In C++, ASCII characters can be represented using their integer values, allowing you to manipulate and display characters easily through their corresponding ASCII codes.
Here's an example:
#include <iostream>
int main() {
char letter = 65; // ASCII value for 'A'
std::cout << "The character for ASCII 65 is: " << letter << std::endl;
return 0;
}
Understanding ASCII Values in C++
What are ASCII Values?
ASCII (American Standard Code for Information Interchange) is a character encoding scheme that represents text in computers and other devices that use text. The standard ASCII set includes 128 characters, ranging from 0 to 127, where each number corresponds to a specific character, action, or symbol. For instance, the letter “A” corresponds to 65, while the symbol ”@” corresponds to 64.
Understanding ASCII values is crucial for C++ developers, as it lays the foundation for effectively managing text and character data within their programs.
Representing ASCII in C++
In C++, characters are typically represented using the `char` datatype. Each `char` in C++ can store a single ASCII character. The relationship between a character and its ASCII value is straightforward:
char character = 'A'; // ASCII value is 65
When you assign a character to a variable defined as type `char`, you are implicitly using its ASCII value behind the scenes.
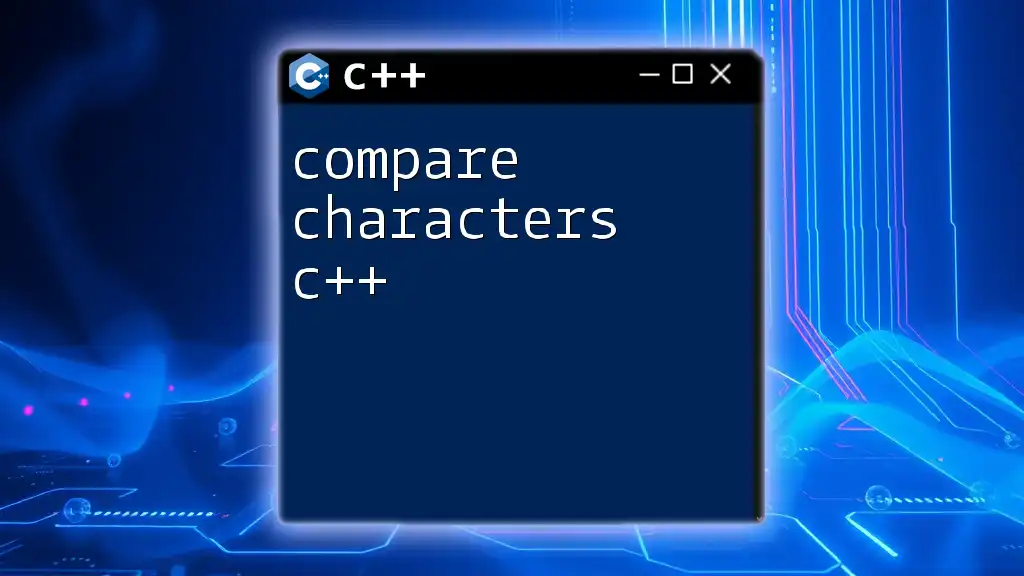
Using ASCII Values in C++
Working with Characters
When working with characters, you can easily access their ASCII values. This can be particularly useful when you want to manipulate characters or convert them based on their ASCII values.
Consider this example:
char letter = 'C'; // Here, 'C' has an ASCII value of 67.
int asciiValue = static_cast<int>(letter); // Convert char to int
In the above code snippet, `static_cast<int>(letter)` converts the character `C` to its corresponding ASCII value, which is 67. Using `static_cast` allows you to perform type conversion in a safe and clear manner.
ASCII Codes and Characters Table
A reference to common ASCII codes is beneficial for quick lookup. Here are some common ASCII characters and their corresponding values:
- 'A' - 65
- 'B' - 66
- 'C' - 67
- 'Z' - 90
- 'a' - 97
- 'z' - 122
- '0' - 48
- '1' - 49
- '@' - 64
- ' ' (space) - 32
This reference serves as a handy tool when coding or debugging.
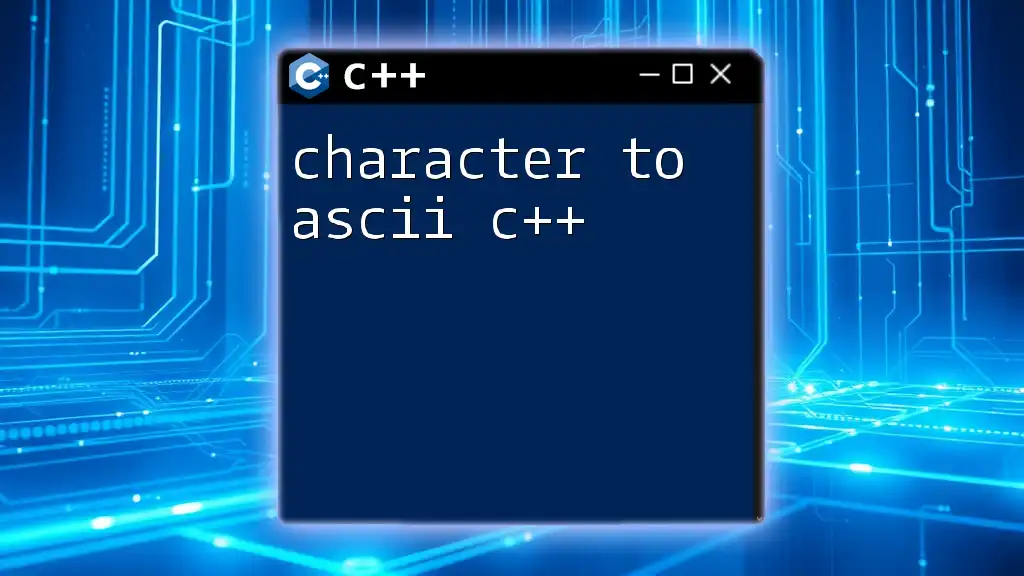
Encoding and Decoding ASCII in C++
Encoding Characters to ASCII
Encoding characters into their respective ASCII values involves straightforward conversions. The process is essential, especially when working with strings or text files.
Here’s an example that encodes a string into ASCII:
std::string text = "Hello";
for (char c : text) {
std::cout << c << ": " << static_cast<int>(c) << std::endl;
}
In the code above, each character in the string "Hello" is printed alongside its ASCII value. The loop goes through each character, converting it into its ASCII representation, thus providing clear insights into how characters are stored in memory.
Decoding ASCII Values back to Characters
Decoding ASCII values back into characters is equally simple, allowing the character representation to be easily restored from its numeric form. Here’s an example demonstrating this conversion:
int asciiValue = 65;
char character = static_cast<char>(asciiValue);
std::cout << "Character for ASCII 65 is: " << character << std::endl;
In this snippet, the integer 65 is converted back into the character A using `static_cast<char>`, which effectively reverses the encoding process.
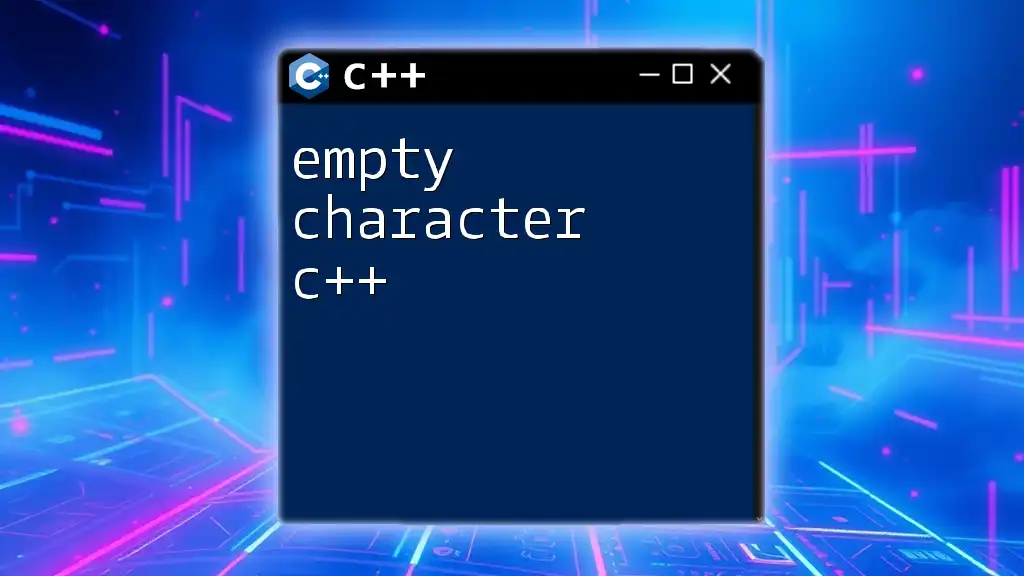
Practical Applications of ASCII in C++
Implementing Unicode with ASCII
While ASCII is a foundational character set, it’s limited to 128 characters. In modern applications, developers often face the need to handle a wider range of characters, which can be accomplished through Unicode. Understanding ASCII values is important as they serve as the building blocks for more complex character encoding systems.
ASCII in String Manipulation
Manipulating strings based on ASCII values can open up numerous applications, from simple text transformations to complex data processing tasks. For example, you might want to shift each character in a string by a specific number of positions in the ASCII table:
std::string str = "abc";
for (auto &c : str) {
c = static_cast<char>(static_cast<int>(c) + 1); // Shift characters
}
In the example above, each character in the string abc is incremented by one in the ASCII table, yielding bcd. This showcases how ASCII values can be manipulated to transform data programmatically.
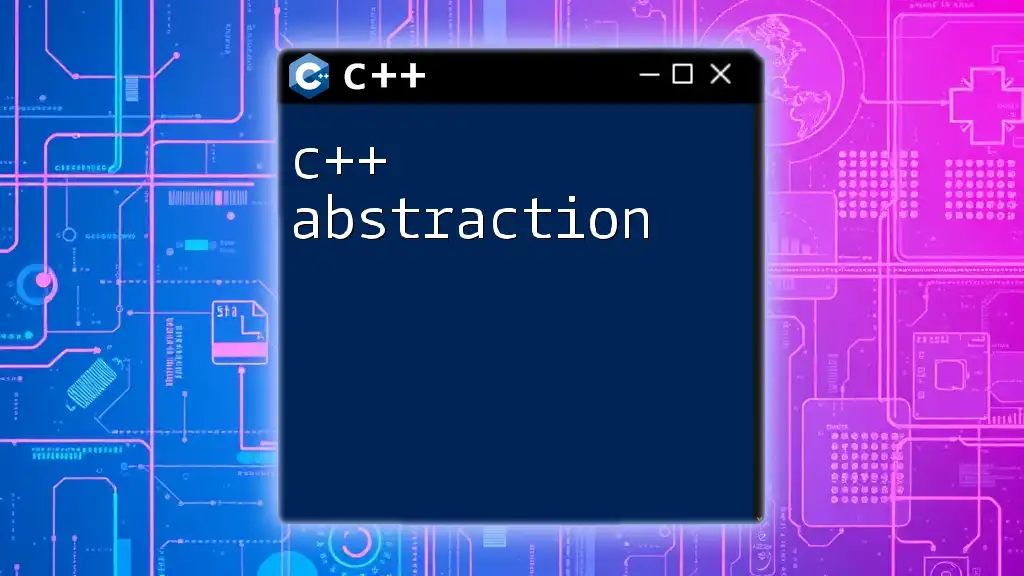
Conclusion
In summary, understanding C++ characters and ASCII is essential for any C++ developer. It not only forms the foundation for how characters are represented but also facilitates various operations involving text manipulation, encoding, and decoding. By experimenting with ASCII values in your projects, you can harness their power to enhance your programming capabilities. As you continue your journey with C++, remember the significance of ASCII in effectively managing text within your applications.
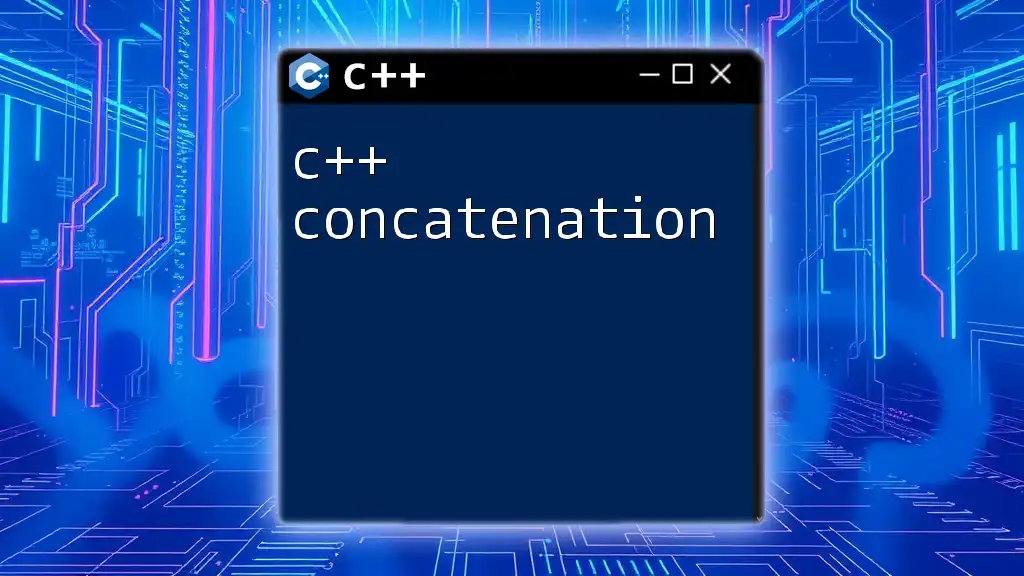
Additional Resources
For an in-depth exploration of ASCII and its role in C++ programming, consider the following resources:
- Official C++ documentation
- Online tutorials focusing on character encoding and manipulation
- Community forums for discussion and troubleshooting on ASCII-related topics
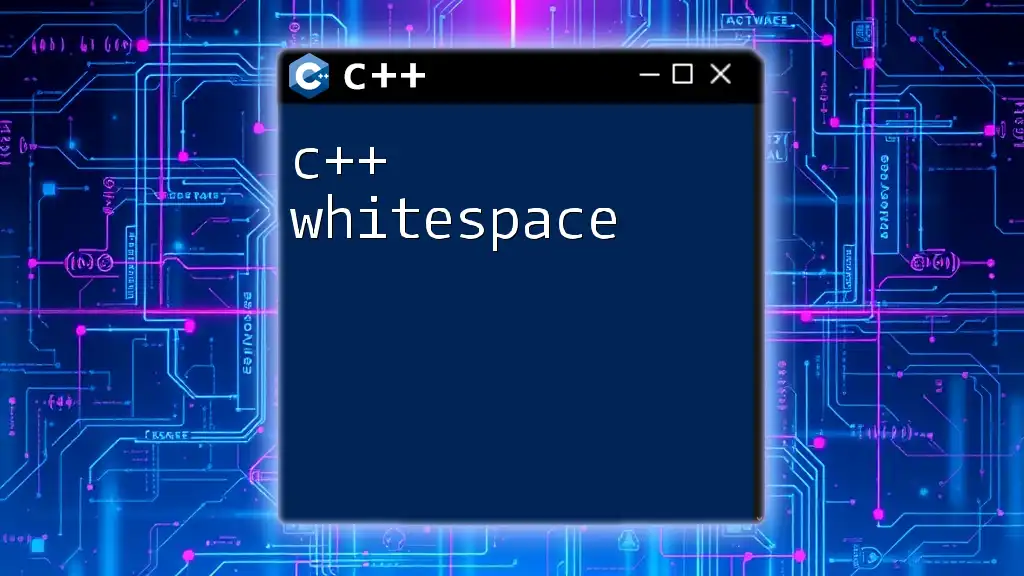
FAQs about ASCII in C++
Common Queries About ASCII Handling in C++
-
What is the highest ASCII value I can use in C++?
The highest ASCII value is 127, which corresponds to the delete control character. However, extended ASCII can include values up to 255 for various symbol representations in certain character sets. -
Are there any special characters in ASCII?
Yes, ASCII includes control characters (values 0-31) such as newline (10) and tab (9), which are not printable but serve critical functions in text formatting and command interpretation.