An ASCII chart in C++ provides a visual representation of ASCII characters and their corresponding integer values, allowing users to easily reference and utilize these characters in their programming. Here's a simple code snippet to display the ASCII values for characters from 32 to 126:
#include <iostream>
int main() {
std::cout << "ASCII Value Chart:\n";
for (int i = 32; i <= 126; i++) {
std::cout << "Character: " << static_cast<char>(i) << " -> ASCII: " << i << std::endl;
}
return 0;
}
What is ASCII?
ASCII, which stands for American Standard Code for Information Interchange, is a character encoding standard widely used in computers and other electronic devices. First developed in the early 1960s, ASCII became a crucial component in enabling different machines to communicate with each other using a common set of characters.
The Importance of ASCII in C++
In C++ programming, understanding ASCII is essential because many characters, strings, and text manipulations rely on ASCII values. ASCII values allow programmers to perform various operations such as sorting, comparison, and graphical representation of data, making it a foundational concept in computer science.

Understanding the ASCII Chart
Structure of the ASCII Chart
The ASCII chart consists of 128 characters divided into two main categories: Control Characters and Printable Characters. Control characters include non-printable characters that control how text is processed, while printable characters are those that represent symbols and letters you interact with directly. A visual representation typically lays out these characters corresponding to their decimal values.
ASCII Decimal, Hexadecimal, and Binary Values
ASCII characters can be expressed in various numerical systems:
- Decimal: Base 10 representation commonly used in everyday life.
- Hexadecimal: Base 16 representation utilized in programming, especially in memory addresses.
- Binary: Base 2 representation fundamental to computer operation.
For example, the ASCII character 'A' has:
- Decimal value: 65
- Hexadecimal value: 41
- Binary value: 01000001
To illustrate these conversions in C++, you can create a program that demonstrates how to display the different formats for a given character.
Printable vs. Control Characters
What are Control Characters?
Control characters are non-printable and used to control how text is processed. Some of the most common control characters include:
- NULL (0): Often used as a string terminator.
- Backspace (8): Moves the cursor backward.
- Escape (27): Used to initiate escape sequences.
What are Printable Characters?
Printable characters, on the other hand, are the letters, digits, and symbols that we can see and use in everyday text. Examples include 'A', 'a', '1', '?', etc. Each of these characters has a corresponding ASCII value that can be referenced in programming.

Working with ASCII in C++
Accessing ASCII Values in C++
You can easily retrieve ASCII values of characters in C++ using typecasting. For example, if you want to find the ASCII value of the character 'A', you can use the following code snippet:
#include <iostream>
int main() {
char c = 'A';
std::cout << "ASCII value of " << c << " is " << int(c) << std::endl;
return 0;
}
In this code, we cast the character 'A' to an integer, allowing us to print its ASCII value.
Using ASCII for Character Manipulation
C++ provides several built-in functions for character manipulation that rely on ASCII values. For instance, you can change the case of characters using functions like `toupper()` and `tolower()`. Here’s a code example that demonstrates how to convert a lowercase letter to uppercase:
#include <iostream>
#include <cctype>
int main() {
char lower = 'b';
char upper = toupper(lower);
std::cout << "Uppercase of " << lower << " is " << upper << std::endl;
return 0;
}
Generating an ASCII Table in C++
Creating an ASCII table programmatically can help you understand the character encoding better. The following code will generate a complete ASCII table, printing out both the ASCII values and their corresponding characters:
#include <iostream>
int main() {
std::cout << "ASCII Table:\n";
for (int i = 0; i < 128; i++) {
std::cout << i << " : " << char(i) << std::endl;
}
return 0;
}
Executing this code will display a comprehensive table, promoting your understanding of the various ASCII values and their meanings.

ASCII Art in C++
What is ASCII Art?
ASCII art is a graphic design technique that uses printable characters from the ASCII standard to create visual representations of images or text. It showcases creativity, turning simple characters into intricate designs, often seen in programming communities.
Creating ASCII Art with C++
Creating ASCII art in C++ involves using string manipulation and outputting characters in specific arrangements to create a visual representation. Below is a simple example that draws a face using ASCII characters:
#include <iostream>
void printFace() {
std::cout << " ^ \n";
std::cout << " (o) \n";
std::cout << " ~ \n";
}
int main() {
printFace();
return 0;
}
This basic code snippet highlights how to structure an ASCII art design in a C++ program.
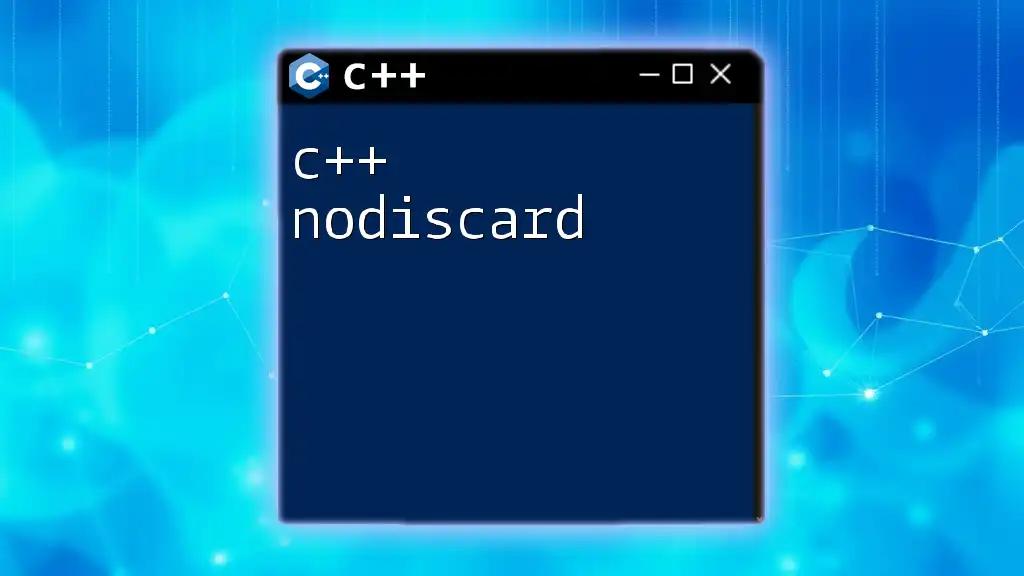
Practical Applications of ASCII in Software Development
Text File Handling
ASCII plays a vital role in reading and writing text files. You can create and manipulate .txt files using ASCII, as demonstrated in the following example:
#include <fstream>
#include <iostream>
int main() {
std::ofstream out("example.txt");
out << "Hello, ASCII World!" << std::endl;
out.close();
std::ifstream in("example.txt");
std::string line;
while (std::getline(in, line)) {
std::cout << line << std::endl;
}
in.close();
return 0;
}
In this code, we are writing to and reading from a text file, showcasing how ASCII characters form the basis of data stored in these files.
Data Transmission
ASCII also plays a critical role in data transmission, especially in networking protocols. When data is sent over a network, it often gets encoded in ASCII, facilitating compatibility across different systems and platforms. This ensures that messages are readable and interpretable regardless of where they originate.
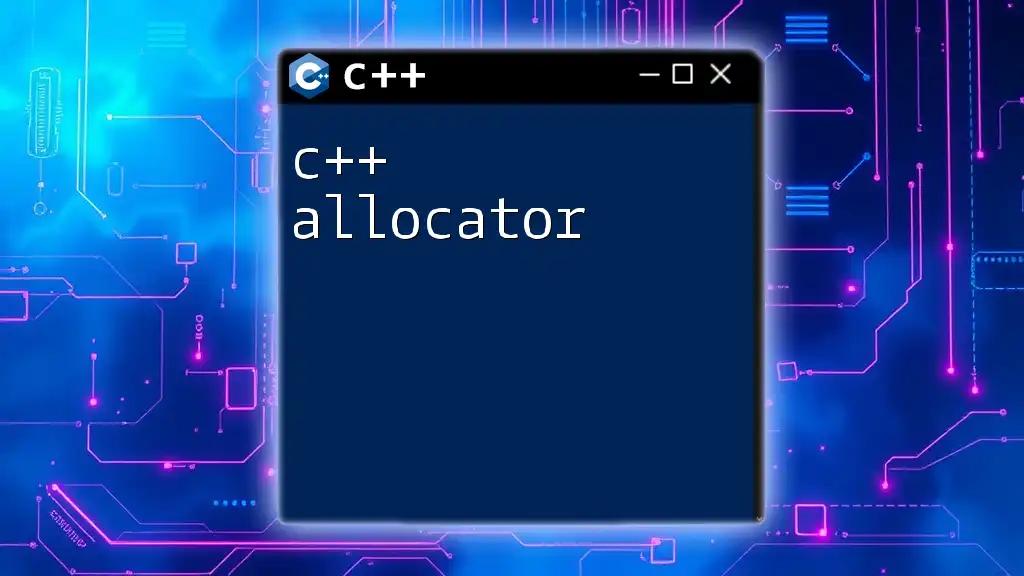
Conclusion
In summary, the C++ ASCII chart is a foundational aspect of programming that allows developers to leverage character codes for a wide range of applications. Understanding ASCII values not only improves your proficiency in C++ but also enhances your capability to manipulate data effectively.
Further Resources
For those looking to deepen their understanding of ASCII and its applications in C++, various resources are available. Books that cover character encoding and C++ fundamentals can provide additional insights, while many online tutorials offer practical exercises and examples related to C++ programming.
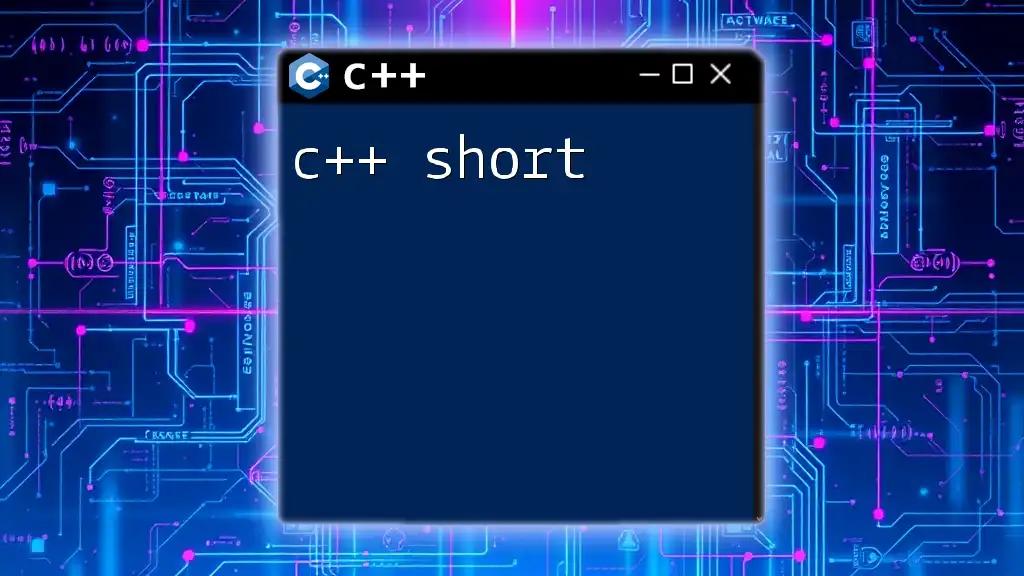
Frequently Asked Questions (FAQs)
What is the ASCII value of a newline character?
The ASCII value for a newline character is 10.
How many characters does the standard ASCII chart include?
The standard ASCII chart includes 128 characters ranging from values 0 to 127.
Can I use non-ASCII characters in C++? How?
Yes, you can use non-ASCII characters in C++ programming. However, you will need to ensure that your text encoding supports those characters, such as UTF-8. You can use libraries that handle Unicode to work with a wider range of characters.