In C++, `map` maintains sorted key-value pairs and allows for faster search operations at the cost of insertion speed, while `unordered_map` uses a hash table for faster average lookup times but does not guarantee order.
#include <iostream>
#include <map>
#include <unordered_map>
int main() {
std::map<int, std::string> orderedMap;
orderedMap[2] = "two";
orderedMap[1] = "one";
std::unordered_map<int, std::string> unorderedMap;
unorderedMap[2] = "two";
unorderedMap[1] = "one";
// Displaying elements
for (const auto& pair : orderedMap) {
std::cout << pair.first << ": " << pair.second << std::endl; // Output will be sorted
}
for (const auto& pair : unorderedMap) {
std::cout << pair.first << ": " << pair.second << std::endl; // Output may not be sorted
}
return 0;
}
Understanding C++ Maps
What is a Map?
A map in C++ is a container that stores elements in key-value pairs, similar to an associative array. Maps are particularly useful for maintaining a collection of unique keys linked to specific values. The key characteristics of maps include:
- Associative Array: Keys can be used to locate values.
- Unique Keys: Each key must be unique within the map.
- Automatic Sorting: The keys within a map are automatically sorted based on the key's less-than operator.
How Maps Work in C++
In C++, maps utilize a balanced binary tree (most commonly a Red-Black tree) as the underlying data structure. This allows for efficient insertion, deletion, and access operations. The map is part of the Standard Template Library (STL).
A typical declaration of a map looks like this:
#include <map>
map<KeyType, ValueType> mapName;
Example of Map Usage:
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, int> age;
age["Alice"] = 30;
age["Bob"] = 25;
for (const auto &pair : age) {
cout << pair.first << ": " << pair.second << endl;
}
return 0;
}
In this example, a map named `age` is created to store the ages of individuals. When iterated over, the output will demonstrate that the keys (names) are sorted in alphabetical order.
Performance Characteristics
The performance of maps is largely dictated by their underlying tree structure. Specifically, both insertion and deletion operations, alongside lookups, operate at a time complexity of O(log n). This efficiency makes maps suitable for scenarios where order and search speed are critical. In terms of space complexity, maps typically require additional memory to maintain their structure, compared to linear data structures like arrays.
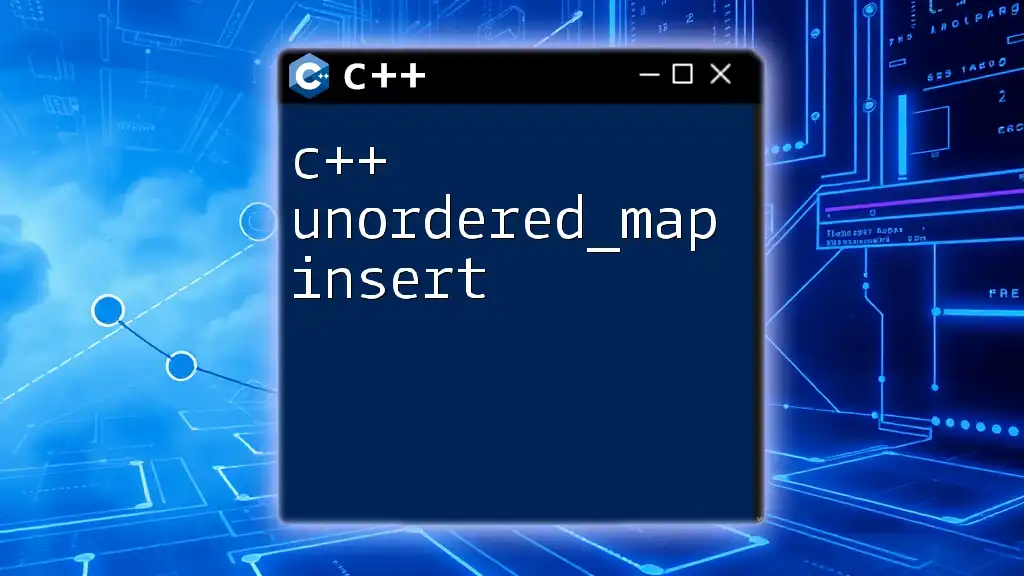
Understanding C++ Unordered Maps
What is an Unordered Map?
An unordered map is another container in C++ that allows for storage of key-value pairs. However, as the name indicates, the elements are not stored in any particular order, thanks to the use of a hash table, which provides faster access times.
How Unordered Maps Work in C++
Unordered maps utilize a hash table as their underlying data structure, allowing for average-case constant time complexity for insertion and access. In this instance, the hashing function must map keys to specific indices in the table.
A basic declaration of an unordered map would be:
#include <unordered_map>
unordered_map<KeyType, ValueType> mapName;
Example of Unordered Map Usage:
#include <iostream>
#include <unordered_map>
using namespace std;
int main() {
unordered_map<string, int> age;
age["Alice"] = 30;
age["Bob"] = 25;
for (const auto &pair : age) {
cout << pair.first << ": " << pair.second << endl;
}
return 0;
}
In this example, an unordered map named `age` stores the same data as previously, but the iteration order may vary with each run due to the nature of hash maps.
Performance Characteristics
Unordered maps boast average-case time complexity of O(1) for operations such as insertion and access, but in the worst-case scenario (e.g., hash collisions), this can degrade to O(n). The space complexity can also be higher due to the overhead of the hash table and its resizing policy.
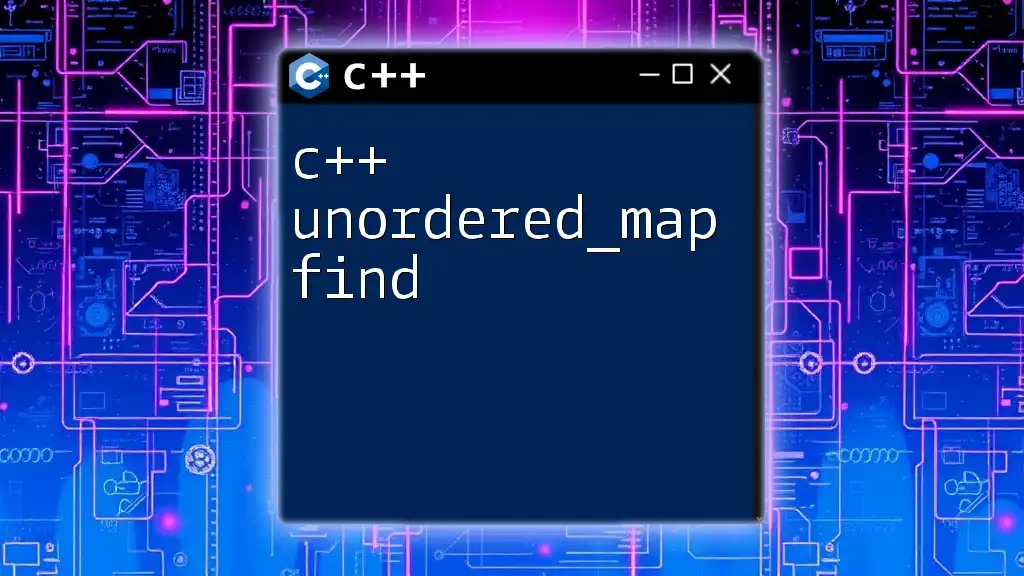
Key Differences Between Map and Unordered_Map
Ordering of Elements
The most fundamental difference between `map` and `unordered_map` is how they handle ordering. Elements in a map are sorted based on the keys, enabling predictable iteration order. Conversely, unordered maps do not maintain any order, which can result in different output sequences during iterations.
Performance Comparison
When considering performance, maps lend themselves to tasks where you require sorted data or a guaranteed order in iteration. Meanwhile, unordered maps are preferable when speed is essential, and the order of elements does not matter.
Memory Usage
Maps can be less memory-efficient due to their tree structure and node overhead compared to unordered maps, which can consume more memory due to the maintaining of a hash table. Developers should weigh these aspects based on their use cases and requirements.
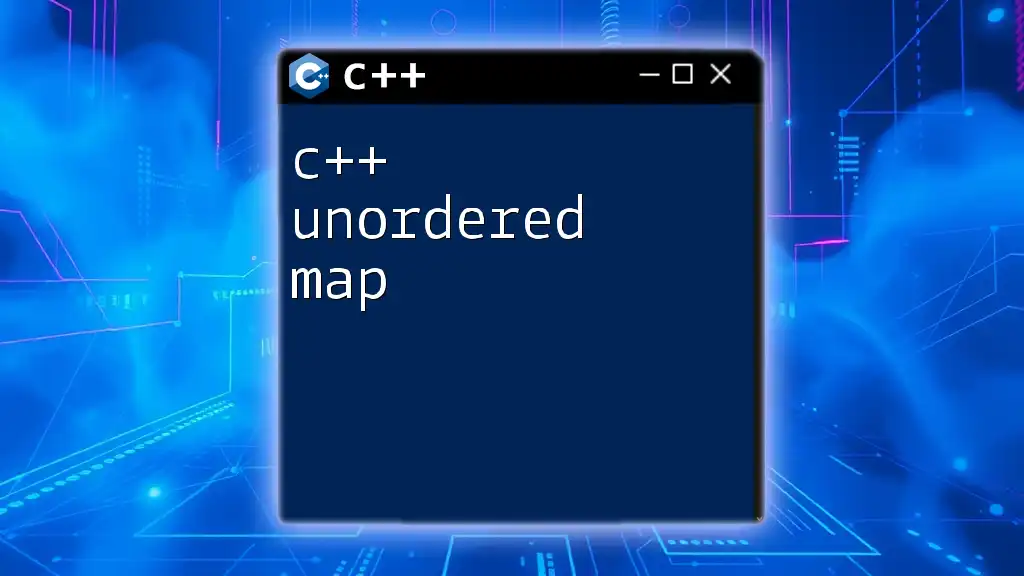
When to Use Map vs Unordered_Map
Use Cases for Map
Maps are beneficial when the order of elements is necessary or when you need to perform range queries. For instance, they are commonly used in maintaining sorted data collections, such as leaderboards or categorical information where sorting is essential.
Use Cases for Unordered_Map
Unordered maps are ideal for scenarios requiring fast lookups without caring for the order of keys, such as caching data or counting occurrences of elements where performance is prioritized over structure.
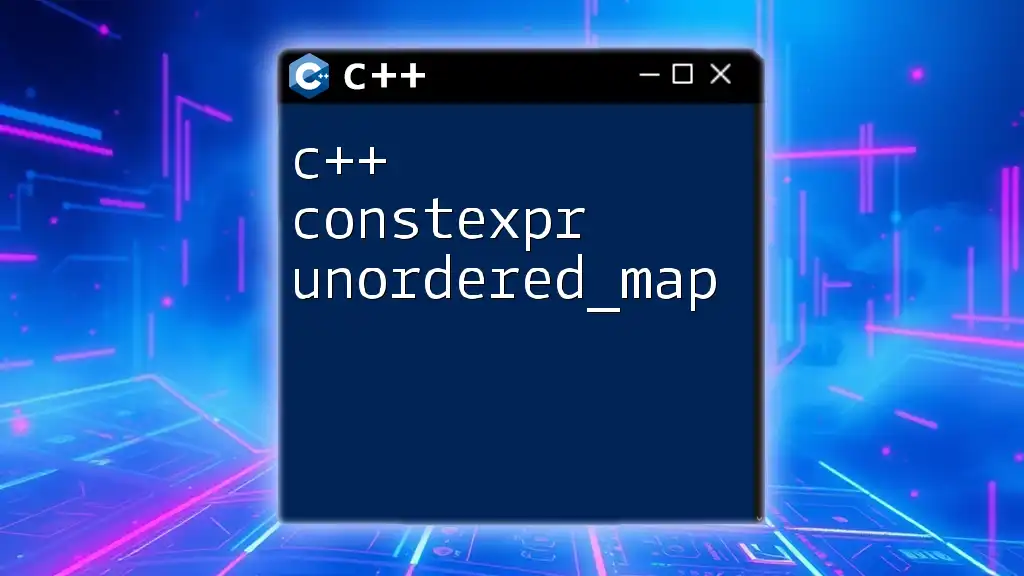
Real-World Applications
Example Scenarios
Imagine building a web application that tracks user sessions. An unordered map can be used to quickly look up active sessions based on user IDs. In contrast, a map might be used to log transactions where outcomes need to be sorted by transaction ID or date.
Best Practices
- Choosing the Right Data Structure: Always evaluate whether you need order (use `map`) or prioritize fast access (use `unordered_map`).
- Avoiding Common Pitfalls: Be wary of potential performance hits with `unordered_map` due to hash collisions and ensure to choose an effective hash function.
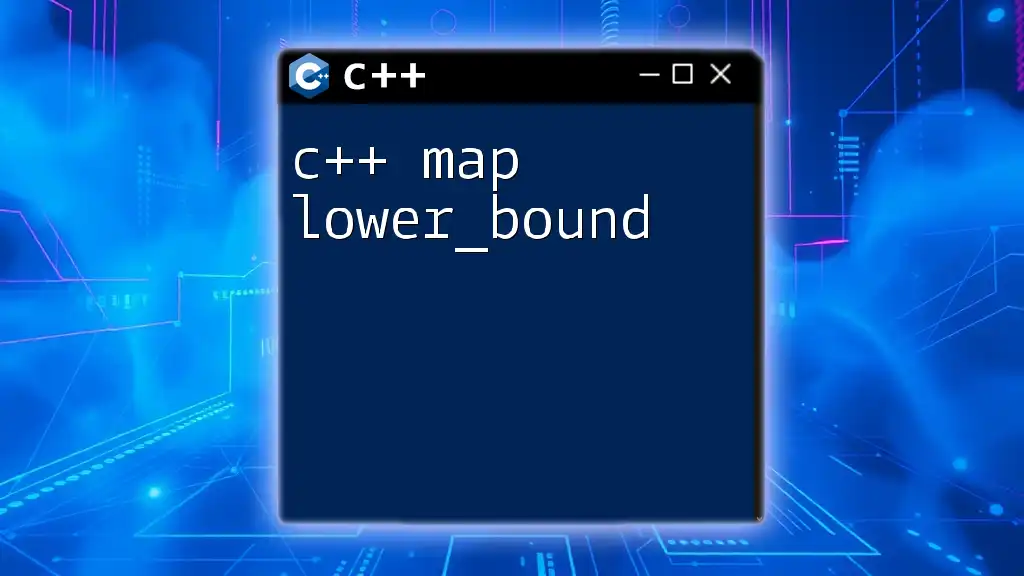
Conclusion
In conclusion, understanding the differences and when to use `map` vs `unordered_map` is vital for optimizing performance in your C++ applications. The choice between these two powerful data structures can significantly influence the efficiency and clarity of your code. Experimenting with both structures will deepen your understanding and enable you to make informed decisions tailored to specific problems.
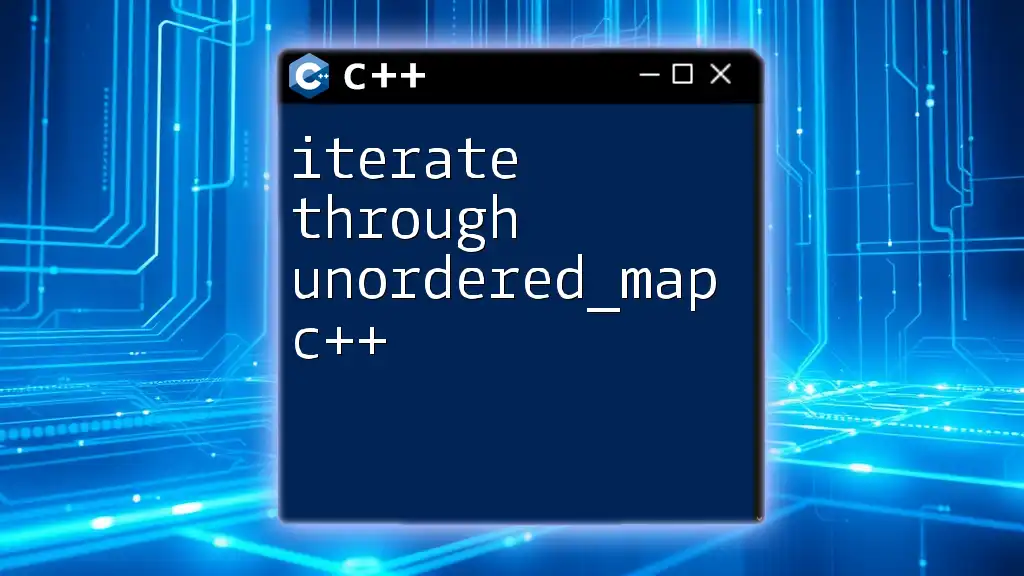
Further Reading
To further develop your knowledge, explore additional resources such as the official C++ documentation, STL tutorials, and community-driven coding forums.