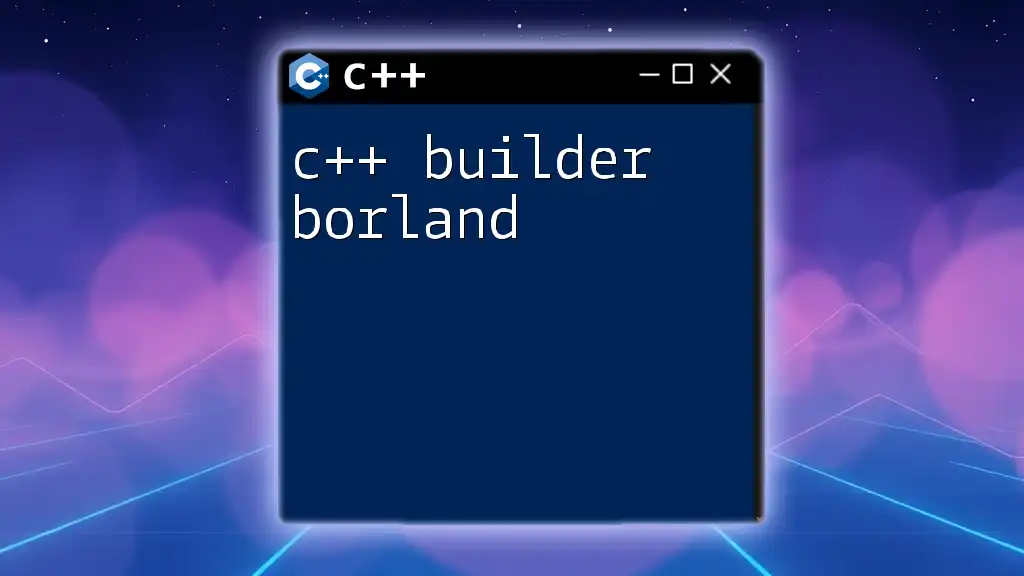
How to Download C++Builder Free
Step-by-Step Download Guide
To get started with C++Builder Free, follow these simple steps:
- Visit the Official Embarcadero Website: Navigate to [Embarcadero's official website](https://www.embarcadero.com).
- Find the C++Builder Free Download Page: Look for the section dedicated to C++Builder Free.
- Check System Requirements: Ensure your system meets the necessary requirements for installation, which typically include compatible versions of Windows and sufficient hardware specifications.
- Download the Installer: Click the download link to obtain the C++Builder Free installer.
- Run the Installation: Follow the on-screen instructions to install the software on your machine.
Troubleshooting Common Download Issues
If you encounter issues during the download or installation:
- Finding the Download Link: Double-check the website, as links may change. Consider searching for "C++Builder Free download" directly in your preferred search engine.
- Error Messages during Installation: Make sure you have administrative privileges. If errors persist, uninstall any previous versions of C++Builder before attempting the new installation.
- Compatibility Issues: Verify that your operating system meets the specified requirements. Upgrading your OS or hardware may resolve compatibility issues.
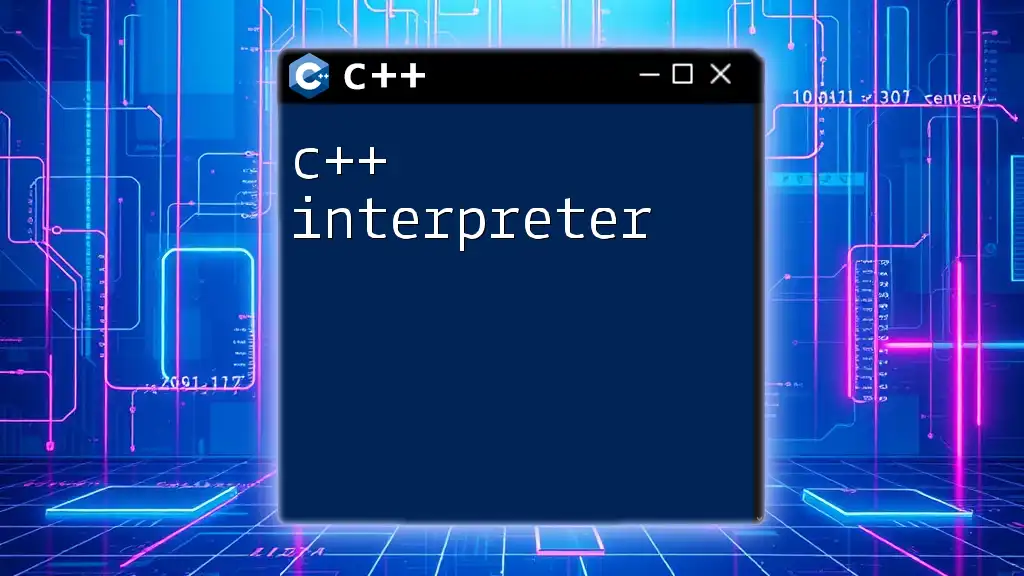
Getting Started with C++Builder Free
Setting Up Your First Project
Once installed, you can begin your journey with C++Builder Free by setting up your first project:
- Creating a New Project: Launch C++Builder and select "File" > "New" > "VCL Forms Application". This will create a basic Windows form application.
- IDE Interface Overview: Familiarize yourself with the IDE, which includes the tool palette, form designer, and properties window. Each of these components plays a vital role in your development workflow.
- Project Settings: Customize project settings such as target platform, compiler options, and debugging preferences to suit your needs.
Writing Your First C++ Program
Now, let’s write a simple program to get started. Create a "Hello, World!" application as follows:
#include <vcl.h>
#pragma hdrstop
USEFORM("Unit1.cpp", Form1); /* TForm1 */
int WINAPI WinMain(HINSTANCE, HINSTANCE, LPSTR, int)
{
try
{
Application->Initialize();
Application->MainFormOnTaskbar = true;
Application->CreateForm(__classid(TForm1), &Form1);
Application->Run();
}
catch (Exception &exception)
{
Application->ShowException(&exception);
}
catch (...)
{
try
{
throw Exception("");
}
catch (Exception &exception)
{
Application->ShowException(&exception);
}
}
return 0;
}
In this example, the `WinMain` function initializes the application, creates a form (TForm1), and runs the application loop. After creating the form in the form designer, you can add components like labels and buttons to enhance user interaction.
- Compiling and Running the Program: Once you have written your code, click the "Run" button or press F9. This compiles your project and launches the application, allowing you to see your code in action.
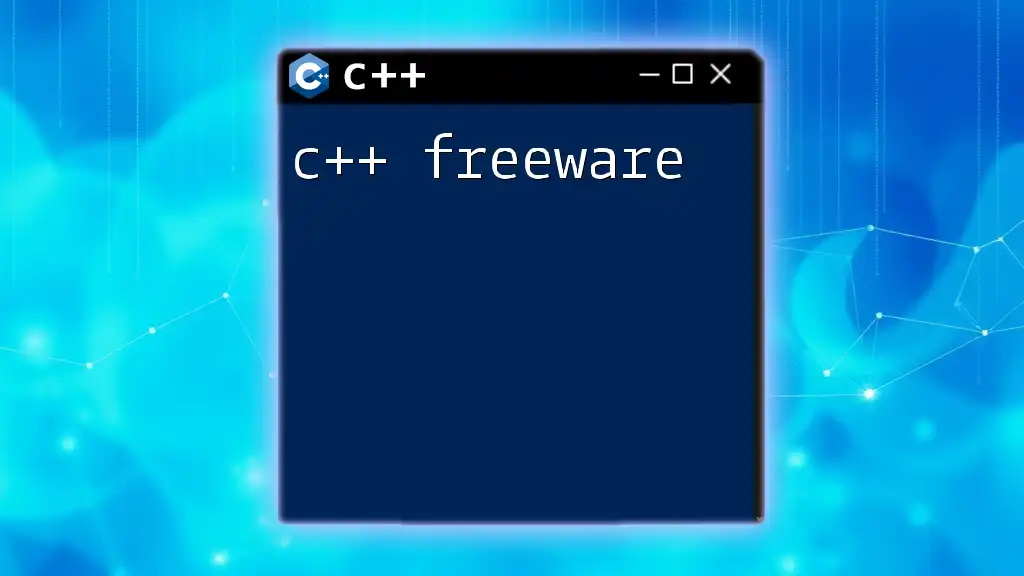
Exploring C++Builder Free Features
Visual Component Library (VCL)
One of the standout features of C++Builder is the Visual Component Library (VCL).
- Overview of VCL Components: VCL provides a collection of pre-designed components (like buttons, text boxes, and grids) that can be dragged and dropped onto forms in the designer.
- Creating a Simple Form: You can quickly create user interfaces by dragging components from the tool palette to the form, setting their properties in the properties window, and adding event handlers as needed.
FireMonkey: Cross-Platform GUI Framework
The FireMonkey framework extends the capabilities of C++Builder, allowing for cross-platform application development:
- Capabilities of FireMonkey: It supports advanced graphics and visual effects, making it ideal for modern UI design across multiple platforms.
- Building a Multi-Platform Application: Using FireMonkey, you can write code once and compile it for various targets. With just a few adjustments, the same code can run on Windows, macOS, iOS, and Android, simplifying development for multiple environments.
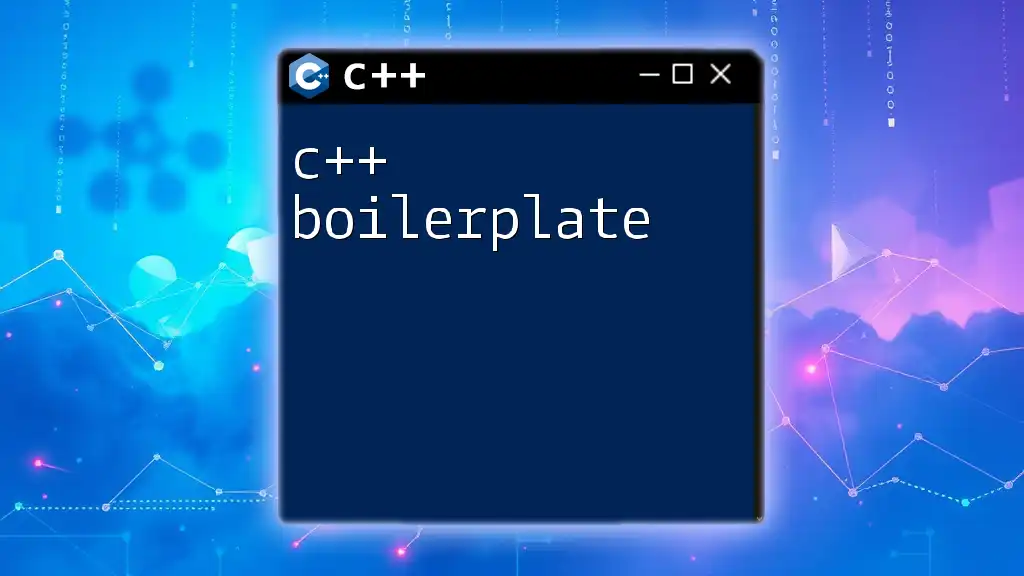
Resources for Learning C++Builder
Online Tutorials and Documentation
Embarcadero provides excellent official documentation, which is crucial for novice and experienced users alike. You can also find numerous online tutorials and video courses that guide you through specific aspects of C++Builder and RAD development.
Community and Support
Engagement with the community can be invaluable. Online forums, user communities, and social media groups allow developers to share insights, ask questions, and collaborate on projects. Resources like Stack Overflow are also great for troubleshooting problems and sharing best practices.
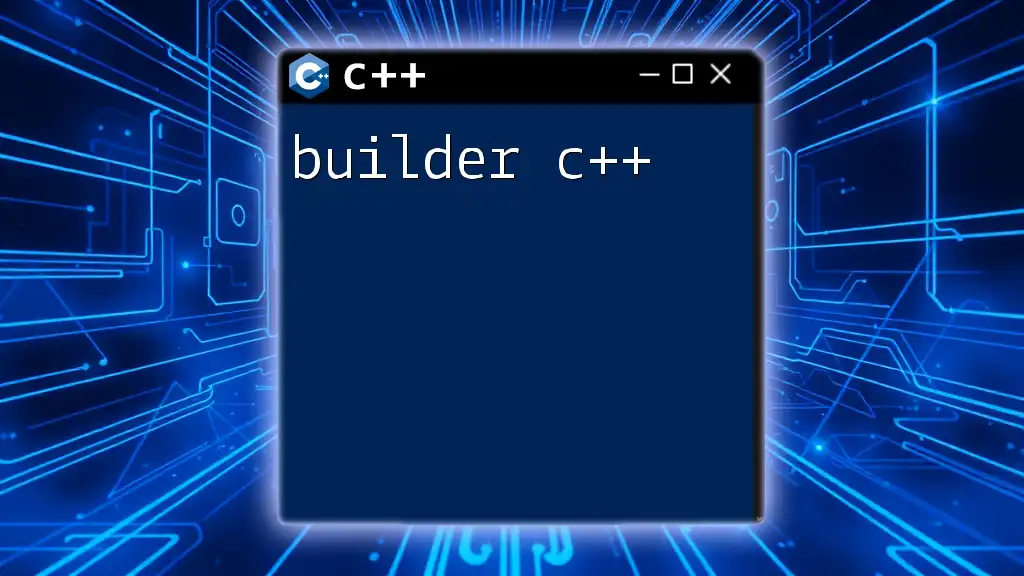
Conclusion
C++Builder Free provides a compelling entry point for developers to explore the capabilities of an advanced IDE without financial commitments. From rapidly building applications to leveraging the benefits of both VCL and FireMonkey, C++Builder Free is a valuable tool in your development toolkit. Whether you’re a student starting or a hobbyist working on personal projects, it encourages exploration and innovation.
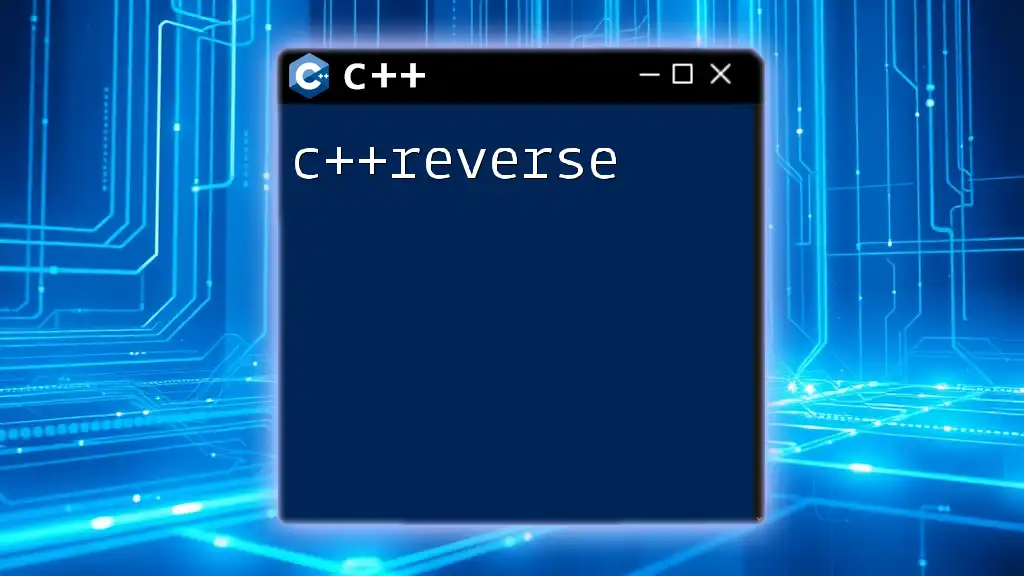
FAQs
Can I use C++Builder Free for commercial projects?
Generally, C++Builder Free is designated for personal and educational use. For commercial projects, users should consider upgrading to a paid version to ensure compliance with licensing agreements.
What are the best alternatives to C++Builder Free?
Alternative development environments include Microsoft Visual Studio, Code::Blocks, and Qt Creator. Each has unique features and strengths, so it's essential to consider your specific needs and project requirements when making a choice.
How do I upgrade from C++Builder Free to a paid version?
Upgrading is straightforward. Visit Embarcadero's official website, where you can choose from various licensing options that best suit your business needs or project scale. Follow the purchase process, and you will receive a new license key to unlock the additional features in your C++Builder environment.