C++ is the primary programming language used in Unreal Engine for creating high-performance games and applications, allowing developers to write efficient, custom gameplay mechanics and systems.
Here’s a simple example of a C++ class in Unreal Engine:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYGAME_API AMyActor : public AActor
{
GENERATED_BODY()
public:
AMyActor();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
};
Introduction to C++ in Unreal Engine
Unreal Engine is a powerful game engine widely used for creating high-quality games and interactive experiences. It offers an extensive toolkit that supports graphics rendering, physics, and gameplay mechanics, making it an industry favorite among developers.
C++ is the primary programming language for Unreal Engine. Its significance lies in its ability to provide high performance and control over system resources, which is crucial in game development. While the Unreal Engine also supports Blueprints—a visual scripting language—C++ gives developers the flexibility to create custom gameplay mechanics and optimize the engine's performance.
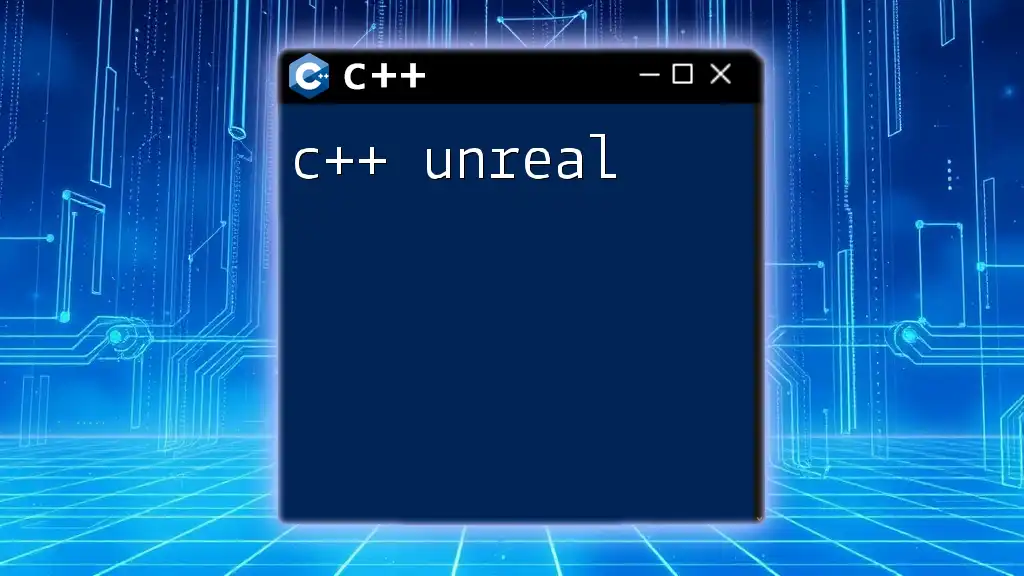
Setting Up Your Unreal Engine Environment
To get started with C++ for Unreal, you first need to install Unreal Engine. Head over to the Epic Games Launcher and download the latest version of Unreal Engine. Once installed, choose your preferred installation options.
After installation, creating a new C++ project is straightforward. Open the Unreal Engine, select "New Project," and choose the C++ tab. You will see various templates, such as "First Person," "Third Person," and "Blank." Select the one that suits your needs, name your project, and set the desired project settings.
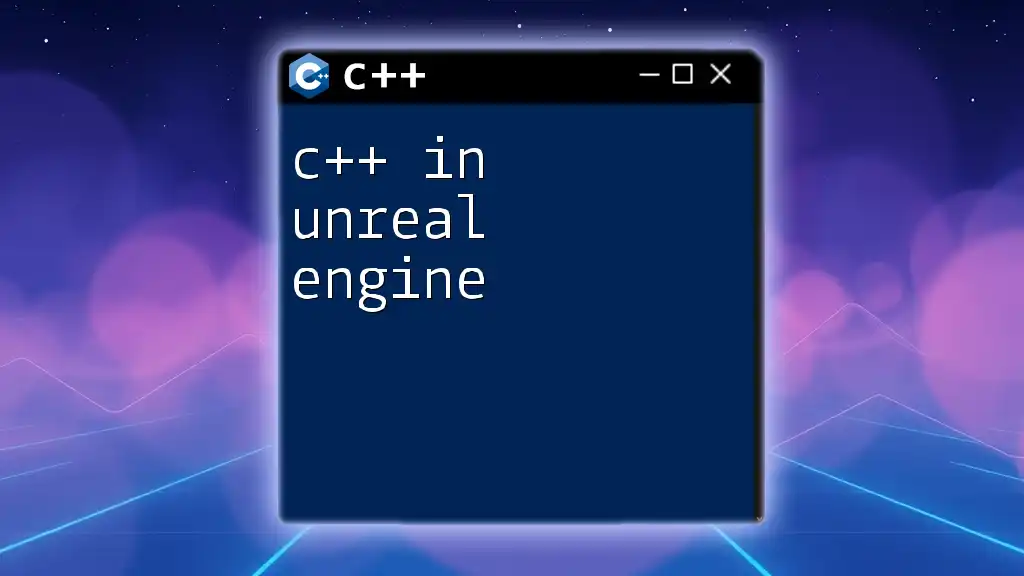
Understanding Unreal Engine’s C++ Structure
When you create a C++ project, you will notice a specific organization for files and folders.
- The Source folder contains your C++ code files, with each module having its own subdirectory.
- Within the module's directory, you’ll find `.cpp` and `.h` files, which represent the implementation and declaration of classes, respectively.
Understanding the structure will help you navigate the project efficiently and understand where to place your code.
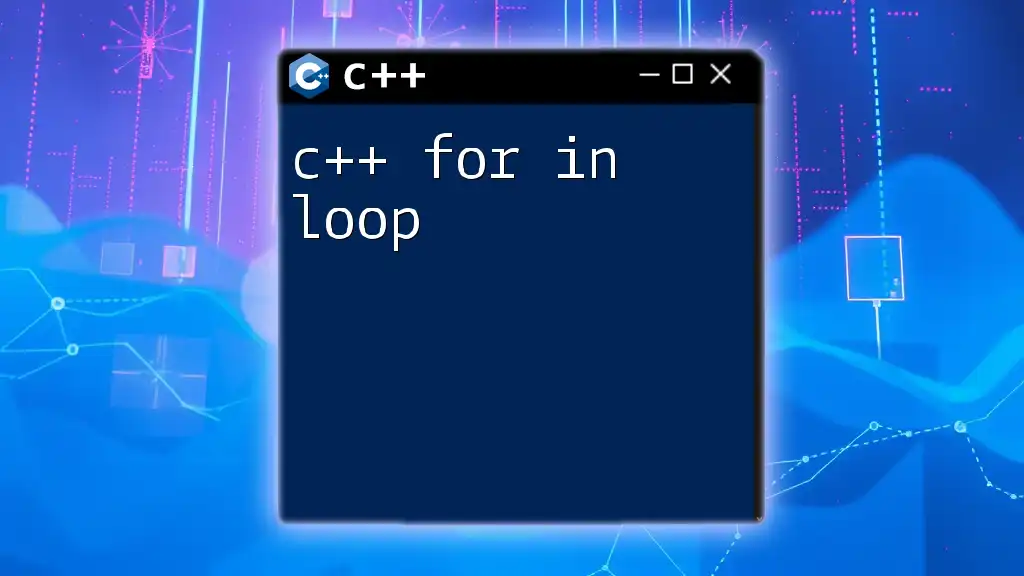
Core C++ Concepts for Unreal
Classes and Objects
In Unreal Engine, C++ classes represent various game elements such as characters, items, and environments. These classes can inherit properties and methods from other classes, allowing you to create complex hierarchies effortlessly.
For example, you can create a basic actor class as follows:
class MyActor : public AActor {
public:
MyActor();
void BeginPlay() override;
};
In this snippet, `MyActor` is a derived class from `AActor`, which is a fundamental class in Unreal that represents all objects that can be placed in the world.
Inheritance and Polymorphism
Inheritance allows you to create a new class based on an existing one, enabling code reuse and logical structure. Unreal Engine heavily employs polymorphism, allowing you to override methods for specific behaviors while keeping a consistent interface. Here is an example of overriding a method in a derived class:
class AMyCharacter : public ACharacter {
public:
virtual void Jump() override;
};
This approach makes modifying or extending the functionality of existing classes simple and intuitive.
Pointers and References
C++ makes extensive use of pointers and references, especially in the context of Unreal Engine. Understanding the distinction between pointers and references is crucial, as pointers allow dynamic memory management, which is essential for game development. For instance:
APlayerCharacter* MyCharacter = new APlayerCharacter();
This snippet dynamically allocates memory for a new player character, while understanding reference counting will help manage memory efficiently.
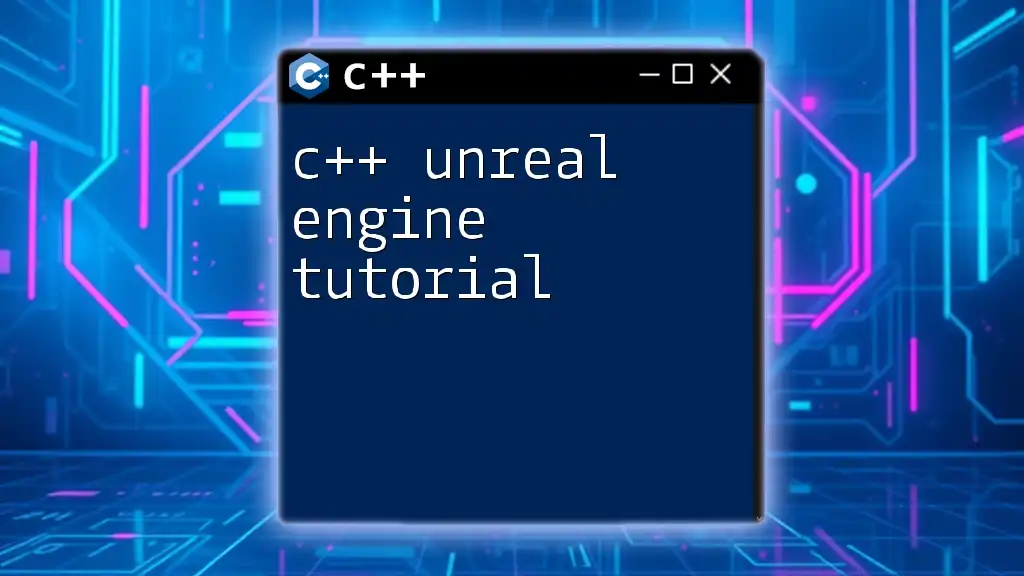
Creating Your First C++ Class
Once you've grasped the core concepts, it's time to create your first C++ class in Unreal Engine.
Defining Your Class
To define a new class, simply create a new `.h` file where you will declare your class. Here is an example of a basic actor class:
UCLASS()
class MYGAME_API AMyActor : public AActor {
GENERATED_BODY()
public:
AMyActor();
};
The `UCLASS()` macro tells Unreal to treat this class as a blueprintable class for the Engine, while `GENERATED_BODY()` is necessary for Unreal's reflection system.
Implementing Basic Functions
Now, let's implement a method to initialize the object when the game starts:
void AMyActor::BeginPlay() {
Super::BeginPlay();
// Initialization code here
}
The `Super::BeginPlay()` call ensures that any base class logic is executed before your custom logic, maintaining proper order of operations.
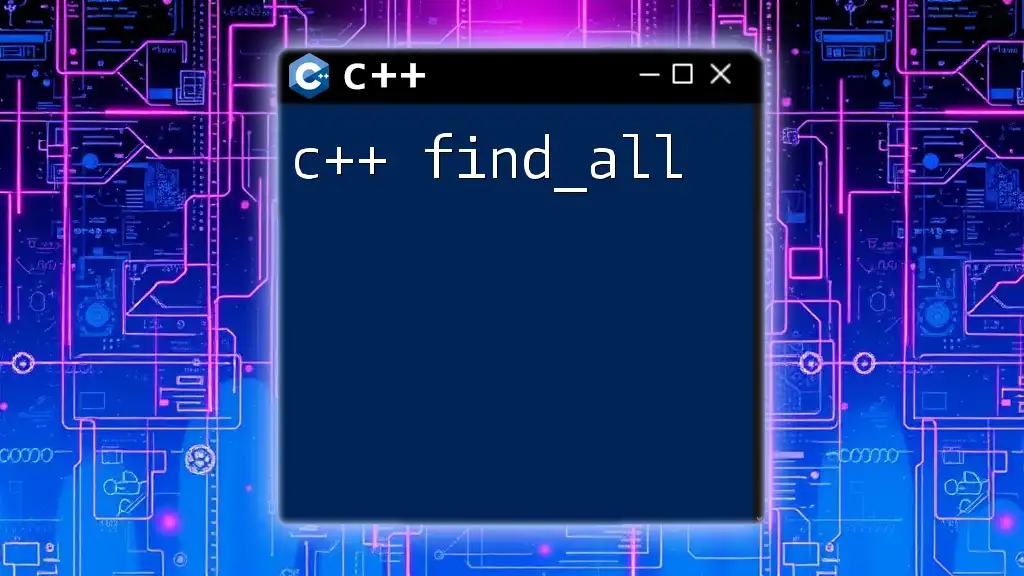
Working with Unreal Engine's APIs
Understanding the Unreal API
Unreal provides numerous APIs for functionalities such as rendering, networking, and gameplay mechanics. Familiarizing yourself with these APIs can drastically enhance your development capabilities in C++ for Unreal.
Using the Actor and Component Classes
Actors are the cornerstone of Unreal gameplay, while components allow you to add modular features to your actors. You can create custom components by inheriting from `UActorComponent`:
class UMyComponent : public UActorComponent {
GENERATED_BODY()
// Your code for the component's functionality
};
Creating and Managing Gameplay Elements
You can create other gameplay elements—like enemies or collectibles—by extending existing classes. For instance, a collectible item could be structured in the following way:
class ACollectibleItem : public AActor {
public:
void OnOverlapBegin();
void OnOverlapEnd();
};
These methods could handle logic for when a player interacts with the item, showcasing easy integration into Unreal’s event-driven architecture.
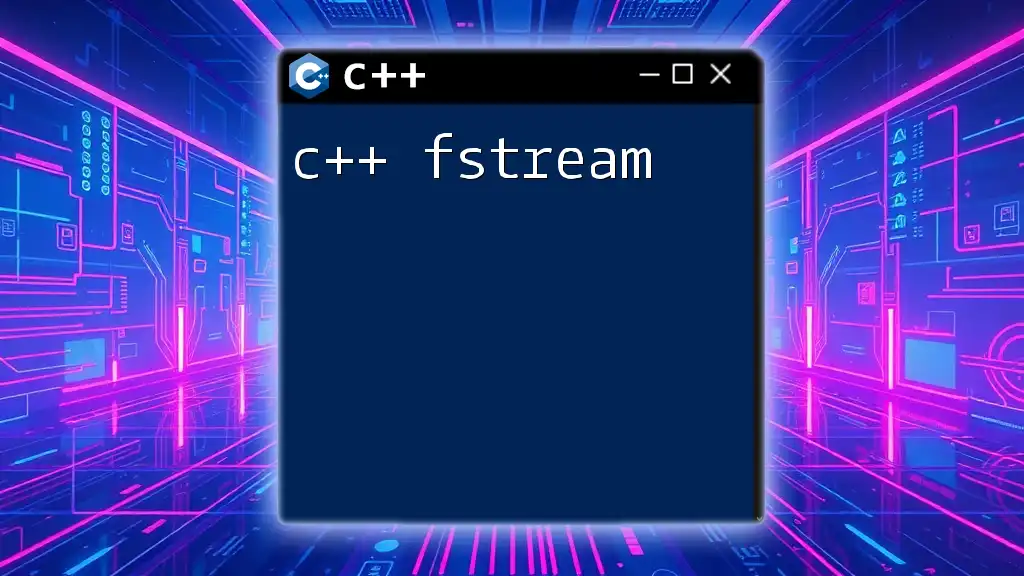
Memory Management in Unreal Engine
Understanding Garbage Collection in Unreal
One of the standout features of Unreal Engine is its garbage collection system. This system automatically manages memory, helping developers avoid memory leaks. It's essential to understand which types of objects are subject to garbage collection and which are not.
Smart Pointers vs. Raw Pointers
When managing memory, you must decide whether to use smart pointers or raw pointers. Smart pointers, like `TSharedPtr` and `TWeakPtr`, provide automatic memory management and ensure that memory is cleaned up when no longer needed. For example:
TSharedPtr<MyObject> MyObj = MakeShared<MyObject>();
Using smart pointers can enhance the reliability of your code and reduce the chance of memory-related errors.
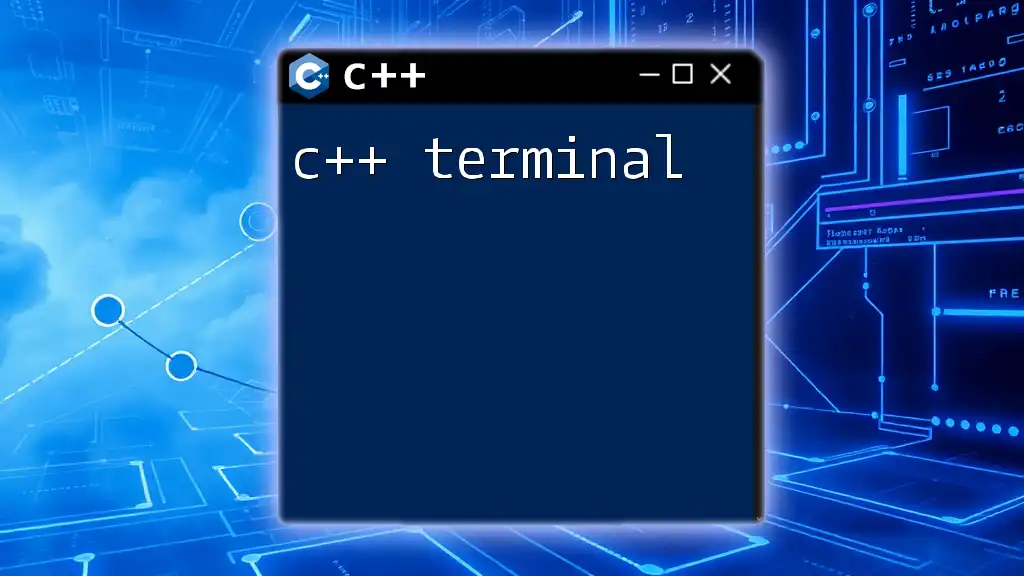
Debugging C++ in Unreal Engine
Setting Up Your Debugging Environment
Debugging is crucial for a successful development process. Ensure you have set up your IDE, such as Visual Studio, to allow for effective debugging of your C++ code in Unreal Engine.
Common Debugging Techniques
Leverage breakpoints and logging to troubleshoot your code. For logging, Unreal Engine provides an easy-to-use logging macro:
UE_LOG(LogTemp, Warning, TEXT("Debug message here"));
This line of code will print the specified message in the output log, assisting you in tracking down problematic areas in your code.
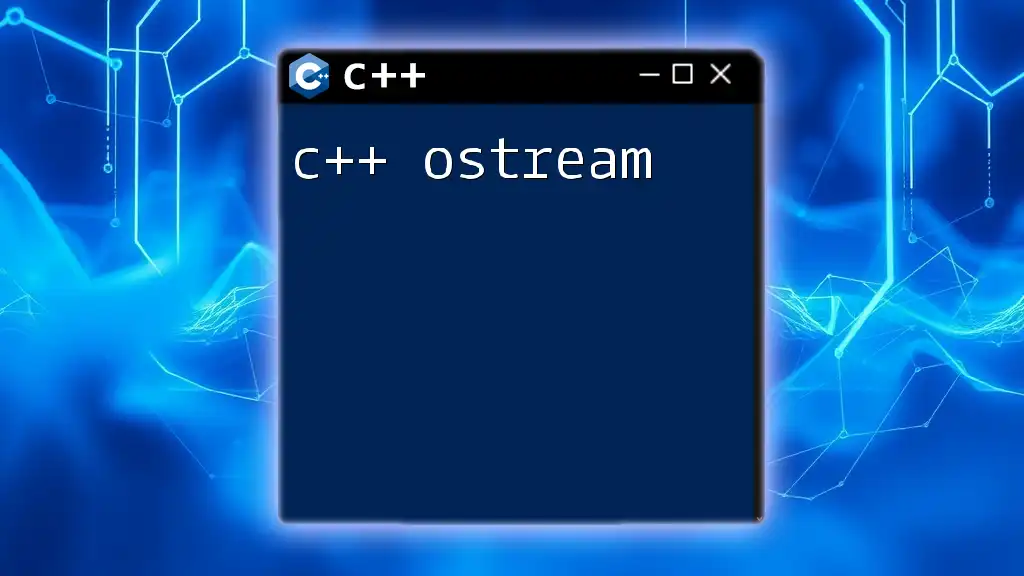
Best Practices for C++ in Unreal Engine
Organizing Your Code
Good organization of your code is paramount for maintainability. Use a consistent naming convention for classes, variables, and methods, making it easier for both you and others to read and understand the code.
Performance Optimization Techniques
To make the most of Unreal Engine's capabilities, prioritize performance optimization. Keep an eye on ticking frequency of actors, and utilize object pooling where applicable to manage resources effectively.
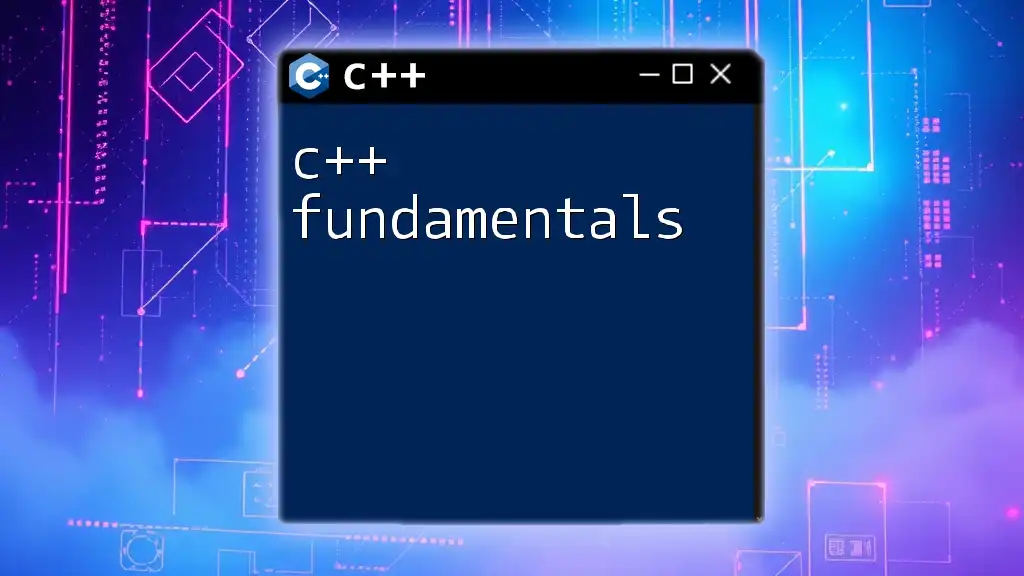
Conclusion
C++ for Unreal Engine opens up a world of possibilities in game development. By grasping the core principles outlined in this guide, you'll be well-equipped to leverage the power of C++ and build efficient, high-performance games. Remember, the path to mastering C++ in Unreal is filled with experimentation and practice. So, get out there, start coding, and bring your creative ideas to life!
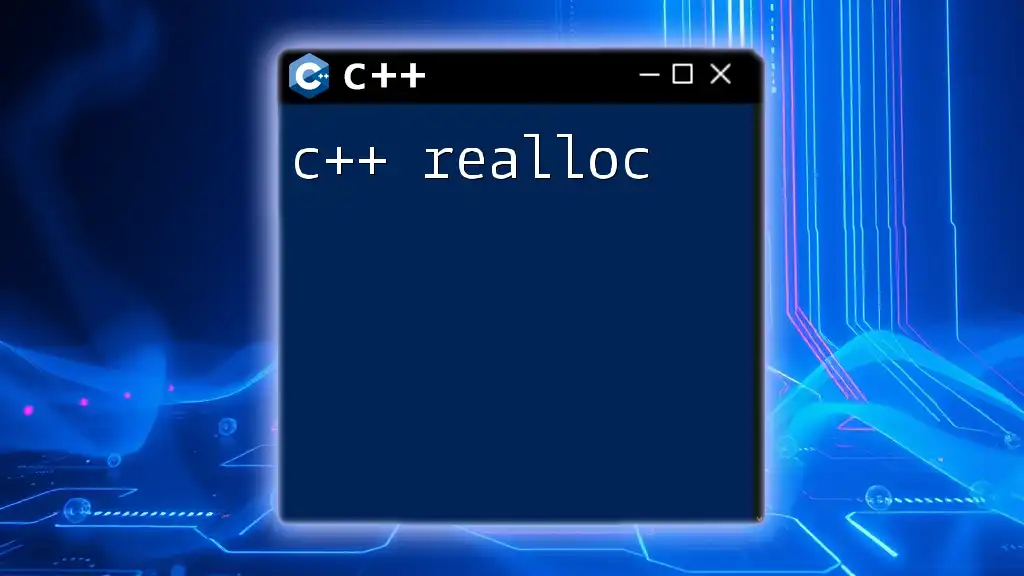
Resources and Further Reading
For more advanced topics and tutorials, don't forget to check out the official Unreal Engine documentation, participate in community forums, and explore specialized online courses to expand your knowledge and skills in C++ for Unreal.