In C++, an accumulator is a variable that sequentially adds values together, often used in loops to compute a total sum.
Here’s a simple code snippet demonstrating an accumulator in action:
#include <iostream>
int main() {
int sum = 0;
for (int i = 1; i <= 10; ++i) {
sum += i; // Accumulate the sum of numbers from 1 to 10
}
std::cout << "The total sum is: " << sum << std::endl;
return 0;
}
What is an Accumulator?
An accumulator is a programming concept used to maintain a running total or cumulative value as a collection of data is processed. Rather than storing data in isolation, an accumulator updates its value based on new inputs or operations. This process is fundamental in programming since it allows for the aggregation of results from functions or inputs into a single variable.
In the context of C++, accumulators play a vital role in operations ranging from simple arithmetic calculations to complex data analysis. By utilizing accumulators, programmers can efficiently handle data without needing to maintain discrete collections or variables for each individual data point.
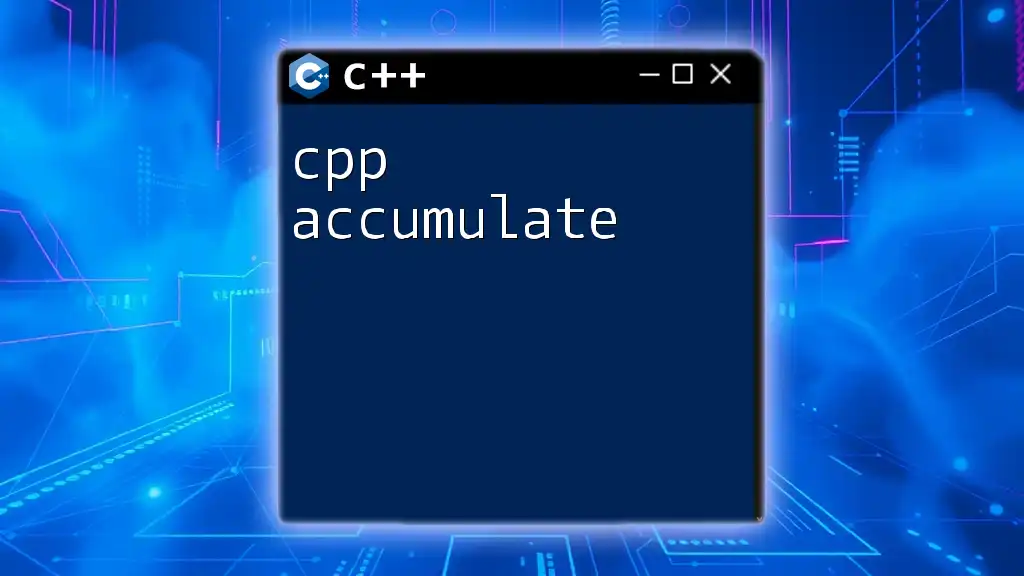
Importance of Accumulators in C++
Understanding the significance of accumulators in C++ goes beyond just keeping tabs on totals. Accumulators can enhance both program performance and readability. They allow developers to streamline their code by reducing the number of variables needed and concentrating the logic into a single, manageable entity.
For practical applications, consider scenarios such as calculating scores in games, summing customer purchases, or even gathering statistics in data analysis. In these contexts, using an accumulator simplifies code, enhances clarity, and potentially improves performance.
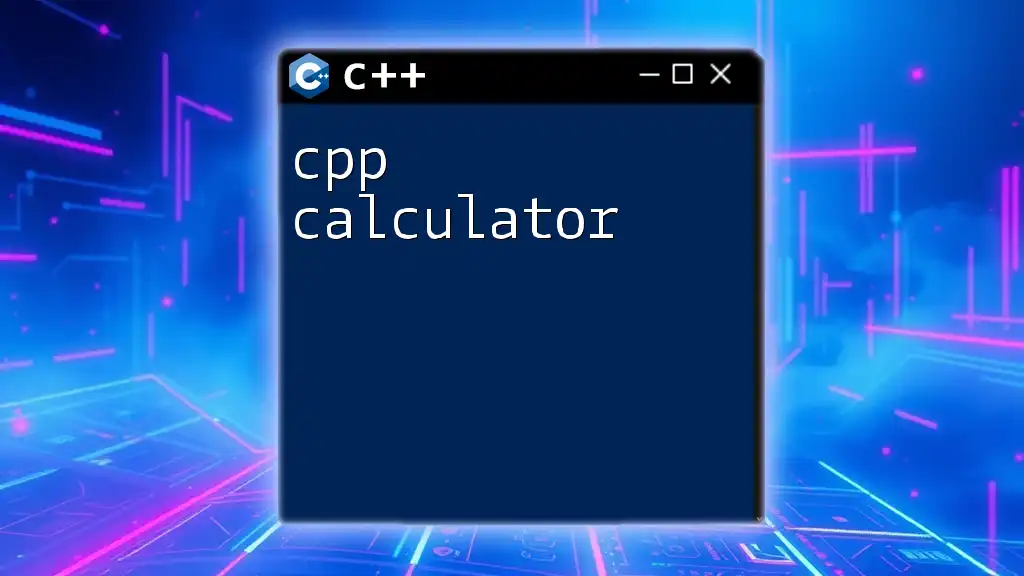
How C++ Accumulators Work
Basic Concept of Accumulation
Accumulation involves generating a total or a composite value from a stream of inputs. It typically employs a loop structure where a variable (the accumulator) is updated repeatedly based on user inputs or calculations. Unlike aggregation, which often combines several data points into a single output without keeping track of individual values, accumulation focuses on a continual tally.
Types of Accumulators
-
Numeric Accumulators: These accumulate values through arithmetic operations, typically for summing up numbers, calculating averages, or performing statistical functions.
-
String Accumulators: These concern the concatenation of strings to create a single longer string. This can be particularly useful in scenarios such as building user inputs or generating textual outputs from various sources.
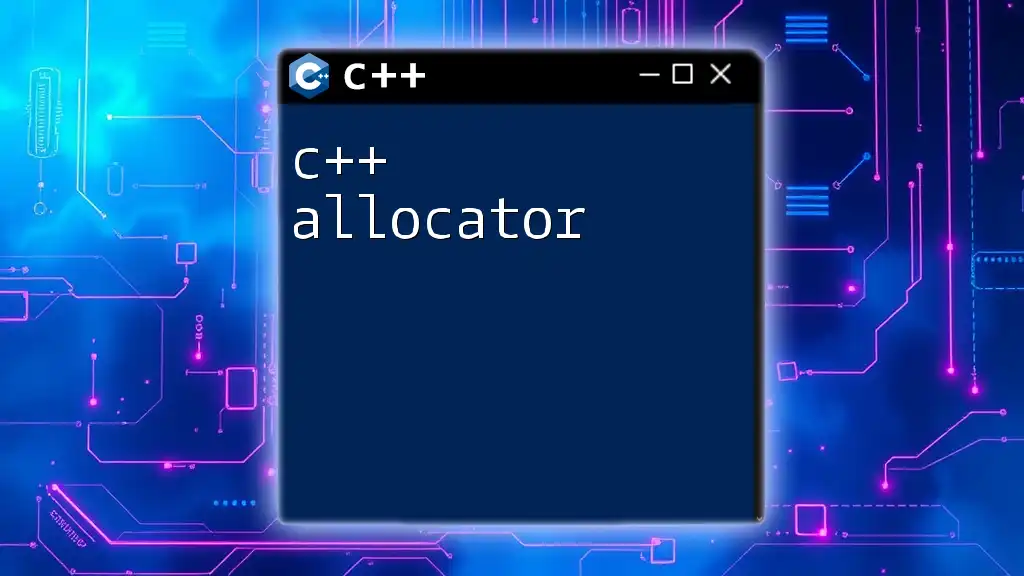
Implementing Accumulators in C++
Setting Up Your C++ Environment
To get started with C++ program development, you'll need an Integrated Development Environment (IDE) or a suitable compiler. Some of the most popular options include:
- Code::Blocks
- Visual Studio
- CLion
Setting up one of these environments allows you to run and debug C++ code seamlessly.
Creating a Simple Numeric Accumulator
Let’s illustrate a simple numeric accumulator example. Below is a C++ code snippet that sums a series of integers until the user inputs zero:
#include <iostream>
using namespace std;
int main() {
int total = 0; // Declare accumulator variable
int num;
cout << "Enter numbers to accumulate (0 to stop): " << endl;
while (true) {
cin >> num;
if (num == 0) break; // Stop condition
total += num; // Accumulate
}
cout << "Total sum: " << total << endl; // Output result
return 0;
}
Explanation of Code: Here, we initialize an integer variable `total` to hold the cumulative sum, starting at zero. The `while` loop repeatedly prompts the user for input. If the user enters zero, the loop terminates. During each iteration, the inputted number is added to `total`, demonstrating how the accumulator updates with each user input.
Creating a String Accumulator
Another relevant example is accumulating strings. Below is a C++ code snippet that concatenates strings until the user decides to quit:
#include <iostream>
#include <string>
using namespace std;
int main() {
string result; // Initialize string accumulator
string input;
cout << "Enter strings to accumulate (type 'quit' to stop):" << endl;
while (true) {
getline(cin, input); // Take multi-word inputs
if (input == "quit") break; // Stop condition
result += input + " "; // Accumulate strings
}
cout << "Accumulated string: \"" << result << "\"" << endl;
return 0;
}
Explanation of Code: This snippet initializes a `string` variable `result` to store the accumulated strings. The program uses `getline` to read full lines of input, allowing for multi-word inputs. It checks for the keyword "quit," and upon its appearance, the program outputs the accumulated string.
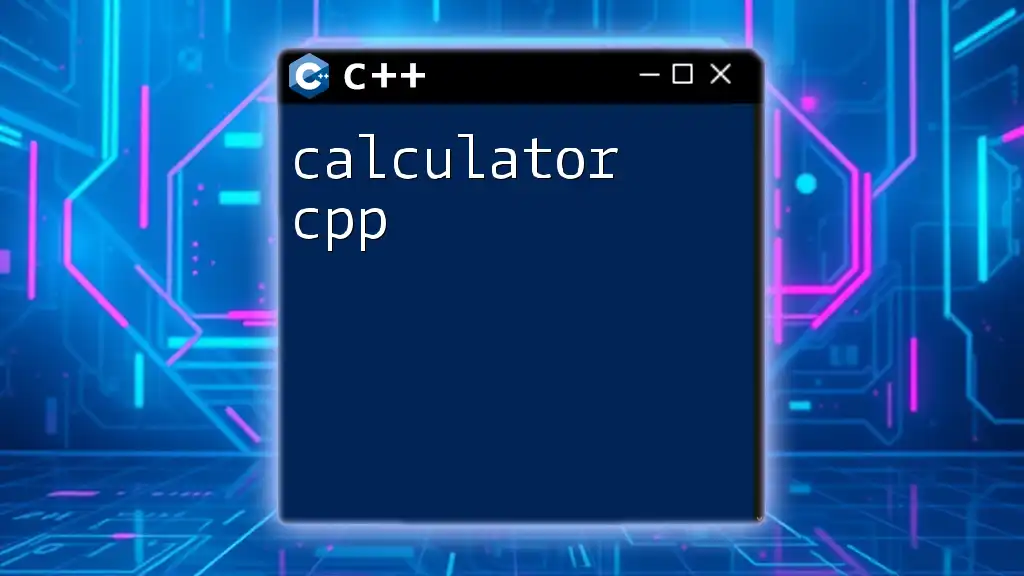
Best Practices for Using Accumulators
Managing Data Types Efficiently
Always choose the most appropriate data type for your accumulator based on the type of data you're handling. Utilizing variables like `int` or `double` for numeric accumulators and `string` for text accumulation enables optimal memory usage and performance.
Minimizing Errors in Accumulation
Common issues may arise, such as overflow errors in numeric accumulators or unexpected concatenation in string accumulators. To avoid these, implement proper validations, including type checks and volume limits on input. Debugging tools can help identify logic errors and ensure the code performs as expected.
Performance Tips
When dealing with large datasets, consider using optimized loops and minimizing repeated calculations within loops. Structure your code to process data in bulk when possible, rather than item-by-item.
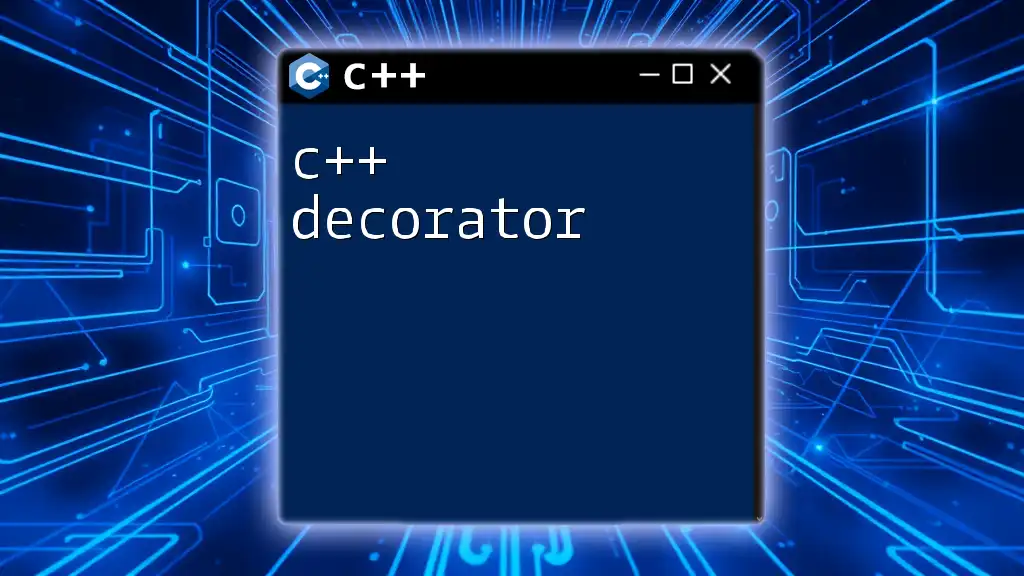
Advanced Accumulator Techniques
Using Accumulators with Collections
Collections such as vectors offer substantial benefits for dynamic data storage and manipulation. Below is an example of how to use an accumulator with a vector:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> numbers = {3, 5, 1, 6, 2}; // Example dataset
int total = 0;
for (int num : numbers) {
total += num; // Accumulate values in a vector
}
cout << "Total of vector elements: " << total << endl;
return 0;
}
Explanation of Code: This snippet initializes a `vector` containing a set of integer values. By using a range-based for loop, the program accumulates these values into `total`, showcasing how collections can simplify accumulation tasks.
Combining Multiple Accumulators
It is also possible to use multiple accumulators for more complex calculations. Here’s an example where we calculate both the sum and the average:
#include <iostream>
using namespace std;
int main() {
int sum = 0, count = 0;
double average;
cout << "Enter numbers (0 to stop):" << endl;
int num;
while (true) {
cin >> num;
if (num == 0) break;
sum += num; // Accumulate sum
count++; // Increment count
}
if (count > 0) {
average = static_cast<double>(sum) / count; // Compute average
cout << "Sum: " << sum << ", Average: " << average << endl;
} else {
cout << "No numbers were entered." << endl;
}
return 0;
}
Explanation of Code: In this example, we maintain two accumulators: `sum` for the cumulative total and `count` for the number of entries. After the loop, we check if any numbers were entered before calculating the average. This showcases how multiple accumulators can be effectively integrated for enriched data handling.
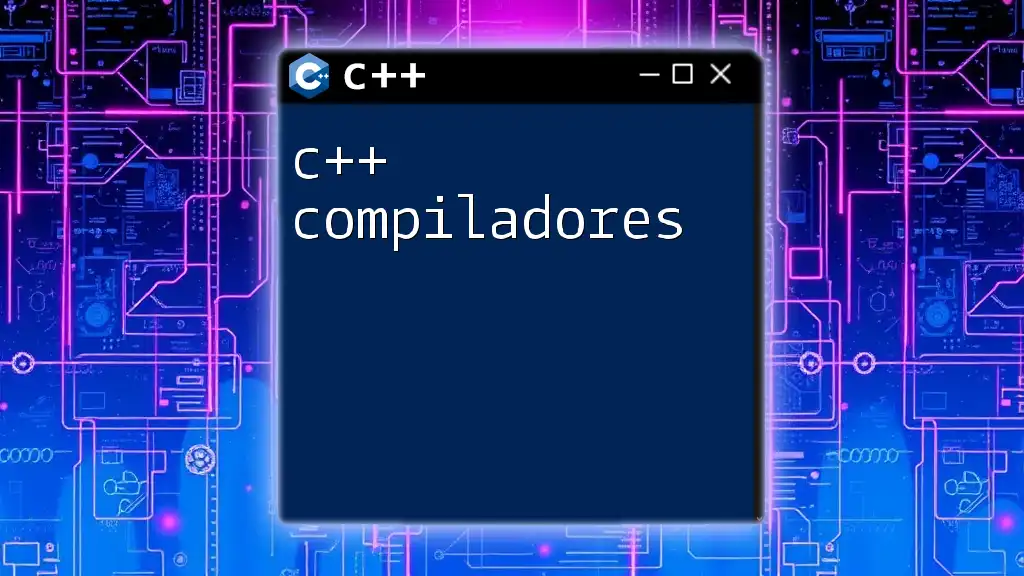
Real-World Applications of Accumulators
Use Cases in Data Analytics
In data analytics, accumulators help compile and analyze data points from various sources. For instance, summing sales data from CSV files often requires maintainability and clarity that accumulators provide. This practice not only streamlines code but also improves performance in data processing tasks.
Use in Game Development
In game development, accumulators are crucial for maintaining player statistics, such as scores. They can also track entities like resource collections over time, enabling dynamic game mechanics and feedback based on player actions. The clarity from using accumulators simplifies logic during game loops.
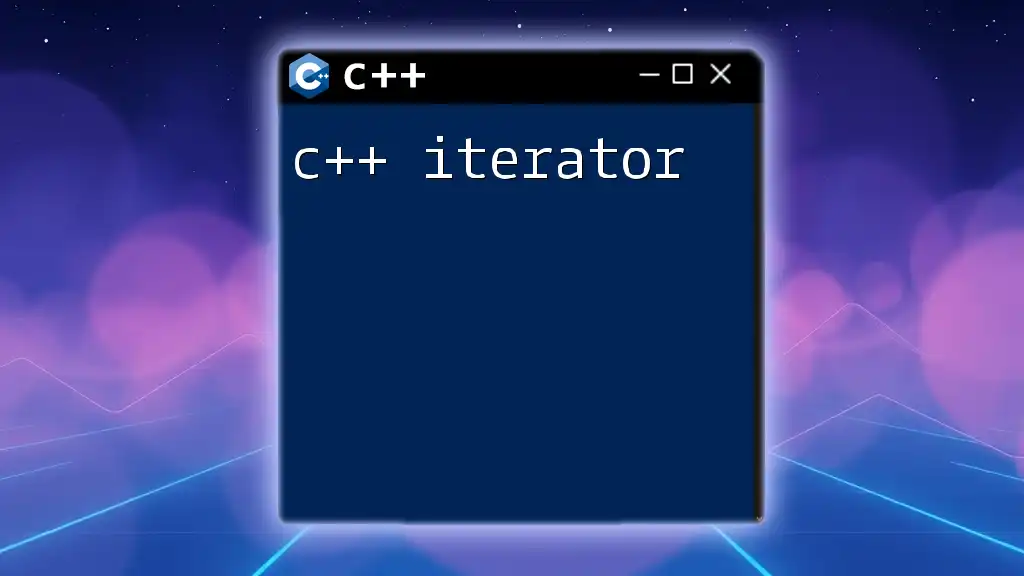
Conclusion
Accumulators in C++ offer a powerful and efficient means to handle cumulative data processing. Whether you are summing numbers, concatenating strings, or working with collections, understanding how to utilize accumulators enhances both your coding skills and your program's performance.
By practicing accumulator concepts and integrating them into projects, you can build more efficient algorithms and improve your understanding of data management in programming.
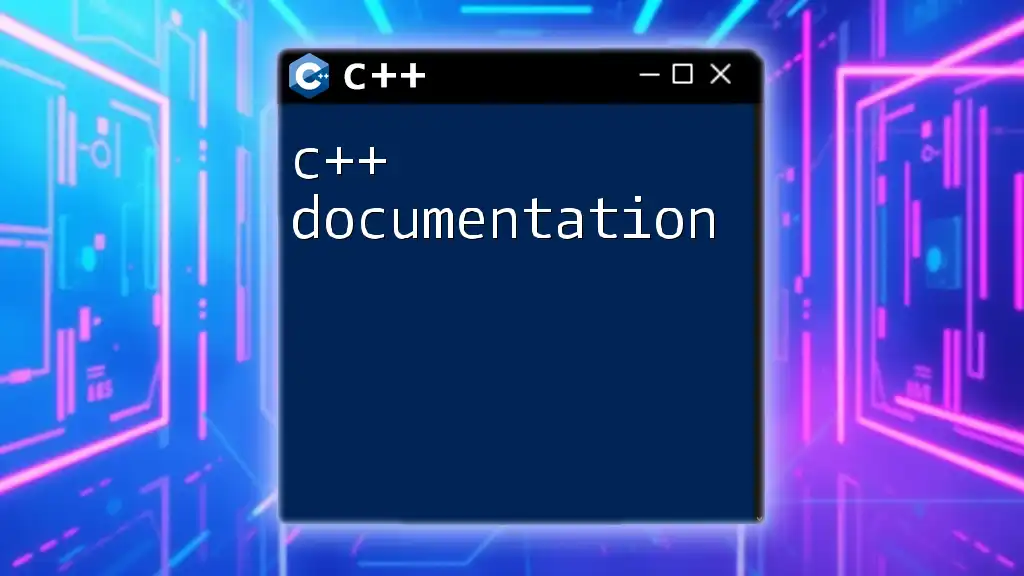
Additional Resources
For further learning, consider exploring books focused on C++ programming and online courses available on platforms like Coursera and Udacity. Engaging with community forums like Stack Overflow or Reddit can also provide invaluable support and opportunities to ask questions as you advance your skills with C++.