In C++, a base class serves as a foundation for derived classes, enabling code reuse and polymorphism through inheritance.
class Base {
public:
void display() {
std::cout << "This is the base class." << std::endl;
}
};
class Derived : public Base {
public:
void show() {
std::cout << "This is the derived class." << std::endl;
}
};
Understanding the Basics of C++
C++ Syntax
The structure of a C++ program is essential to grasp before diving into coding. A basic C++ program includes several key components:
- Headers provide information to the compiler about the functions and definitions used throughout the program.
- The main function serves as the entry point for execution. Every C++ program must have one.
- Statements contain the actual code that performs operations.
Here’s a simple example illustrating these components:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
In this example, `#include <iostream>` allows us to use standard input and output streams. The `main()` function contains a print statement that outputs "Hello, World!" to the screen and a return statement that ends the program.
Data Types in C++
Understanding data types is fundamental in any programming language, specifically in C++. C++ supports various built-in data types:
- Fundamental Data Types: Key examples include:
- `int` for integers,
- `float` for floating-point numbers,
- `double` for double-precision floating-point numbers,
- `char` for single characters.
A simple demonstration of defining these data types would be:
int age = 25;
float height = 5.9;
char grade = 'A';
- User-Defined Data Types: Beyond the built-in data types, C++ allows defining custom types using:
- struct: Useful for grouping related variables.
- class: A more advanced structure used in Object-Oriented Programming (OOP).
- enum: Used to define variables that can hold discrete values.
For example, consider a `struct`:
struct Person {
std::string name;
int age;
};
This `struct` defines a `Person` with a name and age, enabling organized data handling.
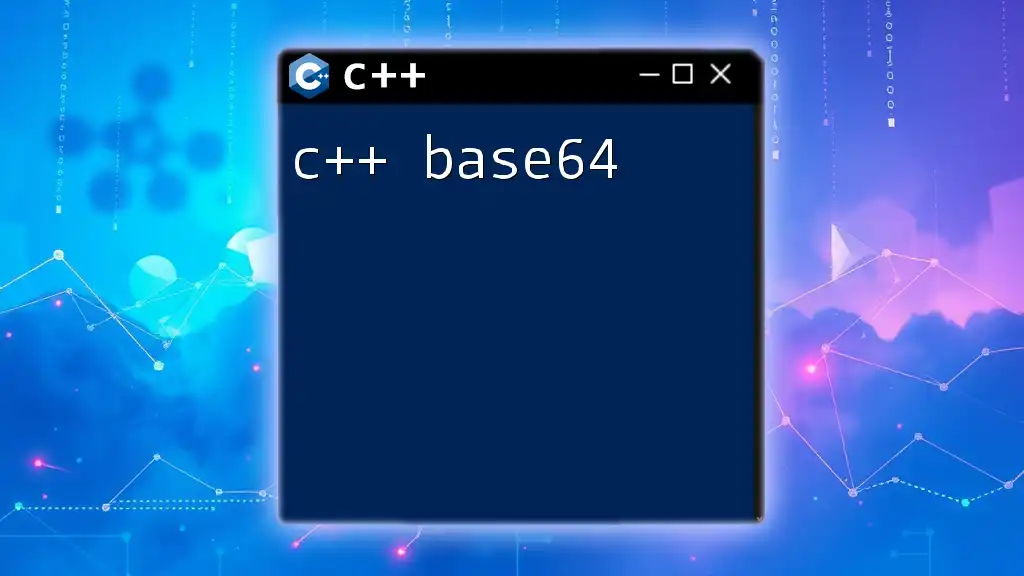
C++ Control Structures
Conditional Statements
Control structures are pivotal in determining the flow of execution in a program. The most common conditional statements in C++ are `if`, `else if`, and `else`.
An example of usage:
if (age >= 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
In this scenario, if `age` is 18 or more, it prints "Adult"; otherwise, it outputs "Minor".
Another powerful feature is the switch case, which is particularly beneficial for handling multiple conditions. An example is as follows:
switch (grade) {
case 'A':
std::cout << "Excellent!";
break;
case 'B':
std::cout << "Good!";
break;
default:
std::cout << "Keep trying!";
}
This structure checks the value of `grade` and prints a corresponding message.
Loop Structures
Loops execute a set of instructions multiple times based on certain conditions. The primary loop structures in C++ include `for`, `while`, and `do while`.
- for Loop: Ideal for situations where the number of iterations is known in advance. An example:
for (int i = 0; i < 10; i++) {
std::cout << i;
}
This code prints numbers from 0 to 9.
- while Loop: Best used when the number of iterations isn't determined beforehand.
int i = 0;
while (i < 10) {
std::cout << i;
i++;
}
- do while Loop: Similar to the while loop but ensures at least one execution of the loop body.
int j = 0;
do {
std::cout << j;
j++;
} while (j < 10);
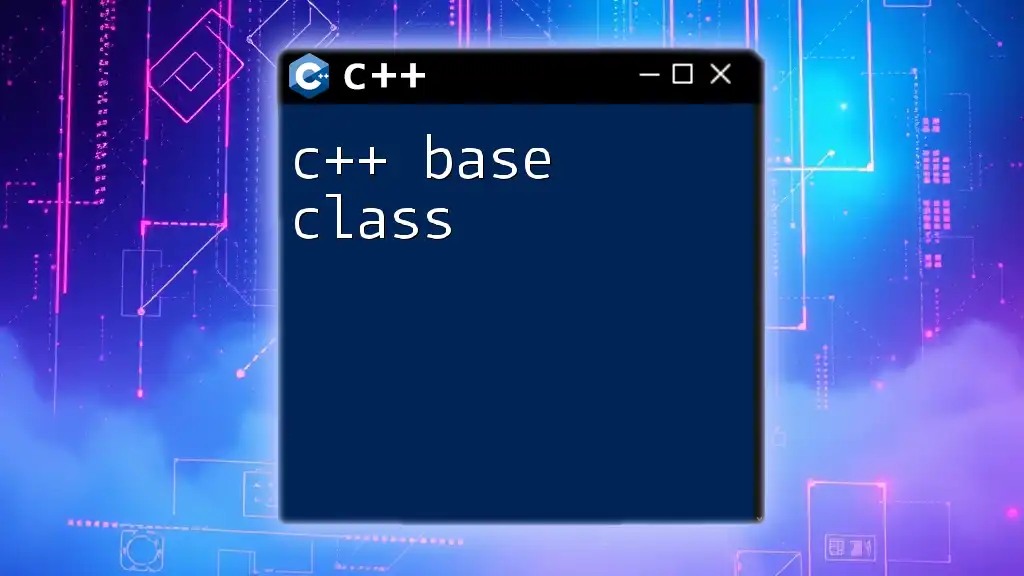
Functions in C++
Definition and Purpose
Functions are crucial for breaking down code into manageable blocks, promoting reusability. A function in C++ is a block of code that performs a specific task.
Function Syntax
To define a function, you specify a return type, a name, and parameters (if any), followed by the body of the function.
Here's a straightforward example of defining and calling a function:
void greet() {
std::cout << "Hello!";
}
int main() {
greet();
return 0;
}
In this example, `greet()` is a function that prints "Hello!". It is then called within the `main()` function.
Understanding how to use function parameters and return types is also essential. You can pass arguments by value or reference. Here’s an example demonstrating pass by reference:
void increment(int &number) {
number++;
}
This function increments the value of `number` directly, altering the original variable.
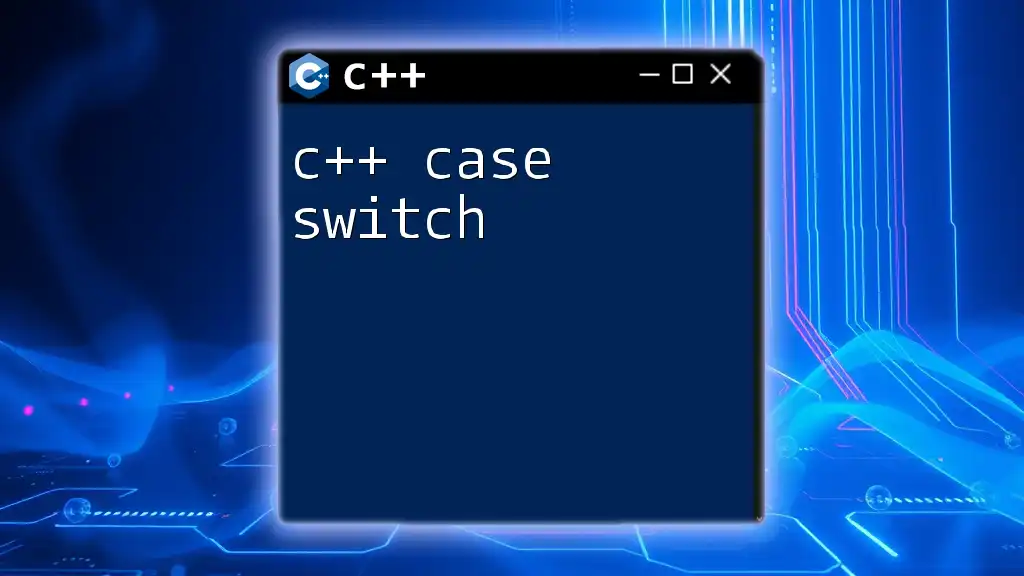
Object-Oriented Programming in C++
Classes and Objects
C++ is fundamentally an object-oriented programming language, allowing developers to create classes and objects. A class is a blueprint for creating objects, encapsulating attributes and behaviors.
Here's how to define a class:
class Animal {
public:
void sound() {
std::cout << "Generic Animal Sound";
}
};
This `Animal` class includes a method, `sound()`, demonstrating its behavior.
Inheritance
One of the powerful features of OOP is inheritance, which allows the creation of new classes from existing ones. Classes can inherit attributes and methods from parent classes, enhancing code reusability.
- Single Inheritance: A derived class inherits from one base class:
class Dog : public Animal {
public:
void sound() {
std::cout << "Bark";
}
};
Here, `Dog` inherits from `Animal` and overrides the `sound()` method to provide specific behavior.
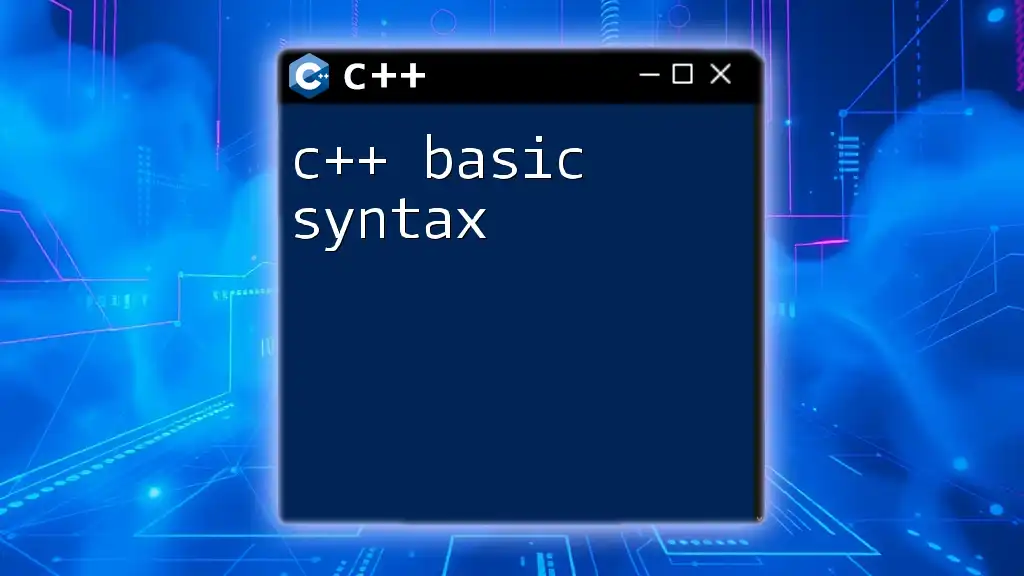
C++ Standard Library
Overview of STL
The Standard Template Library (STL) is a vital part of C++ that provides a set of common classes and functions. With STL, you can effectively manage data through various container types and utilize algorithms for dynamic operations.
Commonly Used Functions and Containers
Two of the most commonly used containers in the STL are `vectors` and `strings`.
Using a `vector` is as simple as:
std::vector<int> numbers = {1, 2, 3};
This code snippet defines a vector containing the integers 1, 2, and 3.
You can leverage algorithms provided by the STL to manipulate these containers. For example, sorting the elements in a vector can be performed using the `sort()` function:
std::sort(numbers.begin(), numbers.end());
This command sorts the numbers in ascending order.
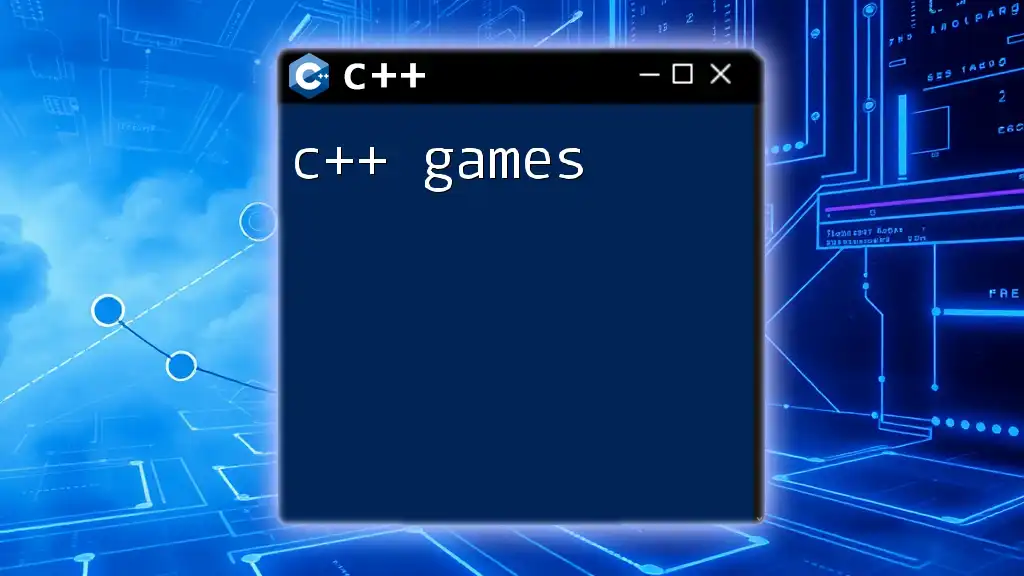
Conclusion
In summary, understanding the C++ base is crucial for leveraging its capabilities effectively in software development. By mastering essential concepts such as syntax, data types, control structures, functions, object-oriented programming, and the Standard Library, you position yourself for success as a C++ programmer.
Next Steps
As you continue your learning journey, consider exploring additional resources such as comprehensive textbooks, online courses, or community forums. Let your curiosity guide you, and engage in coding challenges to hone your skills further. Join our course to deepen your understanding and elevate your C++ prowess!