Borland C++ Builder is an integrated development environment (IDE) used for rapid application development in C++ that combines a powerful visual design interface with a robust C++ compiler.
#include <vcl.h>
#pragma hdrstop
USEFORM("Unit1.cpp", Form1); // Creates a form
int WINAPI _tWinMain(HINSTANCE, HINSTANCE, LPTSTR, int) {
Application->Initialize();
Application->MainFormOnTaskbar = true;
Application->CreateForm(__classid(TForm1), &Form1);
Application->Run();
return 0;
}
Getting Started with Borland C++ Builder
Installation Process
System Requirements
Before installing Borland C++ Builder, it's essential to ensure your system meets the required specifications. While there might be variations based on the version, generally, the following are considered:
-
Minimum Specifications:
- Windows OS (usually Windows 7 or higher)
- At least 2 GB of RAM
- 4 GB of available disk space
-
Recommended Specifications:
- Windows 10 or higher
- 8 GB of RAM or more
- SSD for better performance
Downloading and Installing
To get started with Borland C++ Builder, you need to download the installation files. Here’s a brief guide on how to do this:
- Visit the official Embarcadero website (the current owner of Borland C++ Builder).
- Navigate to the C++ Builder section.
- Choose the appropriate version for your needs (Trial, Professional, Enterprise).
- Follow the download link and save the file.
Once downloaded:
- Double-click the installer.
- Follow the prompts to complete the installation, selecting your desired components along the way.
Setting Up Your First Project
Creating a New Project
Upon launching Borland C++ Builder, you'll be greeted by a welcome screen. Here's how to create your first project:
- Navigating the IDE: Familiarize yourself with the layout; the Project Manager will be on the left, and the Form Designer will be in the center.
- Selecting Project Templates: Choose from various templates. For a standard Windows application, select VCL Application.
Exploring the IDE Layout
Understanding the IDE (Integrated Development Environment) layout is crucial. Key areas include:
- Toolbars: Quick access to commonly used tools like Save, Debug, and Build.
- Project Manager: Displays all files and resources related to your project.
- Form Designer: A visual interface where you’ll design your application's UI by placing components.
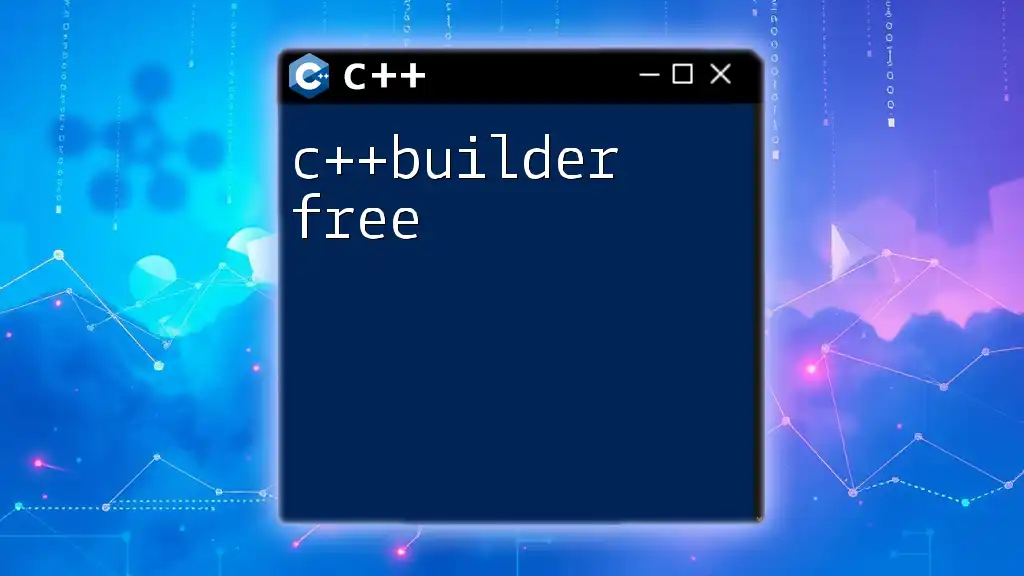
Core Features of Borland C++ Builder
Visual Component Library (VCL)
What is VCL?
VCL (Visual Component Library) is a bundled collection of pre-built components that allow developers to rapidly design user interfaces and implement functionality without writing extensive code from scratch. VCL components cover myriad UI elements, from buttons to complex data grids.
Using VCL to Create User Interfaces
Creating a user interface using VCL is straightforward:
-
Adding Components: Drag and drop components from the Tool Palette to the Form Designer. Popular components you might frequently use include:
- TButton for clickable buttons.
- TLabel for text display.
- TEdit for single-line text input.
-
Customizing Properties: Each component has customizable properties accessible in the Object Inspector. For instance, you can change a button's caption, color, or alignment by selecting the component and editing its properties in this inspector.
Integrated Development Environment (IDE) Features
Editor Features
Borland C++ Builder comes equipped with an advanced code editor which includes:
- Code Completion: As you type, the IDE suggests possible completions, significantly speeding up development.
- Syntax Highlighting: Keywords, variables, and comments are highlighted, making it easier to spot errors.
Debugger Tools
The debugging capabilities in C++ Builder are robust:
- Setting Breakpoints: Click in the margin next to the line numbers to set a breakpoint. This halts execution at that line so you can inspect variable states.
- Inspecting Variables: While debugging, hover over variables or use the Watches panel to understand their current values.
Making Use of Build Configurations
Default Build Configurations
Borland C++ Builder allows for different build configurations, crucial for developing and deploying applications:
- Debug Configuration: Includes additional debug information; helpful during development to trace errors.
- Release Configuration: Optimized for deployment; only includes necessary components for performance.
Creating Custom Build Configurations
If the provided configurations don't meet your needs, you can create custom configurations. Navigate to the Project Configuration settings, duplicate an existing configuration, and make necessary adjustments.
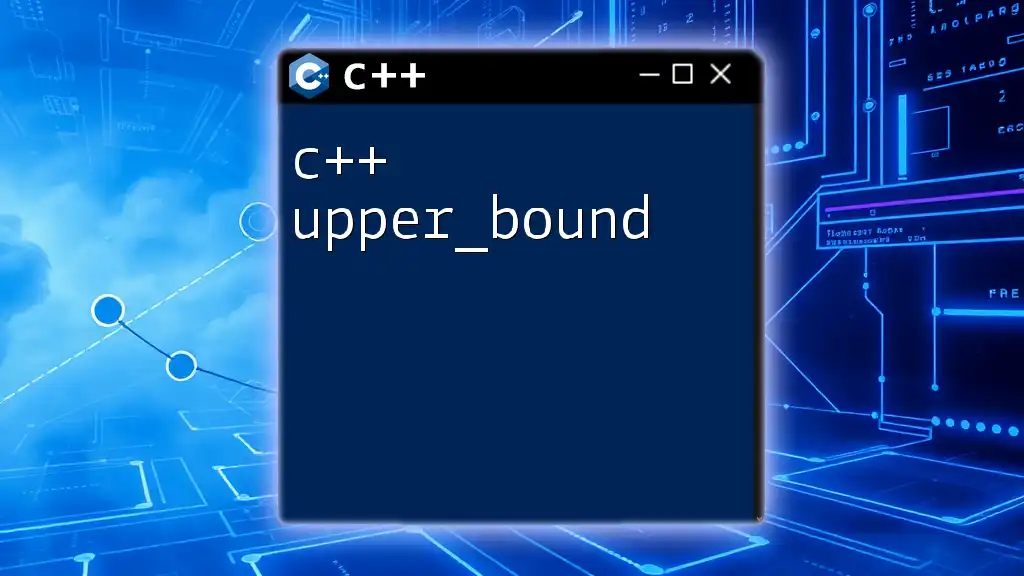
Advanced Topics in Borland C++ Builder
Database Connectivity
Using Database Components
One of the strengths of Borland C++ Builder is its database integration capabilities. It offers comprehensive tools for working with various databases, utilizing components like `TDataSet`, `TDatabase`, and `TQuery`.
Connecting to a Database Example
Here's a basic example of connecting to a database:
TDatabase *db = new TDatabase(this);
db->DatabaseName = "MyDatabase";
db->Connected = true;
In this example, we create a new `TDatabase` object, specify the database name, and establish a connection.
Executing SQL Queries
To retrieve or manipulate data, you can execute SQL queries. Below is a sample of how to use the `TQuery` component:
TQuery *query = new TQuery(this);
query->DatabaseName = "MyDatabase";
query->SQL->Text = "SELECT * FROM MyTable";
query->Open();
This code initializes a new query, connects it to your database, defines an SQL statement, and opens the query to fetch results.
Custom Component Development
Creating a New Component
Sometimes the built-in components may not suffice. Developing your own component allows you to encapsulate unique functionality. Begin by defining the component class and inheriting from a standard VCL component.
Registering Your Component
After creating your custom component, ensure you register it to make it accessible in the IDE:
class PACKAGE TMyComponent : public TComponent {
// Component code here
};
__fastcall Register() {
RegisterComponents("Custom", TMyComponent);
}
This code snippet demonstrates how to create and register a custom component so that it appears under a custom palette within the IDE.
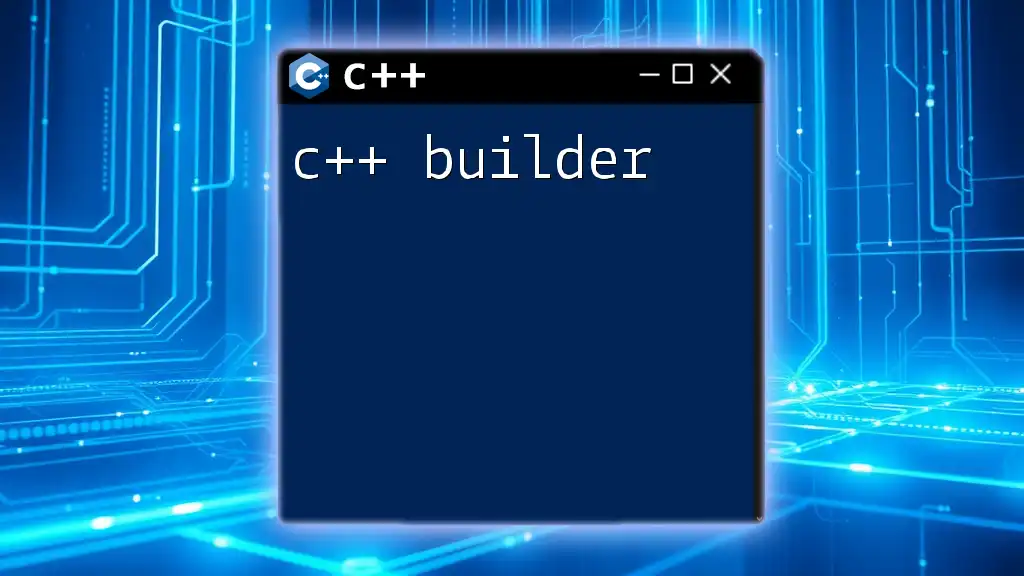
Best Practices and Tips
Code Efficiency
To ensure your application performs optimally, follow these best practices:
- Optimizing Performance in C++ Builder: Avoid unnecessary calculations within loops, use efficient algorithms, and minimize memory usage by properly managing object lifetimes.
- Memory Management Tips: Use smart pointers (found in modern C++) appropriately to avoid memory leaks and ensure that dynamic resources are correctly released when no longer in use.
Community and Resources
Engage with the broader Borland C++ Builder community for support and additional resources. Here are a few recommended avenues:
- Forums: Participate in discussions on platforms that specialize in C++ or Borland technologies.
- Documentation: The official documentation is an invaluable resource, covering everything from basic component usage to advanced features.
Curated sample projects and tutorials can provide hands-on experience and insights into effective development practices using C++ Builder Borland.
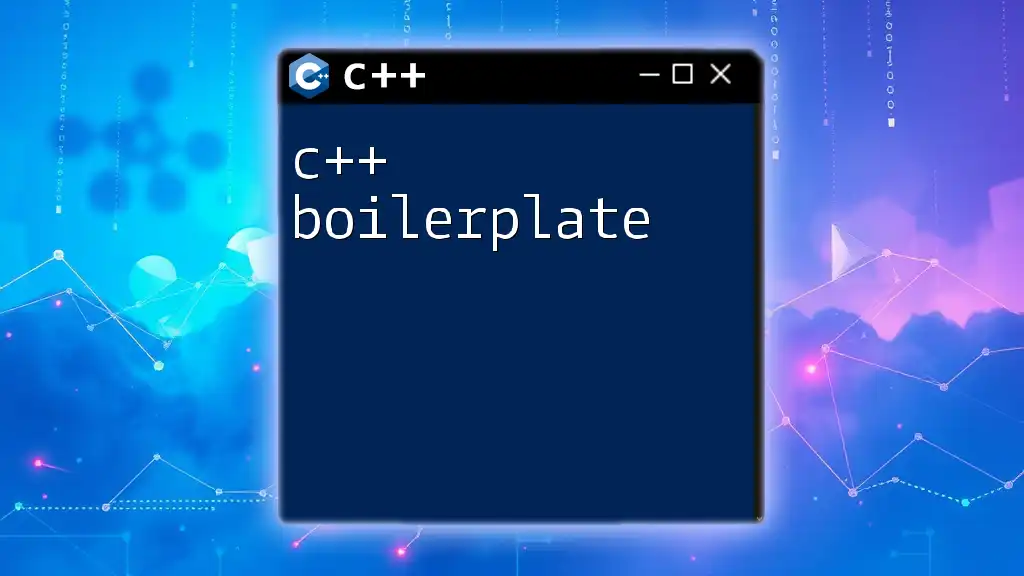
Conclusion
Borland C++ Builder is a powerful integrated development environment, best known for its rich UI components and robust database connectivity. Engaging with the platform opens various avenues for application development while emphasizing efficiency and ease of use. As you become more familiar with its features, you will discover the vast potential C++ Builder offers.
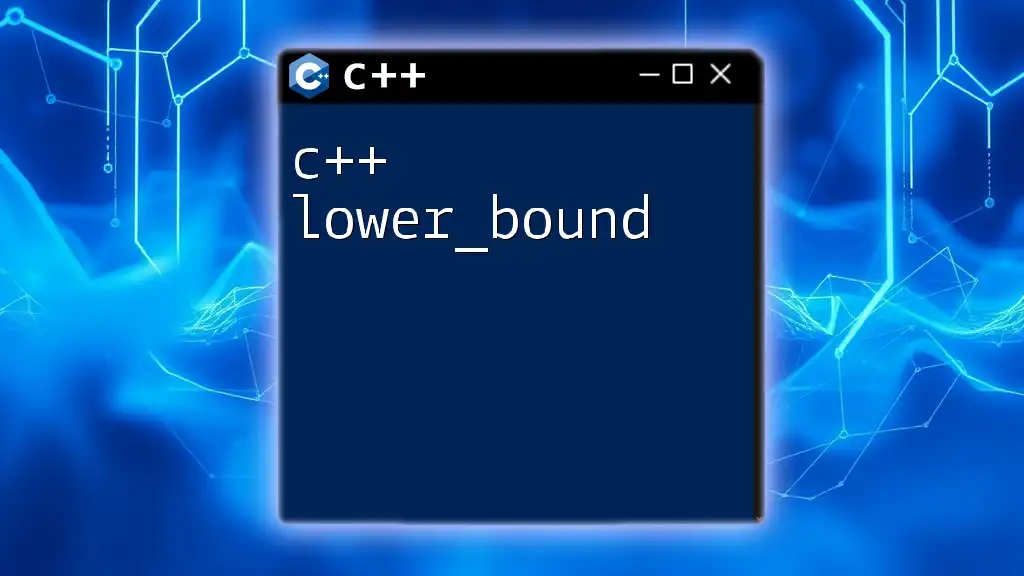
Call to Action
Join our community of learners and developers! Subscribe to our newsletter, register for upcoming webinars, and participate in engaging discussions to further enhance your proficiency in C++ Builder Borland!