In C++, the `floor` and `ceil` functions are used to round a floating-point number down or up to the nearest integer, respectively. Here's a code snippet illustrating both functions:
#include <iostream>
#include <cmath>
int main() {
double num = 5.7;
std::cout << "Floor: " << std::floor(num) << std::endl; // Outputs: 5
std::cout << "Ceil: " << std::ceil(num) << std::endl; // Outputs: 6
return 0;
}
Understanding the Basics of Floor and Ceiling Functions
What is the Floor Function?
The floor function is a mathematical operation that rounds a floating-point number down to the nearest whole number. It is denoted mathematically as \( \text{floor}(x) \). For instance, if you have a number like 5.7, applying the floor function will yield 5.
What is the Ceiling Function?
In contrast, the ceiling function rounds a number up to the nearest whole number. Its mathematical representation is \( \text{ceil}(x) \). For example, applying the ceiling function to 5.2 will give you 6.
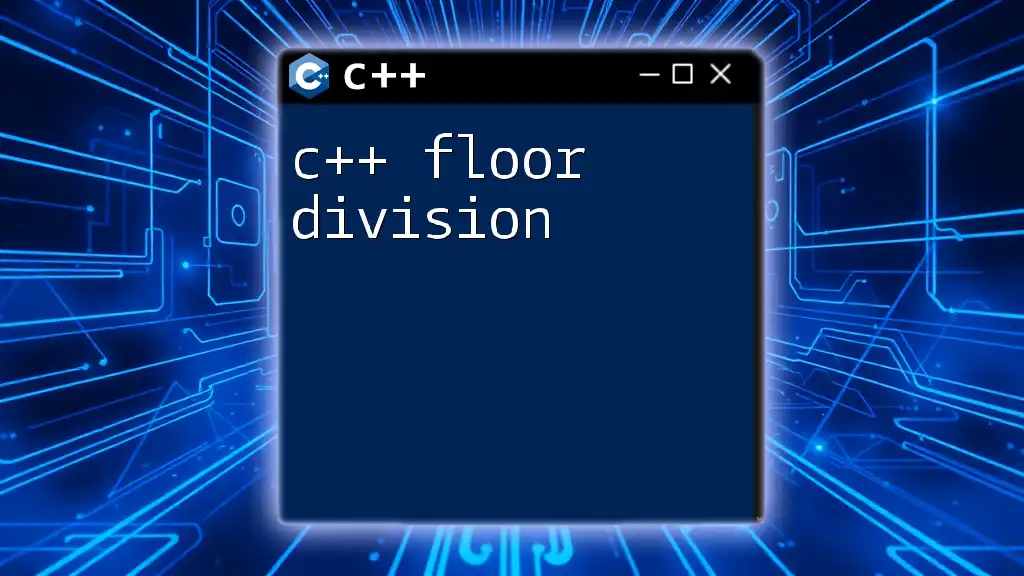
How to Use Floor and Ceiling Functions in C++
Including the Required Header
To utilize the floor and ceiling functions in C++, you need to include the `<cmath>` header file, which provides access to various mathematical functions.
#include <cmath>
Syntax of Floor and Ceiling Functions
Floor Function Syntax
The syntax for the floor function is straightforward:
float floor(float x);
This function takes a single floating-point argument and returns the largest integer value less than or equal to `x`.
Ceiling Function Syntax
Similarly, the syntax for the ceiling function is:
float ceil(float x);
This function also takes a floating-point argument and returns the smallest integer value greater than or equal to `x`.
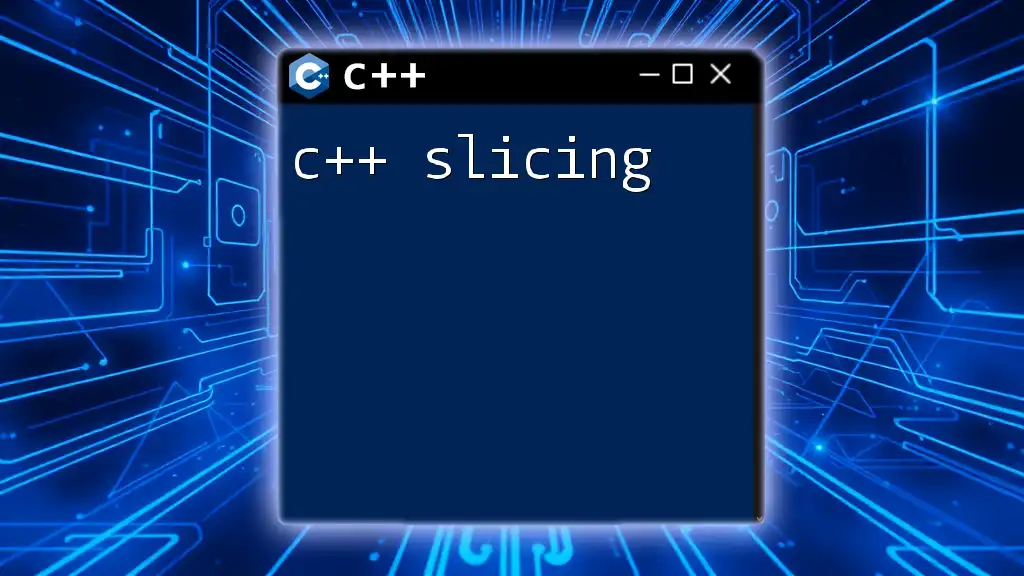
Practical Examples
Example of the Floor Function
Let's explore a practical example of the floor function.
#include <iostream>
#include <cmath>
int main() {
double value = 5.7;
double result = floor(value);
std::cout << "The floor of " << value << " is " << result << std::endl;
return 0;
}
In this code, we declare a `double` variable named `value`, assign it 5.7, and use the `floor` function to round it down. The output will be:
The floor of 5.7 is 5
This demonstrates how the floor function effectively rounds down the given floating-point number to the nearest whole number.
Example of the Ceiling Function
Now let's look at an example using the ceiling function.
#include <iostream>
#include <cmath>
int main() {
double value = 5.2;
double result = ceil(value);
std::cout << "The ceiling of " << value << " is " << result << std::endl;
return 0;
}
Here, we declare a `double` variable named `value`, assign it 5.2, and then apply the `ceil` function to round it up. The expected output will be:
The ceiling of 5.2 is 6
This example illustrates the ceiling function's ability to round a floating-point number up to the nearest integer.
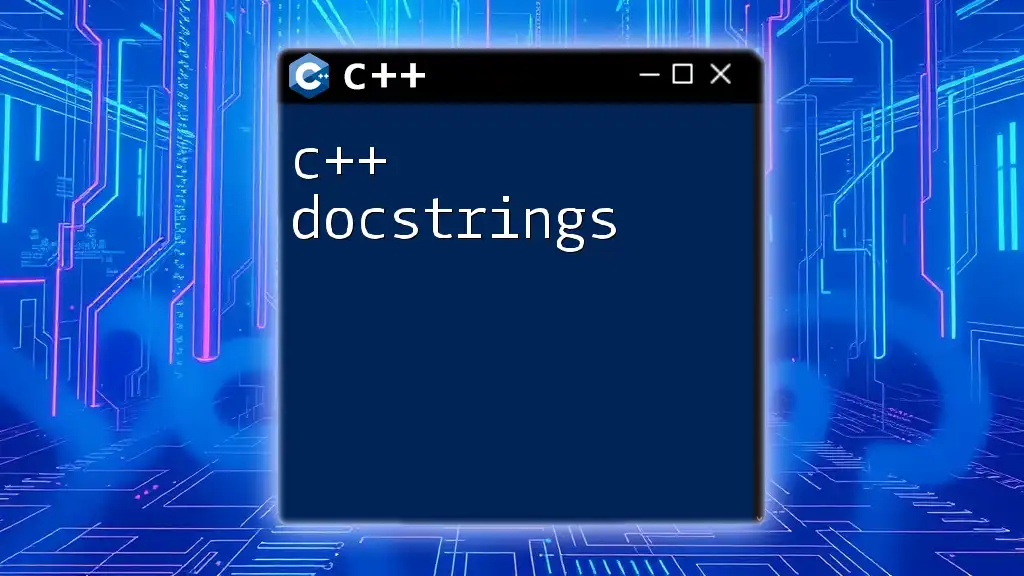
Differences Between Floor and Ceiling Functions
Key Differences
While both functions serve a similar purpose in rounding numbers, they exhibit opposite behaviors. The floor function always rounds down, while the ceiling function always rounds up, regardless of whether the decimal part is small or large.
For instance:
- For 3.4:
- Floor: 3
- Ceiling: 4
- For -2.7:
- Floor: -3
- Ceiling: -2
These differences become crucial in programming scenarios, particularly when different rounding strategies are required.
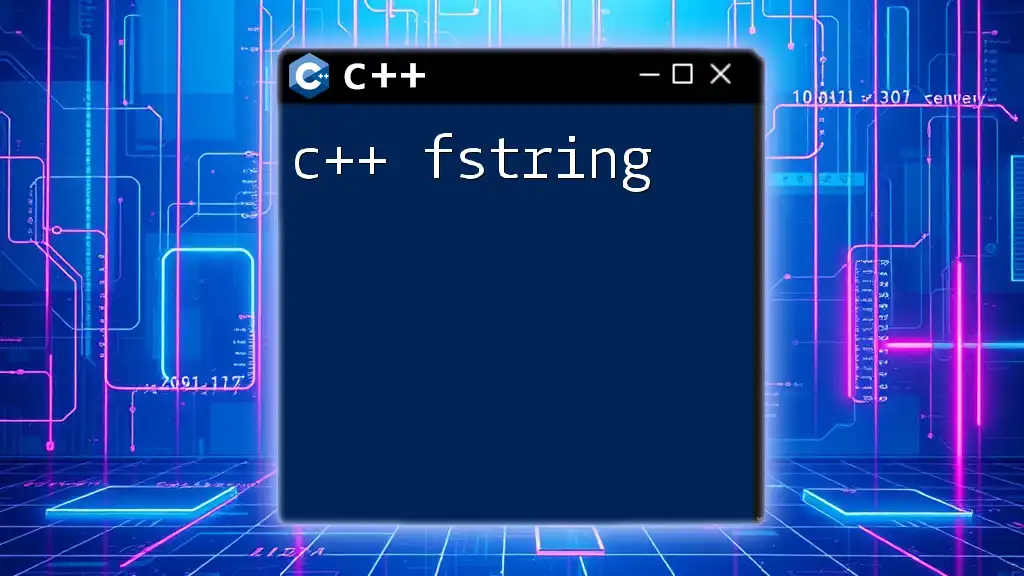
Common Use Cases
When to Use the Floor Function
The floor function is particularly useful in scenarios that demand truncating decimal values. For instance, you might want to calculate the number of whole items a customer can purchase without exceeding a budget, or in financial applications where only whole amounts matter (like calculating the number of batches of items that can be produced).
When to Use the Ceiling Function
On the other hand, the ceiling function is ideal for situations where you need to ensure that a certain quantity is fully covered. For example, if a classroom needs to accommodate a group of students and you want to allocate one room per every ten students, using the ceiling function ensures that if there are 25 students, you will allocate three rooms.
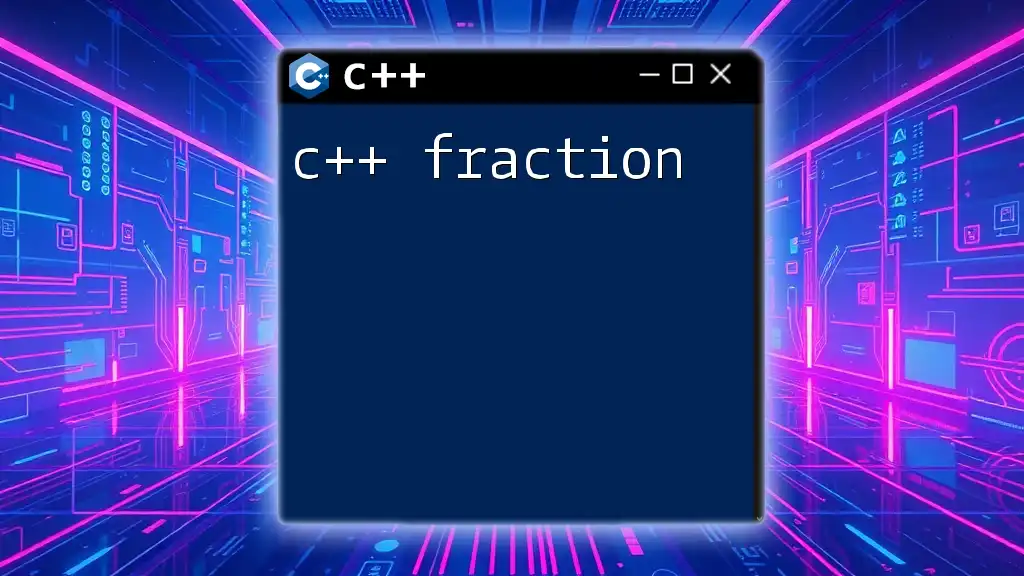
Performance Considerations
Efficiency of Floor and Ceiling Functions
The performance of the floor and ceiling functions in C++ is generally efficient. Since these functions are implemented in the standard math library, they are optimized for speed and reliability.
Potential Pitfalls
Despite their efficiency, developers should be cautious. Floating-point arithmetic can introduce precision issues. For instance, a small error during computation may lead the result to differ slightly from the expected number, especially in complex calculations. It’s essential to test your code comprehensively to avoid unexpected behavior.
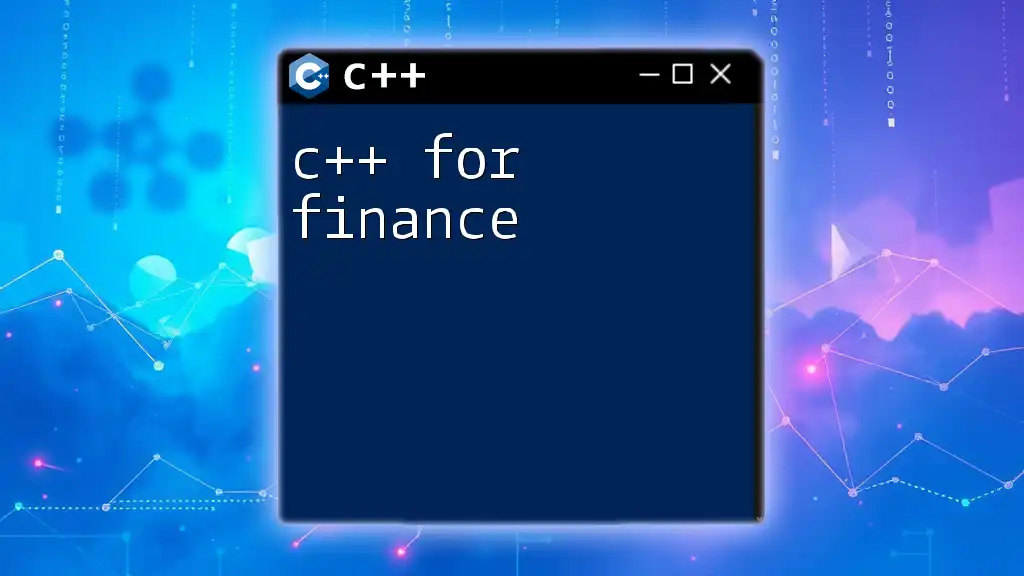
Conclusion
In summary, the C++ floor ceiling functions offer straightforward solutions for rounding floating-point numbers. Understanding how and when to utilize these functions can greatly enhance your programming skills and ensure you handle numerical data accurately. Practicing with the provided examples and exploring real-world applications will further solidify your grasp of these essential mathematical operations.
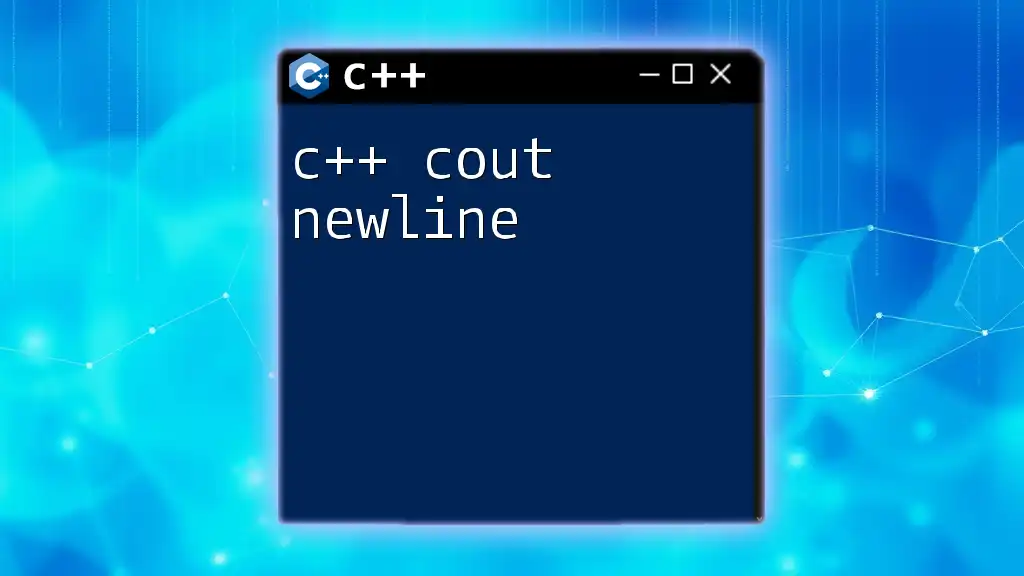
FAQ Section
Common Questions
-
What types of data do floor and ceiling functions accept?
They accept floating-point numbers as input. -
How do these functions behave with negative numbers?
The floor function will round down (toward negative infinity), while the ceiling function rounds up (toward zero). -
Are there any differences between `floor()` and `ceil()` in different compilers?
Generally, they should behave consistently across all standard-compliant C++ compilers, though platform-specific variations might exist in handling edge cases.
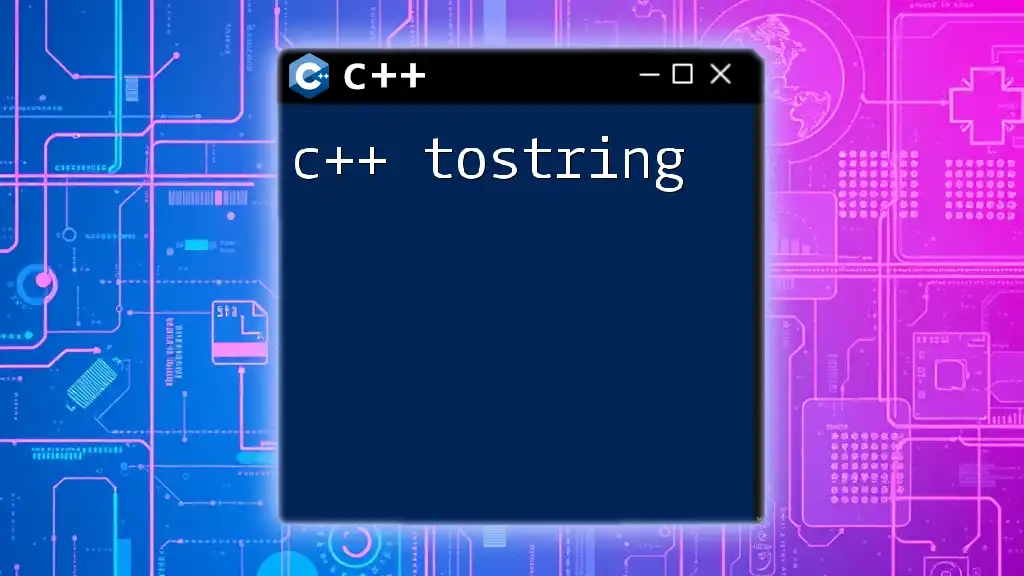
Further Reading
To deepen your understanding and skills with C++ mathematical functions, consider exploring other functions in the `<cmath>` library and additional resources such as tutorials, textbooks, and online programming communities. These will provide further guidance and examples to solidify your knowledge.