The C++ range-based for loop (often referred to as the "for each" loop) provides a simple and concise way to iterate over elements in a vector.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array that can resize itself to accommodate new elements. Unlike regular arrays, vectors automatically handle memory management, making them more flexible. A vector can grow and shrink as needed, allowing developers to avoid issues related to fixed-size arrays. This feature is particularly useful in situations where the number of elements is uncertain.
Why Use Vectors?
Vectors are preferred over arrays for several reasons:
- Automatic Memory Management: Vectors automatically allocate and deallocate memory, reducing the risk of memory leaks and segmentation faults.
- Support for STL Algorithms: C++ offers a rich set of Standard Template Library (STL) algorithms that work seamlessly with vectors.
- Ease of Use: Vectors come with built-in functions for adding, removing, and accessing elements, making them more user-friendly.
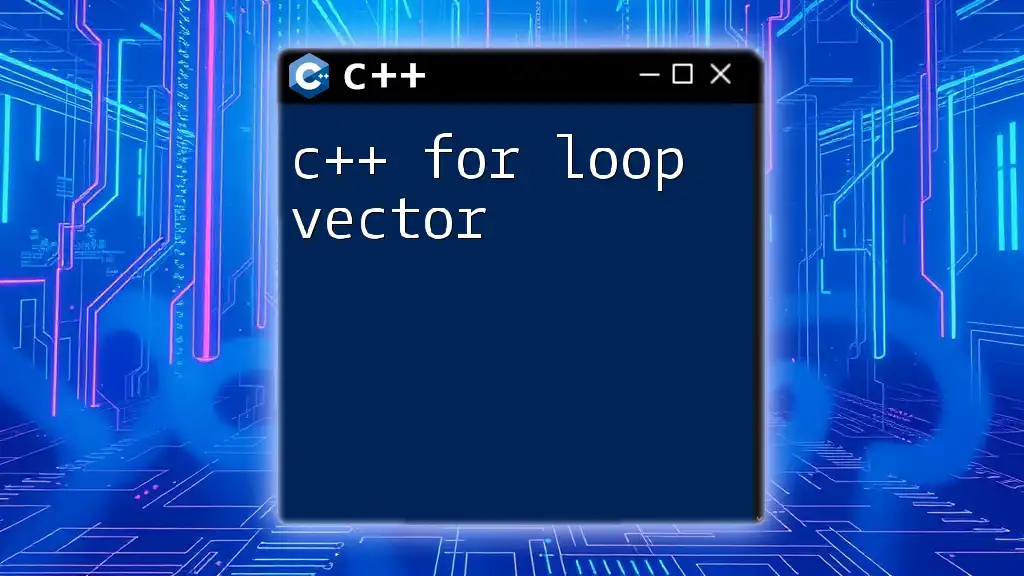
The For Each Loop in C++
Understanding the For Each Loop
The for each loop is a modern C++ construct that simplifies the iteration over collections, such as vectors. This loop allows you to access each element without worrying about the iteration logic or managing index variables. Using a for each loop improves code readability and reduces the risk of errors in iteration logic.
Syntax of the For Each Loop
The syntax of the for each loop is straightforward. It can be summarized as follows:
for (auto &element : container) {
// Code to execute
}
Here, `auto &element` is a reference to the elements in the `container`. By using `auto`, you allow the compiler to deduce the type of the elements automatically.
Key Terminologies
- Auto keyword: Automatically deduces the type of the variable, making your code more flexible.
- Range-based for Loop: A feature that iterates through the elements in a defined range.
- Element: Each individual item in the container.
- Container: A data structure, like a vector, that holds multiple elements.
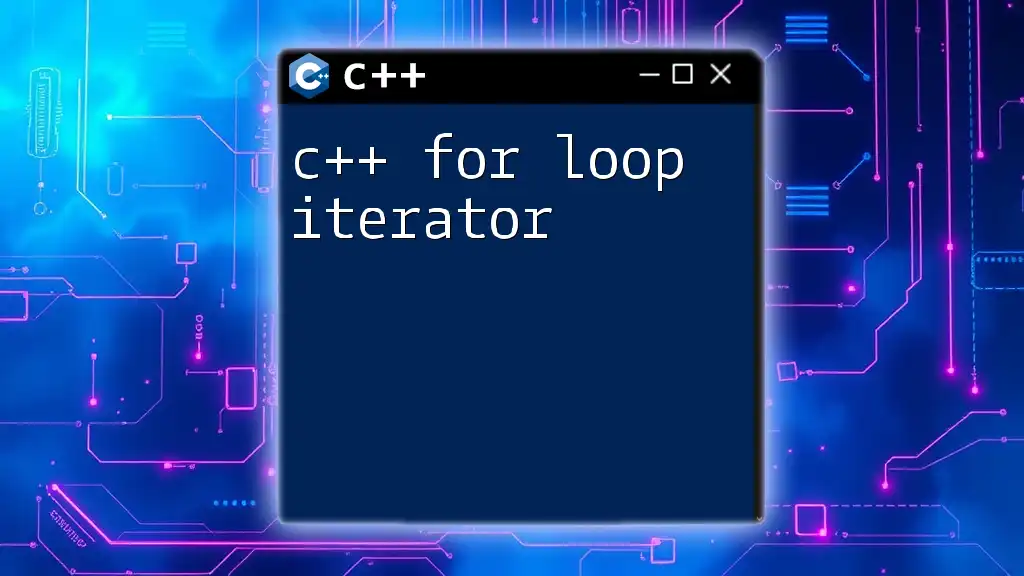
Using For Each Loop with Vectors
Basic Example of For Each Loop with Vectors
A simple example demonstrates how to use the for each loop with a vector of integers:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto number : numbers) {
std::cout << number << std::endl;
}
return 0;
}
In this code, we declare a vector called `numbers` and initialize it with integers. The for each loop prints each number in the vector. The output will be:
1
2
3
4
5
This example shows how effortlessly you can iterate through a collection using the for each loop.
Modifying Elements Using For Each Loop
To modify elements directly within the vector while using a for each loop, it is essential to use references. Here's how you can do it:
for (auto &number : numbers) {
number *= 2; // doubling each number
}
By using `auto &number`, each element is modified in-place. After this operation, the elements in `numbers` become `{2, 4, 6, 8, 10}`.
Using Const to Prevent Modification
If you want to iterate through the vector without modifying its elements, you can use `const`:
for (const auto &number : numbers) {
std::cout << number << std::endl; // this cannot modify 'number'
}
Using `const` ensures that the loop does not alter the elements inside the vector.
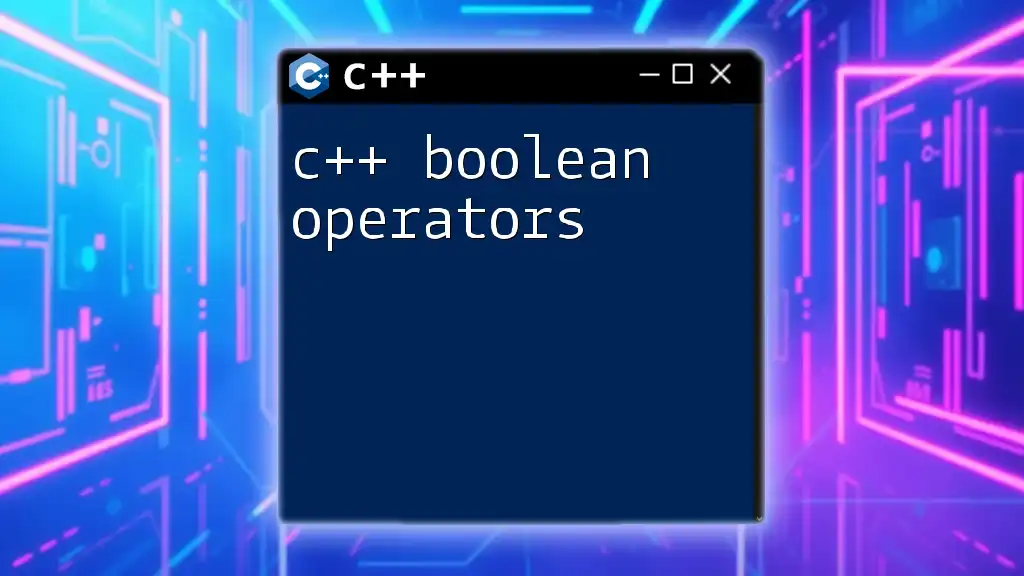
Advanced Usage of For Each Loop with Vectors
Nested For Each Loops
Sometimes, you may work with a vector of vectors (also known as a 2D vector). In such cases, you can nest for each loops. Here is an example:
std::vector<std::vector<int>> matrix = {{1, 2}, {3, 4}};
for (const auto &row : matrix) {
for (const auto &element : row) {
std::cout << element << " ";
}
std::cout << std::endl;
}
This code will output:
1 2
3 4
Each row is iterated through with an inner loop, printing the elements in a structured manner.
Combining For Each Loop with Standard Algorithms
You can also leverage the power of the C++ STL algorithms in conjunction with for each loops. A common case is utilizing `std::for_each`, which applies a specified operation to each element in the vector:
#include <algorithm>
std::for_each(numbers.begin(), numbers.end(), [](int &n) { n += 5; });
In this example, a lambda function adds 5 to each element in the vector. After executing this code, the `numbers` vector will hold the values `{7, 9, 11, 13, 15}`. This technique encapsulates complex logic in a concise manner.
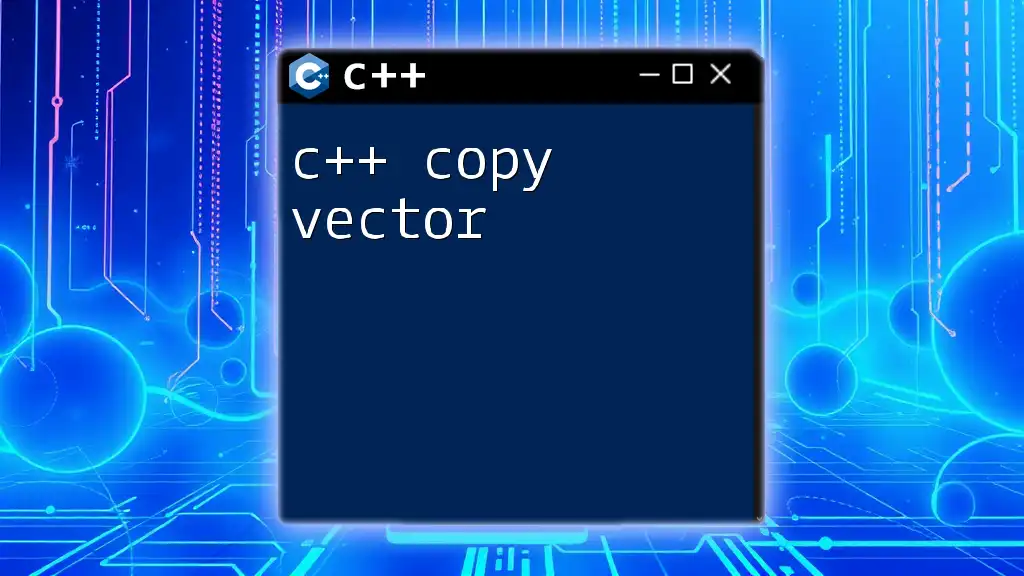
Common Mistakes and Best Practices
Common Errors When Using For Each Loops with Vectors
When working with for each loops, avoid these common pitfalls:
- Misunderstanding Element Types: It is crucial to ensure the correct type of element reference is used to prevent type mismatches.
- Forgetting to Use Const: Omitting `const` in your loop can lead to unintended modifications, especially in read-only situations.
- Modifying Elements Intentionally or Unintentionally: Ensure you understand the implications of using references to prevent accidental changes.
Best Practices
Adhering to best practices can further enhance your coding proficiency:
- Always prefer auto to enhance code flexibility and improve maintainability.
- Use references when you want to modify elements directly while using for each loops.
- Avoid complex expressions inside the loop to keep your code readable.
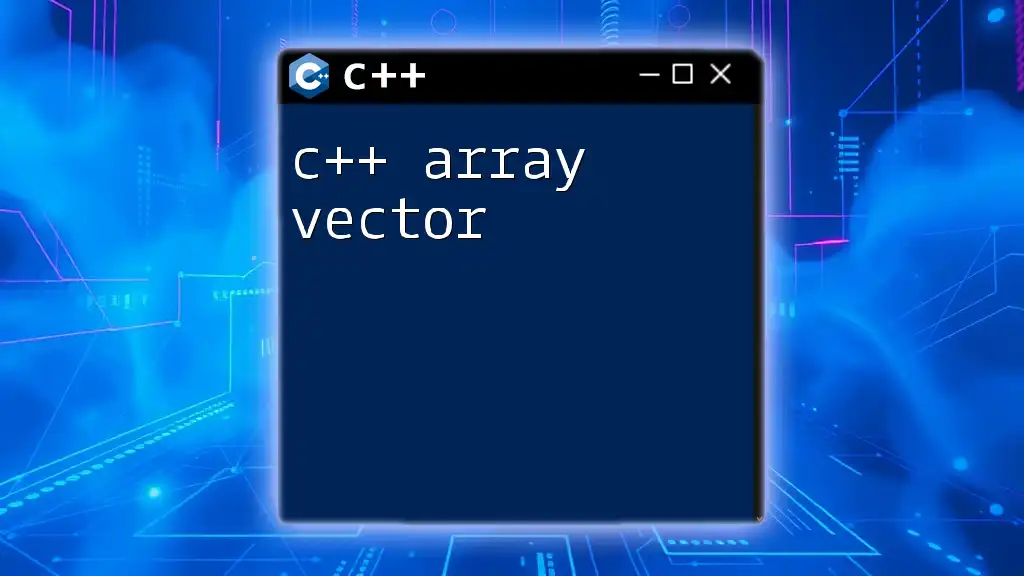
Conclusion
Using the C++ for each loop vector significantly simplifies the process of iterating through collections, making your code cleaner and less error-prone. By leveraging this technique, you can enhance your programming efficiency while gracefully managing collections of data. As you practice and implement these concepts in your code, you'll become more proficient and confident in your C++ skills.
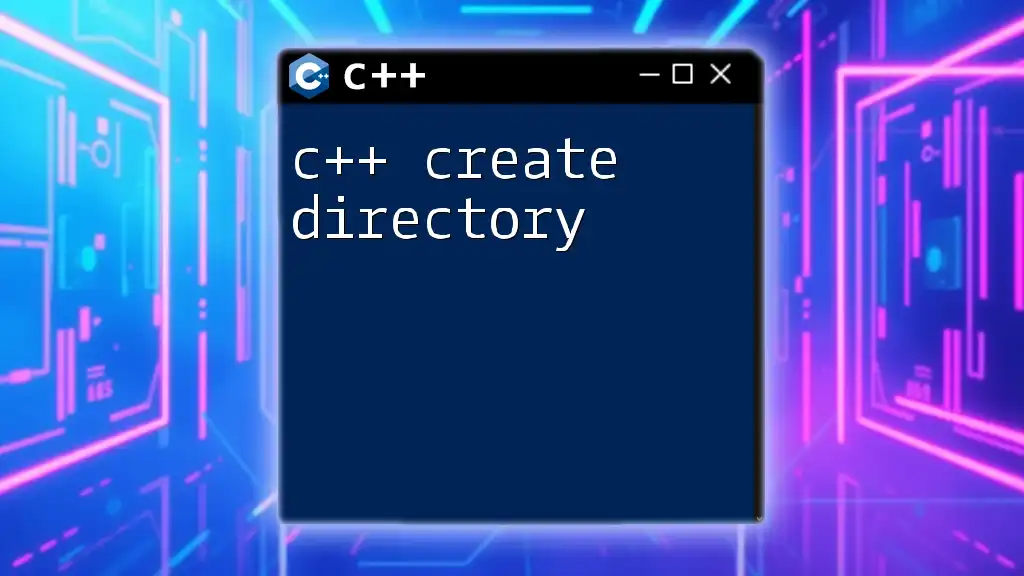
Additional Resources
To further your understanding and capabilities with C++, consider exploring recommended books and online courses that delve deeper into C++ concepts and the powerful STL. Visit the official C++ documentation for comprehensive insights into standard libraries and best practices.