In C++, the `std::space` is a part of the standard library that allows you to define or manipulate whitespace characters, commonly used for string manipulation or parsing.
Here's a simple code snippet demonstrating how to use `std::isspace` to check if a character is a whitespace:
#include <iostream>
#include <cctype> // for std::isspace
int main() {
char ch = ' ';
if (std::isspace(ch)) {
std::cout << "The character is a whitespace." << std::endl;
} else {
std::cout << "The character is not a whitespace." << std::endl;
}
return 0;
}
Understanding C++ Space
What is C++ Space?
In programming, space refers to any blank area in code which enhances readability and affects syntax. In the context of C++, c++ space includes various forms of spacing, such as whitespace in code, memory space used during execution, and namespaces to organize code entities. Understanding how to manage these spaces is crucial for writing clean, efficient, and error-free code.
Importance of Space in C++
The incorrect use of space in C++ can lead to syntax errors that prevent code from compiling. Moreover, proper spacing enhances code readability and maintainability, making it easier for others (and future you) to understand the logic behind the code. A well-structured code with appropriate spacing helps in debugging and updating code efficiently.
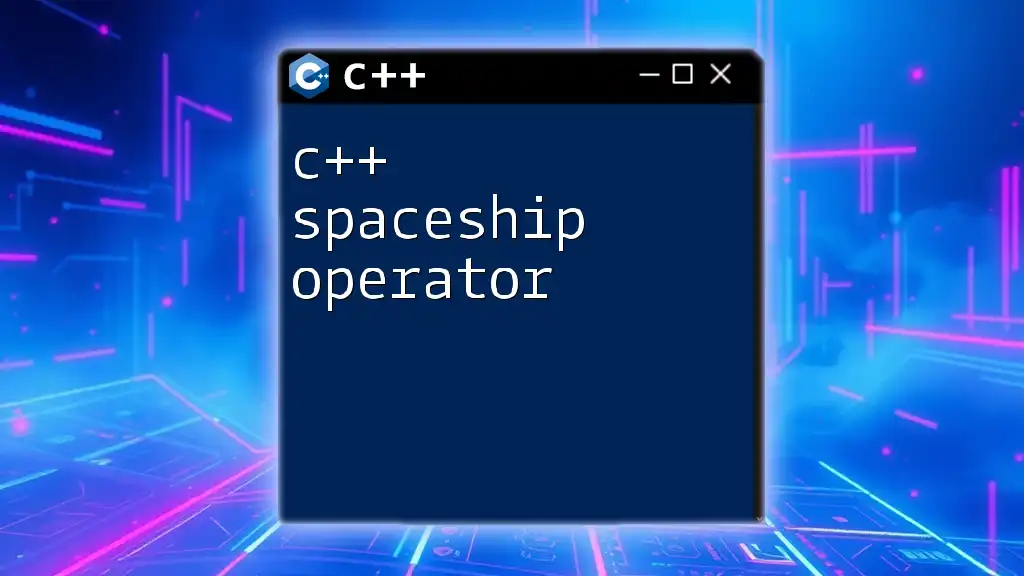
Types of Space in C++
Whitespace
Whitespace consists of areas in code that appear blank, including spaces, tabs, and new lines. These do not impact the execution of the program but are vital for enhancing readability. For example:
int main() {
int a = 10;
int b=20; // Without whitespace
int sum = a + b;
return 0;
}
In the above example, the second declaration without proper whitespace becomes visually cluttered, affecting readability.
Memory Space
In C++, memory space refers to how memory is allocated throughout the execution of a program. It's primarily divided into two types: stack and heap memory.
- Stack memory is used for static memory allocation, where the size of the structure is known at compile time. It is fast but has size limitations.
- Heap memory, on the other hand, is for dynamic memory allocation, allowing for large sizes as needed but comes with slower allocation speeds.
Visual representation can be beneficial to understand how memory space is utilized in various scenarios.
Namespace
A namespace is a declarative region that allows you to group identifiers (like variables and functions) under a name. This is especially useful for avoiding naming collisions, which can occur in larger projects.
To declare and use a namespace:
namespace MyNamespace {
void myFunction() {
// Function implementation
}
}
using namespace MyNamespace;
This code snippet shows how you can define a function within a namespace and use it without prefixing it every time.
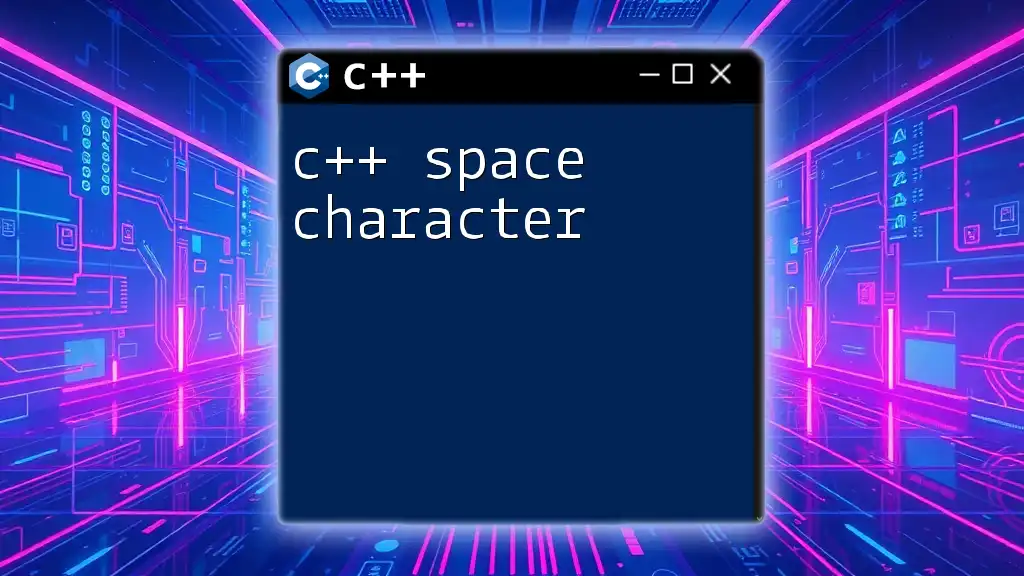
Working with Whitespace in C++
Whitespace Best Practices
Implementing best practices for whitespace involves understanding where to place spaces, tabs, and new lines. Following specific rules not only helps maintain consistency but also makes the code easier to understand.
For example, consider the difference in readability:
for (int i=0;i<10;i++) { // Poor spacing
std::cout << i;
}
for (int i = 0; i < 10; i++) { // Good spacing
std::cout << i;
}
The latter is more readable due to proper use of whitespace.
Impact on Code Compilers
While whitespace does not affect the execution, different compilers can exhibit varied behavior when faced with excessive or insufficient spacing. For example, consider the following code:
int sum(int a,int b){ // Missing spaces might lead to confusion
return a+b; // No spaces
}
Some compilers might still compile this correctly, but human readers may find it less accessible. Clarity over compactness is advised.
Whitespace in Functions
When declaring or defining functions, proper whitespace helps to organize parameters and return types. Look at the difference in function declaration:
int add(int a, int b) {
return a + b; // Proper spacing
}
This clear arrangement not only improves readability but aids the compiler in parsing the code correctly.
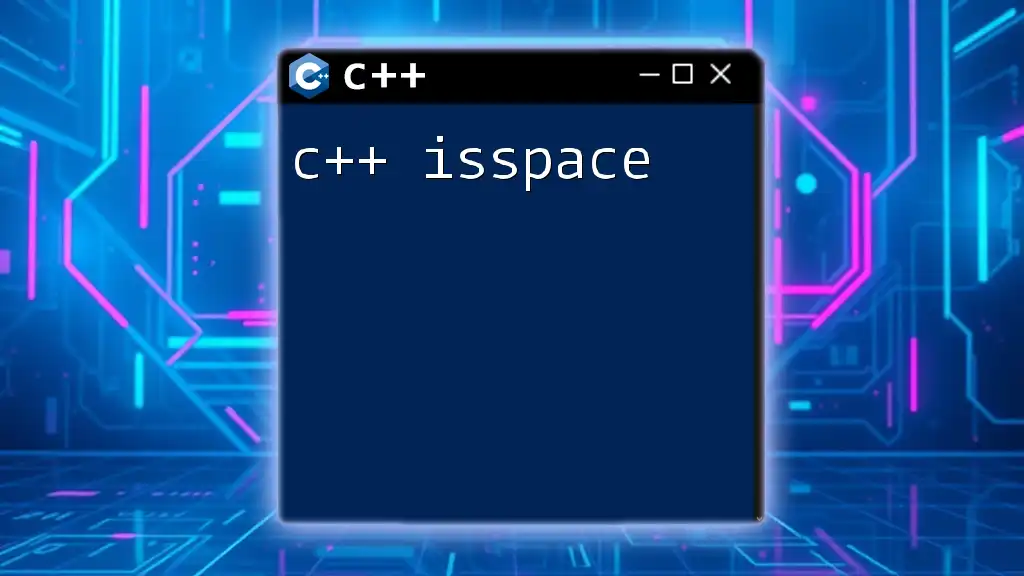
Managing Memory Space in C++
Overview of Stack and Heap Memory
Understanding the difference between stack and heap memory is vital for efficient memory management.
- Stack: Automatically allocated and deallocated; fast but limited in size.
- Heap: Manually allocated and deallocated; flexible size but slower.
When to use which? Prefer stack allocation when the size is known at compile time and memory usage is small. Use heap allocation when dealing with large data structures where the size might change.
For example, consider:
// Stack allocation
int a = 10;
// Heap allocation
int* b = new int(20);
While stack allocation is quicker, heap allocation allows for dynamic memory management.
Memory Management Techniques
Utilizing techniques such as smart pointers enhances memory safety, particularly with heap allocation. For example, a `unique_ptr` automatically deallocates memory when it goes out of scope, preventing memory leaks.
std::unique_ptr<int> p1(new int(10)); // Automatically deallocated
This approach minimizes manual memory management while promoting safe practices.
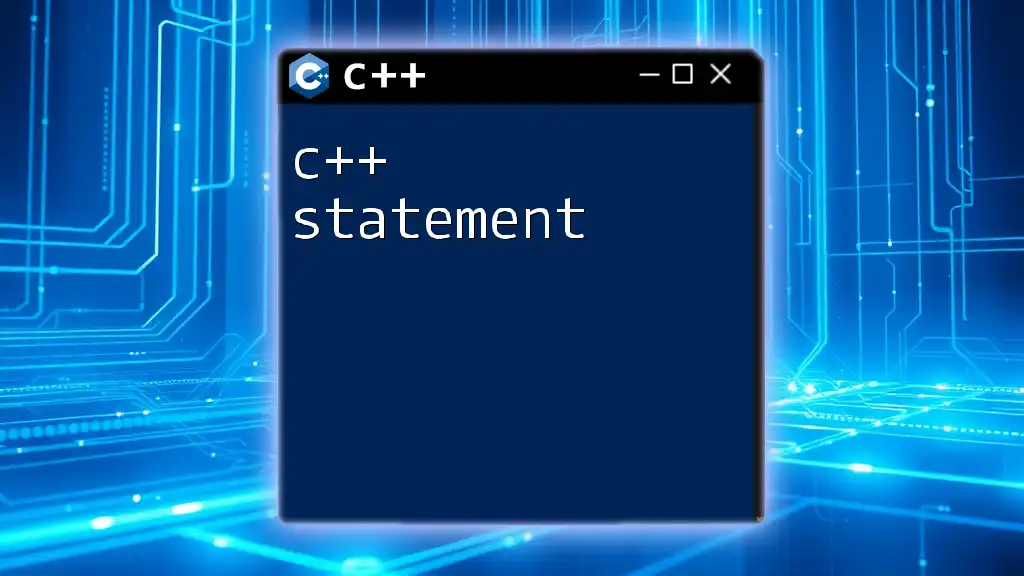
Understanding Namespaces in C++
Creating Customized Namespaces
To create and use custom namespaces in C++, you can define your own grouping of code entities. This helps in organizing your code and avoiding naming collisions.
namespace First {
void functionA() {
// Code for function A
}
}
namespace Second {
void functionB() {
// Code for function B
}
}
In larger codebases, this separation helps maintain organization and can significantly reduce conflicts.
Avoiding Naming Conflicts
Namespaces are particularly effective in preventing naming collisions. Consider the potential for conflict if multiple functions share the same name without namespaces:
void function() {
// Code for function
}
void function() { // Error due to conflict
// Another code for function
}
Using namespaces, you can keep identifiers unique, thus avoiding errors.
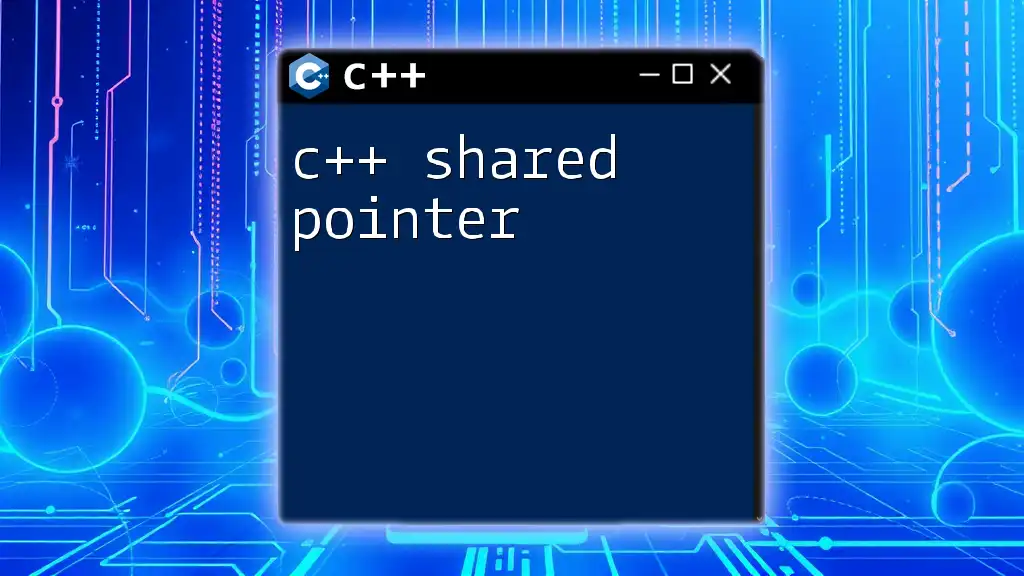
Best Practices for Utilizing Space in C++
Readability and Maintainability
Maintaining high standards of readability and maintainability involves following coding conventions, including appropriate spacing. Always aim for code that is easy to read:
void calculateSum(int a, int b) {
int sum = a + b;
std::cout << "Sum: " << sum; // Clear output formatting
}
This approach will save time during debugging or enhancements in the future.
Debugging Common Space Issues
Common errors often arise from improper use of space, including exceeded line length or incorrect formatting. To debug these issues, consider establishing a coding standard for whitespace usage across your projects, ensuring everyone adheres to the same rules.
int main() {
int a=0; // Potential readability issue
// Fix: use appropriate whitespace
}
Conclusion
Understanding c++ space and its various forms—from whitespace to memory allocation—is essential for writing efficient and understandable code. Proper management of space not only enhances readability but also minimizes the potential for errors. As you continue to practice and refine your skills, strive to implement the best practices discussed. This will significantly contribute to your growth as a proficient C++ developer.
Next Steps
Now that you are familiar with the intricacies of C++ space, continue to explore additional resources, references, and advanced topics to deepen your understanding and elevate your coding capabilities.