In C++, the `cout` object from the iostream library is used to output data to the console, and its formatting can be customized using manipulators such as `std::setw` and `std::setprecision`.
Here's a code snippet demonstrating formatted output with `cout`:
#include <iostream>
#include <iomanip>
int main() {
double value = 123.456789;
std::cout << std::fixed << std::setprecision(2) << "Formatted value: " << value << std::endl;
return 0;
}
Understanding cout in C++
What is cout?
In C++, `cout` stands for "character output" and is used to print output to the console. This command is an object of the class `ostream` found in the `<iostream>` header file. It allows you to display text, numbers, and other data types, which is essential when you want to provide feedback or results of operations to users.
To use `cout`, you must include the `<iostream>` header and utilize the `std` namespace:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Syntax of cout
The basic syntax of `cout` is quite simple. You output data using the insertion operator (`<<`). The data can consist of strings, variables, or expressions.
For instance, you can concatenate different types of data within a single `cout` statement:
int number = 10;
std::cout << "The number is: " << number << std::endl;
It's important to recognize that `cout` is more type-safe and type-friendly compared to the traditional `printf` function. This means that errors related to data type mismatches are less common when using `cout`.
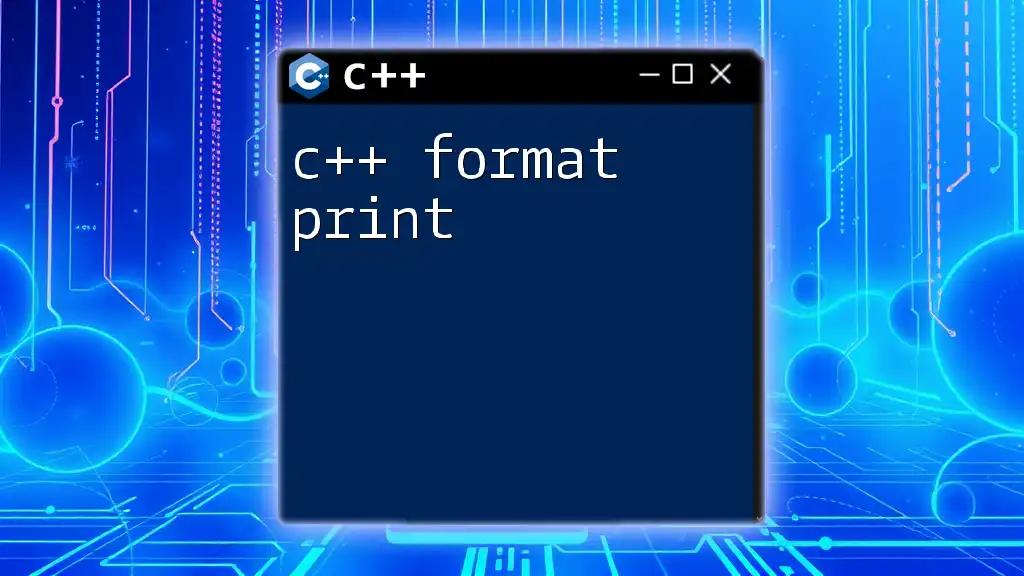
Basic Formatting with cout
Using Basic Manipulators
In C++, manipulators are special functions that help you format output. The most commonly used manipulators are:
- `std::endl`: Inserts a newline and flushes the output buffer.
- `std::flush`: Flushes the output buffer without adding a newline.
Here’s how you can use these manipulators:
std::cout << "Line 1" << std::endl << "Line 2" << std::flush;
Inserting Simple Formatting
Setting Width
You can set the width of the output using `std::setw`, which specifies a minimum number of characters to be displayed. If the content is shorter, it will be padded with spaces by default.
#include <iomanip>
std::cout << std::setw(10) << 42; // Outputs " 42"
This feature is particularly useful for creating well-aligned tabular output.
Aligning Output
C++ supports different output alignment options:
- Left alignment: Use `std::left` to align the content to the left.
- Right alignment: Use `std::right` to align content to the right.
- Internal alignment: Use `std::internal` for numeric values where the sign is aligned.
Example of different alignments:
std::cout << std::left << std::setw(10) << "Name"
<< std::right << std::setw(10) << "Age" << std::endl;
By controlling alignment and setting widths, you can create visually appealing outputs.
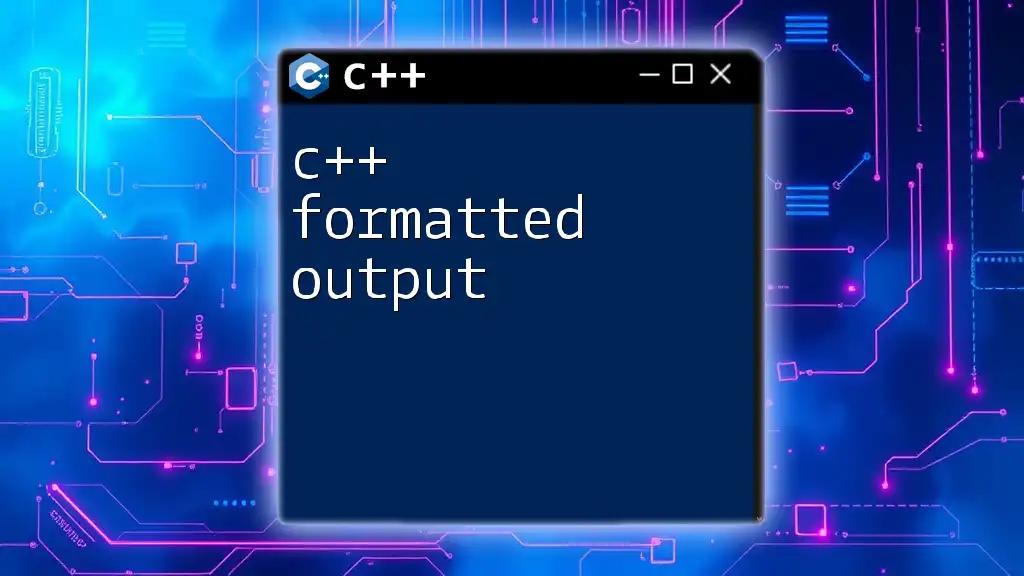
Advanced cout Formatting Techniques
Using setfill for Custom Fill Characters
The `setfill` manipulator allows you to specify a custom fill character for padding. By default, spaces are used, but you can change this:
std::cout << std::setfill('*') << std::setw(10) << 42; // Outputs "*******42"
This can be particularly useful when creating decorative or structured output.
Formatting Numbers with setprecision
Controlling Decimal Points
Precision is crucial when dealing with floating-point numbers. Use `std::setprecision` to control the number of digits after the decimal point. By combining it with `std::fixed`, you can ensure a consistent display format.
std::cout << std::fixed << std::setprecision(2) << 3.14159; // Outputs "3.14"
Formatting Currency
For currency formatting, you can combine `std::setprecision` with `std::fixed` to create outputs that resemble accounting formats.
std::cout << "$" << std::fixed << std::setprecision(2) << 12.345; // Outputs "$12.35"
This formatting is significant for applications that display financial data.
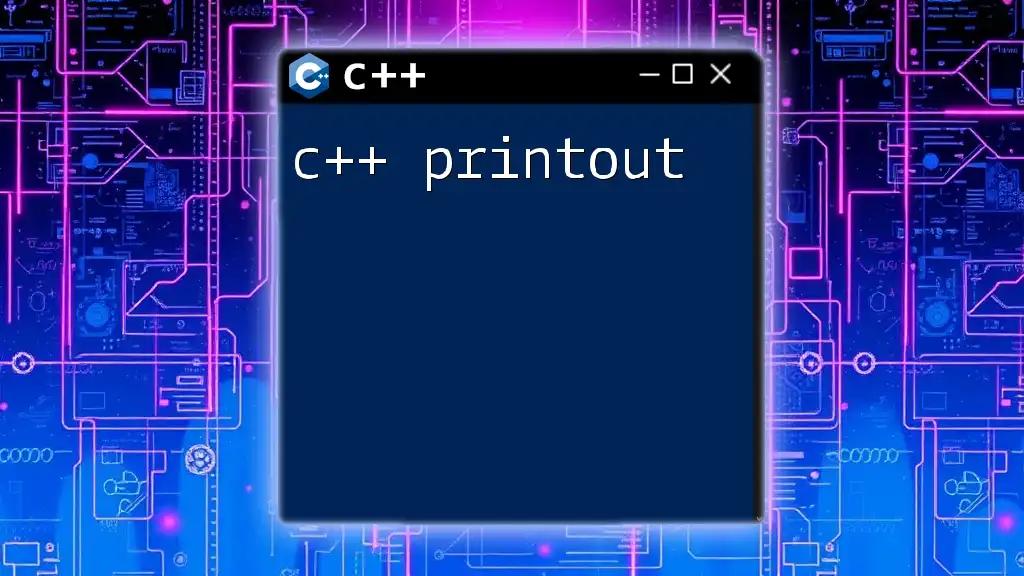
Formatting Strings with cout
Outputting Strings with Various Formats
Manipulating Case
You can convert string cases to enhance user readability or personal preferences. Using the `std::transform` function along with standard functions like `toupper` can achieve this.
std::string name = "John Doe";
std::transform(name.begin(), name.end(), name.begin(), ::toupper);
std::cout << name; // Outputs "JOHN DOE"
This functionality can help create outputs tailored to specific cases.
Padding Strings
You can pad strings for uniformity across displays. `std::setw` can also be beneficial for strings:
std::cout << std::left << std::setw(15) << "Username"; // Left pad output
This capability is excellent for creating tables or formatted logs.
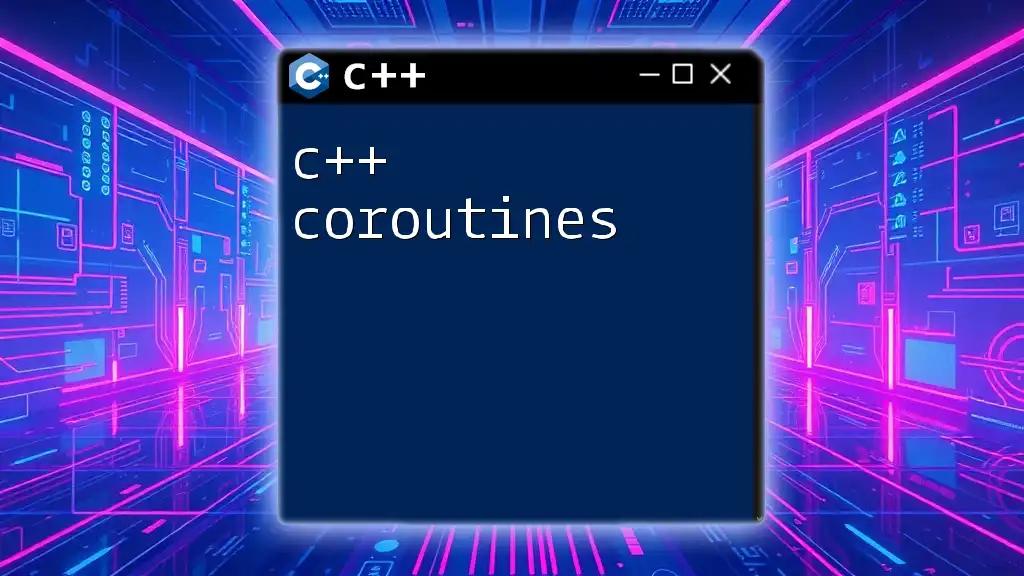
Custom Formatting Using std::ostringstream
Creating a String Stream
Using `std::ostringstream` allows you to build formatted strings that can be used later. This method is often more powerful than direct output through `cout`, especially for complex formatting.
#include <sstream>
std::ostringstream oss;
oss << "Formatted Output: " << std::fixed << std::setprecision(2) << 3.14159;
std::cout << oss.str();
Here, the string stream (oss) lets you prepare the string first, giving you the flexibility to manipulate and format the output thoroughly.
Why Use ostringstream?
`std::ostringstream` offers numerous advantages:
- Flexibility: You can format and manipulate the string before displaying it.
- Ease of use: Ideal for when you need to collect several pieces of information and format them first.
- Performance: It can enhance performance by reducing the number of I/O operations, especially in loops.

Conclusion
Understanding how to c++ format cout is critical for producing clear and well-structured outputs. Mastering the use of manipulators, alignment, precision, and string formatting substantially enriches the user experience and helps convey information effectively.
Whether you are working on simple console applications or more complex systems, effective output formatting can improve the overall functionality and usability of your programs. Practice using these techniques and experiment with various formatting methods to discover what works best for your applications.