In C++, the `signed` keyword is used to declare a variable that can hold both positive and negative values, typically in integer types.
signed int myNumber = -10;
Understanding Signed Data Types
What is a Signed Integer?
In C++, a signed integer is a data type that can represent both positive and negative values. By design, signed integers have a sign bit that allows them to indicate whether a value is positive or negative. This contrasts with unsigned integers, which can only represent non-negative values.
Signed integers use the concept of two's complement for storing negative values. In two's complement, the most significant bit (MSB) indicates the sign of the integer: a 0 means positive, and a 1 means negative. This method simplifies binary arithmetic and allows the use of the same binary addition circuitry for both signed and unsigned values.
Signed Integer Range
The range of values that a signed integer can represent depends on its size. The standard C++ signed data types and their typical ranges are as follows:
- `signed char`: -128 to 127
- `short`: -32,768 to 32,767
- `int`: -2,147,483,648 to 2,147,483,647
- `long`: -2,147,483,648 to 2,147,483,647 (on most platforms)
- `long long`: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
Understanding the range is crucial, especially when designing algorithms that may encounter maximum or minimum values.

Using Signed Data Types in C++
Declaring Signed Integers
To declare signed integers in C++, you would typically use the `signed` keyword or simply use the type directly since integers are signed by default. Here's how to declare a signed integer:
signed int a = -10; // explicitly specifying signed
int b = 20; // signed by default
Using the `signed` keyword can enhance code readability, making it clear what the intended data type is, especially when dealing with a mix of signed and unsigned integers. However, be aware that it is often unnecessary unless you want to emphasize the signed nature directly for clarity.
Default Data Types
In C++, the default type for integers is signed. This means that when you declare a variable using `int`, it is treated as a signed integer unless otherwise specified. For example:
int num = 5; // num is signed by default
This automatic handling allows for more straightforward coding but can lead to confusion when mixing signed and unsigned types.
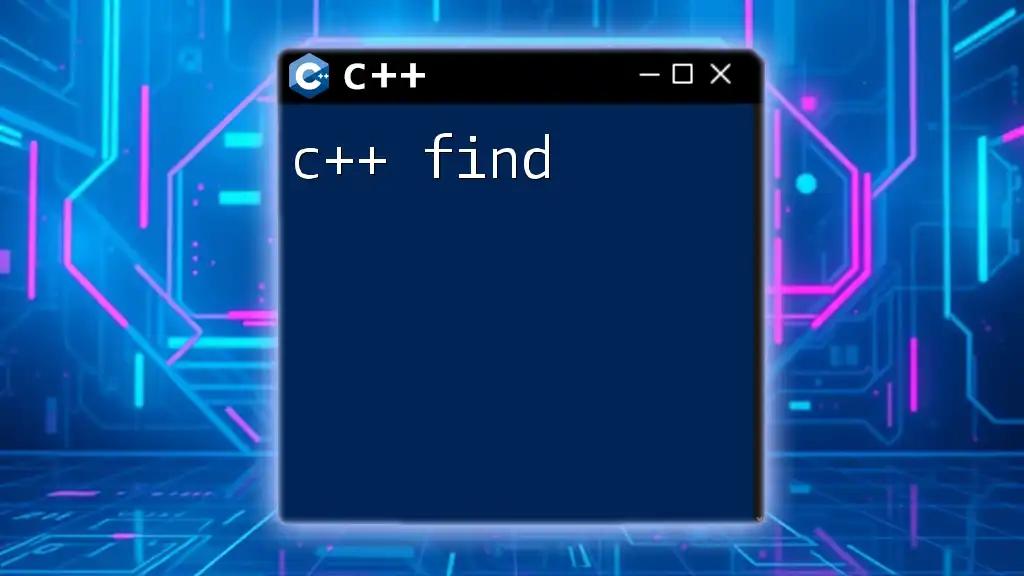
Operations with Signed Integers
Arithmetic Operations
Arithmetic operations on signed integers follow the same basic rules as with standard arithmetic. You can perform addition, subtraction, multiplication, and division:
signed int x = 10;
signed int y = -5;
signed int result = x + y; // result is 5
Important Note: Be cautious when performing calculations that can yield values outside the defined range of the data type, which may lead to overflow or underflow scenarios.
Type Promotion and Conversion
C++ handles type promotion implicitly when performing operations involving mixed data types. If you mix signed and unsigned integers in an expression, C++ promotes the signed integer to unsigned if the unsigned value can represent all potential values of the signed type. This can lead to unexpected results:
unsigned int u = 5;
signed int s = -10;
signed int result = u + s; // result type is signed, but u gets converted to unsigned, leading to unexpected large value.
To avoid such issues, always ensure that the types being combined are compatible or perform explicit type casting where necessary.
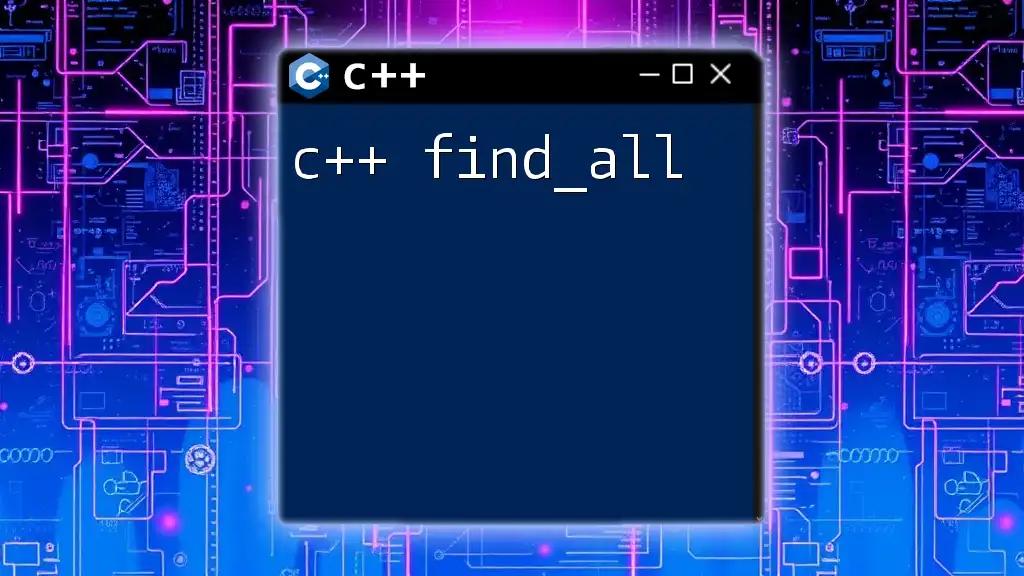
Common Issues with Signed Integers
Overflow and Underflow
Overflow occurs when an operation produces a value greater than the maximum representable value of the signed type, while underflow occurs when a negative result exceeds the lower limit. Here is an example demonstrating an overflow scenario:
signed int max = INT_MAX;
max += 1; // results in undefined behavior
When overflow occurs, it can lead to negative numbers if the maximum limit is exceeded, resulting in unpredictable behavior in your program. Always validate inputs and results when performing arithmetic with signed integers, particularly in scenarios where an overflow risk is present.
Best Practices
To manage the complexities of signed integers, consider the following best practices:
- Validate Inputs: Always check input values to ensure they fit within the allowable range of your signed types.
- Use Safe Mathematics Libraries: When performing complex mathematical operations, consider utilizing libraries that handle overflow and underflow gracefully.
- Avoid Mixing Types: Try to avoid mixing signed and unsigned types unless absolutely necessary to reduce the risk of unexpected behavior.
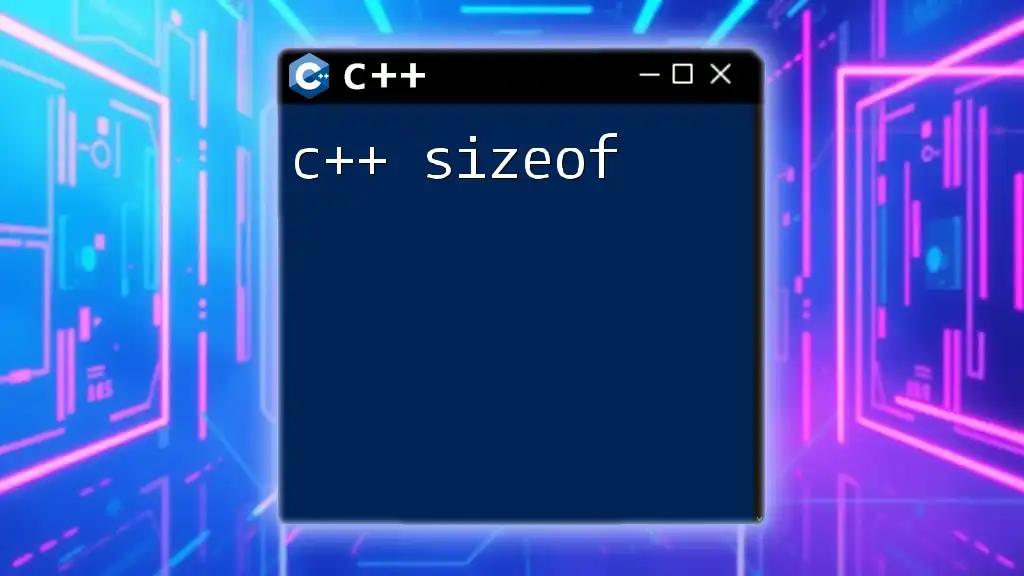
Conclusion
Understanding C++ signed data types is crucial for effective programming. By grasping how signed integers function, their ranges, and the potential issues that may arise, you can write smarter, safer code. As you continue to explore C++, remember to practice and apply your knowledge with signed integers in diverse programming scenarios.
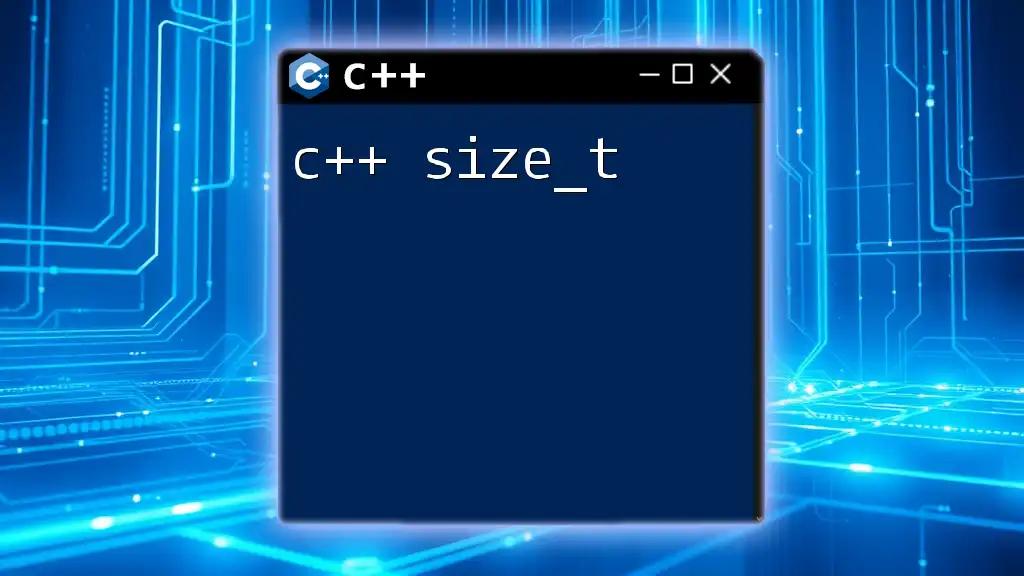
Additional Resources
To further deepen your knowledge, consider reviewing the C++ documentation on data types, suggested programming books, and engaging with community forums. These resources can help enhance your understanding of signed integers and their applications in real-world programming.
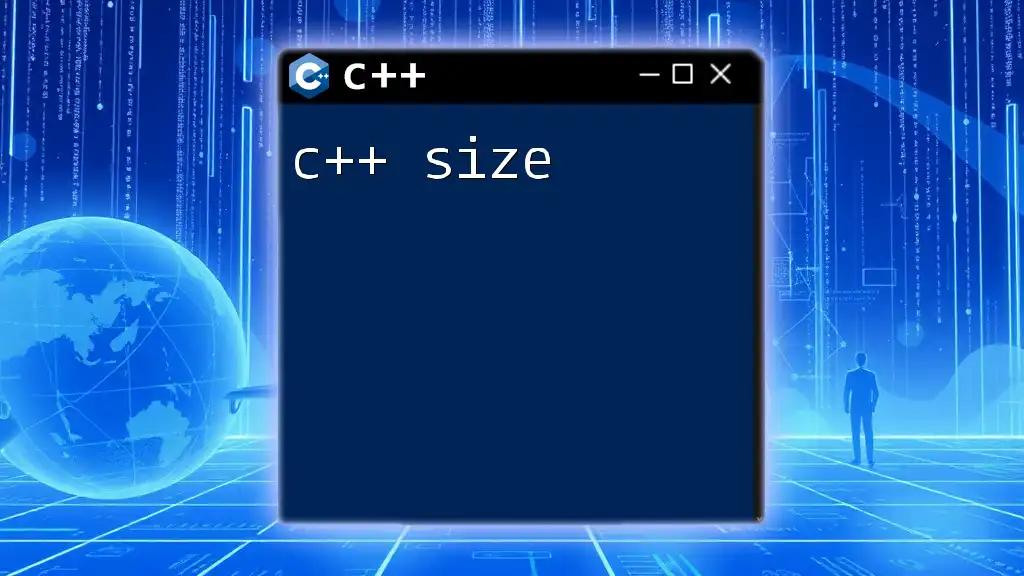
Call to Action
We would love to hear from you! Share your experiences and thoughts on using signed integers in your C++ projects. Engage with us through comments or by sharing this article on social media, helping others discover the significance of C++ signed data types.