In C++, the `nothrow` constant can be used with the `new` operator to indicate that it should return `nullptr` instead of throwing an exception when memory allocation fails.
Here's a code snippet demonstrating its use:
#include <iostream>
#include <new> // For std::nothrow
int main() {
int* arr = new(std::nothrow) int[1000000000]; // Attempt to allocate a large array
if (!arr) {
std::cout << "Memory allocation failed!" << std::endl;
} else {
std::cout << "Memory allocation succeeded." << std::endl;
delete[] arr; // Remember to free the allocated memory
}
return 0;
}
What is `nothrow`?
`nothrow` is a constant provided in C++ as part of the `<new>` header. When this constant is used with dynamic memory allocation, it changes how memory allocation failures are reported. Instead of throwing an exception (which is the default behavior), the `nothrow` constant tells the C++ runtime to return a `nullptr` when memory allocation fails. This feature is particularly useful for creating more robust applications that manage memory without unexpected crashes.
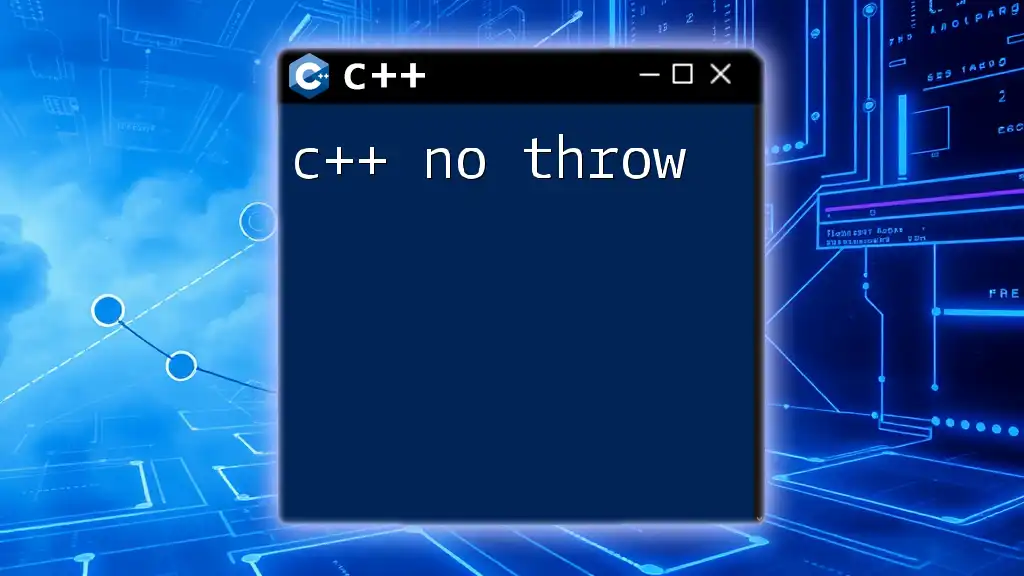
Importance of Using `nothrow`
In C++, memory management plays a pivotal role in ensuring program stability. By using `nothrow`, developers can enhance error handling significantly when an allocation failure occurs.
-
Memory Management: Typically, if you try to allocate memory and the allocation fails, C++ throws a `std::bad_alloc` exception. Using `nothrow`, the program simply receives a `nullptr`, allowing for custom error-checking and handling pathways.
-
Error Handling: By managing memory allocation failures directly, developers can dictate specific actions to perform in the event of a failure, which can be critical in performance-sensitive applications.
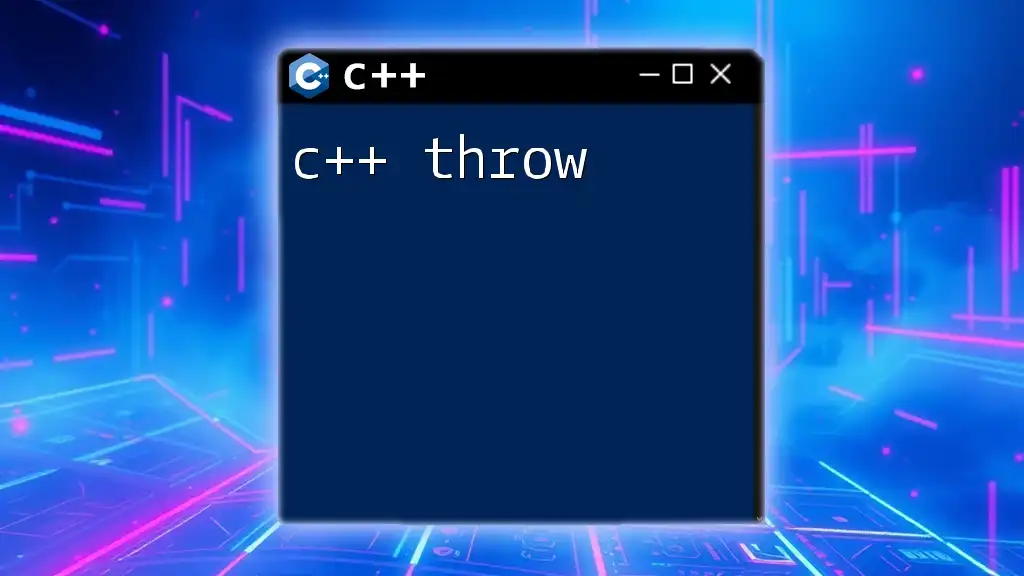
Understanding Exceptions in C++
What are Exceptions?
Exceptions in C++ are a powerful mechanism for error handling. They provide a way to signal that something unexpected has happened in a program, ideally allowing the program to gracefully handle the situation.
Memory Allocation Exceptions
When you allocate memory using the default setup (without `nothrow`), if the allocation fails because there isn't enough memory available, the C++ runtime will throw a `std::bad_alloc` exception.
How `nothrow` Fits In
Behavior Without `nothrow`
By default, if an allocation request fails, an exception is thrown, which could lead to the termination of the program if it is not caught correctly.
Behavior With `nothrow`
With `nothrow`, instead of throwing an exception, the system returns `nullptr`. This allows developers to implement checks around memory allocation, making the program more resilient.
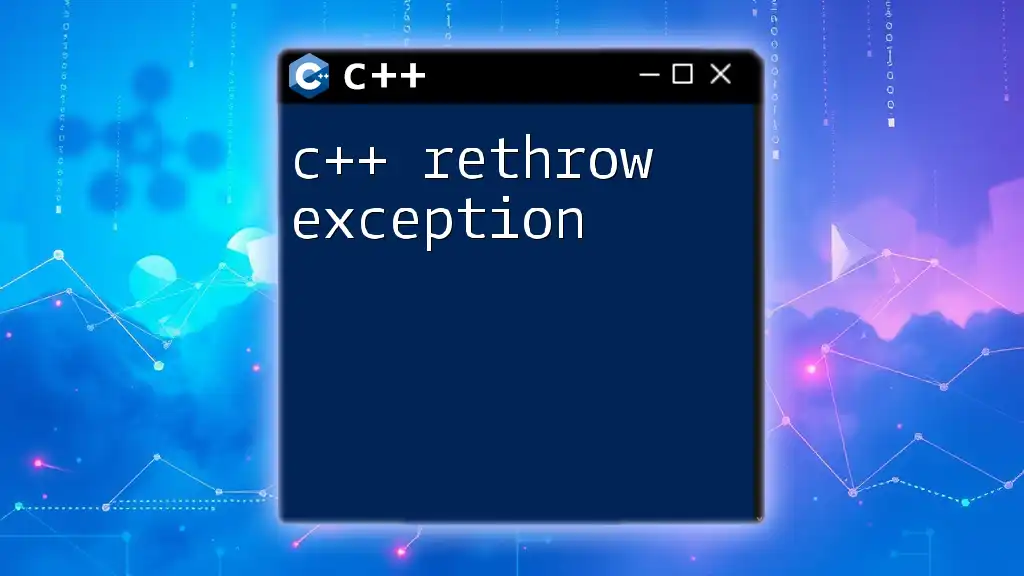
Using `nothrow` in C++
Basic Syntax and Usage
To utilize `nothrow`, include the `<new>` header and use the `new` operator in conjunction with `std::nothrow` like this:
#include <iostream>
#include <new> // Include for std::nothrow
int main() {
int* p = new (std::nothrow) int[1000000000]; // Request large memory
if (!p) {
std::cout << "Memory allocation failed." << std::endl; // Handle error
}
// Clean up if allocated
delete[] p;
return 0;
}
In this code snippet, we are attempting to allocate a large array of integers. The use of `nothrow` allows the program to handle the situation gracefully if the allocation fails.
Practical Example
Simple Memory Allocation Example
Consider this example that illustrates both successful and failed memory allocations:
#include <iostream>
#include <new>
int main() {
int* p1 = new (std::nothrow) int(42); // Allocation succeeds
int* p2 = new (std::nothrow) int[1000000000]; // Likely fails
if (p1) {
std::cout << "Value: " << *p1 << std::endl; // Output: Value: 42
delete p1;
}
if (!p2) {
std::cout << "Memory allocation failed for p2." << std::endl; // Handle error
}
return 0;
}
In this program, memory is successfully allocated for `p1`, allowing us to print its value. For `p2`, which requests a large block of memory, we check if the pointer is null to handle errors appropriately.
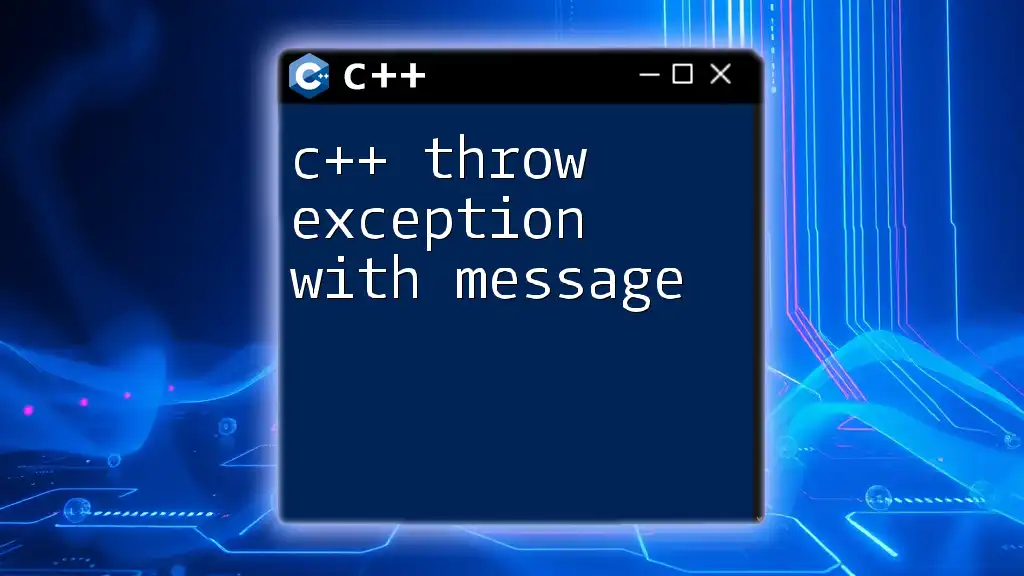
Advantages of Using `nothrow`
Improved Stability
Using `nothrow` enhances the stability of an application by avoiding abrupt terminations due to unhandled exceptions. This can be particularly advantageous in environments where uptime and reliability are critical.
Clear Error Handling
One of the primary benefits of using `nothrow` is that it encourages developers to check pointer validity. This leads to clearer and more manageable error checking as you can simply verify if the pointer is `nullptr`.
Avoiding Exception Overheads
In performance-critical applications, avoiding exception handling can result in performance gains. When using `nothrow`, since you aren't risking exceptions, you don't incur the overhead associated with exception handling.
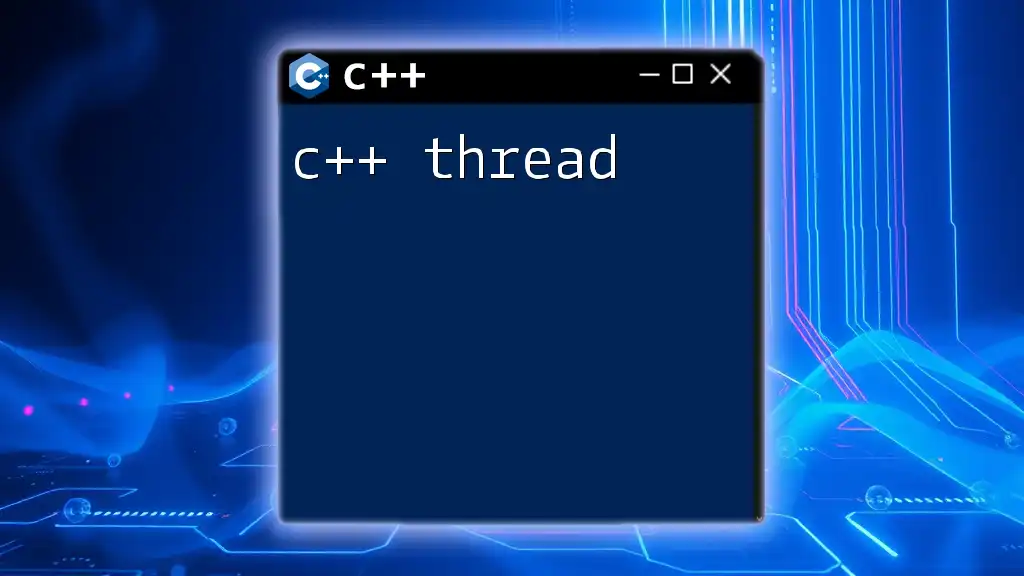
Limitations of `nothrow`
Non-Recoverable Errors
While `nothrow` provides a way to handle memory allocation failures, not catching a `nullptr` can lead to memory leaks or undefined behavior, especially if memory is not freed appropriately.
Not a Replacement for Good Practices
Although `nothrow` provides benefits, it should not replace sound programming practices. Developers should still focus on efficient memory use and proper allocation patterns to avoid low-memory situations in the first place.
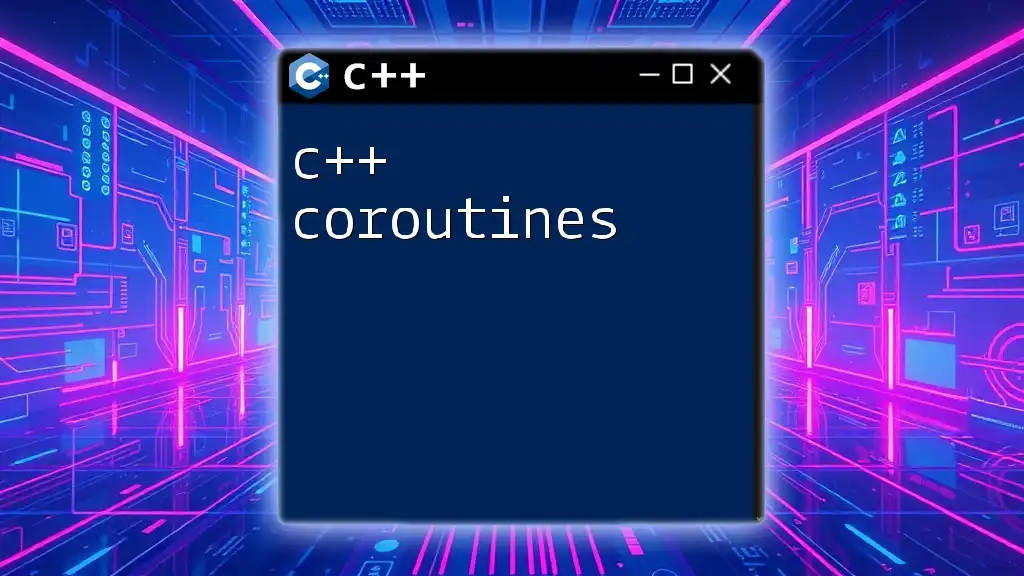
Best Practices for Using `nothrow`
When to Use `nothrow`
`nothrow` is particularly useful in scenarios where performance is sensitive, and you cannot afford to have the program crash due to unhandled exceptions. It is advisable to use it in systems programming, embedded systems, and high-load applications.
Combining with Smart Pointers
Implementing `nothrow` along with smart pointers, such as `std::unique_ptr`, can lead to safer memory management. Consider the following example:
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> p(new (std::nothrow) int(50));
if (!p) {
std::cout << "Memory allocation failed." << std::endl; // Handle error
} else {
std::cout << "Value: " << *p << std::endl; // Safe memory management
}
return 0;
}
Here, if the memory allocation fails, `p` will be `nullptr`, and you can handle the error gracefully. The added benefit is that `unique_ptr` automatically manages the memory, ensuring that there are no leaks.
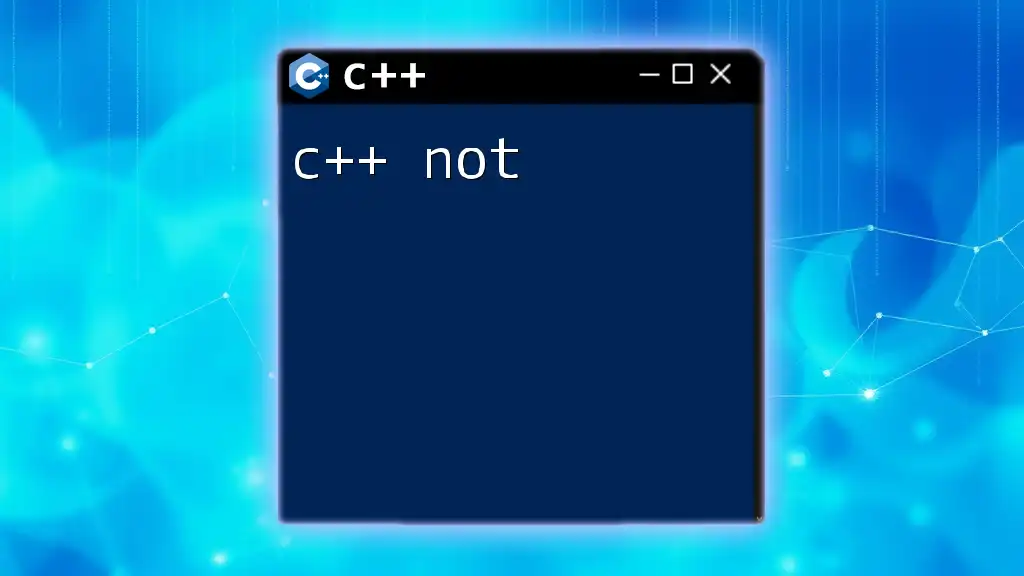
Conclusion
In summary, `nothrow` serves as a significant tool in the C++ memory management arsenal. It switches the paradigm from throwing exceptions to returning `nullptr`, allowing developers to implement more controlled error handling. By being mindful of when and how to use `nothrow`, one can achieve improved stability and performance in their applications. As you explore memory management in C++, consider reaching out for more insights and advanced techniques to fine-tune your C++ skills.