In C++, a browser can be implemented as a simple console application that fetches and displays web pages using libraries like libcurl for handling HTTP requests.
#include <iostream>
#include <curl/curl.h>
size_t WriteCallback(void* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
int main() {
CURL* curl;
CURLcode res;
std::string readBuffer;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, WriteCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
std::cout << "Fetched Content: " << readBuffer << std::endl;
}
return 0;
}
Understanding C++ in Web Development
The Role of C++ in Web Technologies
C++ plays a significant role in modern web development, particularly in performance-critical areas. While languages such as JavaScript and Python dominate the client-side and scripting domain, C++ is often employed for backend systems, rendering engines, and in situations where high efficiency is paramount. Its ability to operate closely with system hardware makes C++ a favorite choice for applications that require robust performance.
Use Cases of C++ in Browsers
C++ has diverse applications in the realm of web browsers. Here are a few notable use cases:
-
Building plugins and extensions: C++ can be utilized to develop lightweight extensions that enhance browser functionality without compromising on performance.
-
Backend systems and frameworks: Several modern web applications rely on C++ frameworks for their backend, ensuring that data is processed swiftly and accurately.
-
Performance-critical applications: When the execution speed of web applications is a primary concern, C++ provides the low-level control necessary to optimize performance.
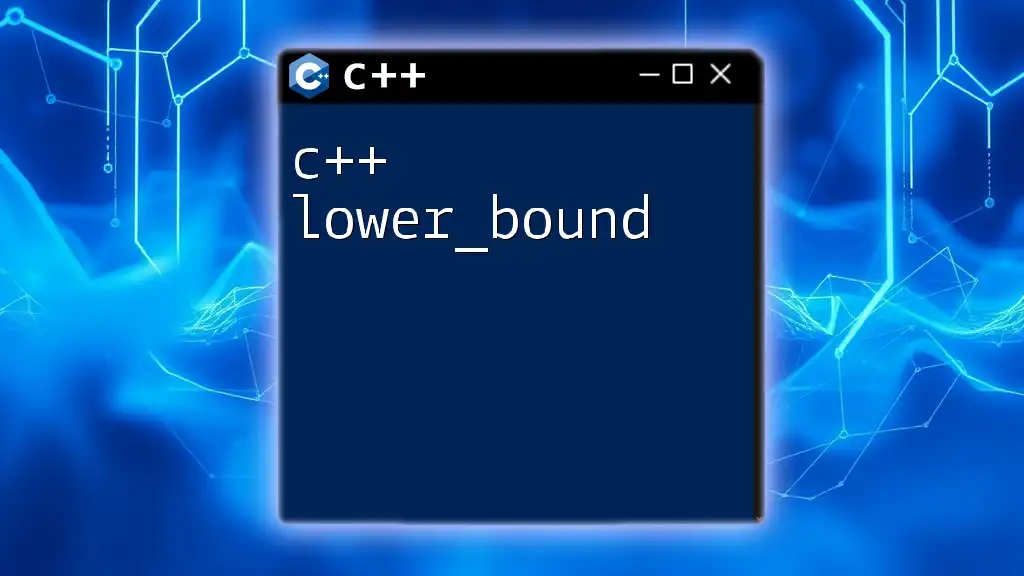
Setting Up Your C++ Development Environment
Required Tools and Software
To kickstart C++ development, you'll need to equip yourself with the right tools:
-
Compilers: Popular C++ compilers include GCC (GNU Compiler Collection), Clang, and Microsoft Visual C++. Each compiler has unique features, so choose one that best fits your project needs.
-
Integrated Development Environments (IDEs):
- Visual Studio provides robust tools for debugging and building applications.
- Code::Blocks offers a customizable interface, suited for C++ development.
-
Browser development tools: Familiarize yourself with browsers' built-in developer tools, which are essential for testing and debugging web applications.
Installing Necessary Libraries
Certain libraries can enhance your C++ browser development experience:
-
Boost: A widely used collection of libraries offering a range of functionalities, from smart pointers to regular expressions.
-
Qt: An open-source framework that provides tools for GUI development. It also includes QtWebKit, an integral part of building web applications in C++.
To install Boost, you can use the following command on a Linux-based system:
sudo apt-get install libboost-all-dev
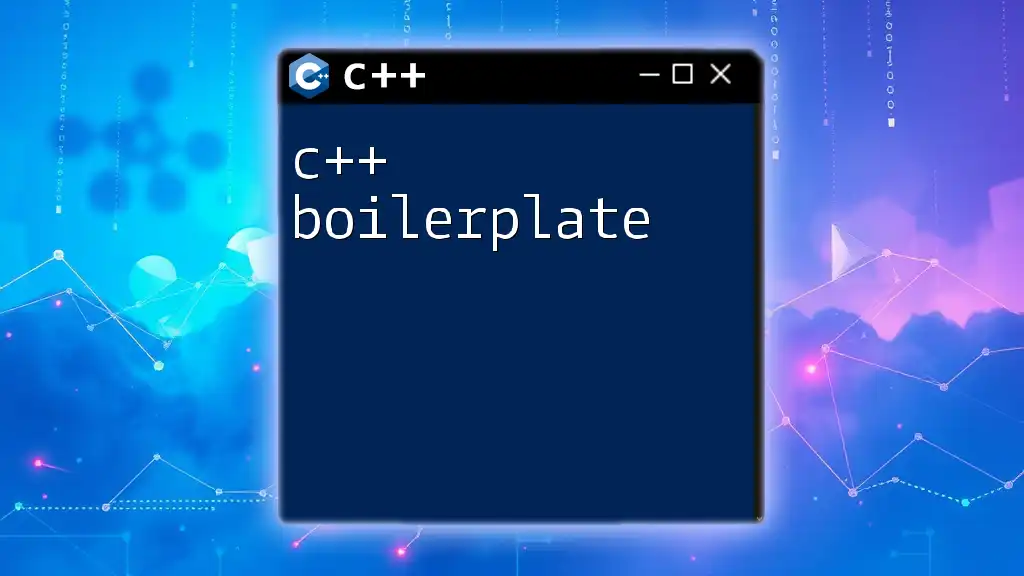
C++ Browsers: An Overview
What is a C++ Browser?
A C++ browser is essentially an application built using C++ that can fetch, render, and display web content. It combines the performance advantages of C++ with the connectivity of modern web technologies, allowing developers to create fast, efficient browsers.
Examples of projects leveraging C++ for browser development include WebKit and Chromium, both of which are renowned for their speed and versatility.
How C++ Browsers Work
Understanding the structure of a C++ browser involves exploring its basic architecture:
-
Rendering engine: Responsible for interpreting HTML, CSS, and JavaScript to display web pages accurately.
-
HTML parser: Converts HTML code into a navigable DOM (Document Object Model), allowing for dynamic interaction with web content.
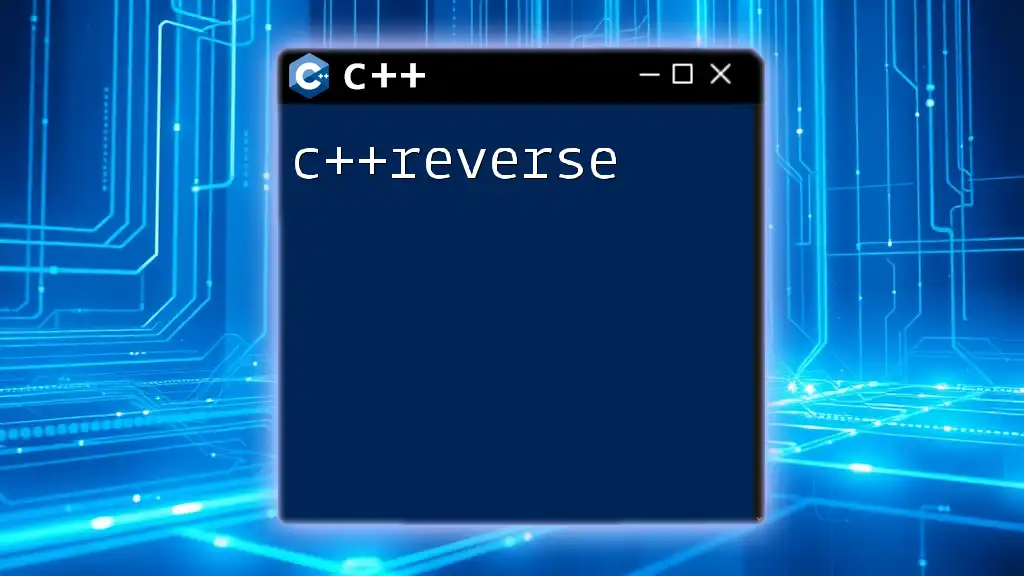
Creating a Simple C++ Browser
Step-by-Step Guide
Setting Up the Project
Begin by creating a new project in your preferred IDE. This will centralize all your files and maintain organization.
Building the Core Functions
Start by writing essential functions to handle HTTP requests, which form the backbone of any browser. Integrating the cURL library can simplify network communications. Here is a simple example of an HTTP request in C++:
#include <iostream>
#include <curl/curl.h>
int main() {
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://www.example.com");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
return 0;
}
This code initializes cURL, makes an HTTP request to "www.example.com", and cleans up the resources afterward.
Rendering Web Pages
For rendering, leveraging libraries like QtWebKit can provide the necessary functionality. The following snippet demonstrates basic rendering of a web page using Qt:
#include <QtWebEngineWidgets/QWebEngineView>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWebEngineView view;
view.setUrl(QUrl("http://www.example.com"));
view.show();
return app.exec();
}
In this section, you create a basic Qt application that opens a browser window displaying the specified URL.
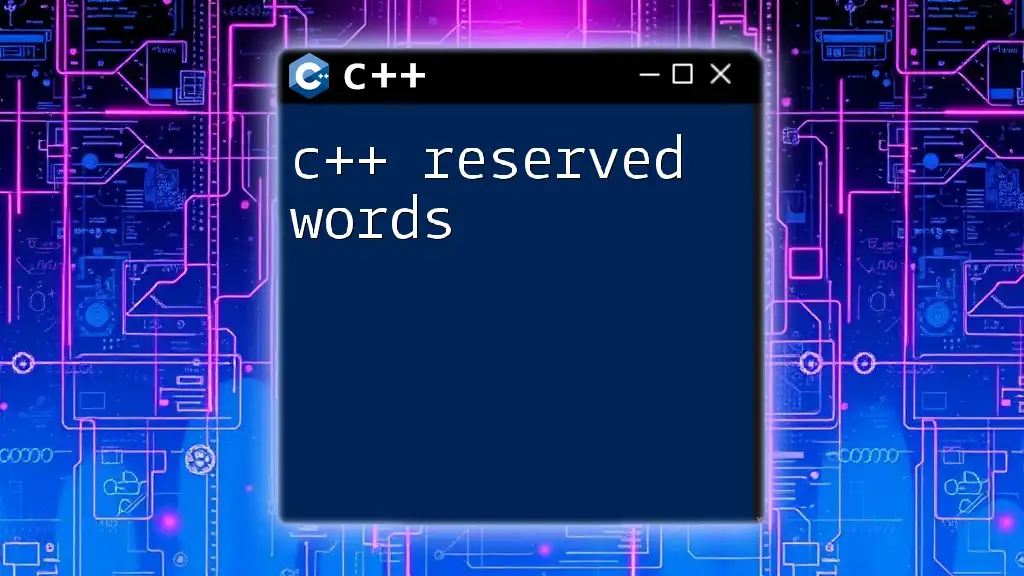
Advanced Concepts in C++ Browsers
Rendering Engines
The rendering engine is vital for interpreting web content. Popular engines include WebKit, Gecko, and Blink. Each has its strengths; for instance:
- WebKit: Known for its fast performance and is utilized by Safari.
- Gecko: Used by Firefox, it emphasizes compliance with web standards.
- Blink: A fork of WebKit; known for its speed and used by Google Chrome.
JavaScript Integration
With C++, it’s essential to integrate JavaScript effectively as it is the primary scripting language for web development. This can be done by using libraries like Node.js, which allows executing JavaScript code from C++ code.
Networking and Security
Implementing security is paramount for any browser. You can achieve this by utilizing SSL/TLS protocols for secure connections and ensuring your browser supports HTTPS. Additionally, exploring peer-to-peer networking capabilities can extend functionality, allowing direct communication between users.
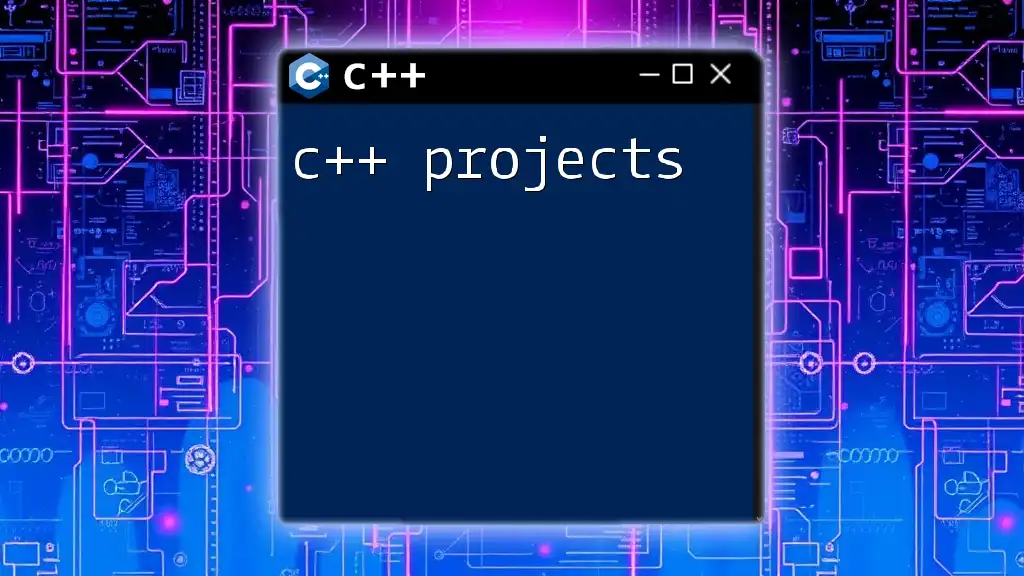
Debugging and Testing Your C++ Browser
Tools for Debugging
Effective debugging tools such as gdb (GNU Debugger) and valgrind allow developers to identify and fix errors in code efficiently. Learning how to use these tools is essential for maintaining high-quality code in your C++ browser.
Testing Best Practices
Adopting testing best practices ensures your browser's reliability. Writing unit tests helps to validate individual components, while automated testing frameworks allow for comprehensive tests across the entire application.
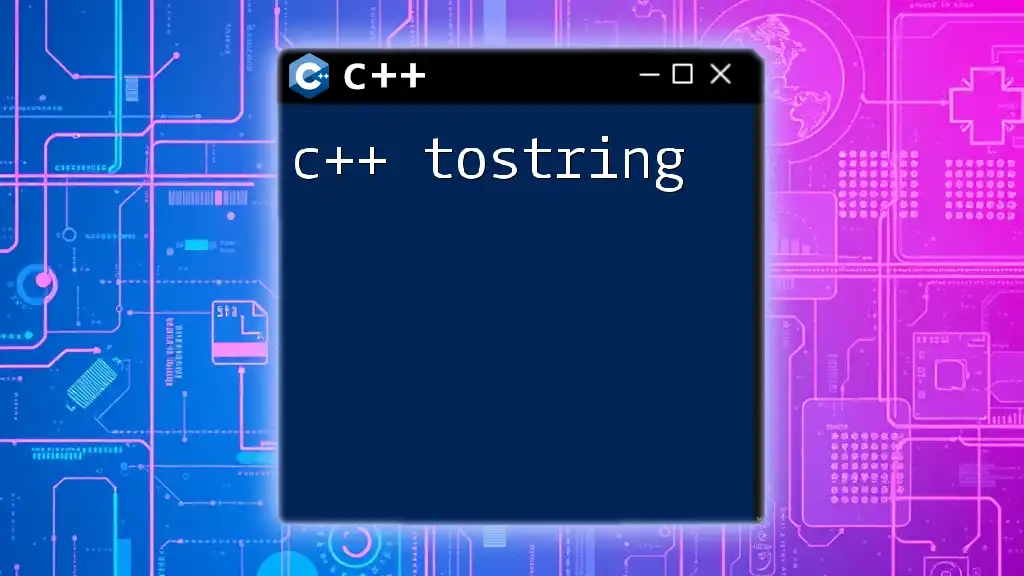
Resources and Further Learning
Recommended Books and Tutorials
Enhance your learning with a selection of C++ programming books, each offering valuable insights into best practices and advanced techniques. Online tutorials that focus on web development with C++ can provide hands-on experience and further understanding.
Community and Forums
Engaging with online communities, such as Stack Overflow and GitHub, can offer support, collaboration opportunities, and networking with other C++ developers.
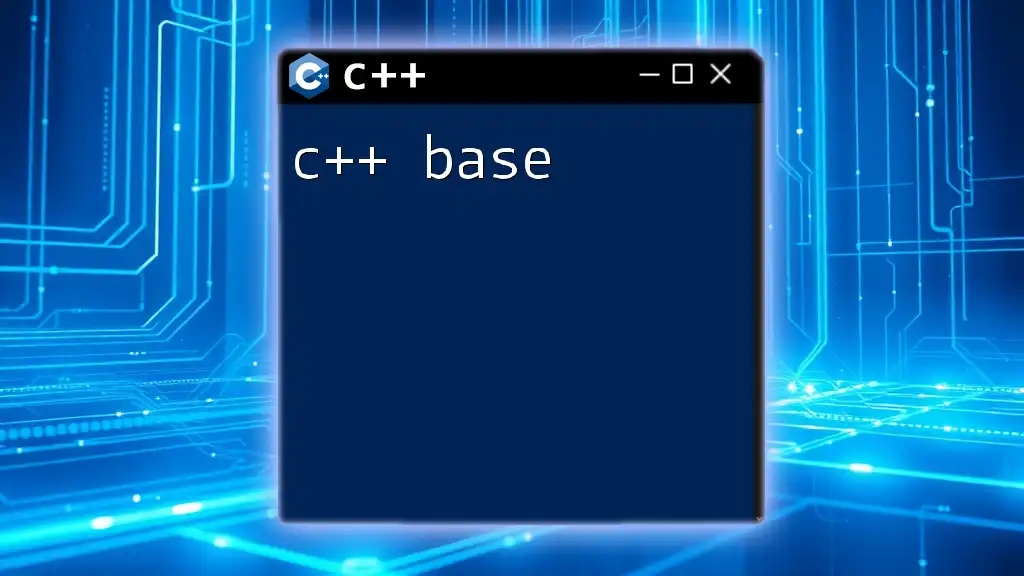
Conclusion
Exploring the world of C++ browsers opens up numerous opportunities for harnessing the power of this language in web development. Whether working on backend systems, plugins, or even creating a complete browser, the potential is vast.
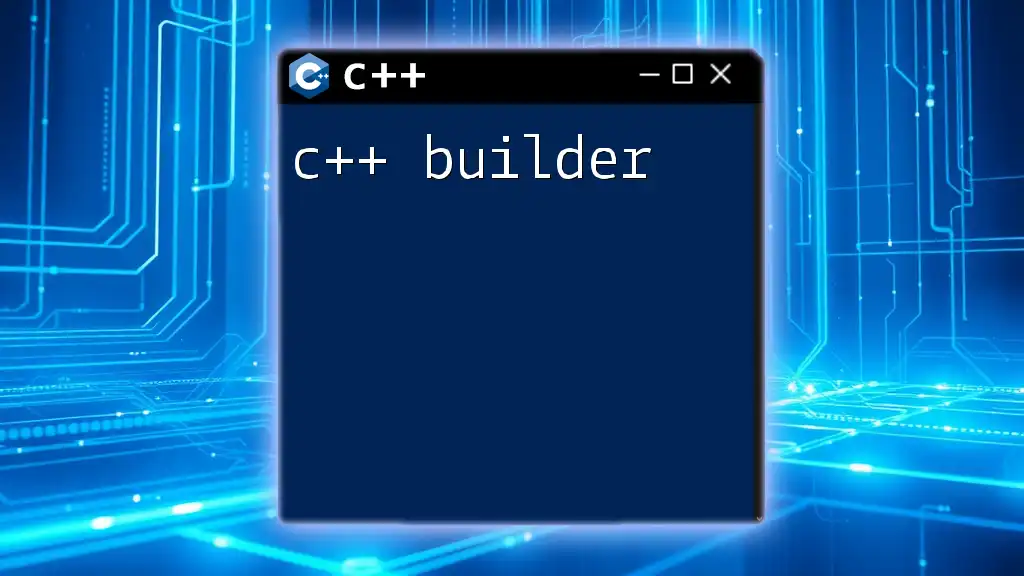
Call to Action
Dive into your C++ development journey! Explore available resources, share your experiences, and engage in discussions surrounding C++ browsers. The world of web development awaits your contributions!