In C++, you can combine two vectors using the `insert` function along with an iterator to append the elements of one vector to another. Here's a code snippet demonstrating this:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
vec1.insert(vec1.end(), vec2.begin(), vec2.end());
for (int num : vec1) {
std::cout << num << " ";
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is part of the Standard Template Library (STL) and represents a dynamic array that can grow or shrink in size. Unlike static arrays, vectors can manage their own memory and allow for efficient access, insertion, and deletion of elements.
Basic Operations on Vectors
Working with vectors involves several fundamental operations that enable you to manipulate the contents effectively:
-
Declaring and initializing vectors can be done easily with syntax like:
std::vector<int> numbers; // An empty vector std::vector<int> numbers = {1, 2, 3}; // A vector with initial values
-
Adding elements can be achieved using the `push_back()` method:
numbers.push_back(4); // Adds 4 to the end
-
Removing elements can be handled with `pop_back()` or `erase()`:
numbers.pop_back(); // Removes the last element
-
Accessing elements can be performed using the index operator:
int first = numbers[0]; // Access the first element
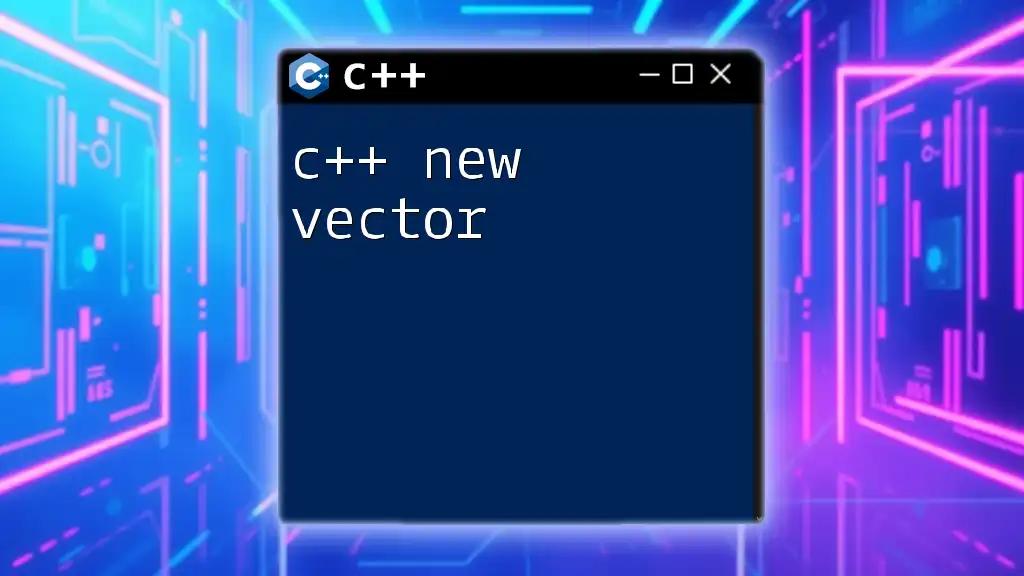
Combining Two Vectors in C++
Why Combine Vectors?
Combining vectors can be valuable in various scenarios such as merging datasets, flattening structures, or transforming data for analysis. By using vectors, you ensure memory flexibility and efficiency, making operations on collections of data straightforward.
Methods to Combine Vectors
Using `insert()` Method
The `insert()` method is a convenient way to append elements from one vector to another.
Syntax:
vector.insert(position, first, last);
This syntax allows you to specify the insertion position and the range of elements to be inserted.
Code Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
vec1.insert(vec1.end(), vec2.begin(), vec2.end());
for (int i : vec1) {
std::cout << i << " ";
}
return 0;
}
In this example, we append the elements of `vec2` at the end of `vec1`. The `begin()` and `end()` functions provide iterators that signify the starting and ending points of `vec2`.
Using `std::copy()` Function
Another powerful approach to combine vectors is using `std::copy()` from the `<algorithm>` library, which can provide greater flexibility.
Code Example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::vector<int> combined(vec1.size() + vec2.size());
std::copy(vec1.begin(), vec1.end(), combined.begin());
std::copy(vec2.begin(), vec2.end(), combined.begin() + vec1.size());
for (int i : combined) {
std::cout << i << " ";
}
return 0;
}
This method first creates a new vector, `combined`, large enough to hold both `vec1` and `vec2`. The `std::copy()` function fills this new vector accordingly.
Using `std::vector::assign()`
You can also leverage the `assign()` method to fill a vector with another's contents.
Code Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::vector<int> combined;
combined.reserve(vec1.size() + vec2.size()); // Reserve memory upfront
combined.insert(combined.end(), vec1.begin(), vec1.end());
combined.insert(combined.end(), vec2.begin(), vec2.end());
for (int i : combined) {
std::cout << i << " ";
}
return 0;
}
By using `reserve()`, you optimize memory allocation, which can be especially useful when dealing with large datasets.
Performance Considerations
When you choose to combine two vectors, it's essential to consider the underlying performance implications. The `insert()` method can be relatively slower if the vector needs to resize. On the other hand, using `std::copy()` or `assign()` with proper memory reservations can lead to more efficient operations. Always strive to minimize the number of times the vector grows by preallocating memory.
Best Practices for Combining Vectors
Avoiding Redundant Copies
To improve performance, especially in larger applications, it is crucial to avoid unnecessary copying of vectors. Always prefer methods that allow for direct insertion or merging without creating intermediate copies whenever feasible.
Ensuring Type Safety
Maintain consistency in vector types when combining them. Mixing different types will lead to compilation errors. Here's an example of what to avoid:
std::vector<int> vec1 = {1, 2, 3};
std::vector<float> vec2 = {4.1f, 5.2f, 6.3f}; // Different type
// std::vector<float> result = vec1 + vec2; // This will fail
Maintaining Readability
Code readibility is vital when combining vectors. Ensure you comment thoroughly your code and organize it logically for others (and your future self) to understand. Here’s a quick comment guideline:
// Combine vec1 and vec2 into combined
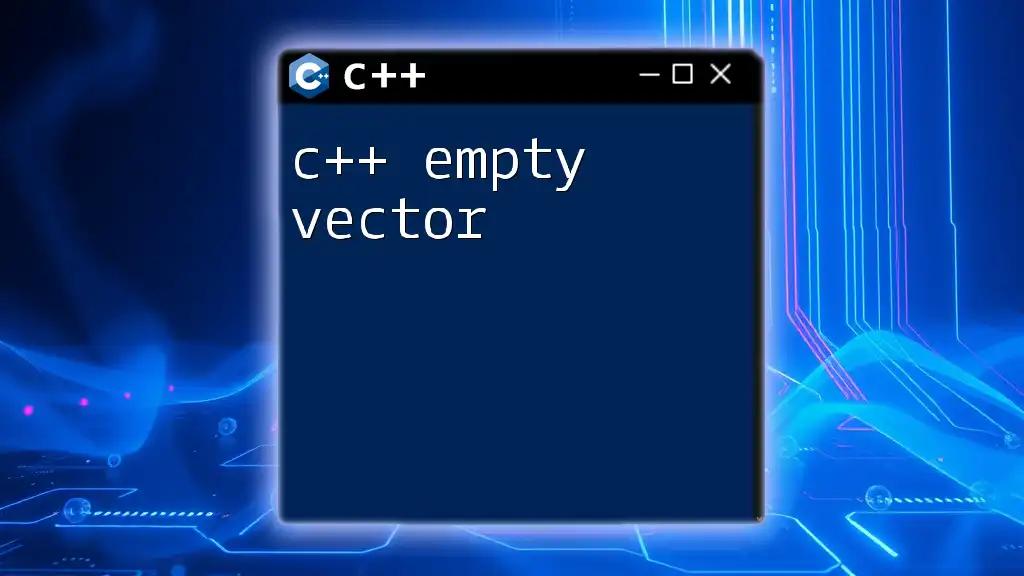
Conclusion
Combining vectors in C++ offers robust tools that are simple to implement and provide considerable flexibility. By understanding the various methods available, you can enhance the performance and efficiency of your applications. As you continue to explore C++, remember that experimentation is a key part of the learning process. Dive into more complex scenarios and enjoy the versatility that vectors provide!
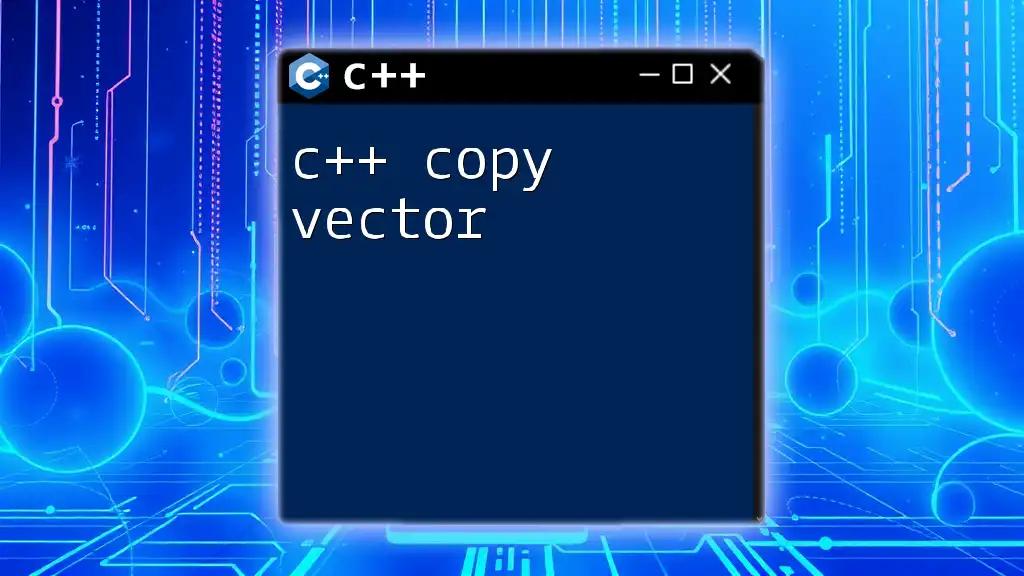
Additional Resources
To further your understanding, consider exploring additional literature on C++ vectors and STL algorithms. Engaging in online communities or enrolling in C++ courses can also significantly boost your programming prowess.