CMake C++ modules provide a modern way to organize and manage your C++ codebase using the `import` keyword for better modularity and easier dependency management.
# Example of a CMakeLists.txt file using C++ modules
cmake_minimum_required(VERSION 3.8)
project(MyProject)
# Enable C++20 for module support
set(CMAKE_CXX_STANDARD 20)
# Specify the module as a library
add_library(MyModule MODULE my_module.cpp)
# Create an executable that imports the module
add_executable(MyApp main.cpp)
target_link_libraries(MyApp PRIVATE MyModule)
Overview of CMake
CMake is a powerful cross-platform build system generator that simplifies the development process by automating the build configuration for C++ projects. It streamlines the building process across different environments, ensuring that your code runs consistently regardless of the platform.
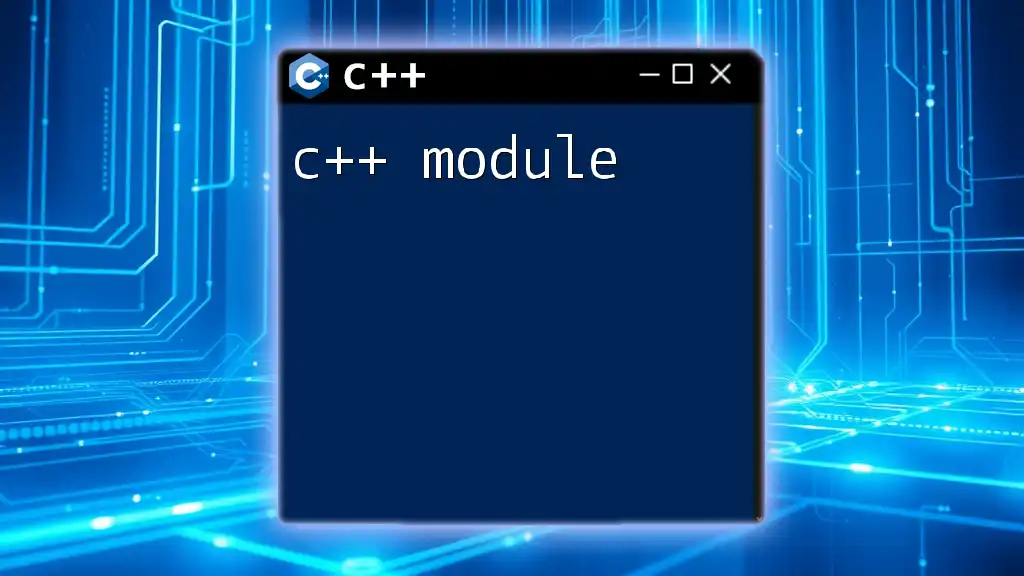
What are C++ Modules?
C++ modules are a modern feature introduced in C++20, designed to improve the way we structure and manage our code. They aim to address longtime issues related to header files, such as include guards and multiple inclusions, which can lead to longer compile times and a messier codebase. By encapsulating code, modules enable better organization and isolation, ultimately enhancing readability and maintenance.
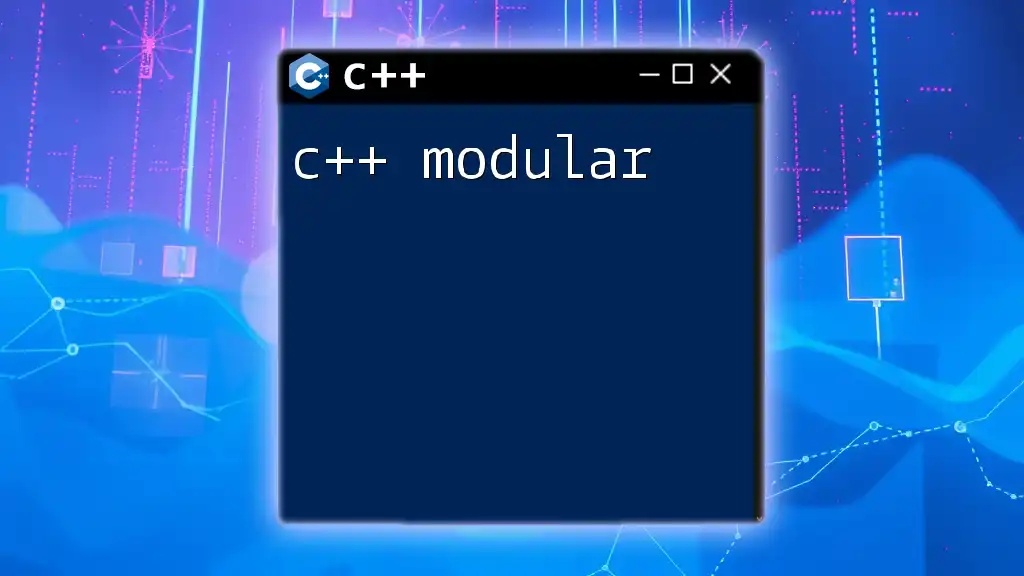
Why Use Modules with CMake?
Using C++ modules with CMake offers several advantages, including:
- Improved Compile Times: Modules allow the compiler to process modules in isolation, reducing the need for redundant operations that are typical with header files.
- Better Organization: Modules encourage a more modular approach to coding, making it easier to manage dependencies and relationships within your project.
- Reduced Name Conflicts: By encapsulating code within modules, you minimize the potential for naming collisions, which is common in large projects.
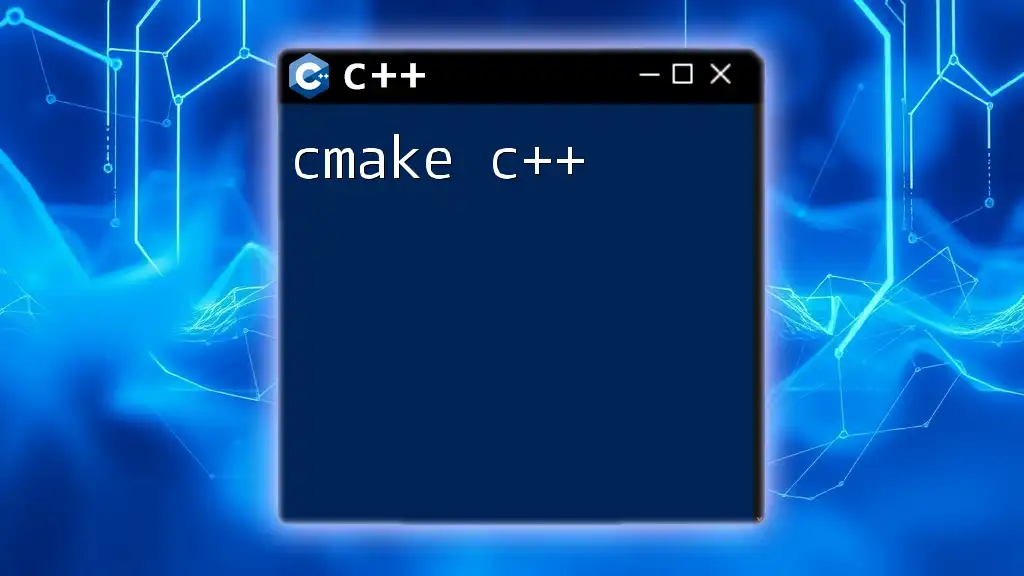
CMake Basics
CMake Syntax and Structure
CMake uses a file named `CMakeLists.txt` to define the build configuration. This file contains commands that express to CMake how to build the project, manage files, and set compilation options. A basic understanding of this syntax is key to using CMake effectively for C++ modules.
Setting Up a CMake Project
Setting up a basic CMake project involves creating the directory structure and writing your `CMakeLists.txt`. A typical setup looks like this:
MyProject/
├── CMakeLists.txt
└── src/
├── main.cpp
└── MyModule.ixx
Your `CMakeLists.txt` file for the project would look like this:
cmake_minimum_required(VERSION 3.20)
project(MyProject)
set(CMAKE_EXPORT_COMPILE_COMMANDS ON)
# Enable C++20 to use modules
set(CMAKE_CXX_STANDARD 20)
add_executable(MyExecutable src/main.cpp)
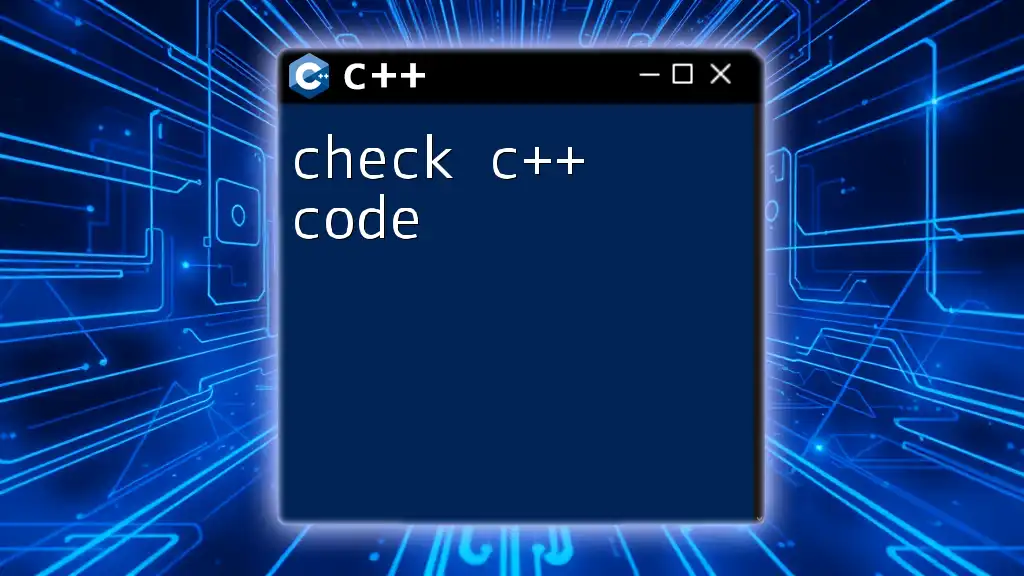
Introduction to C++ Modules
Module Basics
Defining a module involves using the `export` keyword followed by the `module` keyword, which informs the compiler about the module definition. A simple module definition might look like this:
// MyModule.ixx
export module MyModule; // Declare the module
export int add(int a, int b) {
return a + b;
}
This declaration establishes the module MyModule and exports a simple function add, making it available for other code files that import this module.
Why Modules Matter
The impact of C++ modules is profound. They significantly reduce compilation overhead by allowing the compiler to parse and cache modules independently. This not only speeds up builds but also provides a cleaner, more maintainable code structure.
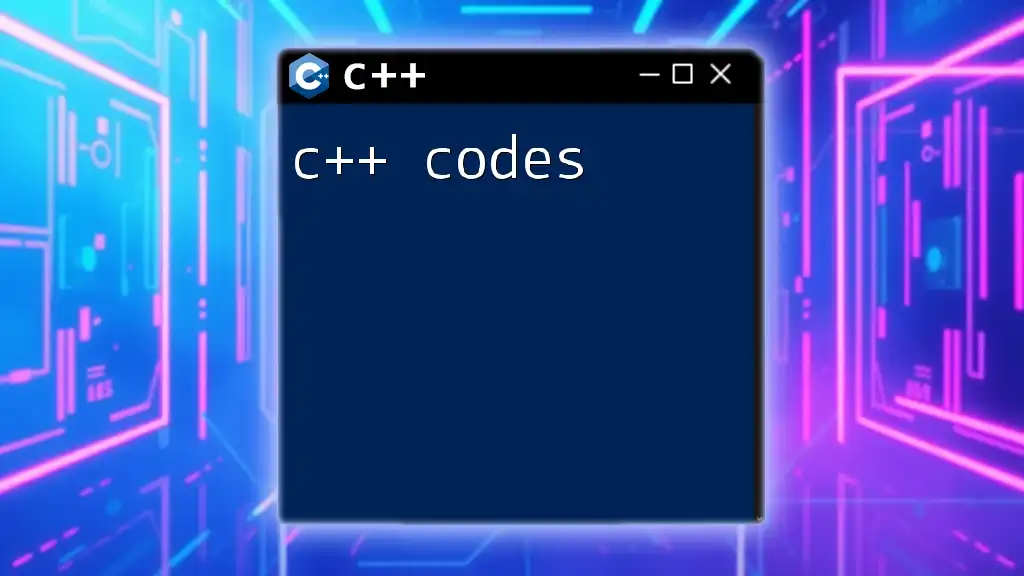
Using C++ Modules with CMake
Setting Up C++ Module Support in CMake
CMake must be configured to support C++ modules in the project. The minimum required version for module support is 3.20. You can enable modules through specific properties in your CMakeLists.txt:
cmake_minimum_required(VERSION 3.20)
project(MyProject)
set(CMAKE_EXPORT_COMPILE_COMMANDS ON)
# Enable C++20 to use modules
set(CMAKE_CXX_STANDARD 20)
Creating Modules
To create a module, you'd typically define it in a file with the `.ixx` extension, such as MyModule.ixx. Here is a simple example showcasing the module definition and its usage:
// MyModule.ixx
export module MyModule; // Declare the module
export int add(int a, int b) {
return a + b;
}
Importing and Using Modules
To import modules in your CMake project, you use the `import` statement in your C++ files. Here's how to set up and call module functions:
- CMakeLists.txt for Executable
add_executable(MyExecutable src/main.cpp)
set_target_properties(MyExecutable PROPERTIES
CXX_STANDARD 20)
- Utilizing the Module in Your Code
// main.cpp
import MyModule;
int main() {
int sum = add(5, 10);
std::cout << "Sum: " << sum << std::endl;
return 0;
}
In this case, the executable MyExecutable uses the function add from the MyModule module to calculate and print the sum.
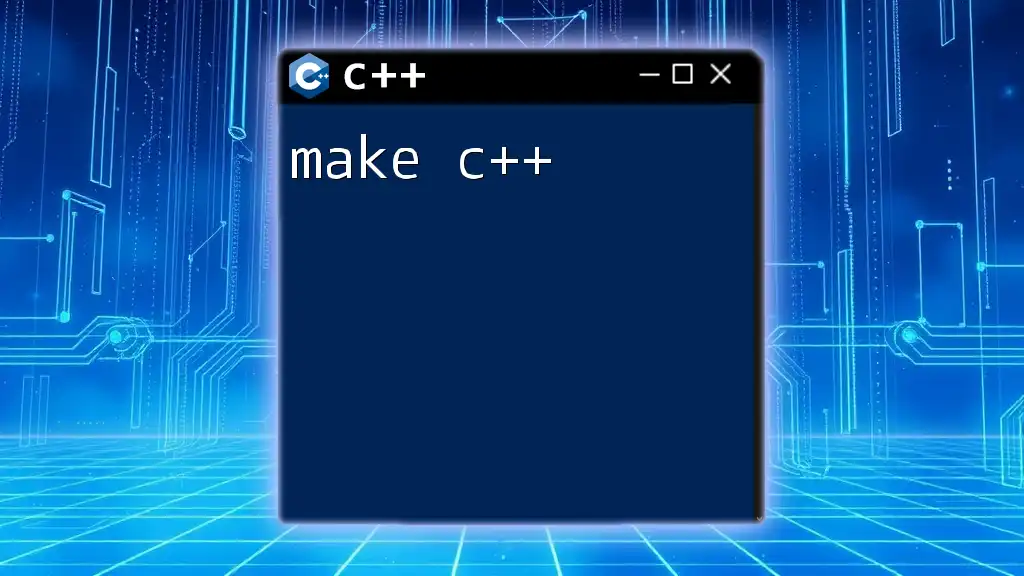
Advanced Module Concepts
Module Partitioning
Module partitioning allows you to divide a module into multiple parts, which can help with both compile times and code organization. A simple example of partitioning might look like this:
// MyModule.ixx
export module MyModule; // Main module definition
import :math;
By employing partitions, you can separate implementation details while still maintaining the modularity of your code.
Using Module Interfaces
Understanding interfaces in modules is essential, as they allow you to expose only specific parts of your module while keeping other elements hidden. This enhances encapsulation and reduces unintended dependencies.
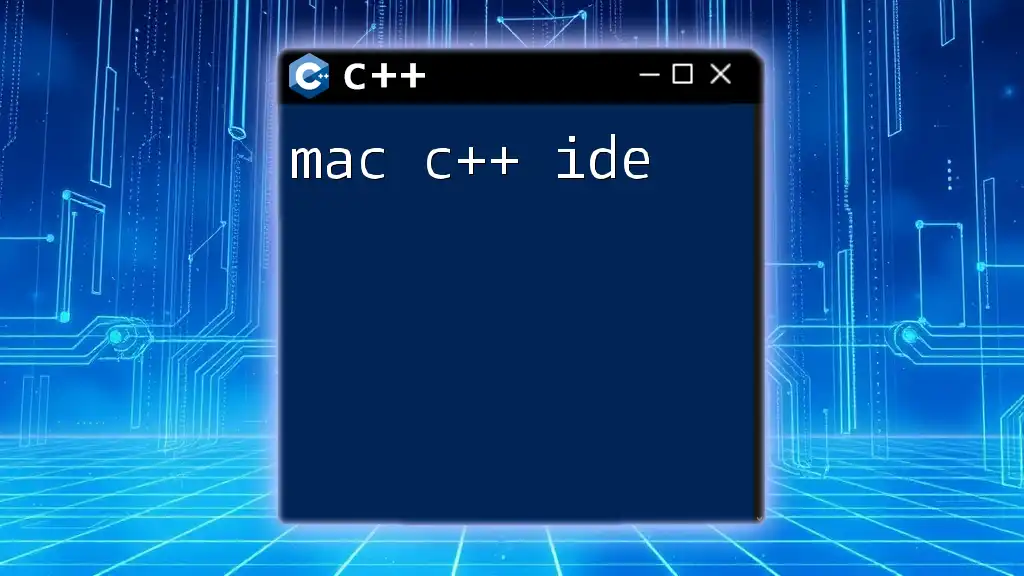
Best Practices for CMake C++ Modules
Organizing Your Code
To maintain clarity and organization in a CMake project with modules:
- Keep your module files in a separate directory (e.g., `src/modules/`).
- Use clear and concise naming conventions for modules to reflect their purpose.
Debugging Modules
Debugging modules can be tricky at times. Common issues include:
- Missing Imports: Ensure all required modules are correctly imported.
- Circular Dependencies: Avoid circular references by carefully managing module dependencies.
- Compatibility: Ensure that you are using a compatible CMake version that supports C++ modules seamlessly.
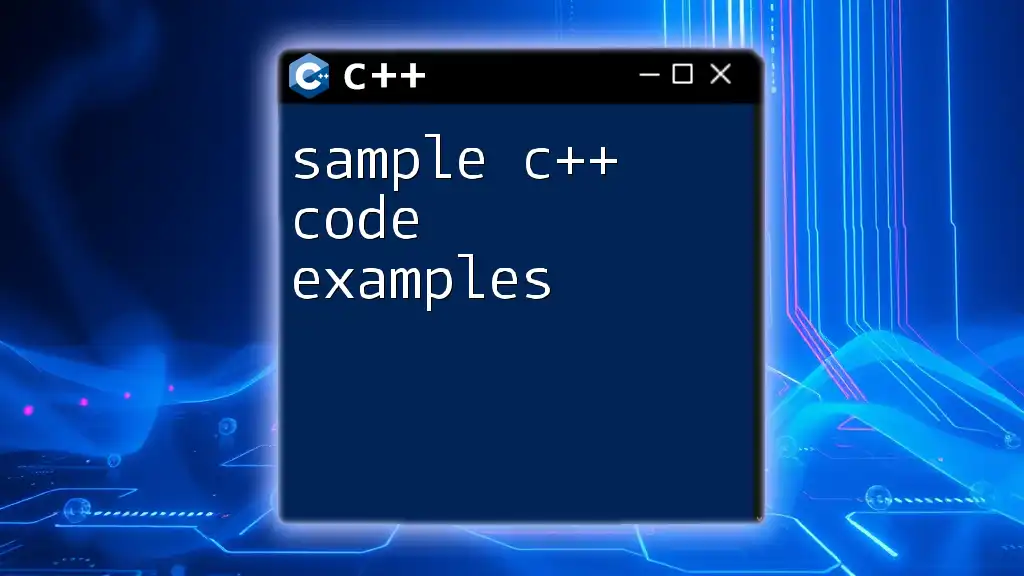
Conclusion
The ability to effectively utilize CMake C++ modules represents a significant advancement in modern C++ programming. By adopting modules, developers can enhance code organization, eliminate redundancy, and ultimately improve compile times. As CMake and C++ continue to evolve, staying informed will be crucial for leveraging the best practices in your projects.
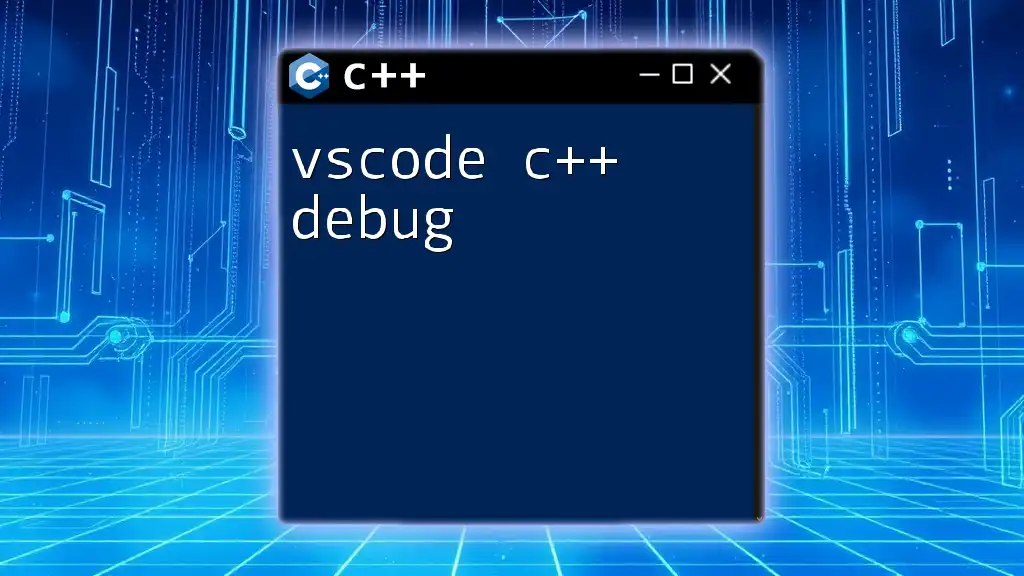
Additional Resources
For further exploration of C++ modules and CMake, consider the following:
- Official CMake Documentation: A comprehensive resource for all CMake features.
- Books and Tutorials: Aim for those focusing on modern C++ and CMake practices.
- Community Forums: Engage with fellow developers for advice, support, and collaborative problem-solving.