In C++, whitespace is used to separate tokens in the code and improve readability, with spaces, tabs, and newlines having no effect on the execution of the program.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // This is a line of code with whitespace
return 0; // Another line with whitespace
}
Understanding Whitespace in C++
What is Whitespace?
Whitespace refers to any character or series of characters that represent horizontal or vertical space in a C++ program, excluding visible characters. This includes space characters, tabs, newlines, and carriage returns. While these characters do not directly affect the execution of the code, they play a crucial role in how it is structured and read by both human developers and the compiler.
Importance of Whitespace
Whitespace is not just about aesthetics. Proper use of whitespace is essential for code readability, allowing developers to easily navigate, understand, and maintain the code over time. A consistent and well-structured layout using whitespace enables developers to identify blocks of code, making it easier to spot logic errors or unintended behavior. Ultimately, clean code is less prone to bugs and easier to collaborate on in team environments.
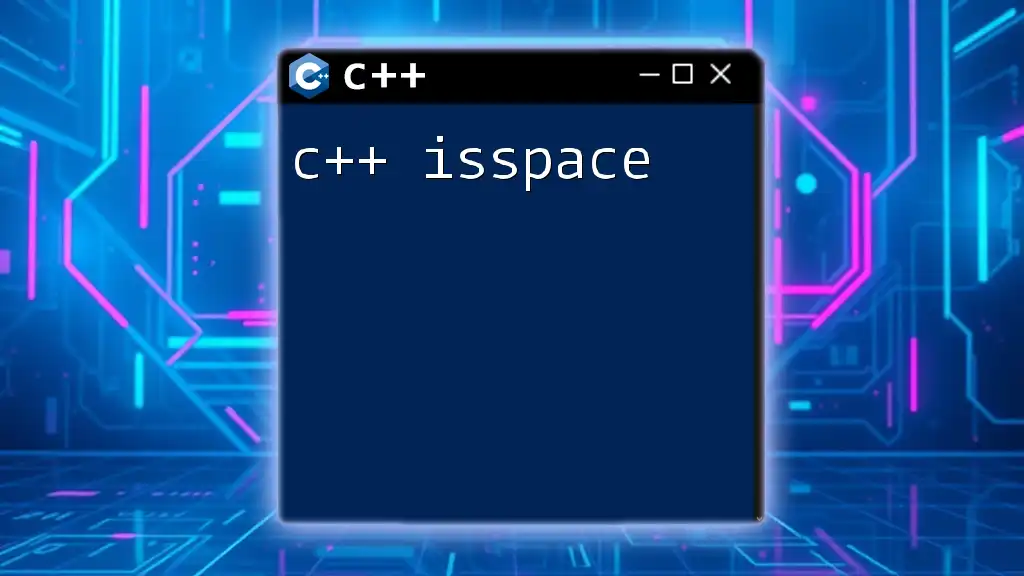
Types of Whitespace in C++
Space Characters
Space characters are the most commonly used whitespace in C++. They separate identifiers, keywords, and operators, helping to make code more readable. For example:
int a = 5; // Space between the variable type, name, and assignment
In this example, spacing enhances clarity, making it clear that `a` is an integer variable assigned a value of `5`.
Tab Characters
Tab characters serve as an integrated way to indent lines of code, which is particularly important when defining control structures and nested blocks. However, the use of tabs can be a double-edged sword. While tabs allow for a customizable width depending on individual preferences, they can lead to inconsistent formatting when viewed in different text editors. Consider the snippet below:
int b = 10; // Tab used for indentation of the variable
Using tabs or spaces consistently across a project is essential for maintainable code.
Newline Characters
Newlines signify the end of a statement and the beginning of a new one. They are indispensable in making code visually comprehensible. For instance:
int c = 15;
int d = 20; // Newline helps in clear separation of variable declarations
Without newlines to segment statements, code can quickly become chaotic and difficult to analyze.
Carriage Return Characters
While not as commonly used in C++ as in some other languages, carriage return characters (`\r`) can still be relevant, especially when dealing with different file formats or output devices. They move the cursor back to the beginning of the line without advancing to the next line. Here’s how it appears:
std::cout << "Hello World!\r"; // Carriage return usage
In this specific instance, subsequent text will overwrite what was displayed, which can create dynamic output effects.
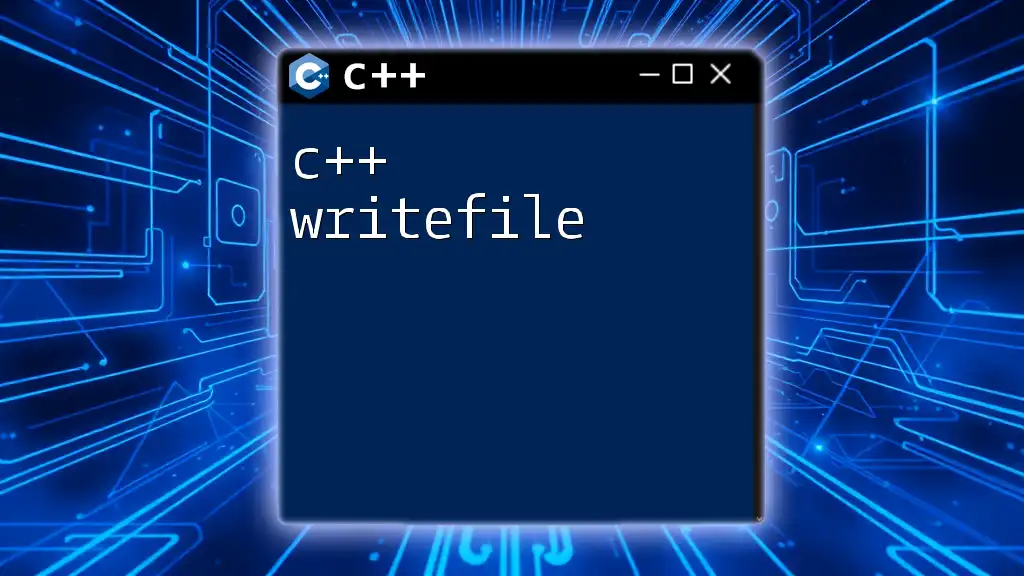
Whitespace in C++ Syntax
Variable and Function Declarations
In C++, whitespace is utilized extensively in variable and function declarations. Properly spaced declarations enhance readability, contributing to a more organized codebase. For example:
int myVariable; // Proper use of whitespace near the variable name
Neglecting whitespace in these contexts can make the code harder to parse, particularly in more complex declarations.
Control Structures and Loops
Whitespace is fundamental in control structures such as `if`, `for`, `while`, and `switch` statements. Indentation not only clarifies which lines of code belong to a control structure but also aids in modifying and debugging the code effectively. For instance:
if (a > b) {
std::cout << "A is greater!" << std::endl; // Clear indentation for the block under the 'if'
}
In the example above, the proper use of indentation makes it clear that the output statement belongs to the conditional statement.
Class and Struct Definition
When defining classes and structs, whitespace is equally important. It helps distinguish between parts of the class or struct, leading to more readable code:
class MyClass {
public:
void myFunction() {
// Function body
}
};
Well-structured use of whitespace in these structures aids other developers in quickly grasping their layout.
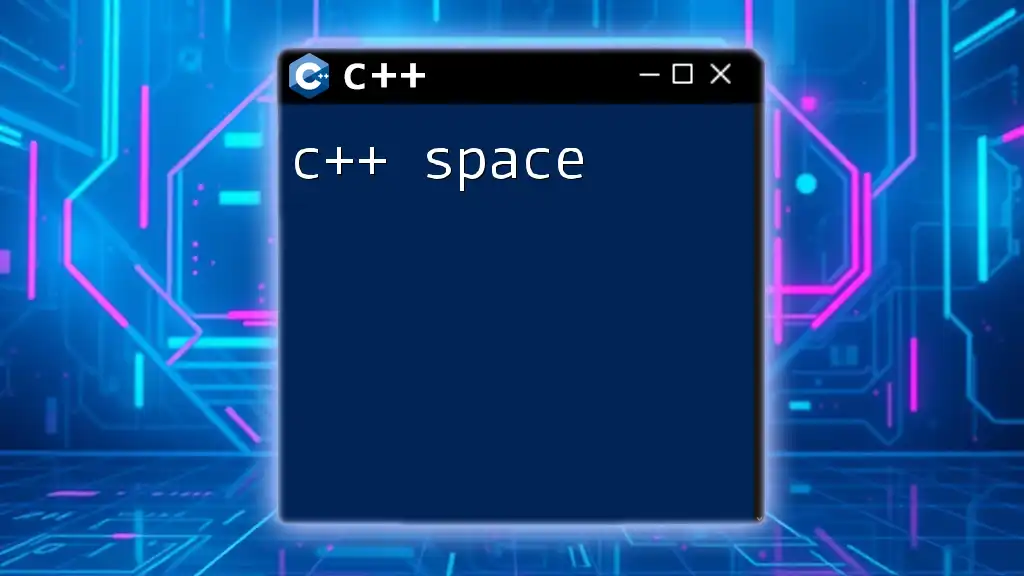
Best Practices for Using Whitespace
Consistent Indentation
One of the most critical aspects of using whitespace is maintaining consistent indentation throughout your code. Inconsistent indentation can lead to confusion and might cause logical errors. Developers often choose between using tabs or spaces for indentation; however, the key is to pick one style and stick with it across the project. Tools like `.editorconfig` can help enforce these choices.
Readable Code Blocks
Creating visually distinguishable blocks of code with whitespace is vital. Not only does it make the code clearer, but it also supports better logical separation of ideas. Use whitespace judiciously with comments to explain the purpose of complex code segments, enhancing understanding:
// Function to add two numbers
int add(int x, int y) {
return x + y; // Clear and readable code
}
Alignment in Code
Proper alignment can contribute significantly to the readability of the code. Group similar variables or functional parameters to improve clarity, as shown in this alignment practice:
int x = 5; // First element
int longerVariableName = 10; // Aligned for clarity
Such alignment helps identify patterns and relationships quickly.
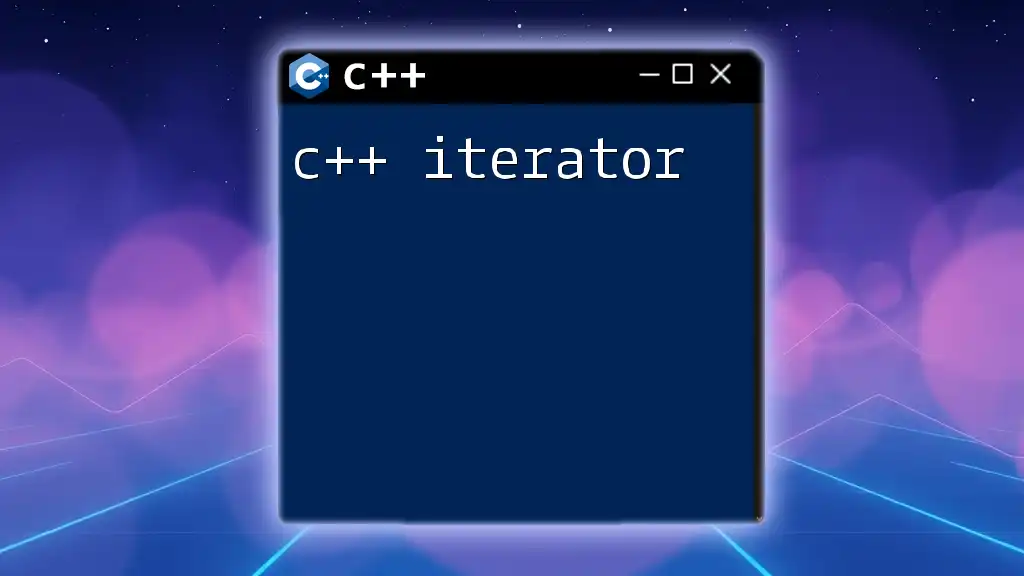
Common Mistakes to Avoid
Excessive Whitespace
While whitespace is important, excessive use of it can detract from readability. It can lead to confusion and misinterpretation of code structure. For instance:
int a = 30;
int b = 40; // Too much whitespace, can lead to frustration
Here, the excessive empty lines add nothing to understanding and can confuse a reader.
Inconsistent Use in Team Environments
In team environments, inconsistent use of whitespace can lead to chaos and diluted productivity. Establish a shared coding standard for your team, ensuring that everyone adheres to the same whitespace and indentation conventions. Using tools that enforce style guidelines can mitigate these issues.
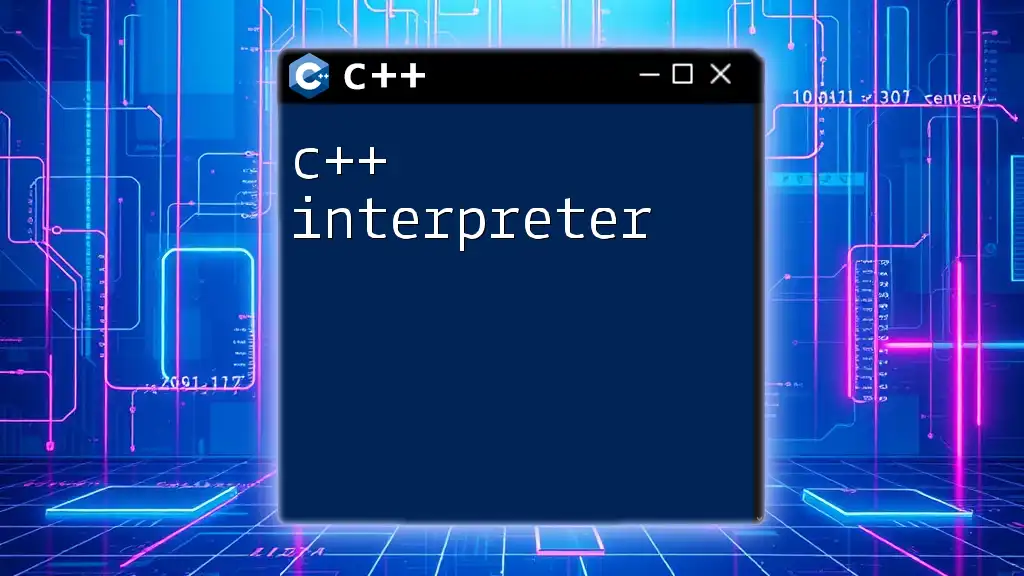
Tools and Resources for Managing Whitespace
Code Formatting Tools
Numerous code formatting tools, like Astyle and Clang-Format, allow developers to automatically format their code according to predefined styles. These tools can effectively handle whitespace management, ensuring a consistent coding style across your codebase.
IDE Features for Whitespace Handling
Most integrated development environments (IDEs) include features that assist in managing whitespace. Code linting tools can help identify whitespace issues proactively, providing feedback before changes are committed to a repository.
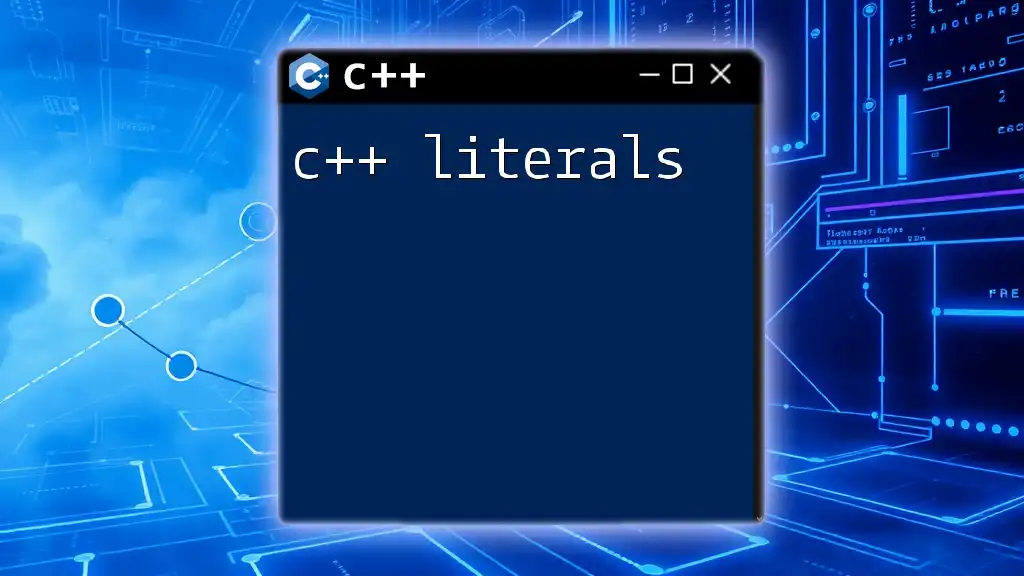
Conclusion
Whitespace in C++ is more than just empty space; it’s an essential aspect of clear, maintainable code. Understanding how to effectively utilize whitespace enhances your coding ability, fosters better collaboration, and ultimately leads to more efficient coding practices. By committing to best practices around whitespace, developers can significantly improve both their individual coding style and the quality of team projects.
Further Reading
To deepen your knowledge on coding standards and practices, consider exploring additional resources on C++ styling guides, best coding practices, and online community discussions. These materials can provide valuable insights into maintaining high standards in coding practices.