In C++, `m_pi` is undefined by default since it's not a built-in constant, and you need to define it or include the appropriate library for mathematical constants.
#include <cmath>
#include <iostream>
// Define m_pi as a constant
const double m_pi = 3.141592653589793;
int main() {
std::cout << "Value of m_pi: " << m_pi << std::endl;
return 0;
}
What is `m_pi` in C++?
`m_pi` is commonly used to represent the mathematical constant Pi (π), which is approximately equal to 3.14159. This constant is essential in many mathematical calculations, especially in geometry, trigonometry, and physics. Constants like Pi can greatly enhance the readability and maintainability of code, providing clear indicators of what values represent without the need for comments. However, it's essential to note that `m_pi` is not part of the standard C++ library, leading to the issue of undefined behavior when it's referenced without being explicitly defined.

Understanding `m_pi`
What Does `m_pi` Represent?
Pi (π) is a fundamental constant that expresses the ratio of the circumference of a circle to its diameter. It occurs in various places in mathematics, such as in formulas for calculating areas and volumes of spheres, circles, and other shapes. Grasping this concept is pivotal, as it forms the basis for many advanced mathematical and engineering calculations.
Why `m_pi` is Undefined in C++
The absence of `m_pi` in the standard C++ library can be confusing for those accustomed to languages like Python or the C math library, where Pi might be readily available as `M_PI`. In C++, when you attempt to use `m_pi` without defining it yourself or including a library that provides it, the compiler will throw an undefined identifier error. This makes it crucial for developers to define mathematical constants themselves when working in C++.

Using Pi in C++
Defining Your Own Pi Constant
A straightforward way to deal with `m_pi` being undefined is to create your own constant. You can define it using `constexpr`, which ensures that the value is evaluated at compile time. Here’s how you can do it:
constexpr double m_pi = 3.141592653589793;
This code snippet establishes `m_pi` with high accuracy. Utilizing `constexpr` provides several benefits, including optimization by the compiler and ensuring that the constant value can be used in constant expressions.
Using the Standard Library for Constants
`<cmath>` Header
The `<cmath>` library offers various mathematical functions and constants. Although it does not include `m_pi` directly, it does provide `M_PI` in some implementations:
#include <cmath>
std::cout << M_PI; // Should print the value of Pi if defined in your library
Always verify if `M_PI` is defined in your specific compiler, as it may not be universally available across all standard libraries.
Defining Your Own Constant in Headers
A more structured approach is to create a header file that includes your mathematical constants, allowing for cleaner code and reuse across multiple source files. Here's a simple header structure:
// constants.h
#ifndef CONSTANTS_H
#define CONSTANTS_H
constexpr double m_pi = 3.141592653589793;
#endif // CONSTANTS_H
This header guard (`#ifndef`, `#define`, and `#endif`) prevents multiple inclusions of the header file, ensuring that `m_pi` remains defined only once.

Common Use Cases for Pi in C++
Geometry Calculations
One of the most common uses of Pi is in geometry, particularly for calculating the area of a circle. The formula for the area of a circle is:
$$\text{Area} = \pi \times r^2$$
Here’s how you can implement this in C++:
double area_of_circle(double radius) {
return m_pi * radius * radius;
}
The function `area_of_circle` takes the radius as an argument and returns the area by utilizing the defined constant `m_pi`. This promotes code clarity and leverages the mathematical property of the constant.
Trigonometric Functions
Pi plays a crucial role in trigonometric calculations, especially in functions like `sin()` and `cos()`, which require angles in radians. Since 180 degrees equal `π` radians, you can use your `m_pi` constant as follows:
double angle = m_pi / 2; // 90 degrees in radians
double sine_value = std::sin(angle);
This snippet shows how `m_pi` helps convert degrees to radians seamlessly, enhancing the accuracy and readability of trigonometric calculations.

Alternatives and Libraries
Boost Library
For those looking for a reliable source of mathematical constants, the Boost library is an excellent choice. It enhances the functionality of C++ significantly. You can include Boost.Math and access Pi with precision:
#include <boost/math/constants/constants.hpp>
std::cout << boost::math::constants::pi<double>(); // Outputs the value of Pi
Using established libraries can save time and reduce the likelihood of errors commonly associated with manual definitions.
Custom Math Libraries
Creating a custom math library for your application can also be beneficial. For example, you can encapsulate mathematical constants and functions in a class structure:
class MathConstants {
public:
static constexpr double pi() { return 3.141592653589793; }
};
This encapsulation provides a modular approach, allowing for upgrades and modifications without changing every reference to Pi throughout your code.
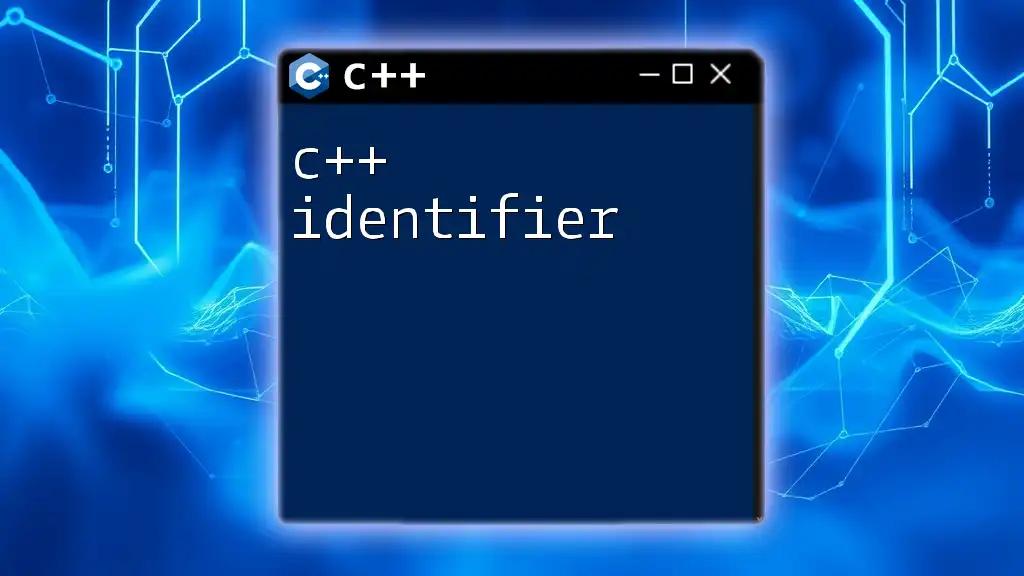
Handling Precision Issues
The Importance of Precision
When dealing with mathematical computations, precision is crucial. Floating-point representations can lead to inaccuracies. Using a constant like `m_pi` can introduce rounding errors, particularly important in applications like simulations and graphics.
Solutions for Increased Precision
To improve precision in your mathematical constants, consider using `long double`. This can significantly enhance the precision of your calculations:
constexpr long double m_pi_precise = 3.14159265358979323846264338327950288L;
By adopting `long double`, you can mitigate issues that arise from rounding errors, ensuring that your calculations yield results that are as accurate as possible.

Conclusion
In summary, while `m_pi` is undefined in C++, defining it yourself can lead to clear and maintainable code. The concept of constants is pivotal in programming, particularly when dealing with mathematical calculations. Whether you choose to define your own constants, leverage the standard library, or explore libraries like Boost, understanding the role of constants like Pi is essential for effective programming in C++. Good coding practices encourage careful consideration of how constants are defined and used, which directly impacts the clarity and efficiency of your code.
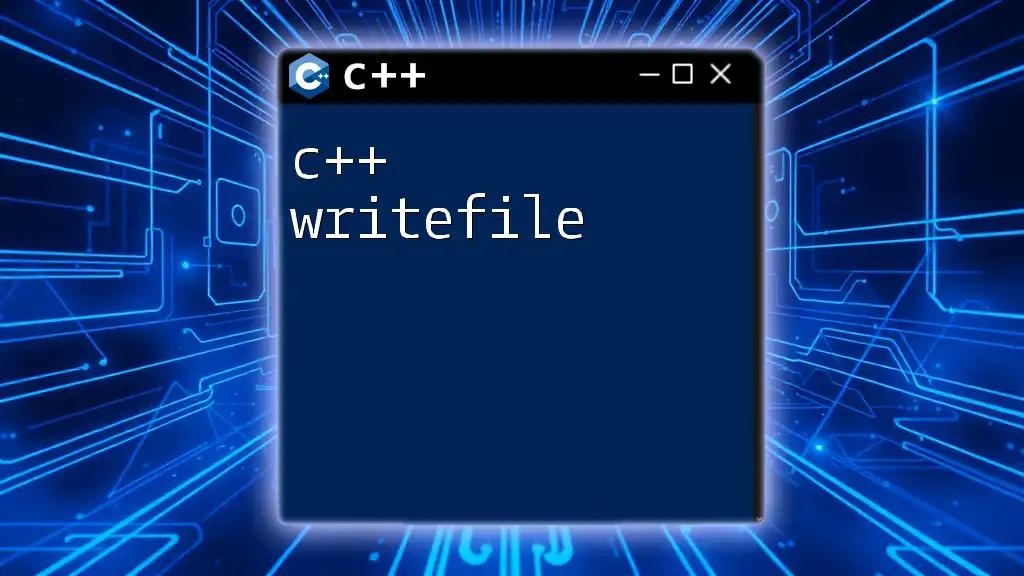
Additional Resources
To further explore mathematical computations in C++, consult various resources including programming books and online documentation, and participate in communities dedicated to programming discussions. Engaging with such resources will deepen your understanding and broaden your skills in employing constants effectively within C++.