C++ TensorFlow is a powerful API that allows developers to create and manipulate machine learning models using C++ with the same efficiency and performance as TensorFlow's Python interface.
Here’s a simple example to create a constant tensor in C++ using TensorFlow:
#include "tensorflow/cc/client/client_session.h"
#include "tensorflow/cc/ops/standard_ops.h"
#include "tensorflow/cc/ops/placeholders.h"
#include "tensorflow/core/framework/tensor.h"
int main() {
using namespace tensorflow; // NOLINT(build/namespaces)
Scope root = Scope::NewRootScope();
// Create a constant tensor
auto constant_tensor = ops::Const(root.WithOpName("constant"), { {1, 2}, {3, 4} });
// Create a session to run the graph
ClientSession session(root);
std::vector<Tensor> outputs;
// Run the session
session.Run({constant_tensor}, &outputs);
// Print the output
std::cout << outputs[0].matrix<int>() << std::endl; // Output: [[1, 2], [3, 4]]
return 0;
}
This snippet demonstrates how to create a constant tensor in TensorFlow using C++.
Why Use TensorFlow with C++?
Using C++ TensorFlow has several compelling advantages that make it an appealing choice for developers and engineers.
Performance Benefits: C++ is known for its high performance and low-level memory manipulation capabilities. Utilizing TensorFlow with C++ allows you to achieve faster execution times compared to Python implementations, particularly in environments where speed and efficiency are critical.
Integration with C++ Projects: If you have existing applications written in C++, incorporating TensorFlow allows you to leverage machine learning capabilities without the need to rewrite your application. It provides a seamless way for developers to add advanced features like neural networks and data predictions.
Expandability: Combining TensorFlow with C++ enables developers to take advantage of numerous C++ libraries and tools, enhancing functionality and streamlining the development process.
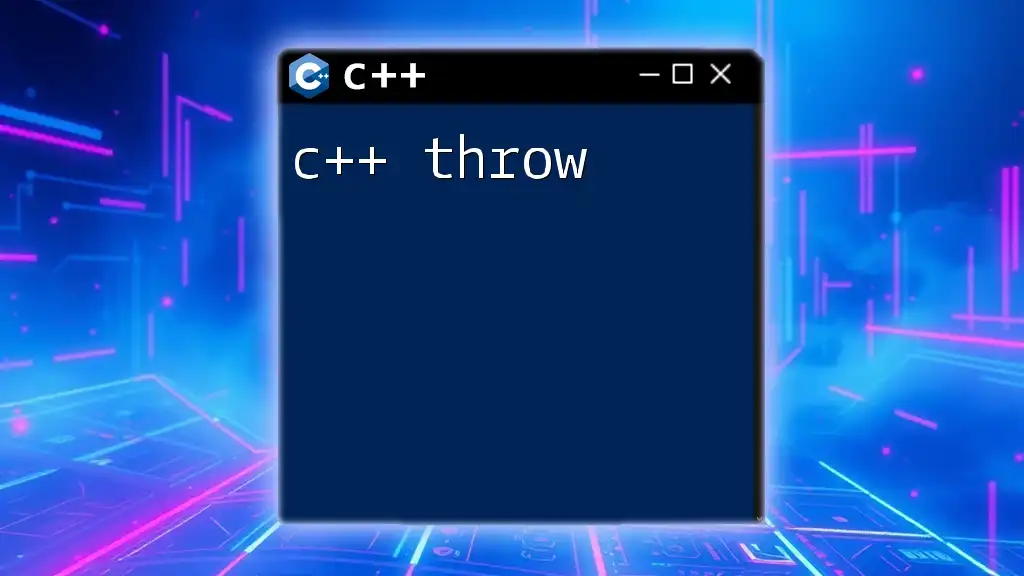
Getting Started with TensorFlow in C++
Before diving into C++ TensorFlow programming, you'll need to ensure that your development environment is correctly set up.
Installation Requirements
To use TensorFlow for C++, you need to confirm you have the following prerequisites:
- Hardware: A machine with a multi-core CPU; a GPU is optional for performance enhancement.
- Software: An operating system that supports TensorFlow (Linux is commonly used).
To install TensorFlow, follow these steps:
- Download the latest TensorFlow binary for C++ from the [official TensorFlow GitHub repository](https://github.com/tensorflow/tensorflow).
- Follow the installation instructions specific to your operating system.
Setting Up Your Development Environment
Choosing an integrated development environment (IDE) can significantly streamline your development process. Popular choices for C++ development that work well with TensorFlow include:
- Visual Studio: Well-suited for Windows users, offering extensive debugging options.
- CLion: A cross-platform IDE that supports CMake, which is particularly useful since TensorFlow uses it for building projects.
- Code::Blocks: A free, open-source IDE ideal for beginners.
Compiling TensorFlow from Source
To compile TensorFlow from source, you'll need to follow these steps:
- Clone the TensorFlow repository:
git clone https://github.com/tensorflow/tensorflow.git
- Install the necessary dependencies according to your operating system.
- Use the following commands to build TensorFlow:
cd tensorflow mkdir build cd build cmake .. -DTENSORFLOW_BUILD_PYTHON_BINDINGS=OFF make
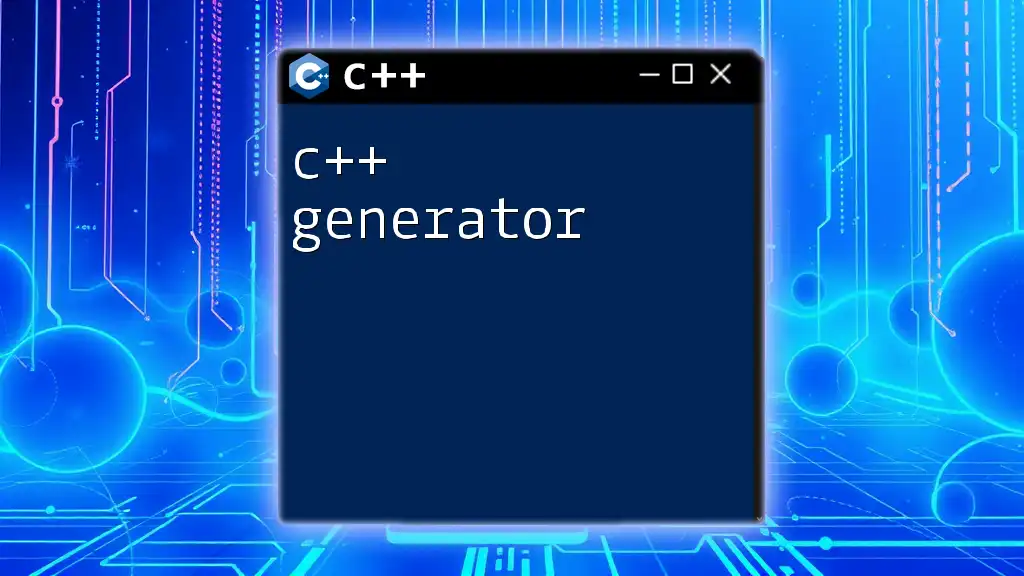
Fundamental Concepts of TensorFlow for C++
Understanding the basic components of TensorFlow will significantly enhance your capability to develop machine learning applications.
Understanding Tensors
Tensors are the fundamental data structure in TensorFlow. They represent multi-dimensional arrays of data. Here’s how you can create and manipulate tensors in C++:
#include "tensorflow/core/public/session.h"
#include "tensorflow/core/platform/env.h"
using namespace tensorflow;
// Create a 1D tensor (vector)
Tensor tensor(DT_FLOAT, TensorShape({3}));
tensor.flat<float>()(0) = 1.0;
tensor.flat<float>()(1) = 2.0;
tensor.flat<float>()(2) = 3.0;
Graphs and Sessions
TensorFlow uses a computational graph framework. A graph consists of nodes and edges where nodes represent operations or variables, and edges represent the tensors flowing between them.
To create and run a session in C++, follow this example:
#include "tensorflow/core/public/session.h"
#include "tensorflow/core/platform/env.h"
using namespace tensorflow;
int main() {
// Create a new session
std::unique_ptr<Session> session(NewSession(SessionOptions()));
// Your graph definition here
// ...
// Run the session
session->Run({}, nullptr);
return 0;
}
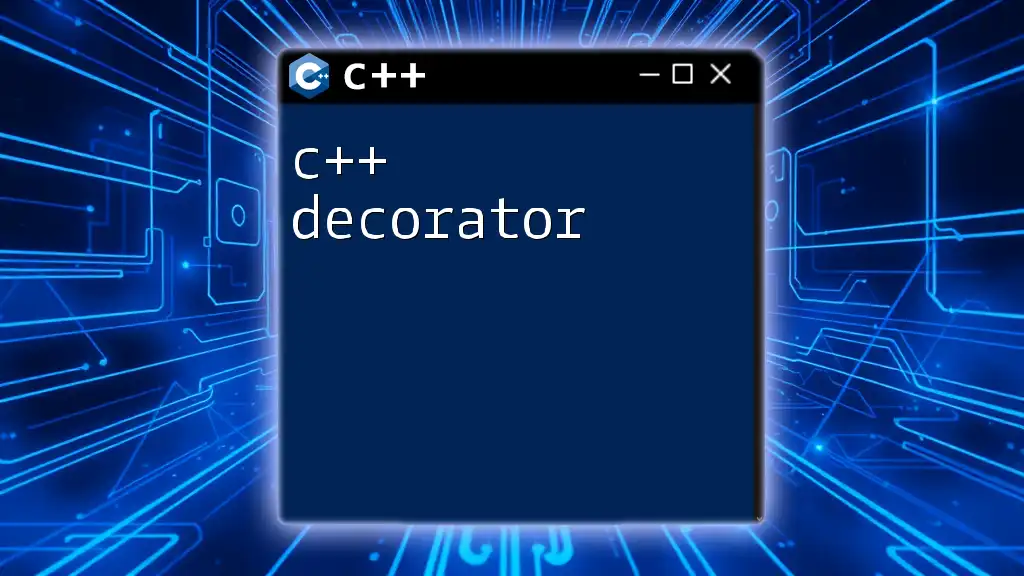
Building a Simple Machine Learning Model with C++
Now, let’s put TensorFlow to work by building a simple neural network for classification.
Defining the Model
The following code outlines a basic neural network structure:
#include "tensorflow/core/public/session.h"
#include "tensorflow/core/platform/env.h"
using namespace tensorflow;
// Function to build a simple model
void BuildModel(GraphDef& graph_def) {
// Define graph (input layer, hidden layers, output layer)
// ...
}
Training the Model
Training the model involves feeding it data and letting it learn to make predictions. Here’s an abstract of how you can train the model using your data:
// Sample training function
void TrainModel(Session* session, const Tensor& input, const Tensor& output) {
// Define training process (forward propagation, backpropagation)
// ...
session->Run({{"input:0", input}, {"output:0", output}}, nullptr);
}
Evaluating the Model
After training, you must test the model's performance. The evaluation consists of running predictions on unseen data and calculating accuracy.
// Sample evaluation function
void EvaluateModel(Session* session, const Tensor& test_data) {
// Assess performance metrics (accuracy, loss)
// ...
session->Run({{"test_data:0", test_data}}, nullptr);
}
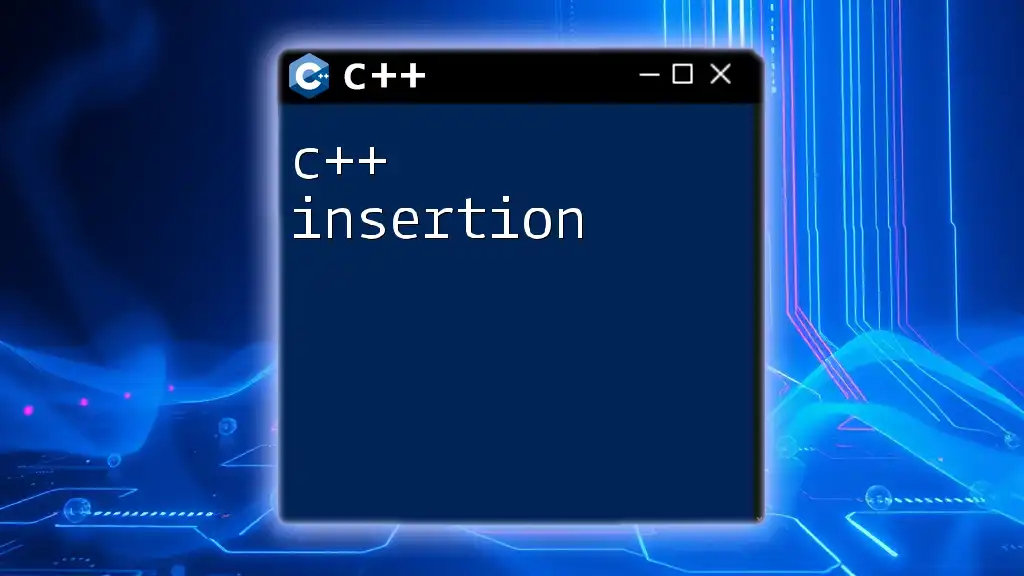
Advanced Topics in TensorFlow for C++
Custom Operations
One of the most powerful features of TensorFlow is the ability to define custom operations. Below is a brief outline for creating a custom op:
REGISTER_OP("MyCustomOp")
.Input("input: float")
.Output("output: float");
Using Pre-Trained Models
With TensorFlow’s vast library of pre-trained models, you can leverage existing knowledge without building from scratch. Here’s a simplified way to load a pre-trained model:
GraphDef graph_def;
ReadBinaryProto(Env::Default(), "model.pb", &graph_def);
// Load and run the model in your session
Optimizing Performance
Performance optimization is crucial when using TensorFlow in C++. Techniques include:
- Memory Management: Use `tf::Tensor` efficiently to avoid memory leaks.
- Performance Profiling: Identify bottlenecks by profiling your application with TensorFlow tools.
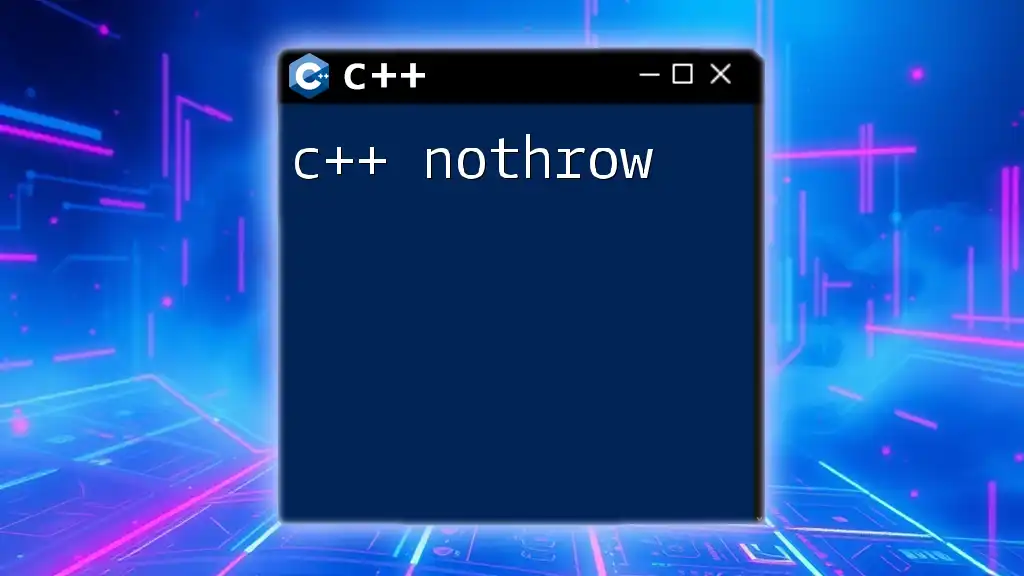
Debugging and Troubleshooting C++ TensorFlow
Common Issues
Working with C++ TensorFlow can sometimes lead to tricky problems. Here are a few common issues:
- Linking Errors: Ensure all TensorFlow libraries are correctly linked in your project settings.
- Version Mismatches: Always check that your C++ API version matches the TensorFlow binaries you’ve downloaded.
Debugging Tips
When debugging C++ applications using TensorFlow, consider the following approaches:
- Verbose Logging: Enable verbose logging in TensorFlow for better insight into runtime issues.
- Debuggers: Utilize IDE debugging features to step through your code and identify runtime issues in real-time.
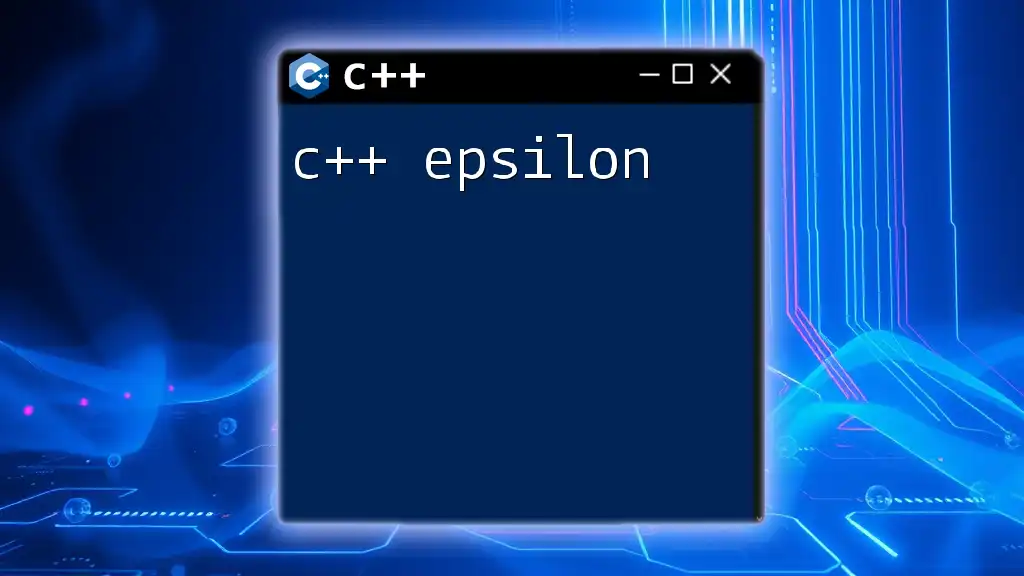
Conclusion
In conclusion, leveraging C++ TensorFlow can profoundly impact the efficiency and performance of your machine learning projects. By following this guide, you've gained insight into setting up your environment, understanding core concepts, and executing advanced tasks. As you delve deeper into C++ TensorFlow, consider exploring more advanced models and optimization techniques to elevate your machine learning expertise.
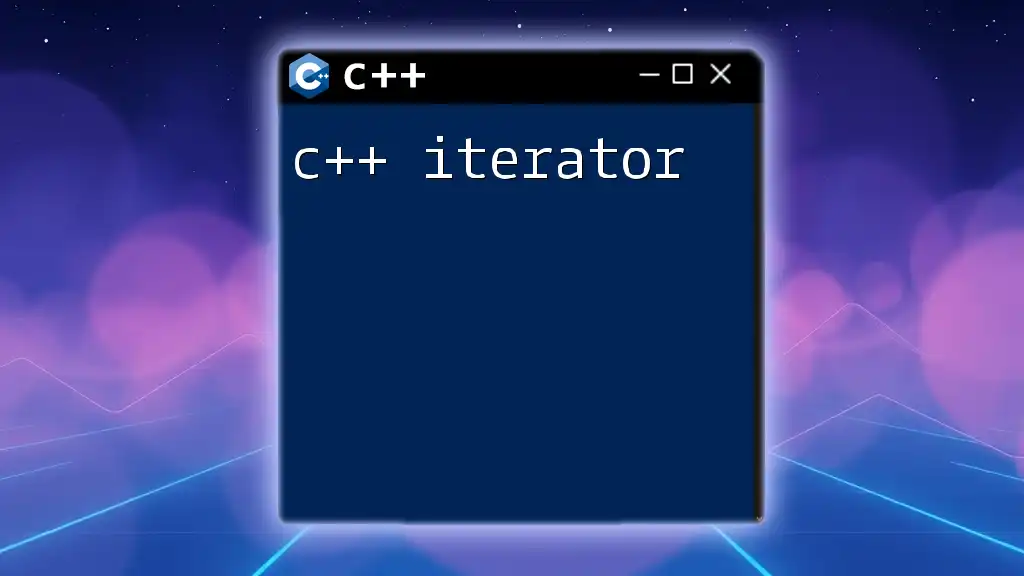
Additional Resources
For further exploration, consult the official TensorFlow documentation and engage with community forums for additional support and learning opportunities.