C++ for finance leverages its powerful performance and efficiency to handle complex calculations and data analyses that are essential for financial modeling and algorithmic trading.
Here's a simple example of using C++ to calculate the future value of an investment:
#include <iostream>
#include <cmath>
double futureValue(double principal, double rate, int time) {
return principal * pow(1 + rate, time);
}
int main() {
double principal = 1000.0; // Initial investment
double rate = 0.05; // 5% annual interest rate
int time = 10; // Investment duration in years
std::cout << "Future value: " << futureValue(principal, rate, time) << std::endl;
return 0;
}
Understanding C++ Basics for Financial Applications
Key Features of C++
C++ is a powerful programming language that integrates both procedural and object-oriented programming paradigms. Understanding its object-oriented programming (OOP) capabilities is essential for anyone looking to apply C++ in finance.
In financial applications, OOP facilitates the structuring of complex systems through:
- Classes and Objects: Creating representations of financial instruments such as stocks and bonds.
- Inheritance: Enabling you to extend existing classes to build specialized financial models.
- Polymorphism: Allowing for multiple forms of functions or classes, which is useful when dealing with various financial products that share similar characteristics.
C++ Syntax and Structure
A solid grasp of C++ syntax and structure is fundamental for effective programming.
Variables and Data Types In finance, dealing with numbers and calculations is crucial. C++ provides various data types, with double being commonly used for currency representation due to its precision. For instance, to store the price of a stock:
double stockPrice = 150.25; // Example stock price
Control Structures Control structures such as conditional statements and loops are vital for executing complex algorithms. Consider calculating the simple interest for different principal amounts:
double calculateInterest(double principal, double rate, int time) {
return (principal * rate * time) / 100;
}
This function can be integrated into a larger financial application to assess different investment scenarios.
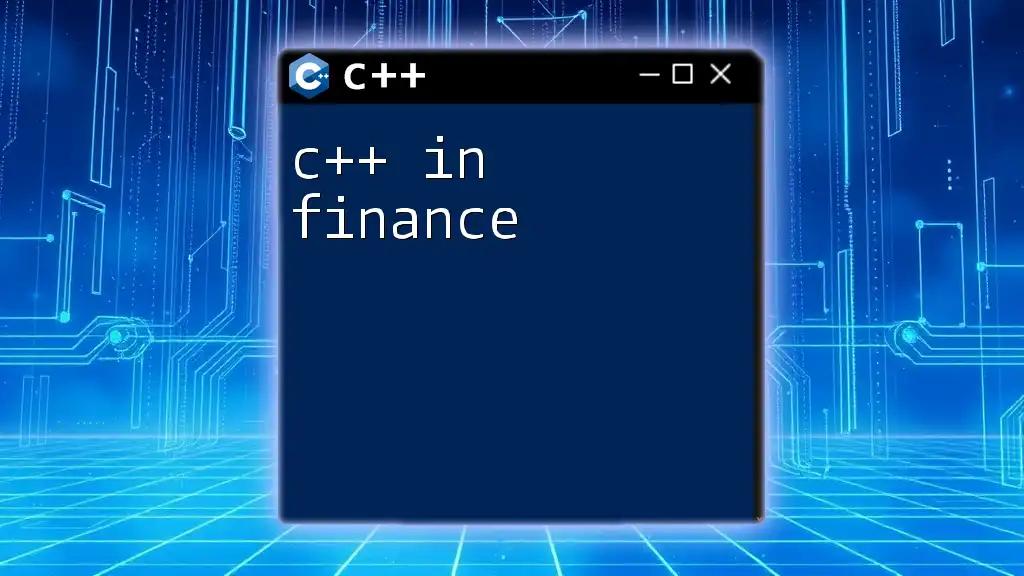
Financial Models and Algorithms in C++
Fundamental Financial Concepts
Time Value of Money (TVM) Understanding the time value of money is pivotal in finance. The core premise is that money available today is worth more than the same amount in the future due to its earning potential.
To calculate the Present Value (PV) and Future Value (FV), use the following formulas:
- PV = \( \frac{FV}{(1 + r)^n} \)
- FV = \( PV \times (1 + r)^n \)
Here’s a simple implementation in C++:
double calculatePresentValue(double futureValue, double rate, int periods) {
return futureValue / pow((1 + rate), periods);
}
Valuation Models When it comes to Discounted Cash Flow (DCF) analysis, you are estimating the value of an investment based on its expected future cash flows. Here’s a simple implementation:
double calculateDCF(double cashFlow, double discountRate, int periods) {
return cashFlow / pow((1 + discountRate), periods);
}
Risk Management Techniques
Value-at-Risk (VaR) Calculation Value-at-Risk is a widely used risk management tool that provides a probability-based estimate of the potential losses in an investment portfolio. Here's a basic representation of the calculation in C++:
double calculateVaR(double mean, double stdDev, double confidenceLevel) {
// Assuming a normal distribution
double zScore = ... // z-score corresponding to the confidence level;
return mean - (zScore * stdDev);
}
Monte Carlo Simulation Monte Carlo methods are a statistical technique used to model potential outcomes of uncertain variables. In finance, it can be used for asset pricing or risk assessment.
#include <cstdlib> // For rand()
#include <ctime> // For time()
double monteCarloSimulation(int simulations, double initialPrice) {
srand(time(0)); // Seed for random number generation
double totalReturn = 0;
for (int i = 0; i < simulations; i++) {
double simulatedReturn = ... // Generate random return based on historical data;
totalReturn += initialPrice * simulatedReturn;
}
return totalReturn / simulations; // Average return
}
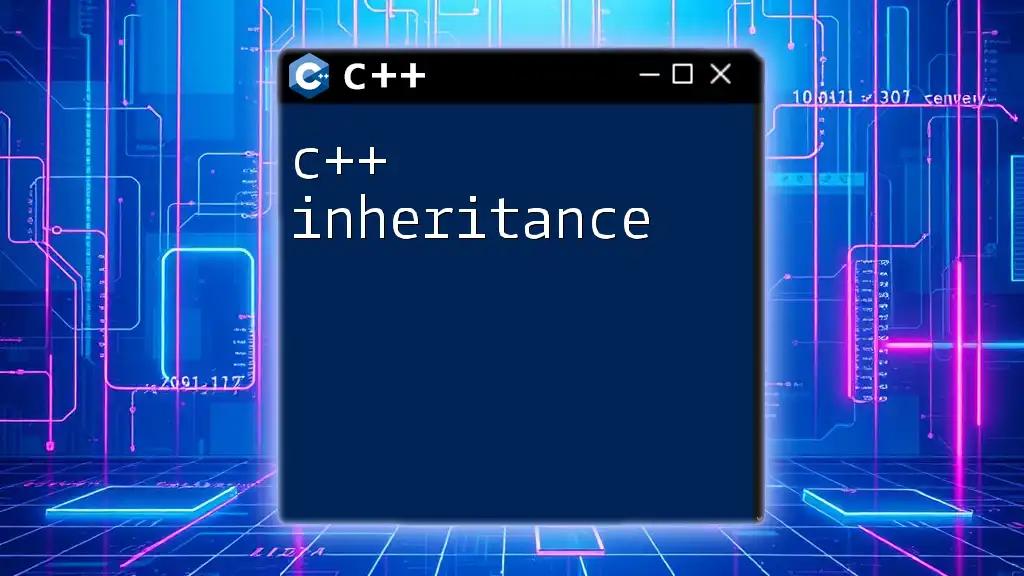
Advanced C++ Concepts for Finance
Templates and Generic Programming
What are Templates? Templates in C++ allow for writing generic and reusable code. This can be especially useful in financial algorithms, where you might need to calculate returns for various financial instruments.
template <typename T>
T calculateReturn(T initialPrice, T finalPrice) {
return (finalPrice - initialPrice) / initialPrice;
}
This simple template function can handle both integer and floating-point return calculations.
Standard Template Library (STL)
Using STL for Financial Applications The STL offers powerful data structures that can streamline development in financial applications. Common containers include vectors, maps, and lists. For instance, you can manage a portfolio using `std::map` to track asset allocations:
#include <map>
#include <string>
std::map<std::string, double> portfolio = {
{"Stock_A", 50.0},
{"Bond_A", 30.0}
};
// Function to print the portfolio
void printPortfolio(const std::map<std::string, double> &portfolio) {
for (const auto &asset : portfolio) {
std::cout << asset.first << ": " << asset.second << std::endl;
}
}

Libraries and Frameworks for Financial Applications
QuantLib
What is QuantLib? QuantLib is an open-source library widely considered the leading framework for quantitative finance. It offers a comprehensive set of tools for pricing derivatives, risk management, and financial modeling.
An example of pricing options using QuantLib in C++:
#include <ql/quadrature.hpp>
#include <ql/instruments/vanillaoption.hpp>
// Define your option and market conditions
VanillaOption option(...);
// Price the option using the appropriate method
option.NPV();
Boost Libraries
Introduction to Boost in Finance Boost provides a variety of libraries that complement C++'s capabilities. It includes libraries for mathematical functions, random number generation, and date/time manipulation, all of which are beneficial in financial applications.
An example of using Boost for advanced mathematical functions:
#include <boost/math/special_functions.hpp>
double calculateCumulativeNormalDistribution(double x) {
return boost::math::cdf(boost::math::normal(), x);
}
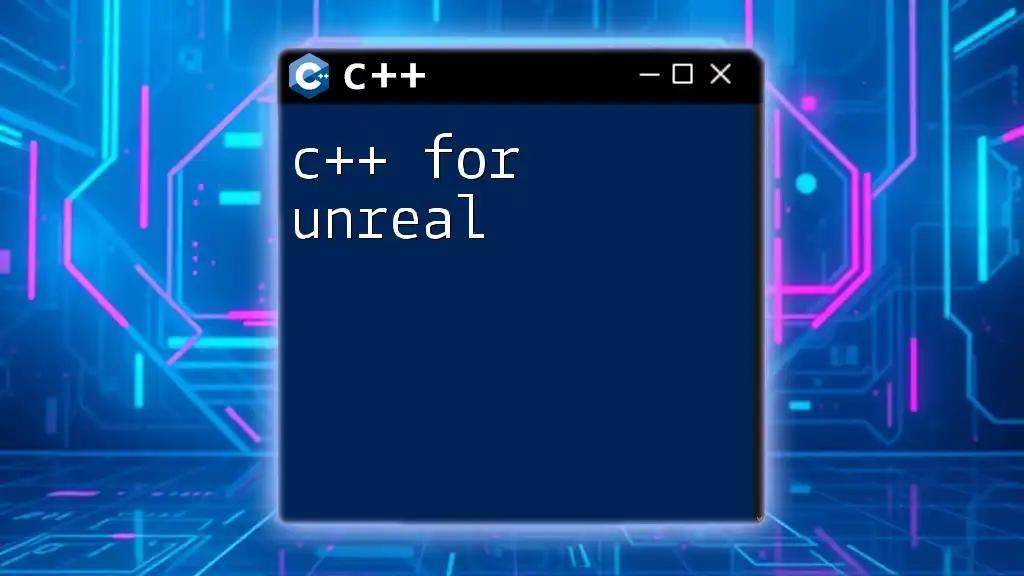
Debugging and Testing Financial Software
Importance of Testing in Financial Applications
In finance, reliability is paramount. Rigorous testing ensures that algorithms return accurate results and that risks are assessed correctly.
Types of Testing Implementing unit tests and integration tests can validate the accuracy of your financial models. Use libraries like Google Test for writing your test cases.
Common Debugging Techniques
Effective debugging techniques are essential for maintaining the integrity of financial software. Logging and error handling help track down issues and ensure the code behaves as expected under various conditions. Utilizing tools such as gdb can provide insights into runtime errors.

Conclusion
C++ plays a significant role in the finance sector, providing the performance and control that financial applications require. With an understanding of its features, models, algorithms, libraries, and best practices, you can effectively harness C++ for a variety of financial applications.
Keeping an eye on future trends, such as the integration of machine learning and AI, will also be crucial. As you embark on your journey to learn C++ for finance, consider engaging with educational resources and communities that can enhance your skills and knowledge.