In C++, the modulus operator (`%`), commonly referred to as "mod," is used to find the remainder of the division of two integers.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a % b; // result will be 1, as 10 divided by 3 gives a remainder of 1
std::cout << "The remainder of " << a << " divided by " << b << " is " << result << std::endl;
return 0;
}
What is the Modulus Operator in C++
Understanding the Modulus Operator
The modulus operator, often referred to as the mod operator, is a mathematical operator that yields the remainder of a division operation. It plays a crucial role in various programming functionalities, especially when performing calculations that involve cyclic behavior, conditions, or even certain algorithms. In C++, the symbol for the modulus operator is the percent sign (`%`).
In programming, the use of the modulus operator can be essential for tasks such as determining even or odd numbers, cycling through arrays, and implementing more complex algorithms like those in cryptography.
Basic Syntax of the Modulus Operator
The basic syntax for using the modulus operator in C++ follows this structure:
result = a % b;
In this snippet:
- `a` and `b` are the operands.
- The `result` variable will store the remainder of the division of `a` by `b`.
- The return type of the operation will depend on the data types of the operands (typically `int`).
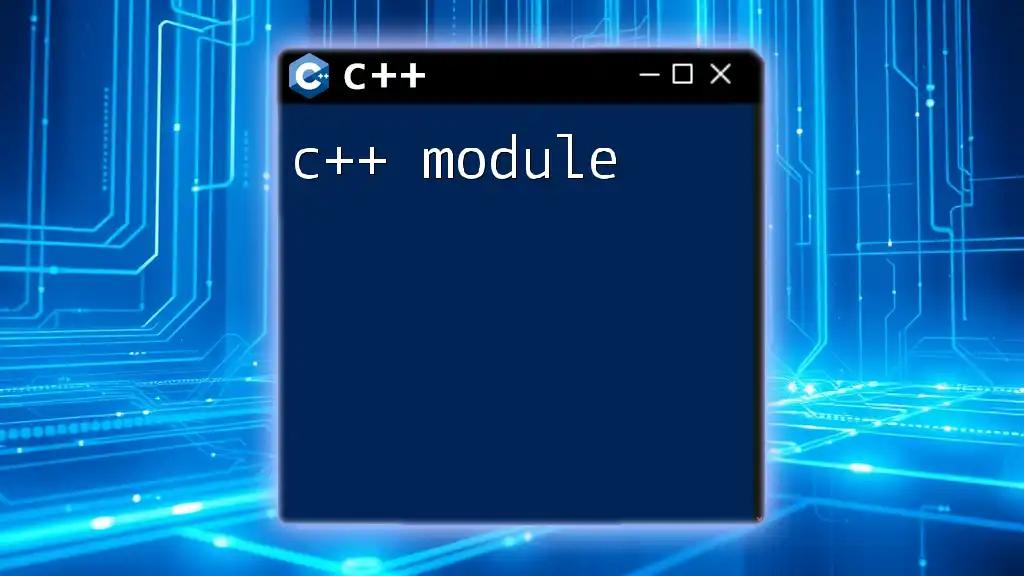
How Does the Modulus Operator Work?
Fundamental Concept of Modulus
The modulus operation can be understood better through the lens of mathematics. For instance, when you divide 10 by 3, you get a quotient of 3 (which is 3 times 3) and a remainder of 1. Thus, `10 % 3` equals 1.
This concept becomes clearer when visualized. The operation essentially divides the first operand by the second, ignoring the quotient and retaining the remainder.
Working with Positive Integers
When utilizing the modulus operator with positive integers, the behavior is straightforward. Here’s an illustration:
int a = 10;
int b = 3;
int result = a % b; // result is 1
In this case, `10 divided by 3 equals 3` (with a remainder of 1).
You can further explore multiple scenarios involving positive integers:
int a = 14;
int b = 4;
int result1 = a % b; // result1 is 2
Here `14 % 4` returns 2, reflecting the remainder from this division.
Working with Negative Integers
When the modulus operator interacts with negative integers, the outcome can be slightly counterintuitive. With negative numbers, C++ yields a result that retains the sign of the left operand (the dividend).
int a = -10;
int b = 3;
int result = a % b; // result is -1
In this example, `-10 % 3` gives -1 because the division of -10 by 3 produces -4 with a remainder of -1, emphasizing how C++ maintains the sign of the dividend.
Modulus with Zero
A critical aspect to remember is what happens when the modulus operator involves zero. In mathematics, division by zero is undefined and throws an error. For instance:
int a = 10;
int b = 0;
// Attempting 'a % b' here will lead to a division by zero error.
If you ever attempt to use zero as the divisor, it results in a runtime error, which can crash your program. It's a good practice to always check if `b` is zero before performing a modulus operation.

Practical Applications of the Modulus Operator
Using Modulus in Loops
The modulus operator excels in controlling iterations within loops, particularly to execute specific tasks at regular intervals. For example, when printing even numbers within a loop, you could implement it as follows:
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
std::cout << i << " is even" << std::endl;
}
}
In this code, the `if (i % 2 == 0)` condition checks if `i` is even. If true, it prints the value.
Modulus in Conditions
The modulus operator can also facilitate conditional checks effectively. For instance, you could determine if a number is divisible by another:
int num = 15;
if (num % 5 == 0) {
std::cout << "The number is divisible by 5." << std::endl;
}
In this case, `15 % 5` returns 0, confirming that 15 is divisible by 5.
Examples in Real-World Applications
The modulus operator is frequently used in applications such as:
- Leap Year Calculation: To determine if a year is a leap year, check if it is divisible by 4.
- Clock Arithmetic: In a 12-hour clock system, you can use modulus to loop through hours without exceeding 12.

Common Pitfalls and Best Practices
Common Mistakes with Modulus
One prevalent misunderstanding with the modulus operator pertains to its behavior involving negative integers. Programmers often expect a positive remainder, but as discussed, C++ returns a result that reflects the sign of the dividend. Another common issue is division by zero, which should always be checked before performing any modulus operation.
Best Practices for Using Modulus
To use the modulus operator effectively, consider:
- Always Validate Input: Make sure that the divisor is not zero to avoid runtime errors.
- Use Clear Logic in Conditions: When applying modulus in conditions, always state your intentions clearly within the conditionals to maintain code readability.
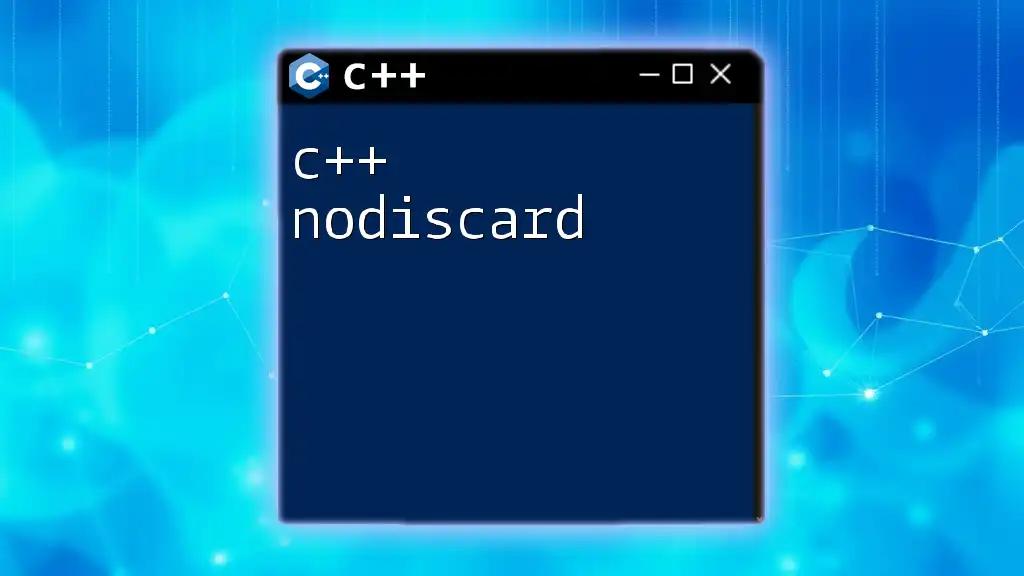
Advanced Modulus Applications
Modulus in Cryptography
In the field of cryptography, the modulus operator is utilized for many algorithms, especially in hash functions. It often helps in keeping values within a certain range, thus allowing secure data transformation.
Modulus in Game Development
The modulus operator finds substantial utility in game development, particularly in scenarios involving character movement or level cycling. Here’s how it can loop within bounds effectively:
int player_position = 10;
int max_position = 4;
player_position = (player_position + 1) % max_position; // Loops within bounds
This example allows the player's position to cycle through available positions effectively, resetting whenever it exceeds the maximum defined.
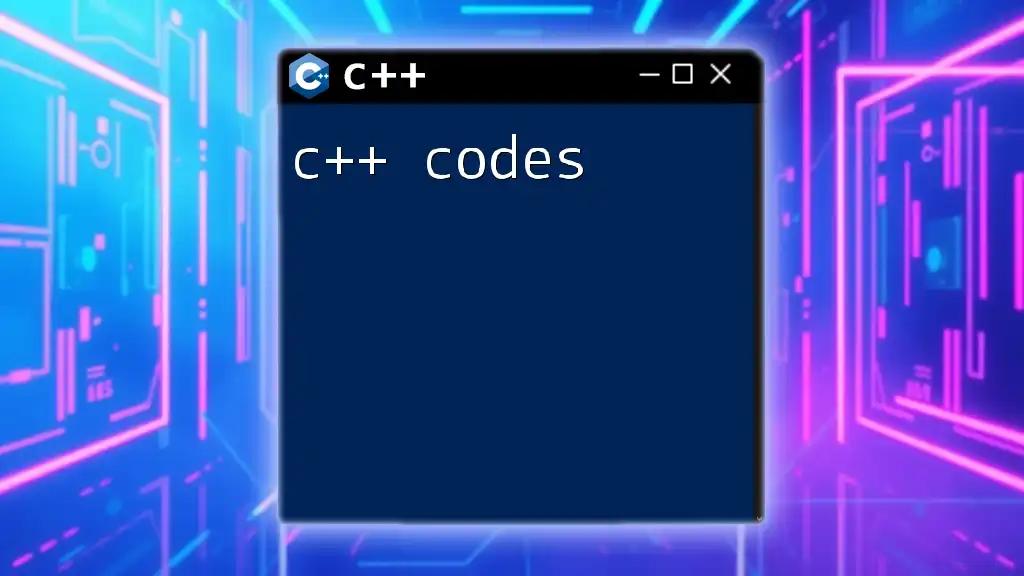
Summary of the Modulus Operator in C++
The modulus operator (`%`) is an essential tool in C++ programming that serves multiple purposes, from simple calculations to complex control flows and applications in game development and cryptography. Understanding how it operates with both positive and negative integers, as well as its pragmatic uses, can significantly enhance your programming efficiency and accuracy.

Additional Resources
For those looking to delve deeper into the intricacies of the modulus operator and C++ as a whole, consider exploring recommended books, online tutorials, and documented resources that cover fundamental to advanced programming concepts. Links to official C++ documentation can provide further insights and examples on its usage.