C++ allows inline assembly code through the `asm` keyword, enabling developers to write assembly instructions directly within C++ code for performance optimization.
Here’s a simple example of using inline assembly in C++:
#include <iostream>
int main() {
int a = 5, b = 10, result;
asm ("addl %%ebx, %%eax;"
: "=a"(result) // output
: "a"(a), "b"(b) // inputs
);
std::cout << "Result: " << result << std::endl;
return 0;
}
Understanding ASM in C++
What is ASM?
Assembly language, or ASM, is a low-level programming language that is closely related to machine code. In simple terms, it is a way to write instructions that a computer's CPU can understand directly. C++ ASM provides a bridge between high-level programming and the machine’s instruction set, enabling developers to manipulate hardware and performance parameters directly.
The importance of ASM in C++ cannot be overstated. It allows developers to fine-tune performance, implement hardware-level features, and gain insights into data processing that high-level C++ programming cannot provide. Use cases typically involve operations requiring maximum speed or specific optimizations, such as graphics rendering, scientific computations, or real-time systems.
Why Use ASM in C++?
There are several compelling reasons to incorporate ASM into your C++ projects:
- Performance Optimization: ASM can allow you to write more efficient code for critical sections where every CPU cycle matters.
- Access to Low-Level Hardware Features: You can directly manipulate hardware registers or use specific CPU instructions that are unavailable or inefficient when using only C++.
- Fine-Grained Control Over Execution: Inline assembly affords solutions that require specific timing and execution control.
- Integration with High-Level Language Constructs: C++ allows you to mix high-level constructs with ASM, granting flexibility in performance tuning.
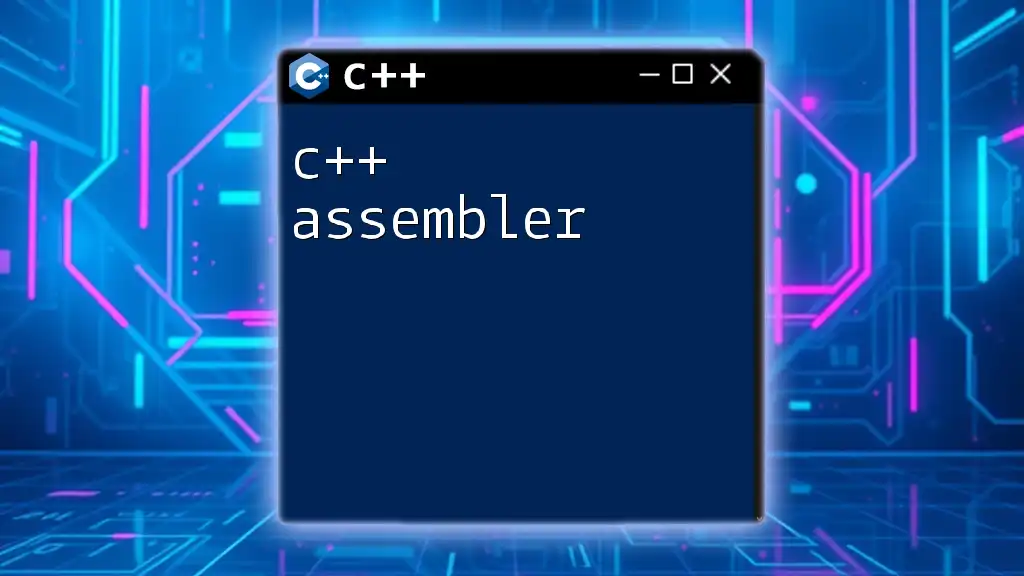
Setting Up Your C++ Environment for ASM
Compiler Support
Most modern C++ compilers offer comprehensive support for inline ASM. Popular choices include:
- GCC: Uses the `asm` directive for inline assembly.
- MSVC: Provides the `__asm` keyword for inline assembly statements.
- Clang: Compatible with GCC-style inline assembly as well.
To enable integration of ASM in your code, ensure you configure the compiler settings according to the documentation corresponding to the toolchain being used.
Development Tools
Choosing the right Integrated Development Environment (IDE) can improve your productivity when working with ASM in C++. Recommended IDEs include Visual Studio, Code::Blocks, and CLion, which offer debugging tools and syntax highlighting for assembly. Similarly, command-line tools like GDB (GNU Debugger) are invaluable for debugging assembly code effectively.
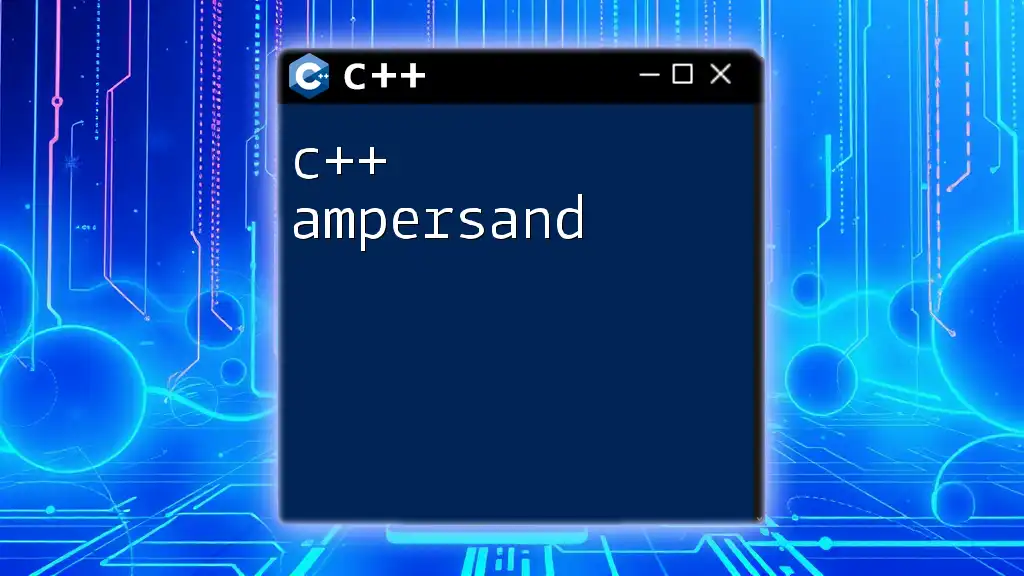
Inline Assembly in C++
Syntax of Inline Assembly
Inline assembly can be embedded directly into C++ code using the `asm` keyword. The basic syntax looks like this:
asm volatile ("assembly code here");
Using `volatile` prevents the compiler from optimizing away your assembly instructions—important for cases where every instruction is critical to execution.
Using Inline Assembly
Consider a simple example of using inline assembly to add two numbers:
int add(int a, int b) {
int result;
asm ("addl %%ebx, %%eax"
: "=a" (result)
: "a" (a), "b" (b));
return result;
}
In this snippet, we utilize the `addl` instruction of x86 assembly to add the values stored in registers. The output operand is specified as `result`, while `a` and `b` are provided as input operands. Each operand can be defined in the syntax using the appropriate prefix, with `a` denoting the EAX register and `b` representing EBX.
Input and Output Operands
Understanding how to properly approach inputs and outputs in inline assembly is critical. The operands can be hierarchically structured along with constraints that indicate where and how they should be used.
For example, in this snippet where a swap operation is performed:
void swap(int &a, int &b) {
asm ("xchg %0, %1"
: "=r" (a), "=r" (b)
: "0" (a), "1" (b));
}
Here, `%0` and `%1` represent the placeholders for the operands, which allow the assembler to swap the values of `a` and `b` in place. The `xchg` instruction exchanges the contents of the registers, demonstrating how ASM can effectively manipulate variables.
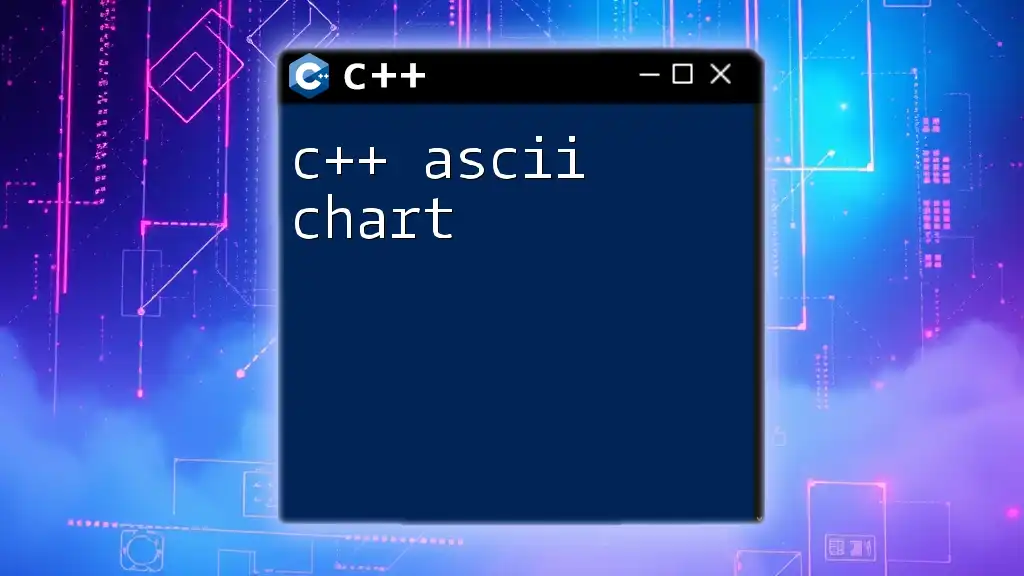
Integrating ASM with C++ Functions
Performance Considerations
When deciding whether to integrate ASM into your C++ application, it is vital to evaluate the performance impact. High-level language constructs can often provide performance that is sufficient for many applications without the complexity of assembly. However, when working on performance-intensive tasks, it’s crucial to leverage ASM where the compiler does not generate optimal code.
Case Study: Performance Tuning
Let’s take a look at an example of a Fibonacci computation to demonstrate performance differences. The recursive version might look like this:
int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
Using inline assembly, however, could lead to significant performance benefits, particularly in a high-demand context. By rewriting this algorithm using assembly, you could reduce overhead, leading to faster execution during runtime.
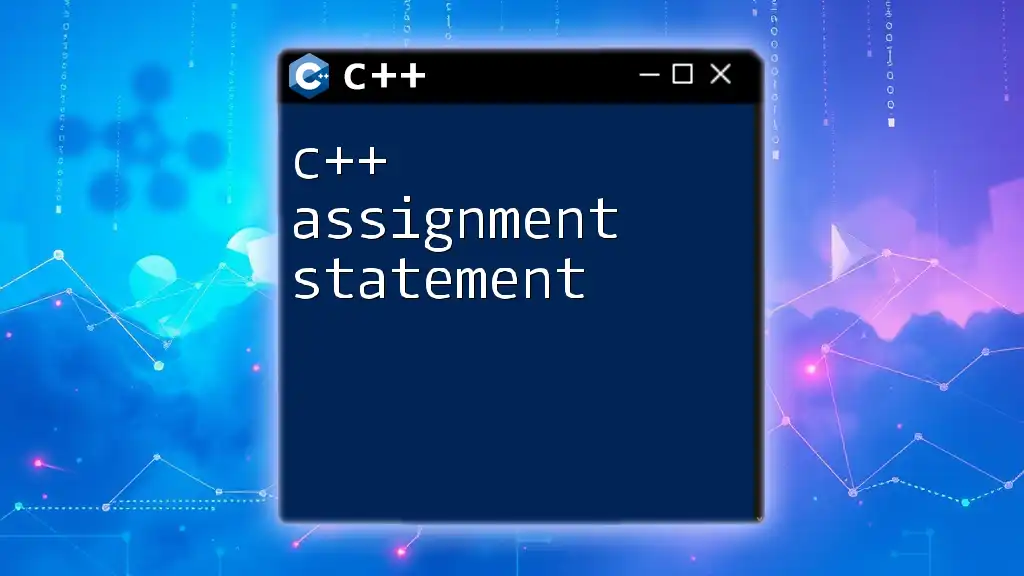
Advanced ASM Techniques
Creating Custom ASM Functions
You can create a standalone ASM function to encapsulate frequently-used assembly operations. Here’s an example illustrating how you might implement a simple ASM-based multiplier:
extern "C" int multiply(int a, int b) {
int result;
asm (
"imull %%ebx, %%eax;" // Multiply EAX by EBX
: "=a" (result) // output operand
: "a" (a), "b" (b) // input operands
);
return result;
}
This custom function utilizes the `imull` instruction to perform multiplication. By isolating this logic, it can cleanly be reused throughout your codebase.
Debugging ASM Code
Debugging assembly code can be tricky, but having a plan is essential. Common pitfalls include mismatched operand types or incorrect register assignments. Use debugging tools like GDB to step through your assembly and inspect register states. Carefully inserting comments in your ASM blocks can help maintain clarity and reduce the chance of errors.
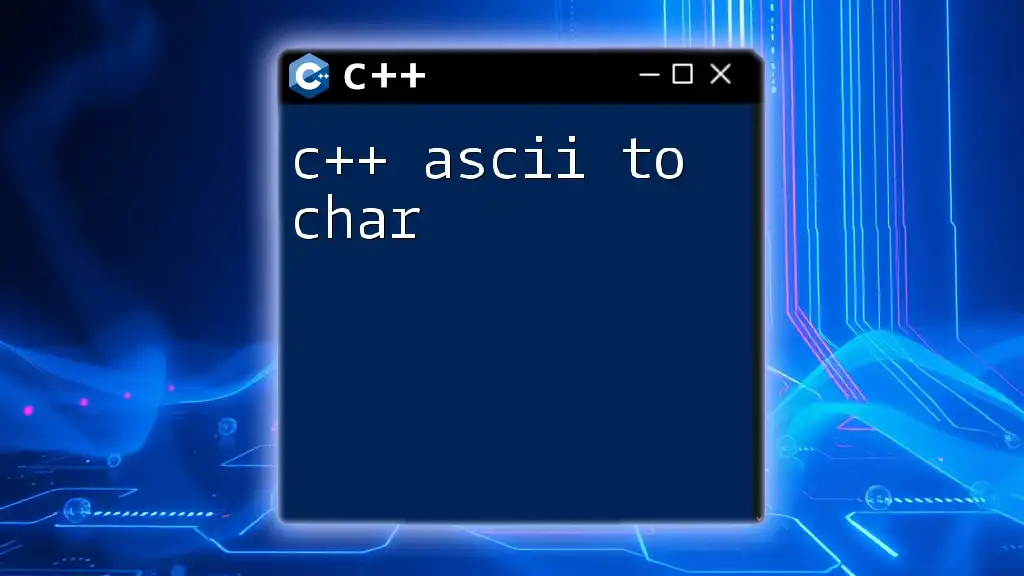
Best Practices for Using ASM in C++
Code Maintainability
When writing inline ASM, clarity of code becomes paramount. Use comments liberally to explain complex instructions or unusual patterns. Make your ASM blocks as legible as possible to facilitate future alterations, especially for team projects.
Portability Issues
One major challenge of using ASM is ensuring cross-platform compatibility. Assembly language is heavily reliant on the CPU architecture, which can lead to portability issues. You’ll want to ensure that any ASM code you write adheres to the architecture you are targeting, such as x86 or ARM, and be wary of using architecture-specific instructions unless absolutely necessary.
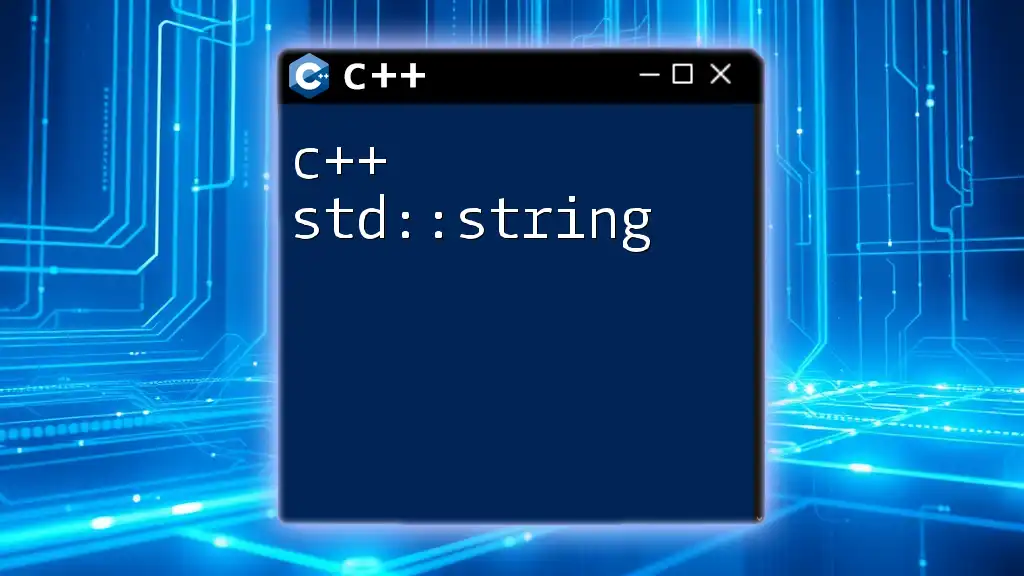
Future of ASM in C++
Trends in Modern CPU Architectures
Modern CPU architectures are evolving, with a strong push towards specialized processing units like GPUs and TPUs. These trends mean that while C++ ASM may be less utilized in everyday applications, certain fields (like game development or high-performance computing) may benefit greatly from blending ASM with C++ to leverage hardware optimizations.
Learning Resources
As you navigate the world of ASM, consider utilizing various resources to bolster your knowledge. Recommended readings include books like "Programming from the Ground Up" by Jonathan Bartlett, and various online courses about assembly language programming. Engaging with communities on platforms like Stack Overflow and Reddit can also provide valuable insights and peer support.
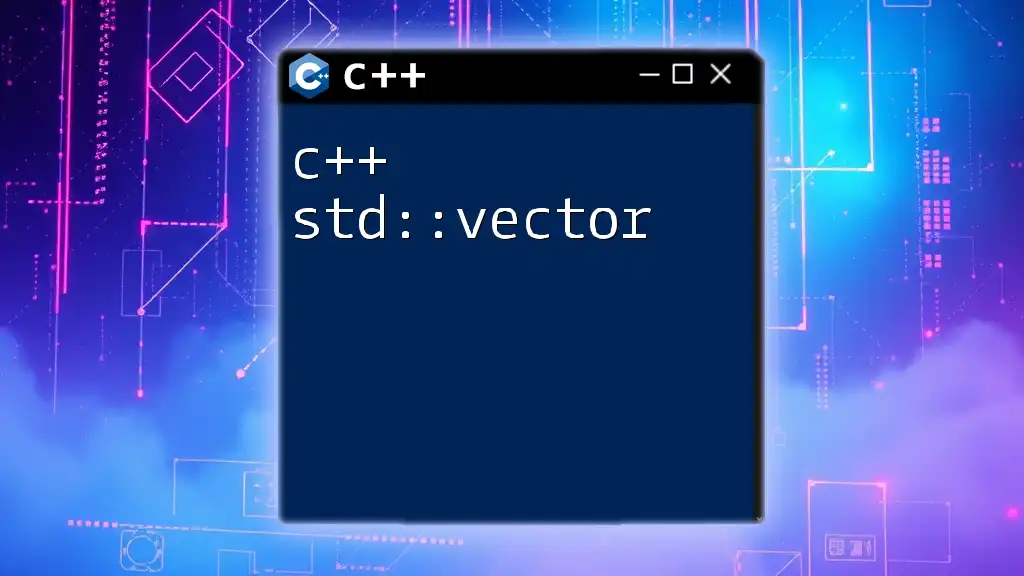
Conclusion
Understanding C++ ASM opens a new dimension of programming that integrates low-level efficiency into high-level constructs. By experimenting with inline assembly, developers can achieve optimal performance gains in specific scenarios, opening a world of possibilities in hardware-manipulation and speed-optimization. Whether you're tuning performance-critical applications or delving into the intricacies of low-level operations, ASM is a powerful tool in your programming toolkit.