C++ contracts, introduced with the C++20 standard, are a way to enforce preconditions, postconditions, and invariants on functions to ensure correctness in your code.
Here's a simple example using C++ contracts:
#include <concepts>
#include <iostream>
void processValue(int value) requires (value > 0) {
// Regular processing here
std::cout << "Processing value: " << value << std::endl;
}
int main() {
processValue(5); // This will work
// processValue(-1); // Uncommenting this line will cause a compilation error due to the contract
return 0;
}
Understanding C++ Contracts
C++ contracts are a powerful feature introduced in C++20 that help ensure the correctness and reliability of code. They promote the idea of contract programming, which is based on the concept of defining clear contractual obligations for functions and classes through preconditions, postconditions, and invariants. This formal specification allows developers to specify what is expected from their code and helps catch errors during the development phase.
Key Concepts
Preconditions are conditions that must be true when a function is called. If the preconditions are not met, the function may exhibit undefined behavior. For example, a function that calculates the square root of a number should not receive a negative input, as this is an invalid state.
Postconditions are conditions that must be true when a function completes its execution. These serve as assurances that certain properties will hold after the function has finished running. Continuing with the previous example, a postcondition for a square root function should ensure that the result is always non-negative.
Invariants are conditions that always hold true for the lifetime of an object. They are crucial for maintaining the consistency of class instances. For example, a bank account class should always maintain a non-negative balance—this is an invariant that must be respected throughout the object's use.
Benefits of Using Contracts
-
Cleaner Code: By defining clear contracts, code becomes self-documenting. When a developer reads a function with preconditions and postconditions, they immediately understand how to properly use that function.
-
Improved Debugging and Maintenance: Contracts can significantly reduce the time spent debugging. If a contract is violated, the obvious feedback directs attention to specific parts of the code that need correction, making maintenance easier.
-
Enhanced Code Reliability: Contracts contribute to building robust software. When developers adhere to contracts, they actively defend against potential errors, thus leading to greater reliability in the software products.

The Components of C++ Contracts
Preconditions
Preconditions are fundamental in ensuring that the environment for a function is correctly set. They can be implemented easily using assertions in C++. For example, consider a function designed to set a person's age.
#include <cassert>
void setAge(int age) {
assert(age >= 0); // Preconditions: age must be non-negative
// Function Implementation
}
In this code snippet, the assertion checks whether the age is non-negative before proceeding with the function execution. If the assertion fails, the program will terminate, indicating that the function was misused.
Postconditions
Postconditions reinforce the expectations after a function has executed. They guarantee certain conditions about the outcome of the function. For instance, let’s examine a function that calculates the square of a number.
#include <cassert>
int square(int number) {
int result = number * number;
assert(result >= 0); // Postconditions: the result must be non-negative
return result;
}
Here, the postcondition guarantees that the result of the squaring operation remains non-negative, which it logically should be. If for any reason a precondition was violated or if unexpected behavior occurs, the contract helps identify discrepancies immediately.
Invariants
Invariants are especially relevant within class definitions. They ensure that certain properties of class instances remain consistent throughout their life. Consider the following `BankAccount` class:
class BankAccount {
private:
double balance;
public:
BankAccount(double initialBalance) : balance(initialBalance) {
assert(balance >= 0); // Invariant: balance cannot be negative
}
void Deposit(double amount) {
assert(amount > 0); // Preconditions: amount must be positive
balance += amount;
assert(balance >= 0); // Postconditions: balance must remain non-negative
}
};
In this example, the constructor of the `BankAccount` class checks the initial balance to ensure no negative values are allowed. Every time money is deposited, the precondition ensures that a positive amount is being added, and a postcondition checks that the balance remains valid afterward.
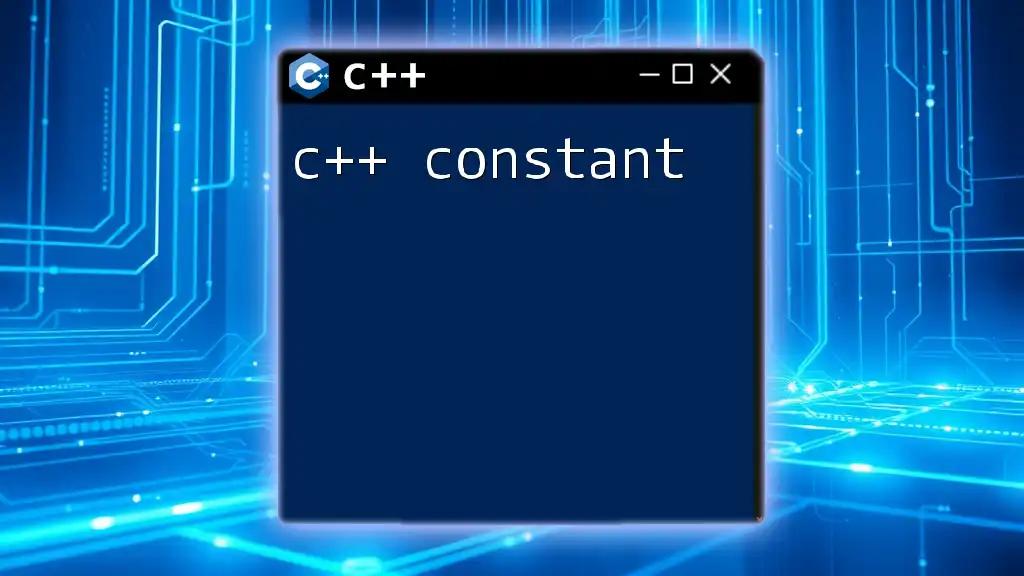
Implementing Contracts in C++
Syntax Overview
With the introduction of Contracts in C++20, developers have access to a more formal and structured syntax meant for defining specifications of functions and classes. Key keywords include `requires`, `ensures`, and `assert`, each serving distinct roles in the context of contracts.
Using `requires` Clauses
The `requires` clause allows developers to specify conditions that must hold for template programming. This feature makes it easier to define constraints on template parameters, ensuring only valid types are passed to templates.
template<typename T>
requires std::integral<T>
T add(T a, T b) {
return a + b;
}
This example defines a template function `add` that only compiles if `T` is an integral type. If a non-integral type is provided, a compile-time error ensures that only valid operations are considered.
Handling Contract Violations
When contracts are violated, it’s important to have strategies in place for handling such occurrences gracefully. One way to achieve this is through exceptions. Rather than relying solely on assertions, which can terminate a program, a more user-friendly approach could look like this:
#include <stdexcept>
void setAge(int age) {
if (age < 0) throw std::invalid_argument("Age cannot be negative");
// Function Implementation
}
In this snippet, attempts to set a negative age will trigger an exception. This prevents your application from crashing and provides meaningful feedback to the user regarding the nature of the error.

Best Practices for Using Contracts
When implementing C++ contracts, consider the following best practices to maximize the benefits:
-
When to Use Contracts: Use contracts primarily for public interfaces where incorrect usage can lead to severe issues. Interfaces to libraries and APIs should always clearly define their contracts.
-
Avoiding Misuse of Contracts: Contracts should not replace regular error handling but complement it. They are designed to prevent bad state rather than to recover from it.
-
Ensuring Performance and Readability: While contracts add robustness to code, they can also introduce overhead. Be sure to weigh the benefits against performance issues, and aim for a balance between clarity and speed.

Tools and Libraries for Contract Programming in C++
The support for contracts in C++ is becoming more robust with advancements in compilers. As of now, many latest versions of GCC and Clang have added compatibility for C++20 features, including contracts. Additionally, several libraries facilitate contract programming:
-
Boost.Contract: An external library offering a flexible and rich interfaces for contract programming.
-
Other Notable Libraries or Tools: Keep an eye on community-driven libraries that might be developed to enhance contract programming capabilities as C++ evolves further.

Conclusion
C++ contracts present a paradigm shift in ensuring code correctness and reliability, offering a structured approach to define functional expectations. By leveraging preconditions, postconditions, and invariants, developers can create more robust software that is easier to maintain and debug. As the C++ landscape continues to evolve, understanding and effectively implementing C++ contracts will become even more essential for quality software development.