The `#ifndef` directive in C++ is used to prevent multiple inclusions of a header file, ensuring that the code within the header is only processed once during compilation.
Here’s a code snippet demonstrating its usage:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Your header file content goes here
#endif // MY_HEADER_H
Understanding the #ifndef Directive in C++
What is #ifndef in C++?
`#ifndef` stands for "if not defined." It is a preprocessor directive used in C++ to check whether a particular macro has been defined earlier in the code. If it hasn't been defined, then the subsequent code will be included in the compilation process. The primary purpose of this directive is to avoid issues with multiple inclusions of header files, which can lead to redefinition errors and bloated compile times.
The key benefits of using `#ifndef` include:
- Preventing Multiple Definitions: Protects against multiple declarations of the same functions or variables, which can lead to confusing compilation errors.
- Improving Compilation Speed: By preventing redundant processing of files, it enhances the overall compilation time of the program.
How #ifndef Works with #define
In C++, preprocessor directives are instructions that are executed before the actual compilation starts. The `#define` directive is used to define a macro. When paired with `#ifndef`, it ensures that a block of code is only included in the compilation if a certain macro has not already been defined.
For example:
#ifndef MYHEADER_H
#define MYHEADER_H
// Code that gets included only if MYHEADER_H was not defined
#endif // MYHEADER_H
In this code, `MYHEADER_H` acts as a unique identifier for the header file. The `#ifndef` directive checks if `MYHEADER_H` is already defined:
- If it is not defined, the code between `#ifndef` and `#endif` is included in the compilation.
- If it is defined, that code is omitted.
The Syntax of #ifndef
The syntax for `#ifndef` is straightforward:
#ifndef UNIQUE_IDENTIFIER
// Code to include
#endif
- Keyword: The `#ifndef` directive, which begins the conditional statement.
- Identifier: A unique identifier that you must create for each header file. This name should be descriptive and preferably use uppercase letters to differentiate it clearly.
For example:
#ifndef MY_UNIQUE_HEADER
#define MY_UNIQUE_HEADER
// Content of your header file
#endif // MY_UNIQUE_HEADER
In the above example, if `MY_UNIQUE_HEADER` has not been defined yet, the code within this block will be processed, defining `MY_UNIQUE_HEADER` in the process.
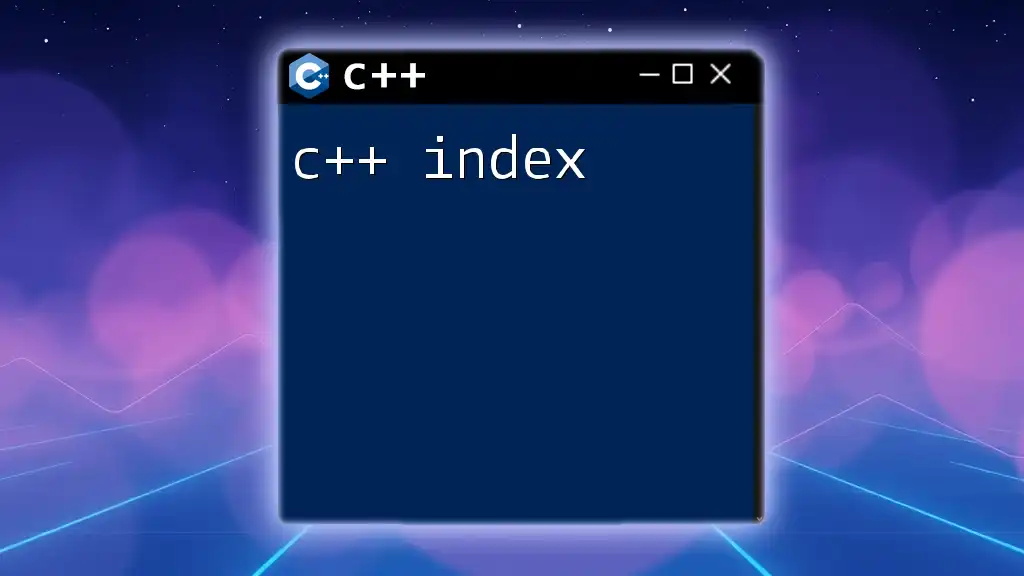
The Importance of #ifndef in Header Files
Preventing Multiple Inclusions
When a header file is included multiple times in different parts of a program, it results in the same declarations being processed again. This can cause the compiler to throw errors about redefinitions. The `#ifndef` directive serves as a safeguard against this by ensuring that the contents of a header file are only processed once.
For instance, without `#ifndef`, if a header file is included in multiple source files or included multiple times in the same source file, the compiler might face errors like:
error: redefinition of 'myFunction'
Using `#ifndef`, this problem is effectively eliminated.
Best Practices for Header Guards
When implementing `#ifndef`, it is crucial to adhere to certain best practices:
- Consistent Naming Conventions: Use all uppercase letters and underscores to separate words (e.g., `MY_HEADER_FILE_H`). This decreases the likelihood of name collisions.
- Include Guards in Every Header File: It’s a good practice to implement header guards in every header file you create to ensure protection against potential redefinitions.
Here’s a code snippet illustrating best practices:
#ifndef MY_HEADER_FILE_H
#define MY_HEADER_FILE_H
class MyClass {
public:
void myFunction();
};
#endif // MY_HEADER_FILE_H
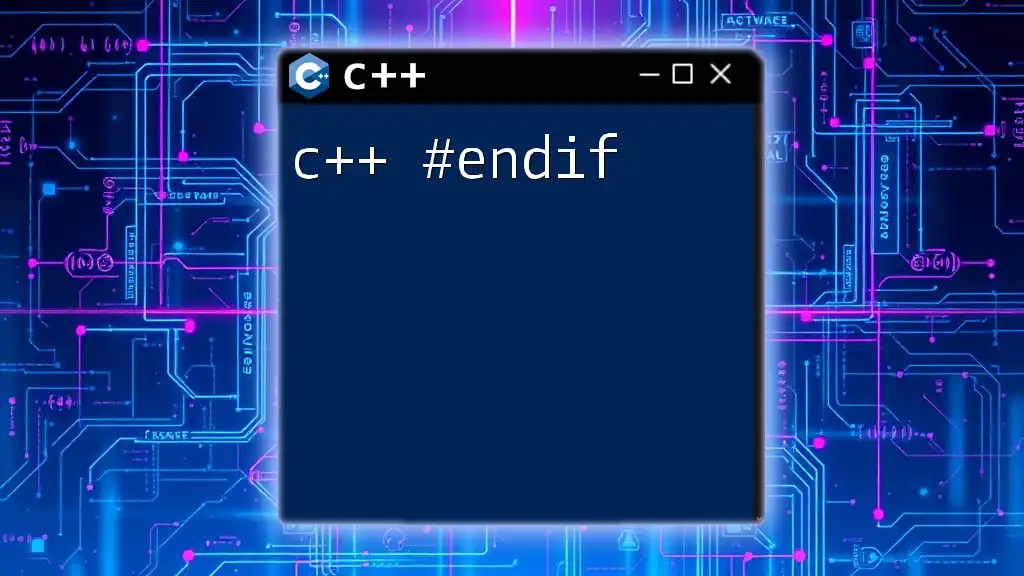
Using #ifndef in Real-world Applications
Practical Example: Creating Header Guards
Let’s go through a step-by-step guide on implementing `#ifndef` in a header file.
- Start with the `#ifndef` Directive: Begin the file with the `#ifndef` statement, followed by a unique identifier.
- Define the Identifier: Use the `#define` directive to define the identifier.
- Add Content: Place your class or function declarations within the guarded section.
- End with `#endif`: Conclude your header file with the `#endif` directive.
Example:
// MyClass.h
#ifndef MYCLASS_H
#define MYCLASS_H
class MyClass {
public:
void display();
};
#endif // MYCLASS_H
Debugging with #ifndef
The `#ifndef` directive can also assist during the debugging process. If you encounter unexpected errors relating to redefinitions, it may indicate that the header file is being included multiple times. Implementing proper header guards with `#ifndef` can quickly resolve such issues, saving you time and limiting frustration.
Common Mistakes to Avoid When Using #ifndef
While using `#ifndef`, there are some common pitfalls to avoid:
- Incorrect Identifier Naming: Ensure that your identifier is unique throughout your project to prevent collision.
- Forgetting to use #define: Every `#ifndef` must be paired with a corresponding `#define`; otherwise, the code may fail to compile properly. Here’s a code snippet illustrating a common mistake:
#ifndef MYCLASS_H
// Missing #define
class MyClass {
public:
void display();
};
#endif // MYCLASS_H
In this case, the missing `#define` will lead to multiple inclusions, causing redefinition errors.
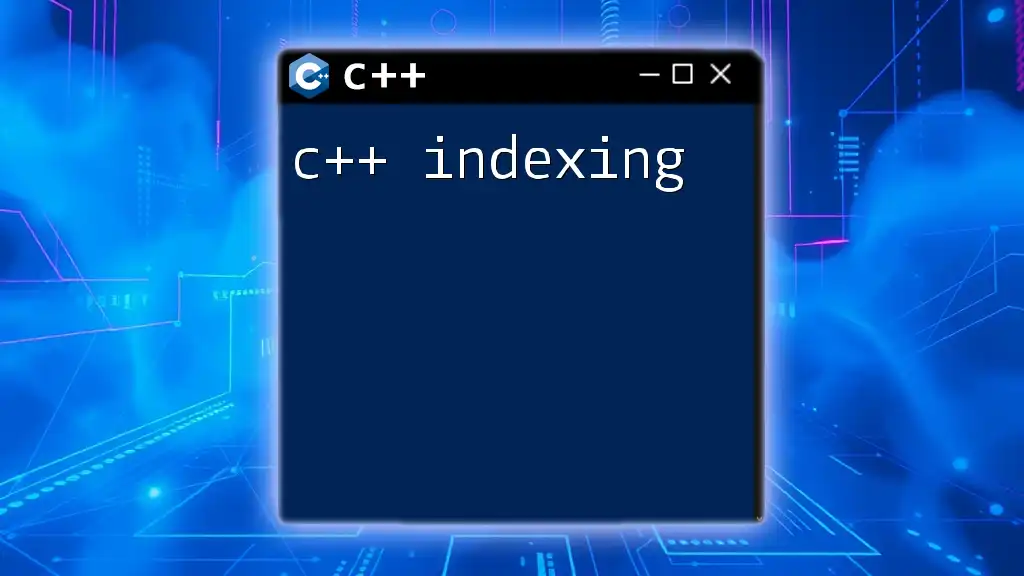
Alternatives to #ifndef
Other Preprocessor Directives
In C++, there are also alternative directives like `#pragma once`. This directive is used to ensure a header file is included only once in a single compilation. While `#ifndef` is standard and widely supported, `#pragma once` can be more convenient and less error-prone, as it does not require the manual definition and management of unique identifiers.
When to Use What
Choosing between `#ifndef` and alternatives like `#pragma once` largely depends on your personal or team's coding standards and the specific requirements of the project. While `#ifndef` is part of the C++ standard and works consistently across all compilers, `#pragma once` may not be supported by all compilers, making it less portable.
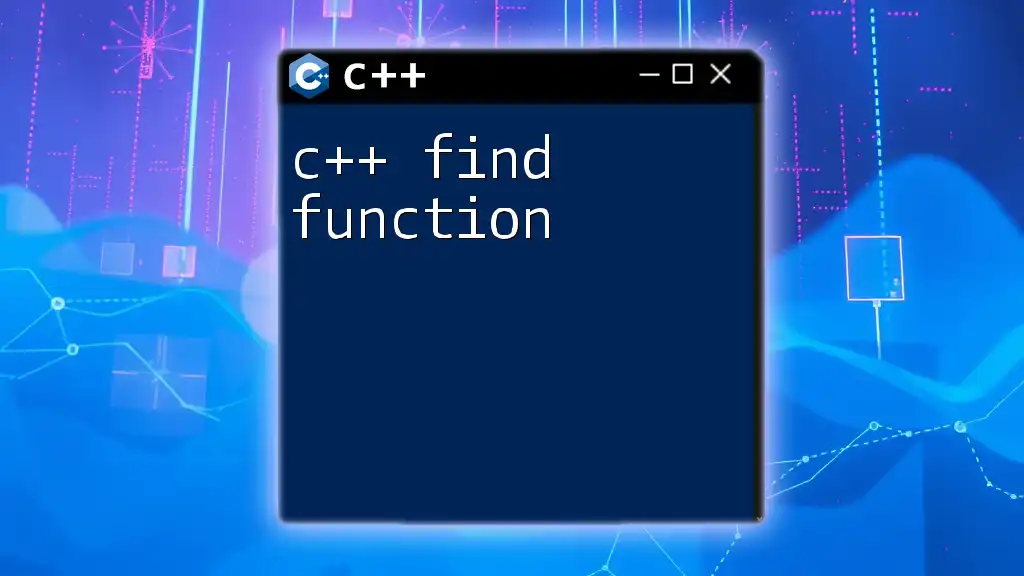
Conclusion
Summary of #ifndef Usage
The `#ifndef` directive is an essential tool in C++ programming that helps prevent multiple inclusions of header files, saving you from compilation errors and reducing build times. By following best practices and recognizing the common pitfalls, you can effectively use `#ifndef` to ensure clean and maintainable code.
Further Resources
To enhance your learning, consider exploring additional resources on C++ best practices, header file management, and preprocessor directives. Websites, tutorials, and official documentation provide excellent pathways to deepen your understanding and expertise in C++.