The C++ range-based for loop allows you to iterate over elements in a collection, such as an array or a vector, in a concise and efficient manner.
Here’s a code snippet demonstrating the usage of the C++ for-in loop:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding the Basics of For Loops
What is a For Loop?
A `for` loop in C++ is a control flow statement that allows code to be executed repeatedly based on a boolean condition. It is one of the most commonly used loops in programming due to its ability to succinctly define the iteration process. Unlike other loops such as `while` and `do-while`, which can sometimes require more code and can become less clear, the `for` loop organizes the initialization, condition-checking, and increment/decrement logic all in one line.
Syntax of a For Loop
The syntax of the `for` loop is structured as follows:
for (initialization; condition; increment) {
// code block
}
This structure includes three main components:
- Initialization: Setting up the loop control variable.
- Condition: A boolean expression that is tested before each iteration.
- Increment/Decrement: Adjusting the loop control variable after each iteration.
This concise structure makes the `for` loop easy to use and read, especially in cases where the number of iterations is known beforehand.
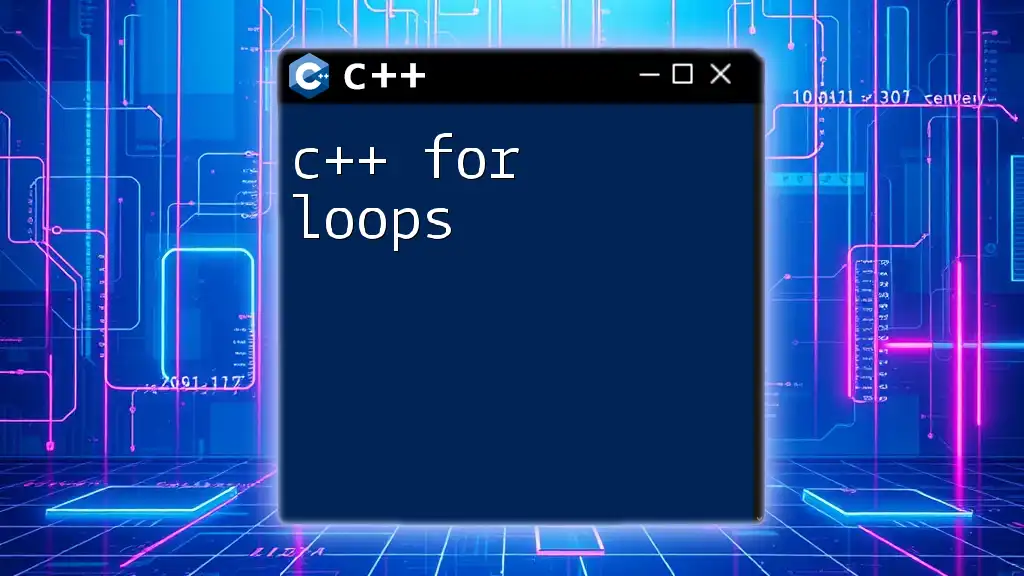
Components of a For Loop
Initialization
Initialization is the starting point for the loop. Here, you typically define your loop control variable, which manages how many times the loop will run.
For instance:
for (int i = 0; i < 10; i++) {
// code block
}
In this example, `int i = 0` initializes `i` to zero. It's crucial to ensure your variable is set correctly so the loop functions as intended.
Condition
The condition is an expression that must be true for the loop to continue executing. Each time the loop runs, this condition is evaluated. If it is true, the loop continues; if false, the loop terminates.
For example:
for (int i = 0; i < 5; i++) {
// Loop will execute as long as i is less than 5
}
This condition checks if `i` is less than 5. When `i` equals 5, the loop ceases execution.
Increment/Decrement
After every iteration of the loop, the increment or decrement operation modifies the loop control variable. This ensures that the loop is progressing toward its termination condition.
Here’s an example of incrementing:
for (int i = 0; i < 10; i++) {
// i increments by 1 each iteration
}
You can also use decrementing:
for (int i = 10; i > 0; i--) {
// i decrements by 1 each iteration
}
Understanding how these components work together is key to mastering the `for` loop.
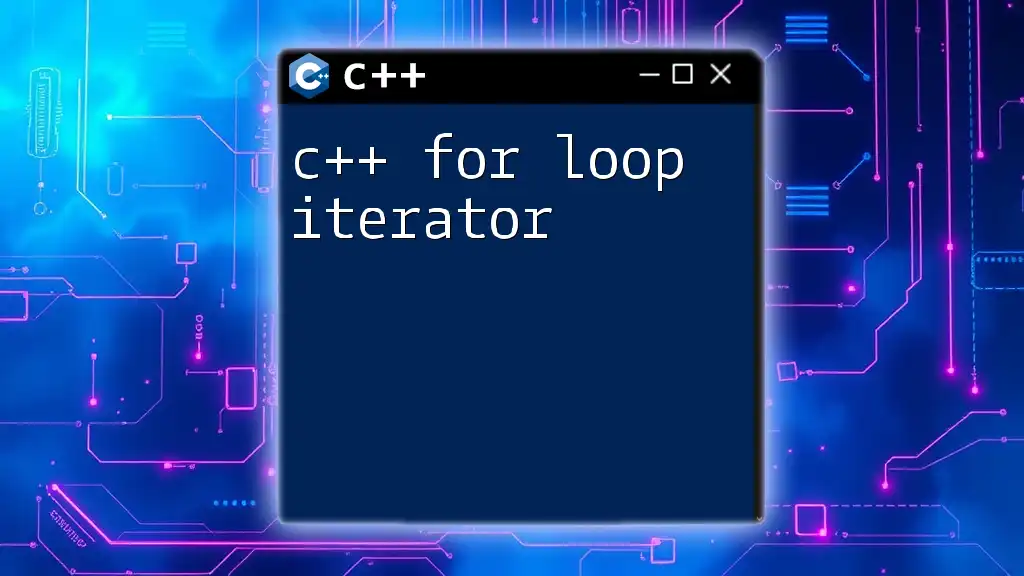
Examples of For Loop in Action
A Simple For Loop Example
Let's consider a simple use-case where we want to find the sum of integers from 1 to 5. The following code snippet demonstrates how to achieve that:
int sum = 0;
for (int i = 1; i <= 5; i++) {
sum += i; // Adding i to sum
}
In this example, `sum` accumulates the value of `i` for each iteration, ultimately yielding a total of 15 after the loop has completed its execution.
Nested For Loops
Nested `for` loops are used when you need to execute a loop within another loop. A common scenario for nested loops is creating a multiplication table:
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= 5; j++) {
std::cout << i * j << " "; // Print the product of i and j
}
std::cout << std::endl; // Move to the next line after inner loop
}
In this structure, the outer loop iterates through values of `i`, while for each iteration of `i`, the inner loop iterates through values of `j`. Together, this allows us to print the products in a matrix format.
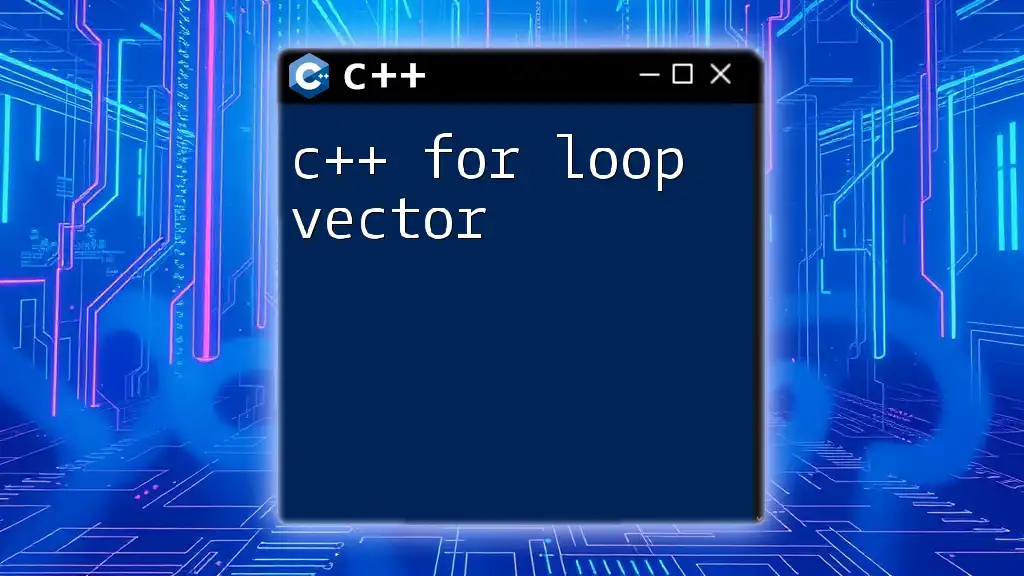
Common Mistakes to Avoid
Off-by-One Errors
One common mistake when using `for` loops is an off-by-one error. This occurs when the loop iterates one time too many or one time too few due to incorrect conditions. For example:
for (int i = 0; i <= 5; i++) {
// This will execute 6 times instead of 5
}
To avoid such errors, always check your loop condition carefully to ensure it aligns with your intentions.
Infinite Loops
An infinite loop happens when the loop’s termination condition is never satisfied, often due to the increment/decrement part being omitted or improperly configured:
for (int i = 1; i <= 10; ) {
// This loop will never end because the increment is missing
}
To prevent infinite loops, make sure that your loop control variable is modified within the loop body, progressing toward meeting the exit condition.
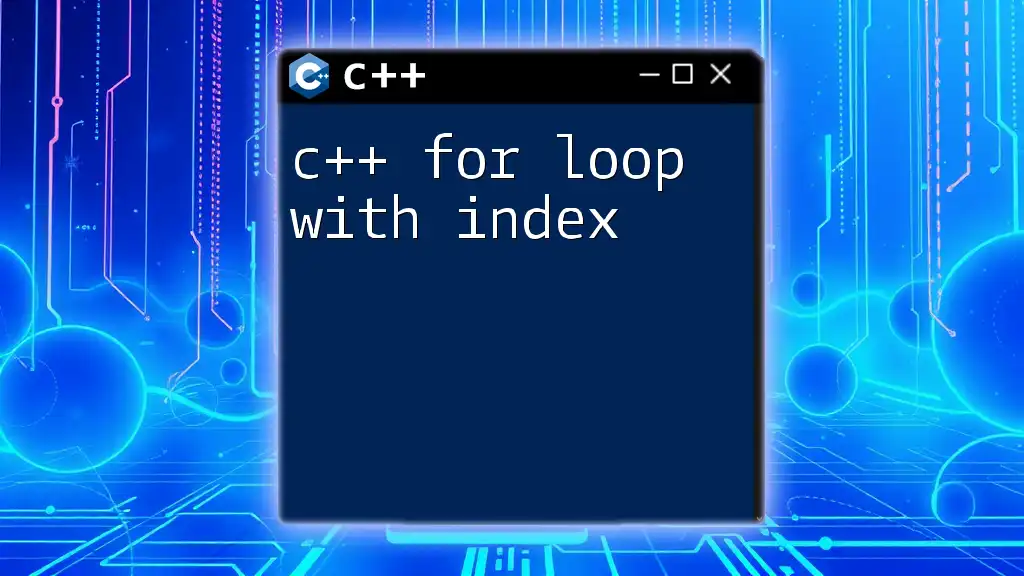
Advanced Use-Cases for For Loops
Using For Loops with Arrays
`For` loops shine when iterating through arrays. They allow efficient traversal of array elements. Here’s how you can iterate through an integer array:
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " "; // Accessing array elements
}
In this case, the loop accesses each index of the array to print its value, demonstrating a straightforward and effective way to manipulate array data.
For Loop with Vectors
Utilizing `for` loops with vectors from the C++ Standard Library is equally as effective. Here’s an example of iterating through a vector:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int i = 0; i < vec.size(); i++) {
std::cout << vec[i] << " "; // Accessing vector elements
}
In this case, `vec.size()` dynamically retrieves the size of the vector, allowing the loop to adapt to different vector sizes easily.
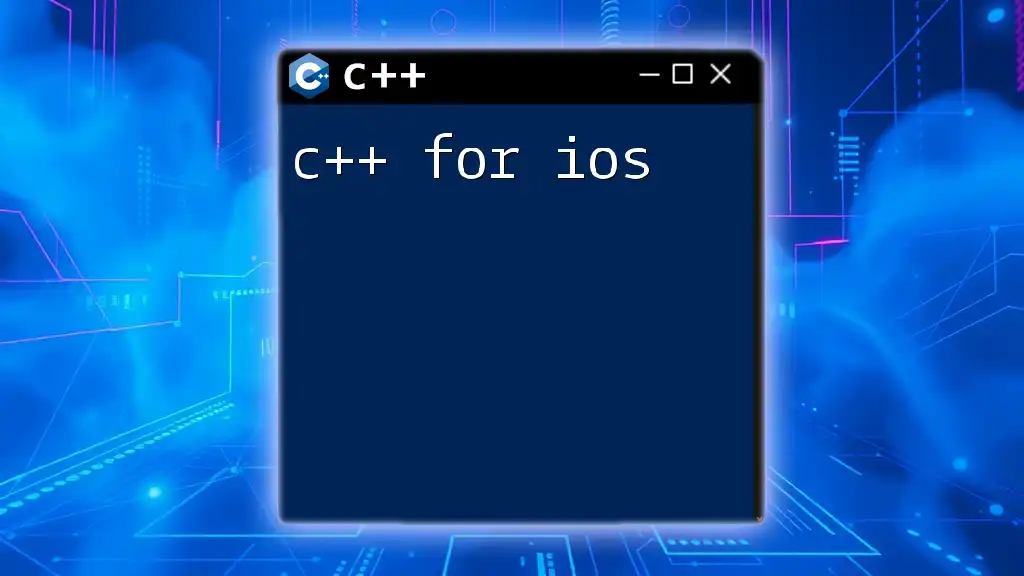
Conclusion
In this comprehensive guide, we've explored the mechanics of the C++ for in loop, including its syntax, components, and practical applications. By understanding how to properly utilize `for` loops, you can create efficient and reliable code structures.
Practice is key in mastering loops. Experiment with different scenarios, utilize both simple and nested loops, and pay close attention to common pitfalls. The more you work with `for` loops, the more intuitive they will become in your programming toolkit.
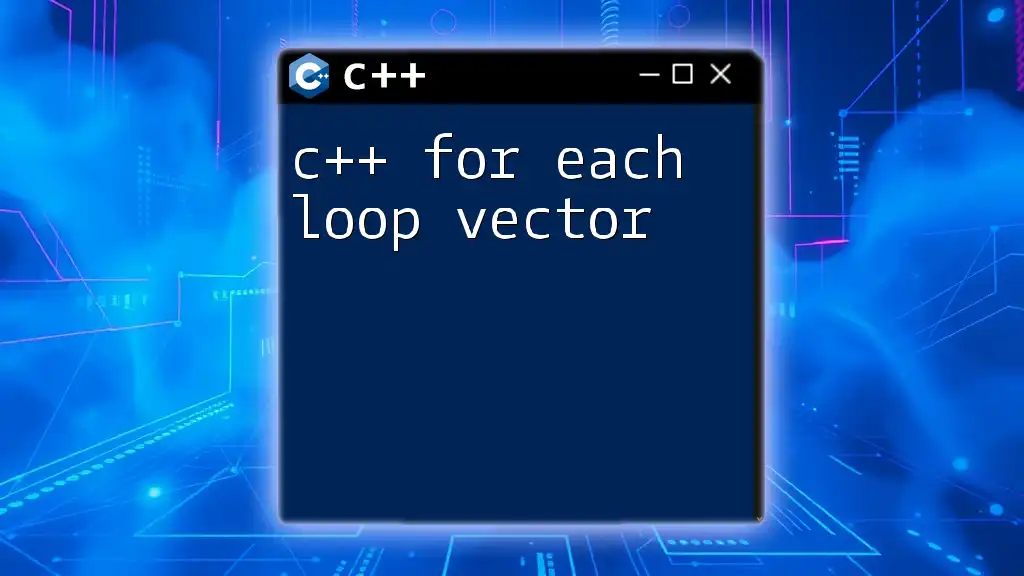
Additional Resources
For further reading and in-depth exploration of the `for` loop in C++, consider checking out video tutorials and specific programming books focused on C++. Engaging with online courses can also enhance your understanding and application of these essential programming constructs.