The `fmod` function in C++ computes the remainder of the division of two floating-point numbers, similar to the modulus operator for integers.
Here’s a simple example:
#include <iostream>
#include <cmath>
int main() {
double dividend = 5.3;
double divisor = 2.0;
double result = fmod(dividend, divisor);
std::cout << "The remainder of " << dividend << " divided by " << divisor << " is " << result << std::endl;
return 0;
}
What is fmod?
fmod (Floating-point modulus) is a function in C++ that plays a crucial role in mathematical computations involving floating-point numbers. It returns the remainder of the division of two floating-point numbers, which can be extremely useful in various programming scenarios.
Mathematical Background
To understand fmod, it’s essential to grasp the concept of the modulus operation. Mathematically, the modulus operation finds the remainder of a division. For instance, when you divide 10 by 3, the quotient is 3, and the remainder is 1. This example elucidates how fmod works, but it is specifically optimized for floating-point numbers.
Key distinction: fmod differs from the percent (%) operator, primarily used with integers. The percent operator throws out the decimal value, while fmod retains it, making it ideal for working with floating points.
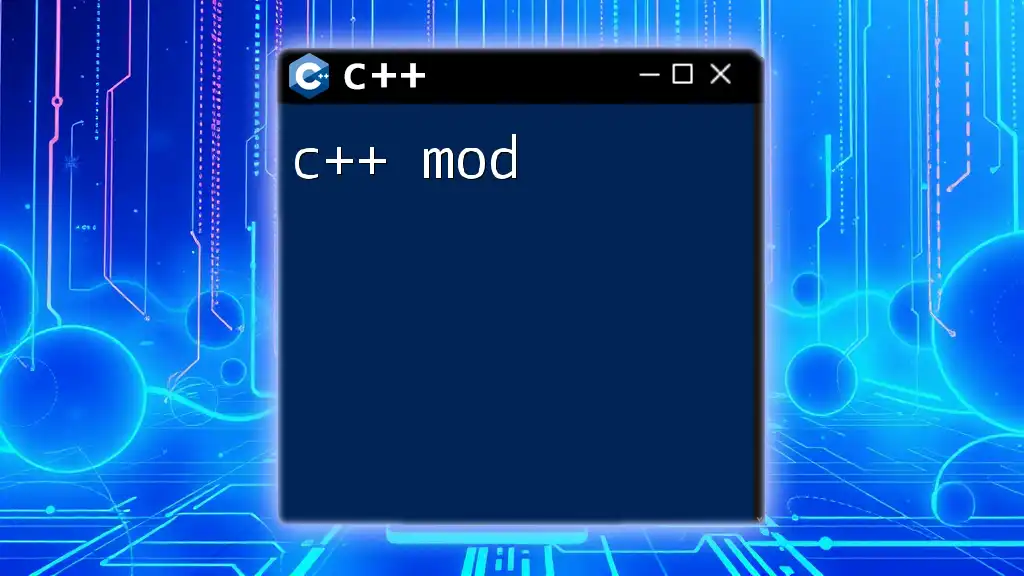
Why Use fmod in C++?
Use Cases for fmod
The fmod function serves several purposes in programming:
-
Game Development: In game design, you might need to loop animations or manage object positions within a screen's dimensions. By using fmod, you can determine an object's position on a loop and reset it once it exceeds certain limits.
-
Financial Calculations: Financial applications often require precise calculations with periodic payments or interests. fmod can help calculate interest that cycles within defined periods, ensuring accurate results.
Advantages of Using fmod
Utilizing fmod comes with multiple benefits:
-
Precision with Floating-point Operations: Unlike integer division, fmod retains the significance of decimal points, contributing to accuracy.
-
Handling Negative Values: fmod conveniently handles negative dividends and divisors, essential for robust computational accuracy.
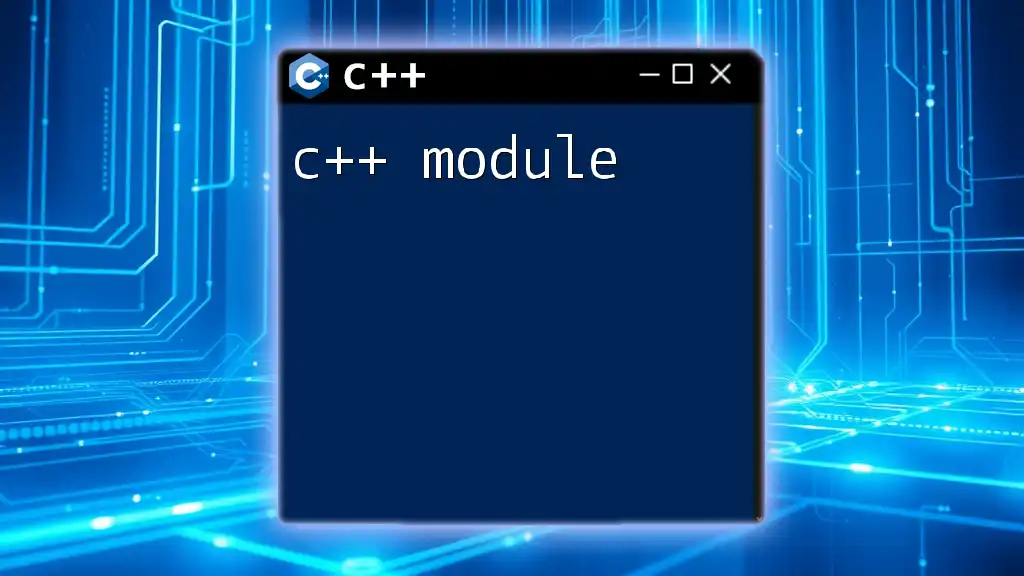
Understanding the Syntax of fmod
To utilize fmod in your code, it is crucial to understand its syntax.
double fmod(double x, double y);
- Parameters Explanation:
- `x`: This is the dividend or numerator.
- `y`: This is the divisor or denominator.
Return Value
The fmod function returns the remainder of the division. If `y` is zero, fmod's behavior is undefined, meaning it's important to handle this case in your code effectively.
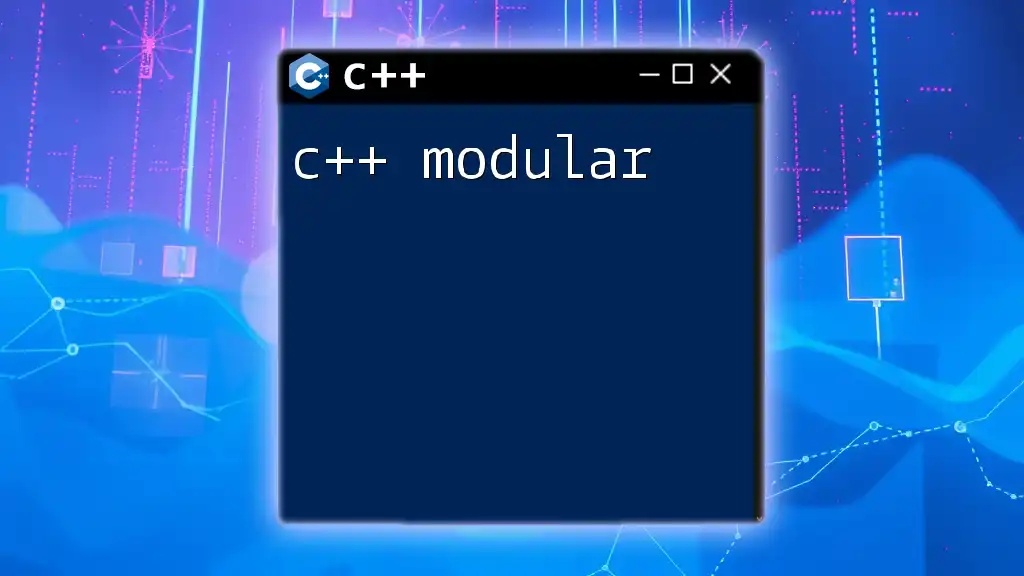
How to Include fmod in Your C++ Program
To leverage fmod in your C++ program, you’ll first need to include the appropriate header file:
#include <cmath>
Sample Code
Here’s a simple example demonstrating the usage of fmod:
#include <iostream>
#include <cmath>
int main() {
double result = fmod(5.4, 2.0);
std::cout << "Result of fmod(5.4, 2.0): " << result << std::endl;
return 0;
}
In this program, `fmod` computes the result of 5.4 divided by 2.0. Hence, the output would be 1.4, which confirms that the fmod function effectively retains the decimal part.
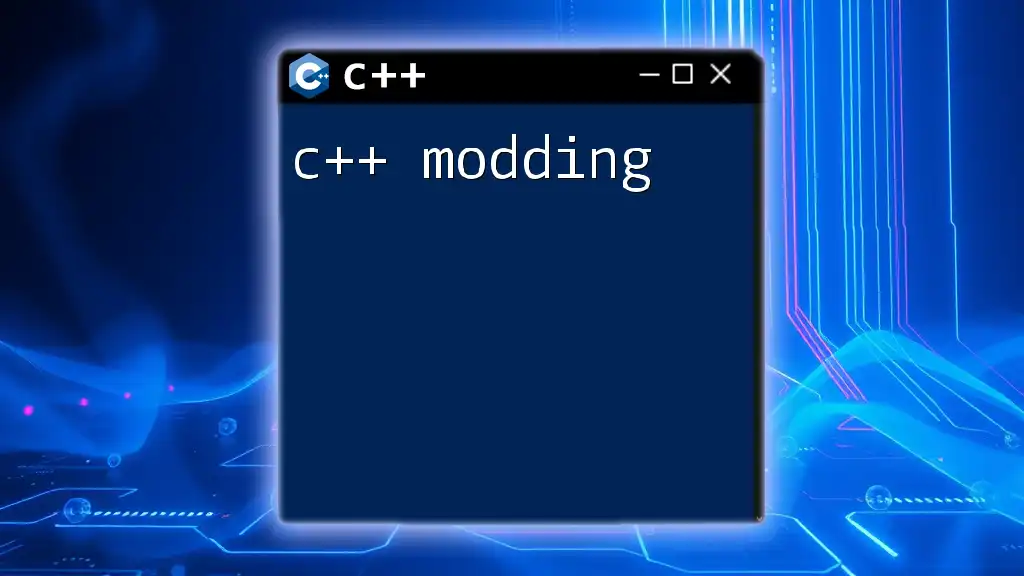
Detailed Examples of fmod in Action
Basic Calculations
In its simplest form, fmod can be used for straightforward calculations. Consider the following example:
double a = 10.5;
double b = 3.0;
std::cout << fmod(a, b); // Output: 1.5
Here, the output of fmod(10.5, 3.0) is 1.5, illustrating its efficacy with positive numbers.
Handling Negative Values
An exciting aspect of fmod is its behavior with negative values. For instance:
double c = -10.5;
double d = 3.0;
std::cout << fmod(c, d); // Output: -1.5
In this case, fmod outputs -1.5 when the dividend is negative. Understanding this behavior is critical when making calculations involving both positive and negative numbers.
Zero Divisor Behavior
Another important consideration is the behavior of fmod when the divisor is zero. The following code attempts to demonstrate this edge case:
std::cout << fmod(10.0, 0.0); // Undefined behavior
Attempting to compute the modulus with zero as the divisor results in undefined behavior. Thus, it’s imperative to implement error handling to avoid this in real-world applications.
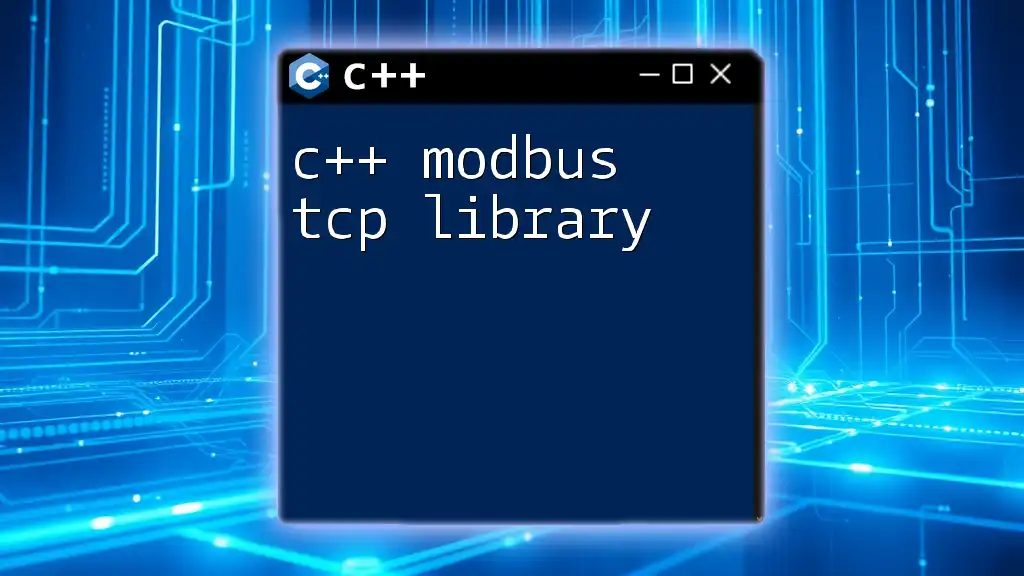
Common Mistakes Using fmod
Mistakes often arise with fmod due to a misunderstanding of the modulus operation with negative values. Many may assume that the result should be positive; however, that is not always the case.
Another common error is improper handling of floating-point precision. Floating-point numbers have limitations due to their representation in computers, and failing to account for this can lead to unexpected results when using fmod or performing comparisons.
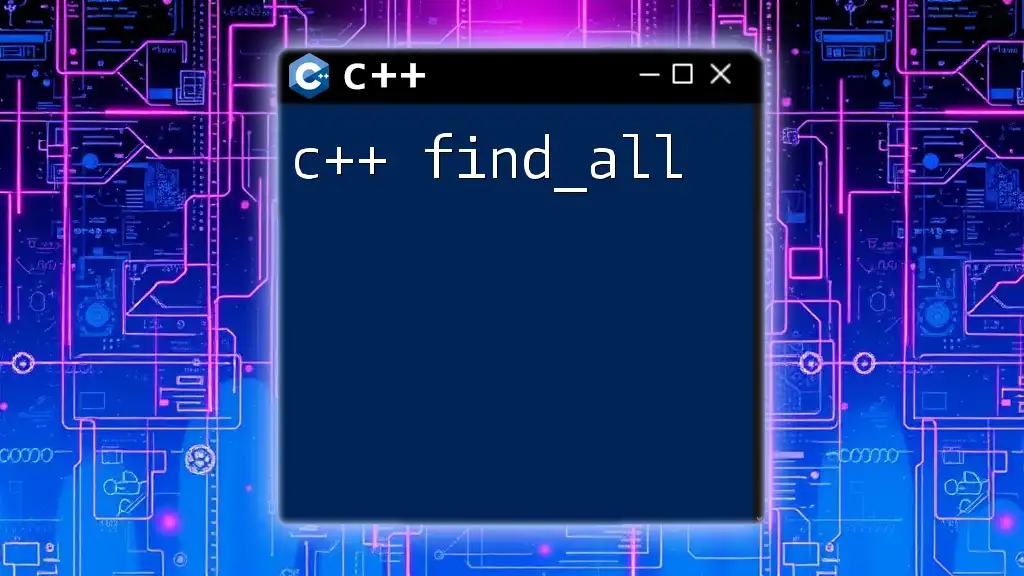
Best Practices for Using fmod
To maximize efficiency and accuracy while using fmod, consider the following best practices:
-
Avoid Common Pitfalls: Always ensure that your divisor is not zero to prevent undefined behavior. Implement checks or assertions where necessary.
-
Review Alternatives: Although fmod has its place, it’s beneficial to be aware of other mathematical approaches available for your specific scenarios, such as using integer modulus when dealing strictly with whole numbers.
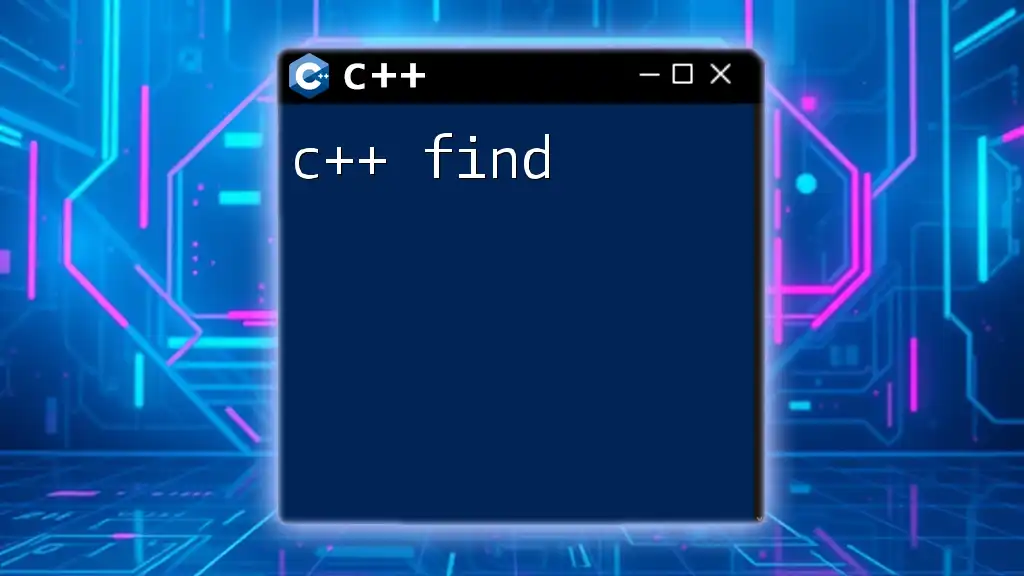
Conclusion
In summary, understanding the C++ fmod function equips you with the tools for precise mathematical operations involving floating-point numbers. Its applications stretch from game development to financial calculations, making it an invaluable asset in software design. As you explore fmod in your projects, remember to experiment with different scenarios and applications to fully grasp its capabilities.
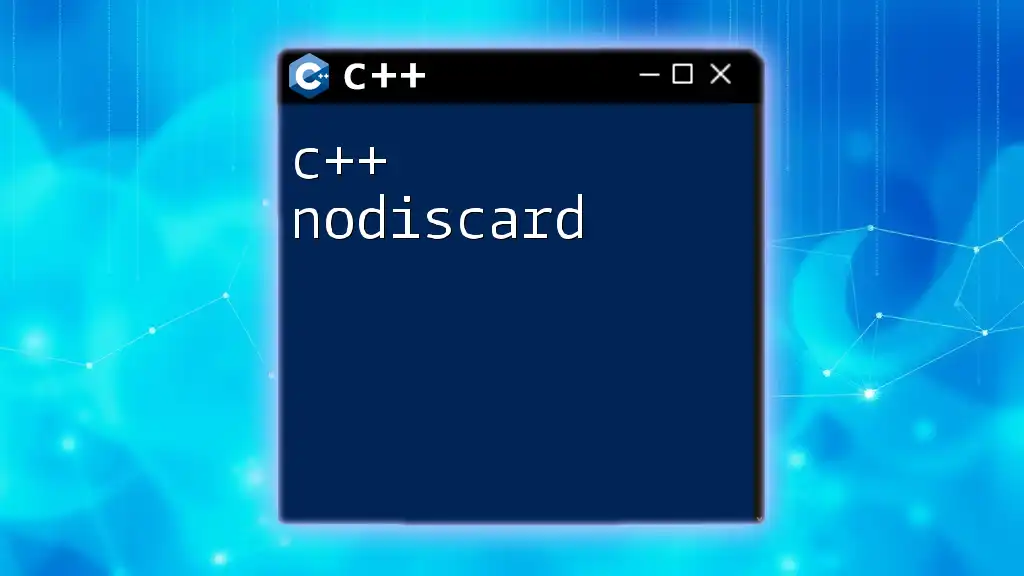
Further Resources
To deepen your understanding of fmod and its applications, consider exploring the official C++ documentation and various online programming resources. Tutorials and programming books can also provide additional context and examples to reinforce your learning.
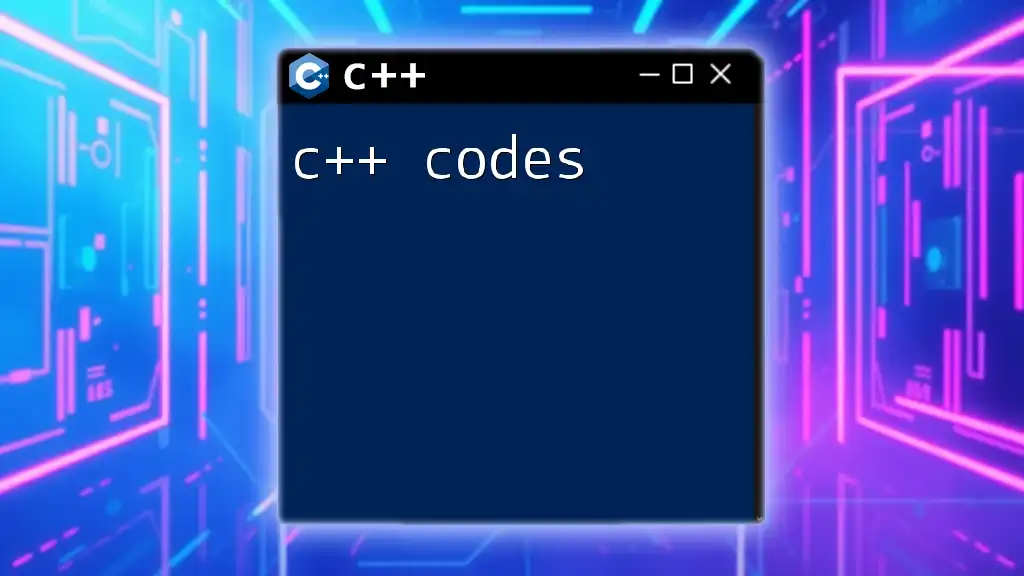
References
- C++ Standard Library Documentation
- Programming Books covering Advanced C++ Concepts
- Online Tutorials focusing on Math Functions in C++