When searching for a C++ IDE for Linux, consider tools like Code::Blocks or Visual Studio Code, which offer robust support for C++ development and ease of use.
Here's an example of a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding IDEs: What’s an IDE and Why Use One?
Integrated Development Environments (IDEs) are powerful tools designed to simplify the software development process by integrating various components needed for coding, debugging, and project management into one application. This includes code editors, compilers, debugging tools, and build automation features.
Using an IDE for C++ development offers significant advantages:
- Code Suggestions and Auto-completions: IDEs enhance productivity by suggesting code completions as you type, reducing the chances of syntax errors and increasing coding speed.
- Debugging Tools: A built-in debugger helps track down issues, allowing developers to step through code, set breakpoints, and examine variables without external tools.
- Project Management Capabilities: IDEs help organize project files and directories, making it easier to manage larger codebases.
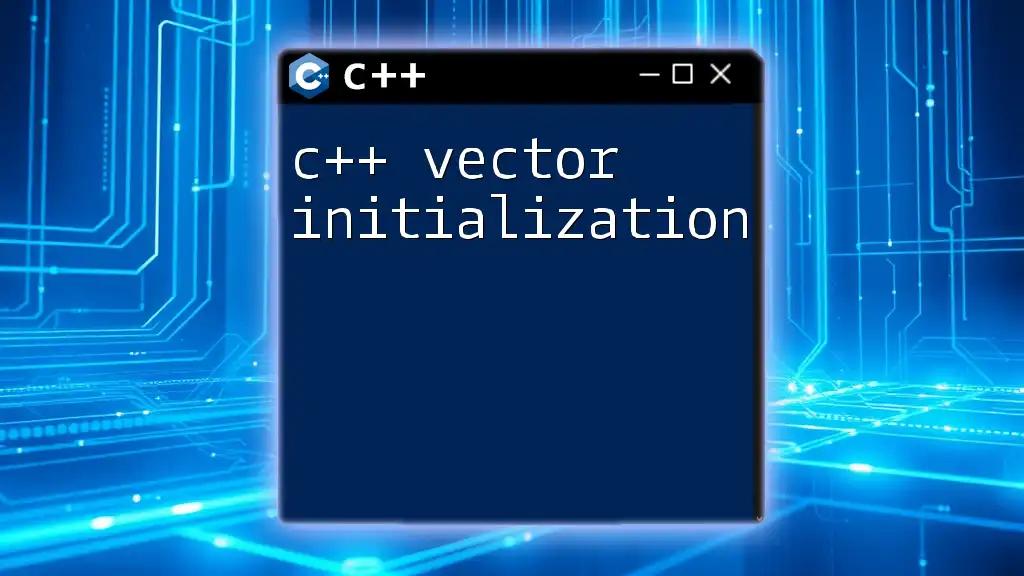
Choosing the Right C++ IDE in Linux
When selecting a C++ IDE for Linux, consider these essential criteria:
- Performance and Speed: The IDE should run smoothly without introducing significant lag, especially for larger projects.
- User Interface and Experience: A clean, intuitive UI enhances the coding experience and reduces the learning curve.
- Extensibility and Plugins: Look for IDEs that support plugins or extensions to tailor functionalities to your needs.
- Community Support and Documentation: Good documentation and an active community can significantly ease the development process.
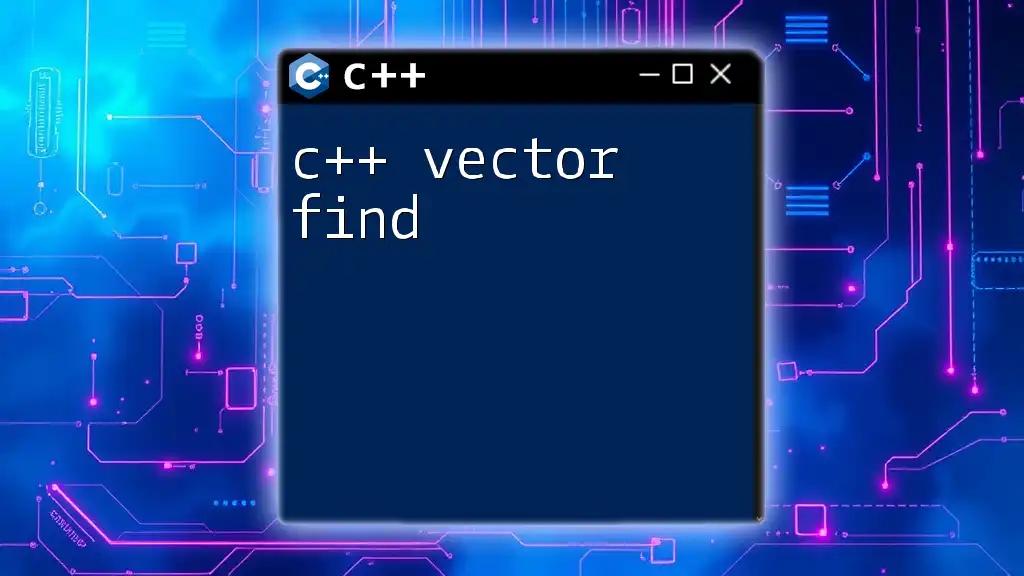
Popular Linux C++ IDEs
Code::Blocks
Code::Blocks is a free and open-source C++ IDE that’s highly flexible and adaptable. It supports various compilers and has a vast array of features.
Getting Started with Code::Blocks
- Installation Steps:
- Use your package manager (like `apt` for Ubuntu) to install:
sudo apt install codeblocks
- Use your package manager (like `apt` for Ubuntu) to install:
- Sample Project Creation:
- Open Code::Blocks, create a new project, select "Console Application," and choose C++.
- Code Snippet Example:
Here's a simple "Hello World" program:
#include <iostream> using namespace std; int main() { cout << "Hello, World!" << endl; return 0; }
User Experience and Community Feedback: Many users appreciate its simplicity and customization options, making it a good choice for both beginners and seasoned developers.
Eclipse CDT
Eclipse CDT (C/C++ Development Tooling) is a powerful IDE that provides a robust development environment equipped with features specifically designed for C++.
Installing Eclipse CDT on Linux
-
Installation Guide:
- Download Eclipse from the official site or using your package manager:
sudo apt install eclipse
- Download Eclipse from the official site or using your package manager:
-
Basic Project Setup:
- Create a new C/C++ project and configure it to use the desired toolchain.
-
Code Snippet for a Simple Program:
#include <iostream> int main() { std::cout << "Welcome to Eclipse CDT!" << std::endl; return 0; }
Pros and Cons of Eclipse CDT: While it offers extensive features and customizability, some users find its heavy resource usage and complex interface challenging.
CLion
CLion, developed by JetBrains, is a cross-platform IDE that provides intelligent code assistance and a powerful debugging environment.
Setting Up CLion on Linux
-
Step-by-Step Installation Process:
- Download from the JetBrains site and install via the terminal:
tar -xzf clion-*.tar.gz cd clion-*/bin ./clion.sh
- Download from the JetBrains site and install via the terminal:
-
Project Creation Guide:
- Open CLion and create a new project, choosing "C++ Executable".
-
Code Example to Illustrate Functions: Here’s a simple program:
#include <iostream> using namespace std; void greet() { cout << "Hello from CLion!" << endl; } int main() { greet(); return 0; }
User Review Insights: Users appreciate CLion's smart code navigation and refactoring capabilities, though it requires a paid license.
Qt Creator
Qt Creator is an IDE tailored for developing applications with the Qt application framework, ideal for both GUI and console applications.
Installing Qt Creator
-
Installation Instructions:
- Install via package manager:
sudo apt install qtcreator
- Install via package manager:
-
Creating a Basic GUI Application:
- Start a new project, select "Qt Widgets Application," then follow the wizard.
-
Code Snippet for a Simple Qt Application:
#include <QApplication> #include <QPushButton> int main(int argc, char *argv[]) { QApplication app(argc, argv); QPushButton button("Hello from Qt Creator!"); button.show(); return app.exec(); }
User Feedback: Developers enjoy the integrated GUI designer, making it easier to create stunning user interfaces, although it may feel complex to newcomers.
Visual Studio Code
Visual Studio Code is a lightweight yet powerful code editor from Microsoft, widely used as a C++ IDE through extensions.
Setting Up VS Code for C++ Development
- Installation of Extensions:
- Install the C/C++ extension from the Extensions Marketplace for debugging and IntelliSense capabilities.
- Creating a 'Hello World' Project:
- Simply create a new `.cpp` file in your workspace and write:
#include <iostream> int main() { std::cout << "Hello, World from VS Code!" << std::endl; return 0; }
Advantages of VS Code: It’s lightweight and fast, with an extensive library of extensions, but may require additional setup for a complete C++ environment.
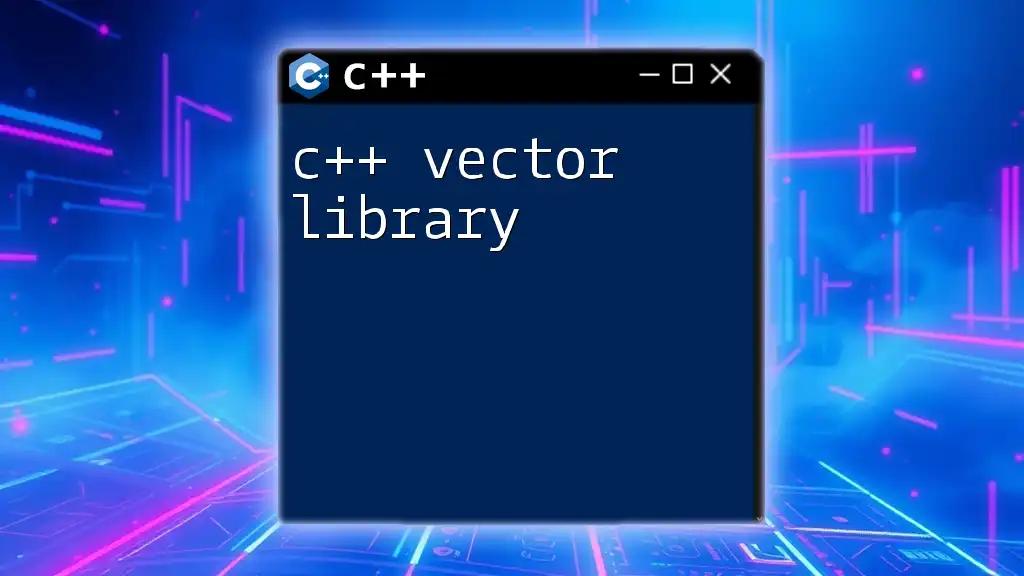
Comparing the Best C++ IDEs for Linux
Feature Comparison Chart
A quick feature comparison can help determine the best IDE for your needs:
IDE | Features | User Experience | Community Support |
---|---|---|---|
Code::Blocks | Free, flexible, customizable | Friendly and simple | Strong community |
Eclipse CDT | Robust, project management | Resource-heavy, complex | Extensive documentation |
CLion | Intelligent code assistance | User-friendly, requires license | Active support |
Qt Creator | Qt design focused | Powerful but complex | Good documentation |
VS Code | Lightweight, highly extensible | Fast and efficient | Large extension library |
Recommendations Based on Use Cases
- Best for Beginners: Code::Blocks or Visual Studio Code due to their simplicity.
- Best for Advanced Users: CLion or Eclipse CDT for their robust feature sets.
- Best for Cross-Platform Development: Qt Creator supports multi-platform applications seamlessly.
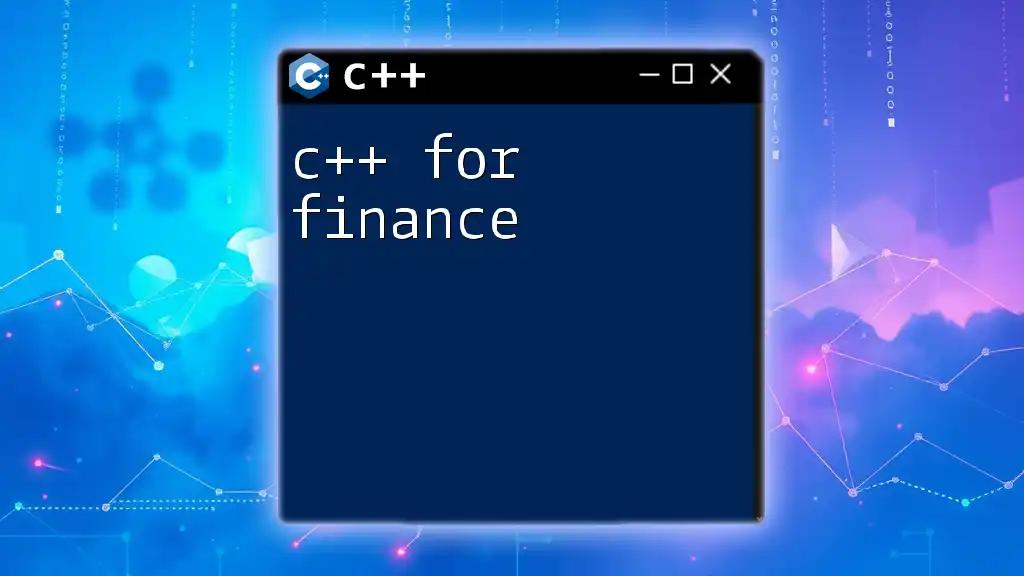
Additional Tools and Tips for C++ Development on Linux
Building Tools and Compilers
Familiarize yourself with build systems like Makefile and CMake to automate the build process. Most of the IDEs integrated CMake support can streamline project configuration.
Debugging Tools
The GNU Debugger (GDB) is indispensable for C++ development. Integrating it with your IDE allows for powerful debugging capabilities. Most IDEs offer built-in GDB integration, enabling you to set breakpoints easily and step through your code.
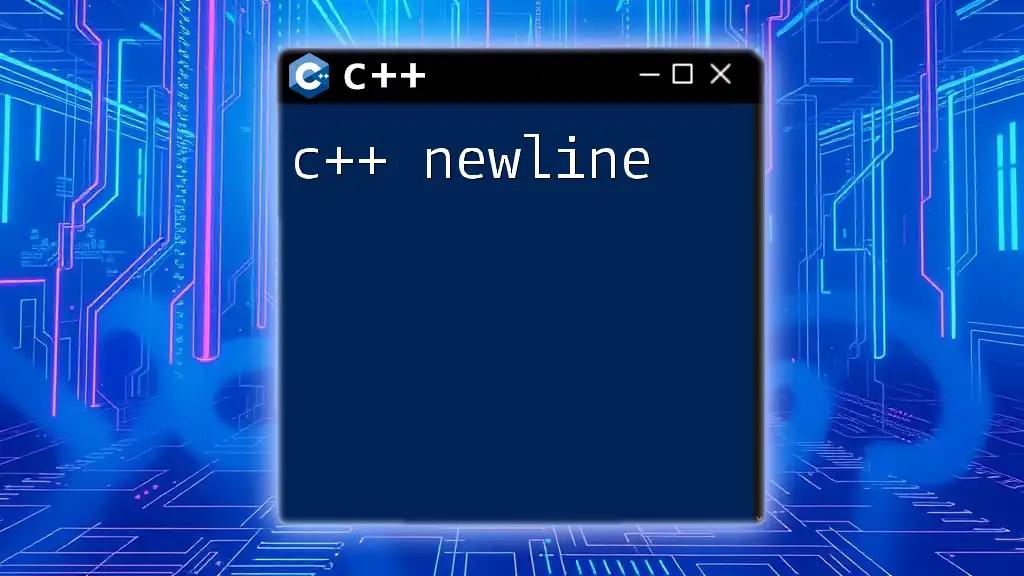
Conclusion
Selecting the right C++ IDE for Linux is crucial for enhancing your coding experience and efficiency. Each IDE has its strengths, and while some are more user-friendly, others offer advanced features that can significantly aid experienced developers. Explore different options, understand their capabilities, and find the one that best fits your workflow.
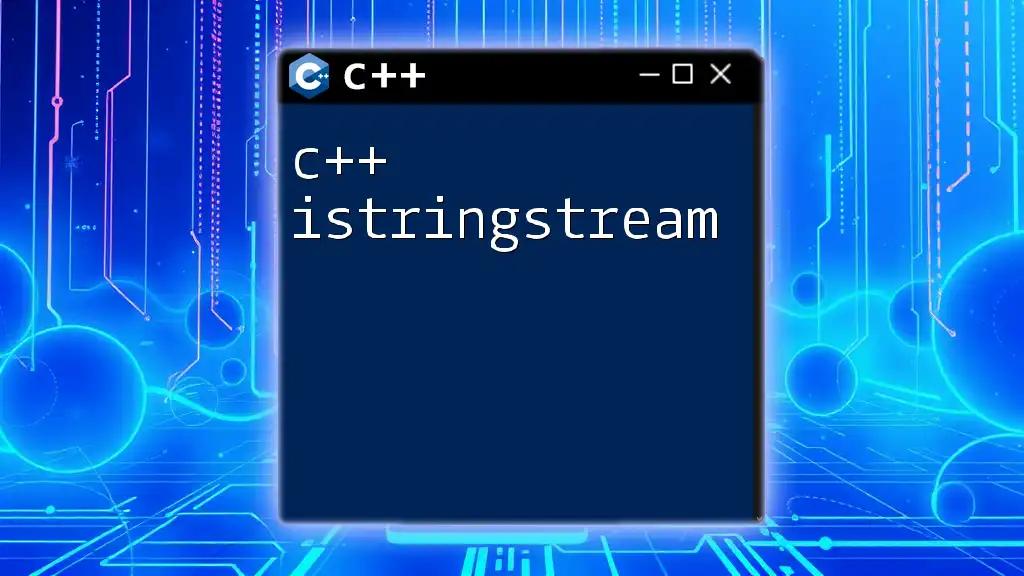
Further Resources
Check out official documentation for each IDE, recommended online tutorials, and community forums for further learning and support as you embark on your C++ development journey in Linux. Happy coding!