The `count` method of a C++ map checks if a specified key exists, returning 1 if it does and 0 otherwise.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
int keyToCheck = 2;
std::cout << "Count of key " << keyToCheck << ": " << myMap.count(keyToCheck) << std::endl; // Output: 1
return 0;
}
What is a Map in C++?
A map in C++ is an associative container that stores elements in key-value pairs. Each element of a map consists of a unique key linked to a specific value. Unlike arrays, where elements are accessed via an index, maps enable fast lookups and are designed to access values using their associated keys. The underlying data structure typically utilizes a balanced binary tree, ensuring that operations on the map, such as insertion, access, and deletion, occur in logarithmic time.
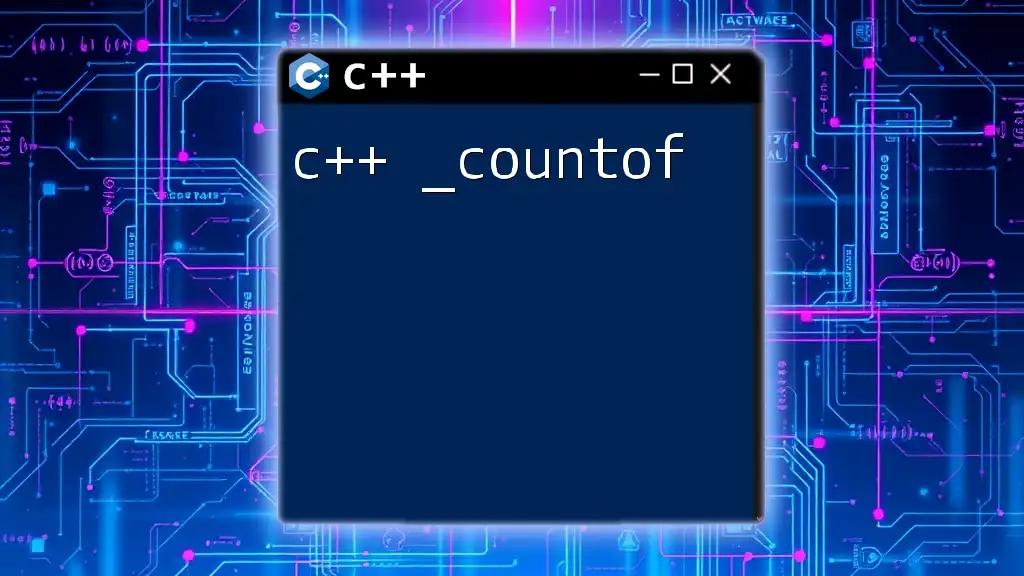
Why Use Maps?
Maps provide several advantages:
-
Efficiency: Maps allow for efficient retrieval of values given their associated keys. With logarithmic time complexity on operations, they are significantly faster than other structures like lists or vectors for search operations.
-
Flexible Keying: You can use various data types for keys, allowing for more complex data structure designs suited to varying needs.
In practical terms, maps can be particularly useful in applications such as implementating dictionaries, caching mechanisms, and associative arrays.

Understanding the Count Function in C++
What Does Map Count Do?
The `count` function in C++ maps serves a straightforward but crucial purpose: it checks whether a specific key is present in the map. When you use `map.count(key)`, the function returns the number of occurrences of that key. In the context of standard maps, this will always be either `0` (if the key does not exist) or `1` (if the key exists) since a map does not allow duplicate keys.
Syntax and Parameters
The syntax for using the count function is as follows:
map.count(key)
Here, `key` is the value of the key for which you want to check the count in the map. No additional parameters are required.
Return Type of Count Function
The return type of the `count` function is an `int`. This signifies how many times the specified key occurs in the map. As emphasized earlier, for a standard map, the return value will be `0` if the key is absent and `1` if it is present.
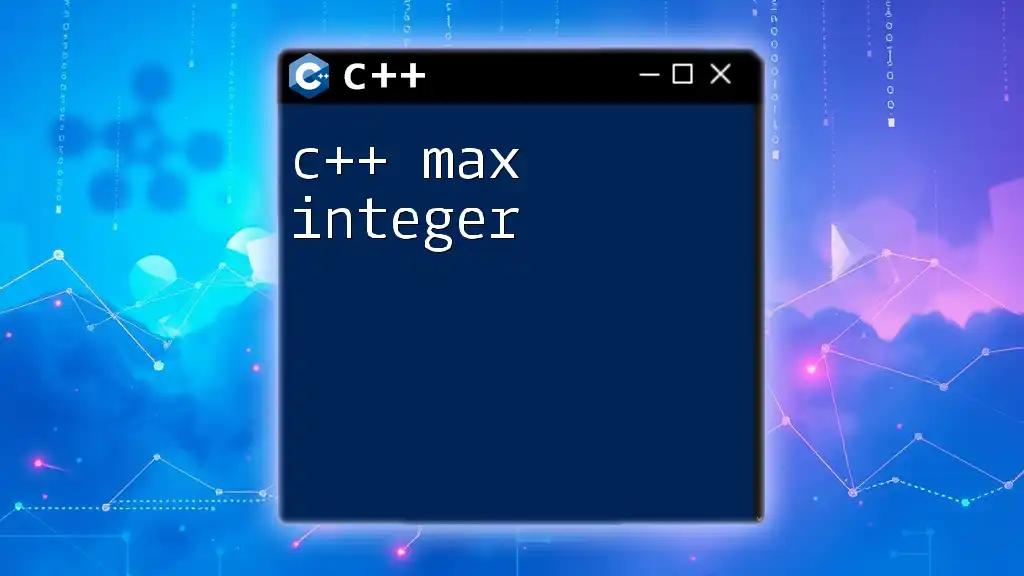
How to Use Map Count in C++
Inserting Elements in a Map
Before utilizing the count function, you'll want some entries in your map. Here's an example of how to insert elements:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
}
In the snippet above, we've created a map named `ageMap` that associates names (as keys) with age values (as values).
Checking Count of a Key
Now that we have a map with some entries, we can utilize the `count` function to validate the existence of a key:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
if (ageMap.count("Alice")) {
std::cout << "Alice is in the map." << std::endl;
} else {
std::cout << "Alice is not in the map." << std::endl;
}
}
In this example, we check if "Alice" exists in `ageMap`. The output for this case will clearly inform us of her presence.

Practical Examples
Example 1: Basic Map Count Usage
To illustrate the straightforward application of the `count` function, consider the following example showcasing different use cases:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> numberMap;
numberMap[1] = "One";
numberMap[2] = "Two";
std::cout << "Count for key 1: " << numberMap.count(1) << std::endl; // Output: 1
std::cout << "Count for key 3: " << numberMap.count(3) << std::endl; // Output: 0
}
In this example, we created a map of integers associated with their corresponding string values and utilized the `count` function to determine the presence of certain keys.
Example 2: Map with Custom Objects
Maps can also store custom objects, allowing for more complex data structures. Here’s how to use `count` on a map that holds custom objects:
#include <iostream>
#include <map>
struct Person {
std::string name;
int age;
};
int main() {
std::map<int, Person> personMap;
personMap[1] = {"Alice", 30};
personMap[2] = {"Bob", 25};
std::cout << "Count for key 1: " << personMap.count(1) << std::endl; // Output: 1
}
This example demonstrates how `count` is versatile across different data types.

Common Mistakes and Troubleshooting
Misunderstanding Return Values
One common misconception regarding the `count` function is assuming that returning `0` signifies the key exists when it actually means it does not. Ensure you understand that `count` returns `1` only when the key exists in a standard map.
Accessing Non-Existent Keys
Handling scenarios with non-existent keys is crucial to avoid runtime errors or incorrect assumptions. For instance:
if (personMap.count(3)) {
std::cout << "Key 3 exists." << std::endl;
} else {
std::cout << "Key 3 does not exist." << std::endl; // Correctly handling the case
}
This code correctly communicates whether key `3` is in `personMap`, demonstrating proper error handling.

Performance Considerations
Efficiency of Count in Large Maps
When working with large datasets, it's essential to consider performance implications. The `count` function operates in logarithmic time complexity, making it computationally efficient even with sizable maps. However, continue to monitor performance, as repeated calls in performance-critical applications may introduce overhead.
Summary of Best Practices for Using Map Count
- Utilize `count` sparingly: If you need to check for existence multiple times, consider storing the result instead of making repeated calls.
- Prefer `count` when looking for existence: The `count` function is ideal when checking for key existence—keep it simple and effective.
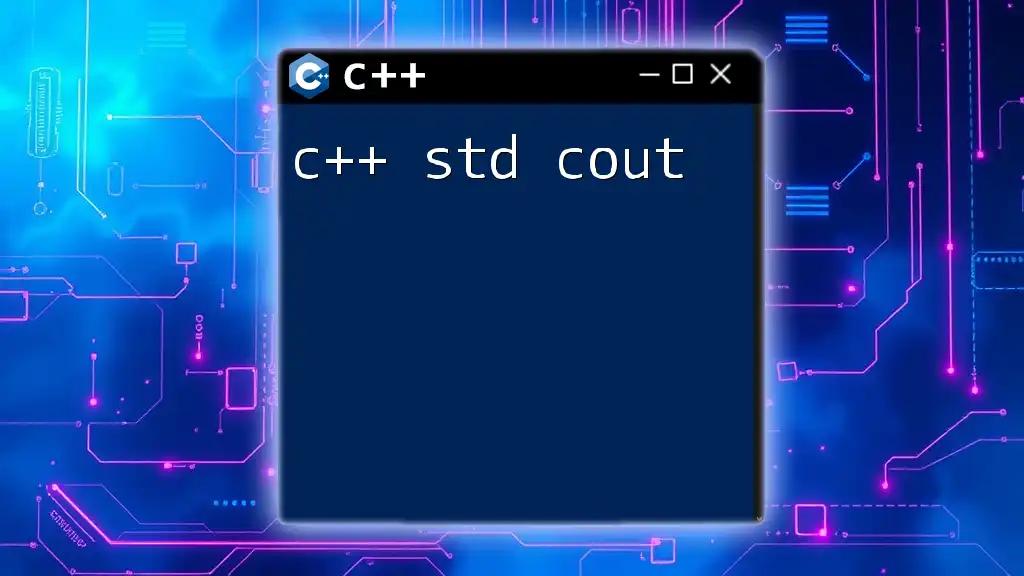
Conclusion
Understanding how to effectively utilize `c++ map count` empowers you to make more informed decisions in your coding endeavors. The count function is a fundamental aspect of working with maps, enabling you to verify the existence of keys efficiently. Practice with various data types and scenarios to build a strong foundation, and explore additional functionalities within C++ maps to further enhance your programming toolkit.

Additional Resources
Learning Materials
To deepen your knowledge of C++ maps and the Standard Template Library (STL), consider consulting the following resources:
- Books: Look for materials specifically covering C++ programming and STL usage.
- Online Courses: Websites like Coursera or Udemy often provide specialized courses on C++.
Community and Support
Join forums like Stack Overflow or C++ specific groups on Reddit for community support. Engaging with fellow learners and experts can provide invaluable insights to advance your understanding of C++.