C++ for automotive applications focuses on utilizing the C++ programming language to develop software solutions that enhance vehicle performance, safety, and functionality.
Here's a simple C++ code snippet for calculating the average speed of a car:
#include <iostream>
int main() {
float distance, time, avgSpeed;
std::cout << "Enter distance (in miles): ";
std::cin >> distance;
std::cout << "Enter time (in hours): ";
std::cin >> time;
avgSpeed = distance / time;
std::cout << "Average Speed: " << avgSpeed << " mph" << std::endl;
return 0;
}
Understanding `auto` in C++
What is `auto`?
The `auto` keyword in C++ is a powerful feature that allows for type inference during variable declaration. This means that instead of explicitly stating the type of a variable, you can use `auto` to let the compiler deduce the type automatically. The primary motivation behind using `auto` is to enhance code readability and reduce verbosity, especially when dealing with complex data types.
How Does `auto` Work?
When you declare a variable with `auto`, the compiler assesses the initializer expression on the right side of the assignment to determine the variable’s type at compile-time. This capability is beneficial, as it allows you to write less code while maintaining clarity.
For example:
auto number = 10; // `number` is deduced as int
auto pi = 3.14; // `pi` is deduced as double
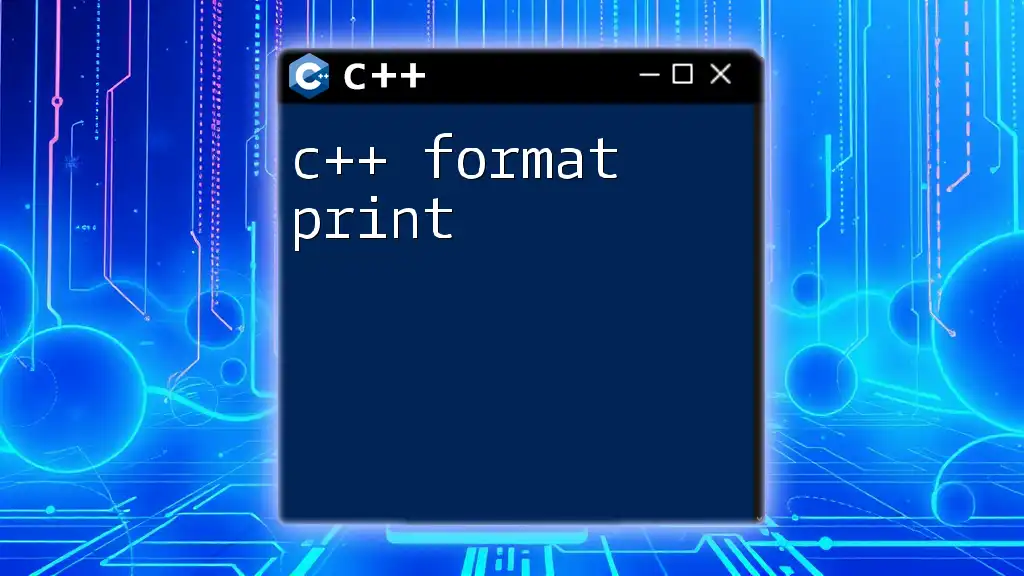
Using `auto` in C++ Code
Basic Examples of `auto`
Using `auto` to declare variables allows for easier programming, especially when handling types that are complex or verbose. Consider the following examples showcasing the simplicity introduced by `auto`:
auto count = 42; // count is deduced as int
auto temperature = 36.6; // temperature is deduced as double
auto name = "Alice"; // name is deduced as const char*
auto price = 12.99; // price is deduced as double
Using `auto` with STL (Standard Template Library)
One notable advantage of using `auto` is its seamless integration with the STL, particularly when working with containers like vectors and maps.
For instance, if you want to iterate through a vector, `auto` simplifies the iteration syntax:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
for (auto it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " "; // Output: 1 2 3 4
}
return 0;
}
In this example, using `auto` for the iterator makes the code cleaner and less error-prone.
Advanced Usage of `auto`
`auto` in Range-based For Loops
C++11 introduced range-based for loops, which allow you to iterate through collections without the hassle of manual indexing. Here, `auto` shines by automatically deducing the element type:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
for (auto element : vec) {
std::cout << element << " "; // Output: 1 2 3 4
}
return 0;
}
Auto with Lambda Expressions
`auto` can also be used effectively in lambda expressions, allowing for greater flexibility:
#include <iostream>
int main() {
auto add = [](auto a, auto b) { return a + b; };
std::cout << add(5, 10) << std::endl; // Output: 15
std::cout << add(2.5, 3.5) << std::endl; // Output: 6.0
return 0;
}
In this case, `auto` allows the lambda to accept any two types, enhancing its versatility.
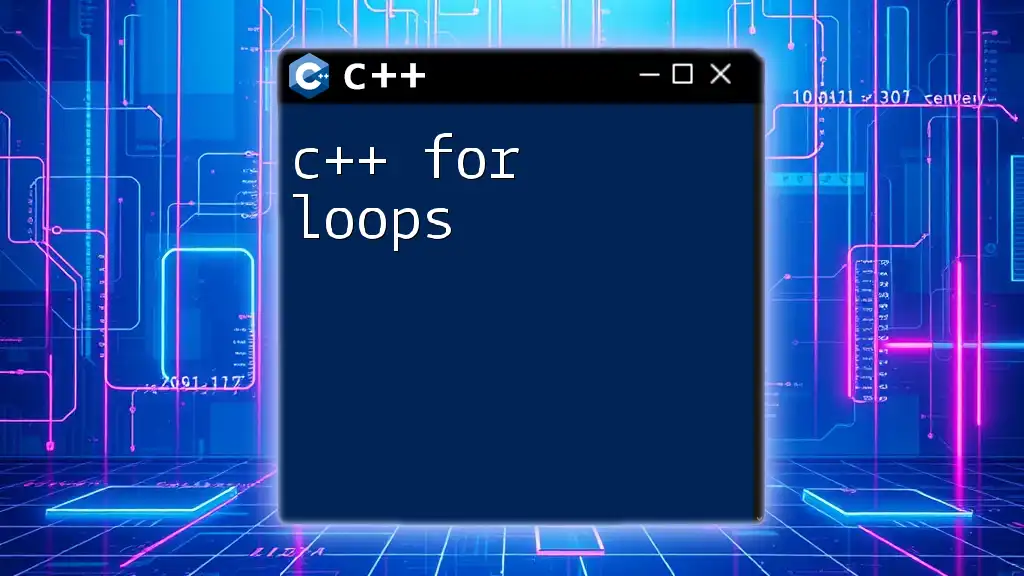
Benefits of Using `auto`
Improved Code Readability
Using `auto` can significantly improve code readability. By reducing verbosity, developers can focus on the logic rather than the types. For example, instead of writing:
std::vector<std::pair<int, std::string>>::iterator it = myMap.begin();
You can simply write:
auto it = myMap.begin();
Reduced Maintenance Overhead
When code structure changes, the use of `auto` minimizes the needed updates. For instance, if the return type of a function changes, there’s no need to adjust the type of the variable:
auto result = complexCalculation(); // If the function's return type changes, no need to modify this line.
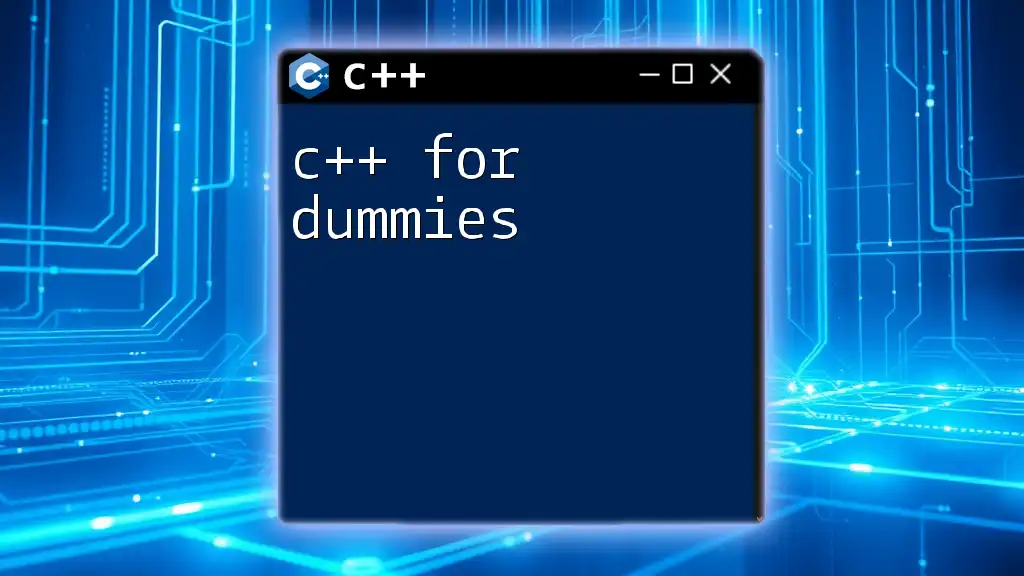
Common Pitfalls of Using `auto`
Losing Type Information
While `auto` is convenient, it can lead to potential pitfalls. Using `auto` may obscure what type a variable is, especially for complex initializers:
auto x = {1, 2, 3}; // `x` is deduced as std::initializer_list<int>, which may not be the intended type.
Ambiguity in Type Deduction
There may also be instances where the type inferred by `auto` could be misleading or not what the programmer expected:
auto ptr = new int(5); // `ptr` is deduced as int*, but this could lead to memory management issues if not handled properly.
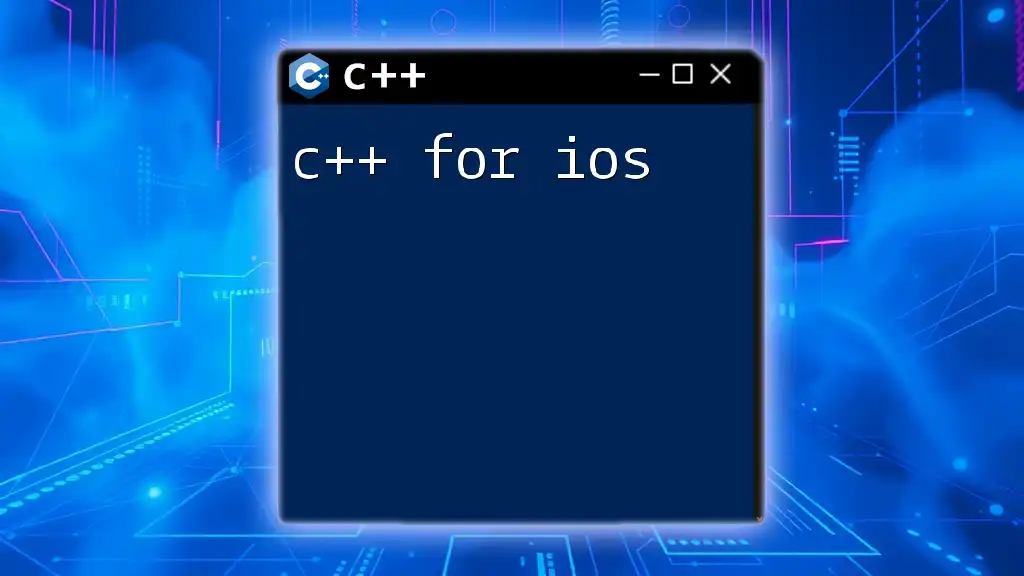
When to Avoid Using `auto`
While `auto` is valuable, it is essential to use it judiciously. In cases where clarity is paramount, such as public APIs or function signatures, explicit types can enhance understanding. Writing:
int add(int a, int b);
is clearer than:
auto add = [](auto a, auto b) { return a + b; };
In the context of publicly available functions, maintaining explicit types helps retain code clarity for other developers.
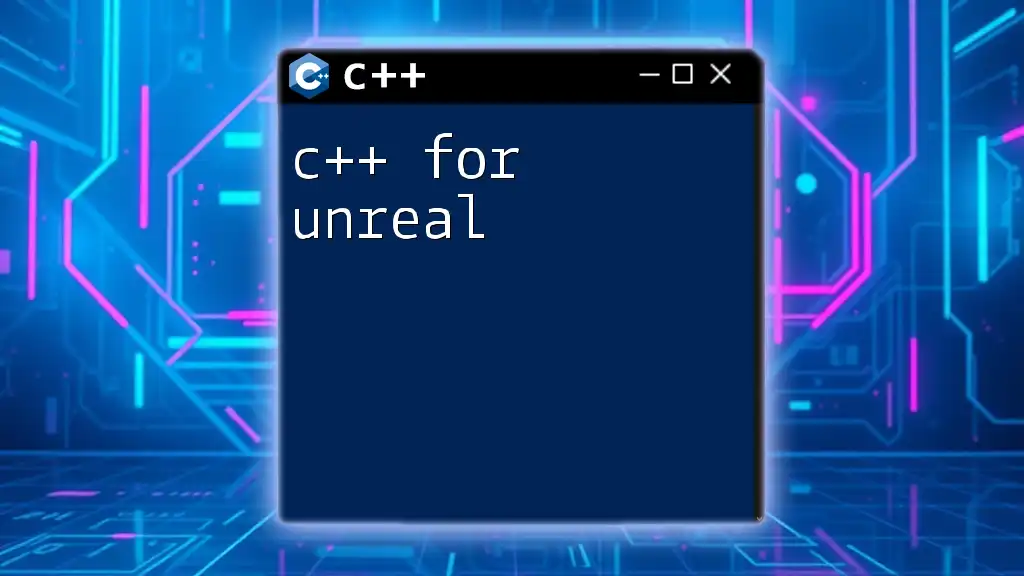
Conclusion
The `auto` keyword in C++ represents a leap towards cleaner, more efficient programming. By leveraging `auto`, developers can enhance readability and reduce boilerplate code, making their programs easier to write and maintain. As you continue your journey through C++, experiment with `auto` in various scenarios to appreciate its full potential.