C++ modular programming encourages dividing your code into separate, self-contained modules that can be developed, tested, and maintained independently, improving readability and reusability.
Here's a simple example of a module in C++:
// math_module.h
#ifndef MATH_MODULE_H
#define MATH_MODULE_H
int add(int a, int b);
int subtract(int a, int b);
#endif // MATH_MODULE_H
// math_module.cpp
#include "math_module.h"
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
// main.cpp
#include <iostream>
#include "math_module.h"
int main() {
int sum = add(5, 3);
int difference = subtract(5, 3);
std::cout << "Sum: " << sum << ", Difference: " << difference << std::endl;
return 0;
}
What is Modular Programming?
Modular programming is a software design technique that emphasizes separating a program into smaller, manageable, and independently developed components, typically called modules. Each module can encapsulate its functionality, making it easier to develop, test, maintain, and improve.
In C++, modular programming allows developers to organize their code into logical segments, which fosters better code maintenance and collaboration among team members. This approach makes large codebases more manageable and reduces the time taken to incorporate changes.
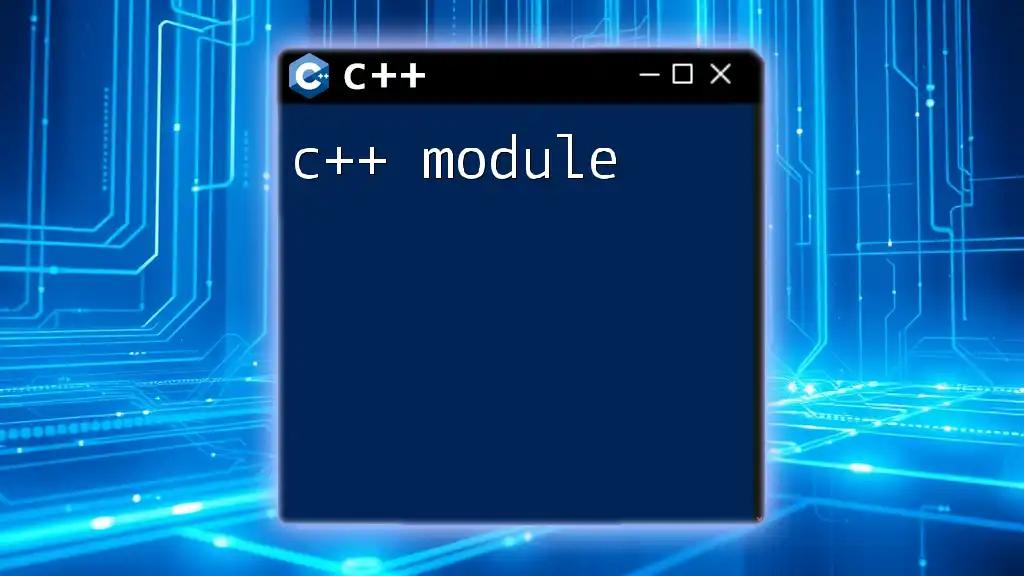
Benefits of Using Modular Programming in C++
Using the C++ modular approach provides numerous benefits that enhance both individual and team productivity:
-
Improved Code Readability: By breaking down a large application into several modules, the code becomes easier to read and understand. Each module serves a specific purpose, making it clear what role it plays within the larger application context.
-
Easier Maintenance and Debugging: When changes need to be made or bugs corrected, it's far simpler to modify a single module rather than wading through a massive codebase. This modularity allows developers to focus on specific areas without unintended side effects.
-
Enhanced Collaboration in Teams: Multiple developers can work on different modules simultaneously, reducing bottlenecks. Each developer can focus on their piece without interfering with others' work.
-
Code Reusability: Once a well-defined module is created, it can be reused in different programs or projects, leading to increased efficiency and decreased time spent troubleshooting recurring issues.

Core Concepts of C++ Modular Programming
Understanding C++ Modules
The concept of modules in C++ represents a sophisticated method of organizing code. Traditional C++ programming primarily relies on header files and implementation files. With the introduction of C++20, the language supports a more structured and powerful approach via modules, which enhance compile-time efficiency and reduce visibility issues.
The Structure of a Module
A module generally consists of two parts: the interface and the implementation. The interface defines how other parts of the program may interact with this module, while the implementation contains the actual code that performs the operations specified by the interface.
Example of a simple module structure:
// math_utils.ixx - Module interface
export module math_utils;
export int add(int a, int b);
export int subtract(int a, int b);
// math_utils.cpp - Module implementation
module math_utils;
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
Module Interface Files
Module interface files outline the functions and types that are available to other modules or components of the program. They define the public API of a module.
Code Snippet: Creating a module interface file:
// math_utils.ixx
export module math_utils;
export int multiply(int a, int b);
export int divide(int a, int b);
The `export` keyword signifies that these functions can be used outside the module.
Module Implementation Files
On the other hand, module implementation files contain the actual code that fulfills the functionality outlined in the interface files. It is in these files where the details of how each operation is performed are defined.
Code Snippet: Creating a module implementation file:
// math_utils.cpp
module math_utils;
int multiply(int a, int b) {
return a * b;
}
int divide(int a, int b) {
if (b == 0) throw std::invalid_argument("Cannot divide by zero");
return a / b;
}

Creating Your First C++ Module
Step-by-Step Guide to Building a Module
Getting started with modules involves a few straightforward steps. Below is a guide on how to create a simple math_utils module.
- Set Up Your Development Environment: Ensure you have access to a C++20-compliant compiler.
- Create the Module Interface: Write out the functions you plan to export.
- Implement the Functionality: Add the definitions of functions.
- Compile the Module: Ensure the module compiles without errors.
Example: Create a module called `math_utils`:
- Create the file `math_utils.ixx` for the interface.
- Create the file `math_utils.cpp` for the implementation.
Importing Modules
To utilize the functionality defined in a module, you need to import it into your code.
Code Snippet: Using `import` with your custom module:
import math_utils;
int main() {
int sum = add(5, 3);
int product = multiply(4, 2);
return 0;
}
Example: A Simple Calculator Module
In this section, we will implement a simple calculator module that allows for basic arithmetic operations.
Code Snippet: Implementing basic operations:
// calculator.ixx
export module calculator;
export int add(int a, int b);
export int subtract(int a, int b);
export int multiply(int a, int b);
export int divide(int a, int b);
// calculator.cpp
module calculator;
#include <stdexcept>
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
int multiply(int a, int b) {
return a * b;
}
int divide(int a, int b) {
if (b == 0) throw std::invalid_argument("Cannot divide by zero");
return a / b;
}
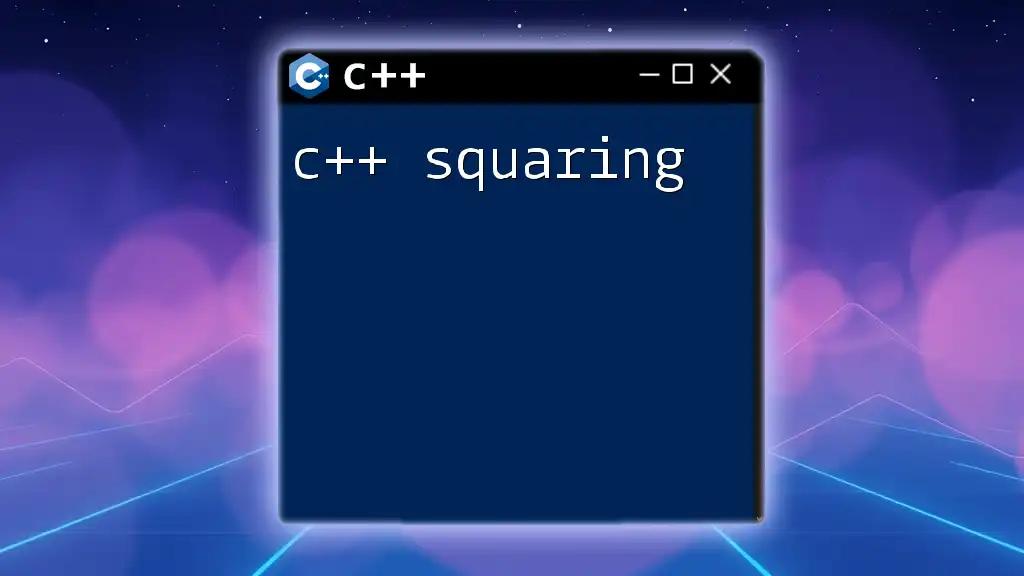
Advanced Modular Techniques in C++
Multiple Module Files
As your projects grow, organizing your code with multiple modules can be crucial. Each module can focus on a specific aspect of your application. This organization enables deep layering of functionalities, allowing for efficient teamwork.
Example: A complex project structure:
- Project
- src
- math_utils.ixx
- calculator.ixx
- app.cpp
- src
Version Control with Modules
Managing module versions is essential for maintaining consistency, especially in larger projects. It’s advisable to establish a versioning strategy that allows for backward compatibility whenever changes are made.
Error Handling in Modules
When building modular applications, error handling should be a priority. You will encounter various possible runtime errors, such as division by zero in mathematical operations.
Code Snippet: Using exceptions in modules:
int divide(int a, int b) {
if (b == 0) throw std::invalid_argument("Cannot divide by zero");
return a / b;
}

Best Practices for C++ Modular Programming
Keep Modules Focused
Each module should have a well-defined responsibility. Adhering to the single responsibility principle maximizes modular efficiency and minimizes confusion.
Tip: Identify what functionality is related and group only those functions within one module.
Documentation and Comments
Proper documentation is vital for any modular programming effort. Ensure each function, its parameters, and return values are thoroughly documented.
Example: Commenting best practices in your code:
// Adds two integers and returns the result.
int add(int a, int b) {
return a + b;
}
Testing Modular Code
Testing should be an integral part of the development process. Each module can be tested independently, ensuring that individual components perform as expected before they are integrated into larger systems.
Code Snippet: Example of a simple test case for a module:
#include <cassert>
int main() {
assert(add(3, 2) == 5);
assert(subtract(5, 3) == 2);
return 0;
}
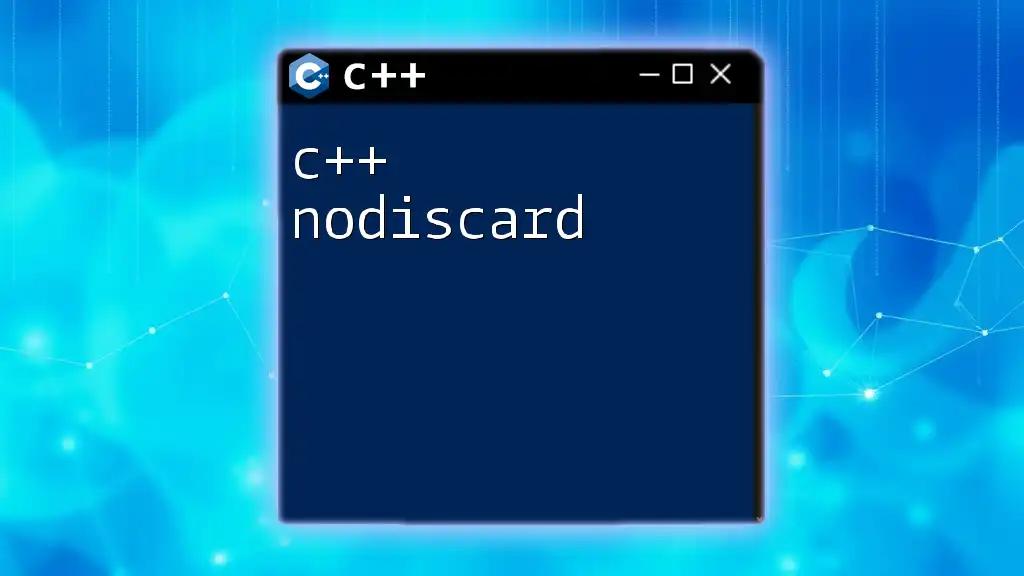
Conclusion
In conclusion, embracing C++ modular programming principles not only enhances code quality but also fosters a productive development environment. The practice of constructing and managing modules ensures that programs remain scalable, maintainable, and robust. As you embark on your programming adventures, integrating modular techniques into your projects will undoubtedly lead to a fulfilling coding experience. Start exploring and building today, and witness the transformative power of modular programming!