A stack overflow in C++ occurs when a program uses more stack space than is available, usually due to excessive recursion or allocation of large local variables.
Here’s a simple code snippet that demonstrates a stack overflow caused by excessive recursion:
#include <iostream>
void recursiveFunction() {
// This function calls itself indefinitely, leading to stack overflow
recursiveFunction();
}
int main() {
recursiveFunction();
return 0;
}
Understanding Stack Overflow
What is Stack Overflow?
Stack Overflow refers to a specific type of error that occurs when a program uses more stack memory than is allocated to it. In C++, the "stack" is a region of memory that temporarily stores local variables and function call information. This memory is limited in size; when a program exceeds that limit, a stack overflow occurs.
Understanding the stack's role in memory management is crucial for efficient programming. The stack operates on a last-in, first-out basis, meaning that the last function pushed onto the stack must be the first to be popped off. This specific arrangement underlines its importance in managing function calls and local variable lifetimes.
How Stack Overflow Occurs
A stack overflow commonly occurs in a few scenarios, especially involving deep recursion—where a function calls itself too many times before reaching a base case. Each call consumes stack space for parameters, return addresses, and local variables. If this goes unchecked, it can quickly exhaust the available stack memory.
One primary source of stack overflow is local variables that consume significant memory. If multiple layers of function calls create large local variables, the cumulative effect can lead to overflow.
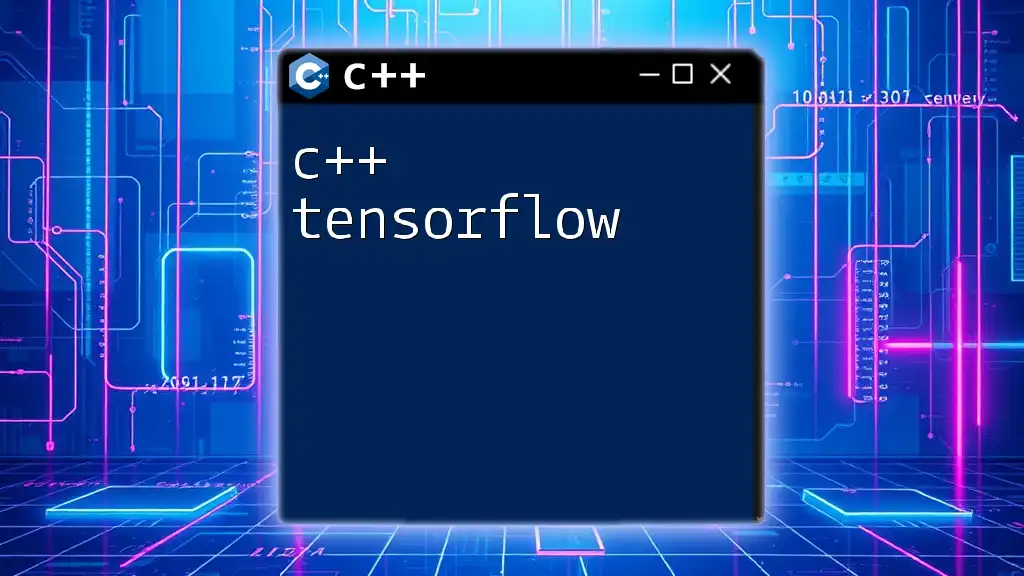
Recognizing Stack Overflow in C++
Symptoms of Stack Overflow
Typical symptoms indicating a stack overflow include application crashes, unexpected behavior, or termination of the program due to exceeding the stack limit. You may see error messages illustrating that the stack space has been exhausted, frequently referred to as "stack overflow exception" errors.
Debugging tools are essential in managing and diagnosing stack overflow issues. Tools like `gdb` (GNU Debugger) or the Visual Studio Debugger can provide insights into where and why the overflow is happening.
Analyzing Stack Overflow Cases
Consider the following example, which illustrates a simple recursive function that leads to stack overflow:
#include <iostream>
void faultyFunction() {
faultyFunction(); // Infinite recursion!
}
int main() {
try {
faultyFunction();
} catch (...) {
std::cout << "Stack overflow occurred!" << std::endl;
}
return 0;
}
In this code, `faultyFunction` calls itself indefinitely, leading to a stack overflow. Debugging tools will show a significant increase in stack depth until the memory limit is reached.
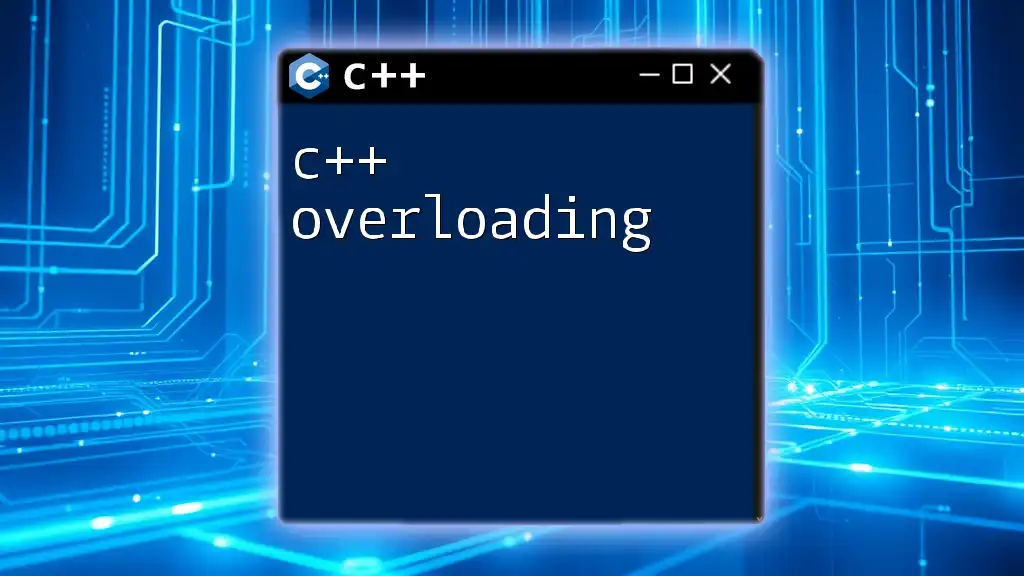
Preventing Stack Overflow
Writing Safe Recursive Functions
To avoid stack overflow, it's vital to write safe recursive functions. Always ensure that a function has a proper base case to stop the recursion. This ensures that the function does not call itself endlessly.
Here’s an example of a well-formed recursive function that effectively prevents stack overflow:
#include <iostream>
int safeFunction(int n) {
if (n <= 0) {
return 0;
}
return n + safeFunction(n - 1);
}
int main() {
std::cout << safeFunction(10) << std::endl;
return 0;
}
In this example, the recursion halts when `n` becomes non-positive, thus avoiding stack overflow.
Using Iterative Solutions
In many cases, using iterative solutions instead of recursion can greatly mitigate the risk of stack overflow. Iterative methods rely on loops rather than function calls, which require stack space. This not only conserves memory but also enhances performance.
For example, here’s how you can convert a recursive function to an iterative version:
#include <iostream>
int iterativeFunction(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
int main() {
std::cout << iterativeFunction(10) << std::endl;
return 0;
}
In this case, the `iterativeFunction` calculates the sum using a loop, significantly reducing the risk of a stack overflow.
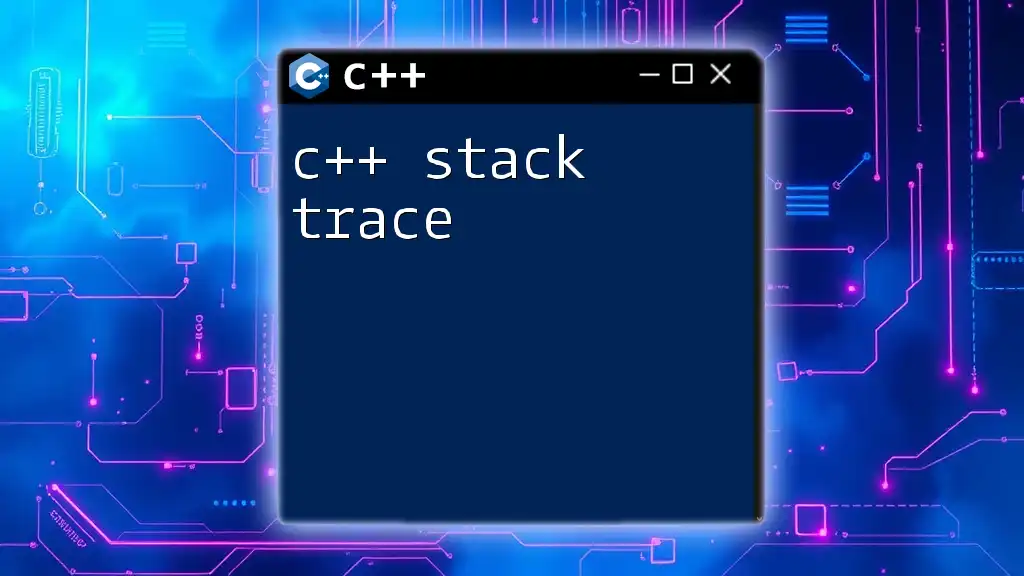
Debugging Stack Overflow Issues in C++
Tools and Techniques
When stack overflow occurs, effective debugging tools can be indispensable. Tools like `gdb` or built-in debuggers in IDEs such as Visual Studio allow you to set breakpoints and analyze stack memory usage as your program runs. You can inspect local variables and monitor function calls to pinpoint where your code exceeds stack limits.
Memory Profiling
Memory profiling techniques can help keep track of stack usage throughout the runtime of your application. Profiling tools such as Valgrind or specialized C++ profilers can track function call depths, variable sizes, and overall stack usage, helping you to optimize your code further. Understanding these metrics can guide you in effective memory management techniques to avoid scenarios that may lead to stack overflow.
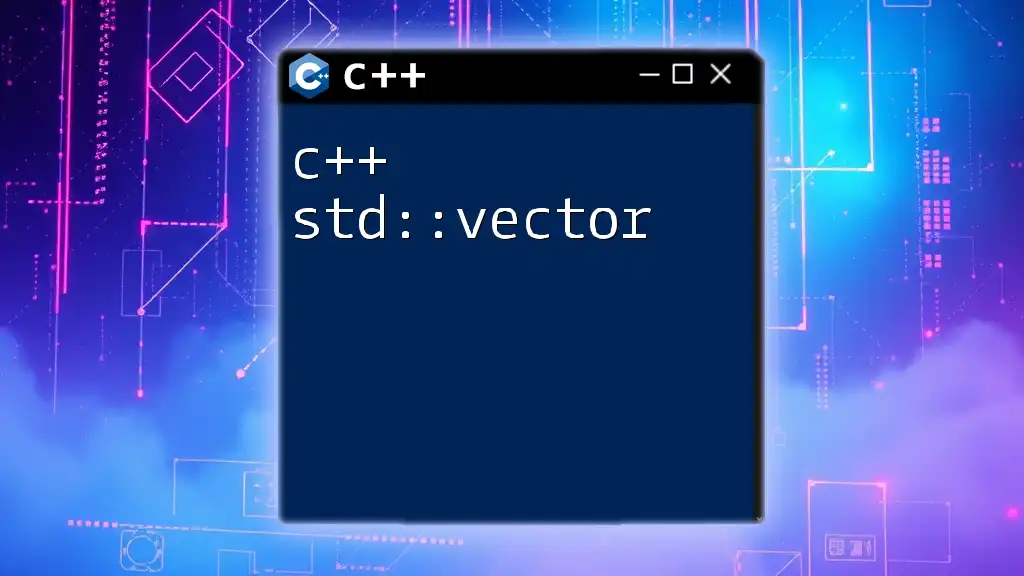
Conclusion
Summary of the Key Points
In summary, C++ stack overflow is a critical concept to grasp for any developer. The nature of stack memory, the role of recursion, and the implications of deep function calls are central to understanding how stack overflow occurs.
Final Thoughts
Implementing safe coding practices and iterating over problems instead of recursing can mitigate these risks. Don’t hesitate to seek further insights from community forums or resources, including platforms like Stack Overflow, as you continue to refine your C++ programming skills.
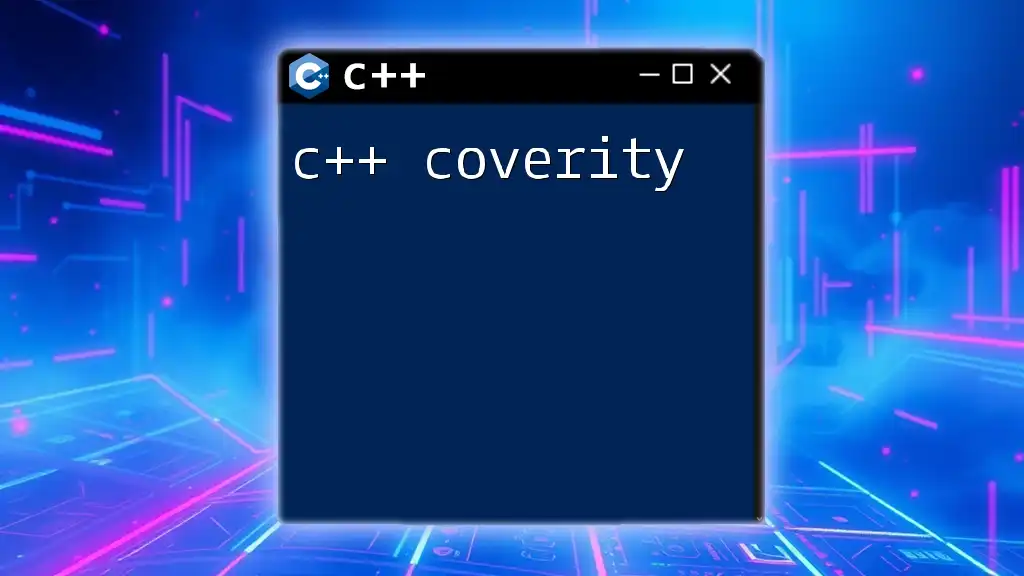
Additional Resources
Recommended Readings and Tutorials
To dive deeper into C++ memory management and programming best practices, explore comprehensive resources and join relevant communities. Engaging with other developers will provide valuable insights, tools, and techniques necessary for mastering C++ and avoiding pitfalls like stack overflow.