C++ signal handling allows a program to respond to asynchronous events or interrupts, enabling developers to define custom behavior when specific signals are received.
#include <signal.h>
#include <iostream>
void signalHandler(int signum) {
std::cout << "Interrupt signal (" << signum << ") received.\n";
exit(signum);
}
int main() {
signal(SIGINT, signalHandler);
while (true) {
std::cout << "Program is running... Press Ctrl+C to exit.\n";
sleep(1);
}
return 0;
}
Understanding Signals in C++
What Are Signals?
In C++, signals are asynchronous notifications sent to a process in response to specific events. When a signal is delivered, the operating system notifies the program that an event has occurred, allowing it to proactively manage these occurrences. Signals can be classified into two types:
- Synchronous Signals: These signals occur as a direct result of the process’s actions, such as division by zero or invalid memory access.
- Asynchronous Signals: These signals occur independently of the program's control flow, such as a user pressing Ctrl+C (SIGINT) in the terminal.
Common Signals in C++
In C++, several signals are commonly encountered. Some of these include:
- SIGINT: Triggered when a user interrupts the process, typically by pressing Ctrl+C.
- SIGTERM: A termination signal sent to gracefully stop a process.
- SIGSEGV: Indicates that an invalid memory reference occurred, often due to segmentation faults.
Understanding these signals and their implications is essential for proper signal handling in your C++ applications.
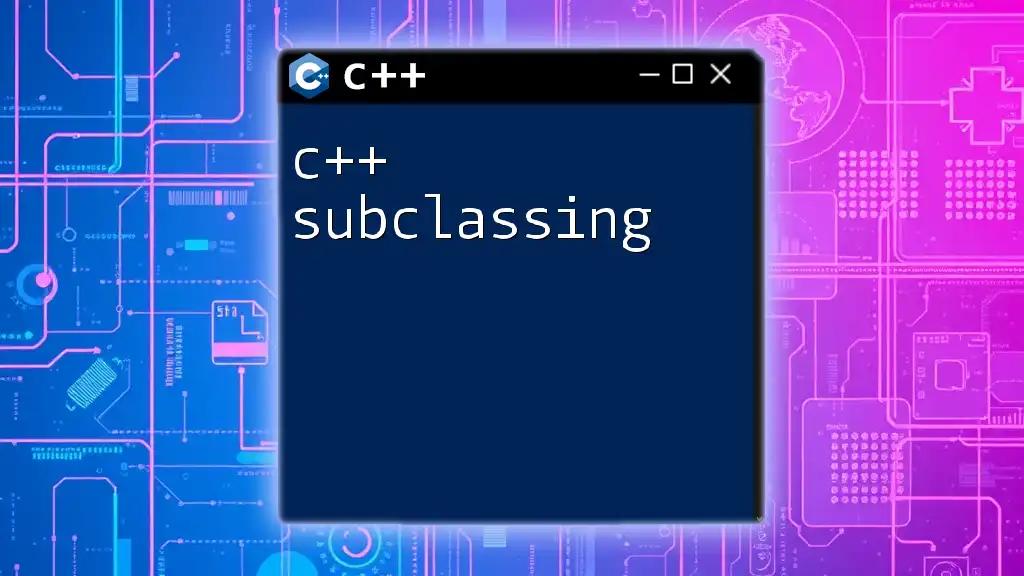
The C++ Signal Handler
What Is a Signal Handler?
A signal handler is a function in your program designed to handle specific signals. When a signal occurs, the associated signal handler is invoked, allowing the program to respond appropriately. This capability is crucial for maintaining control over program execution and gracefully managing unexpected events.
Structuring a C++ Signal Handler
To create a signal handler, you define a function that matches the expected signature. Here's an example of a simple signal handler:
#include <csignal>
#include <iostream>
void signalHandler(int signum) {
std::cout << "Interrupt signal (" << signum << ") received.\n";
exit(signum); // Exit the program after handling the signal
}
In this example, the `signalHandler` function will print a message indicating the received signal and exit the program when the signal occurs.
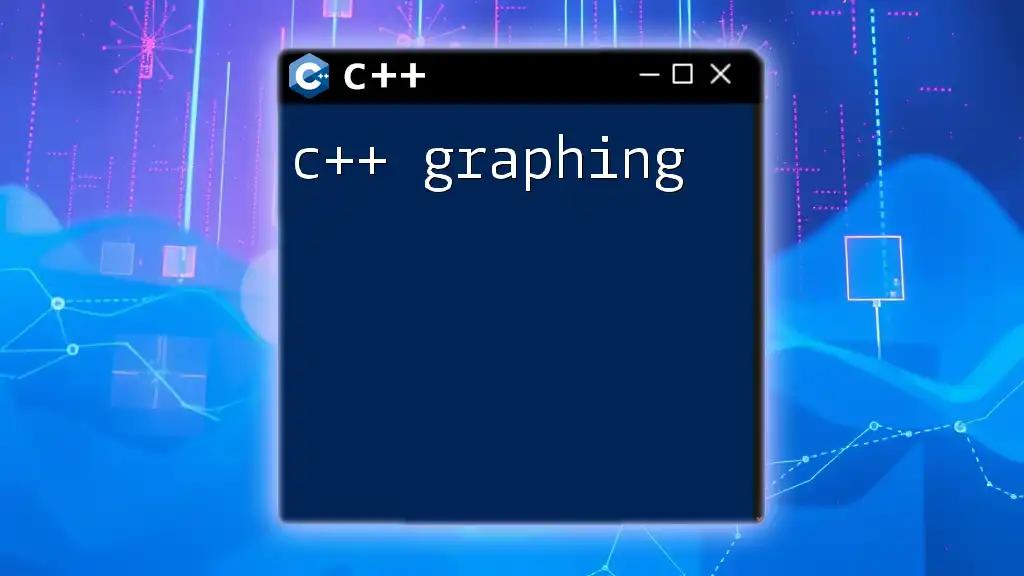
Setting Up a Signal Handler in C++
Installing a Signal Handler
To install a signal handler in C++, you typically use the `signal()` function. Here's a step-by-step process to do this:
-
Include the required header files: You need to include `<csignal>` for signal handling and `<iostream>` for input-output operations.
-
Define your signal handler function: As shown above.
-
Install the handler: Use `signal()` to associate your handler with a specific signal.
Here’s an example of setting up a signal handler for SIGINT:
int main() {
signal(SIGINT, signalHandler); // Install signal handler
while (true) {
std::cout << "Waiting for interrupt signal...\n";
sleep(1); // Pause to avoid busy waiting
}
return 0;
}
In this code, the application enters an infinite loop, waiting for the user to send an interrupt signal. When SIGINT is received, the `signalHandler` function is called.
Handling Multiple Signals
You can manage multiple signals by installing different handlers for each signal. For example:
signal(SIGTERM, signalHandler); // Handle termination
signal(SIGSEGV, signalHandler); // Handle segmentation fault
Here, the `signalHandler` function will respond to both SIGTERM and SIGSEGV, allowing your application to handle various signals with a single handler.
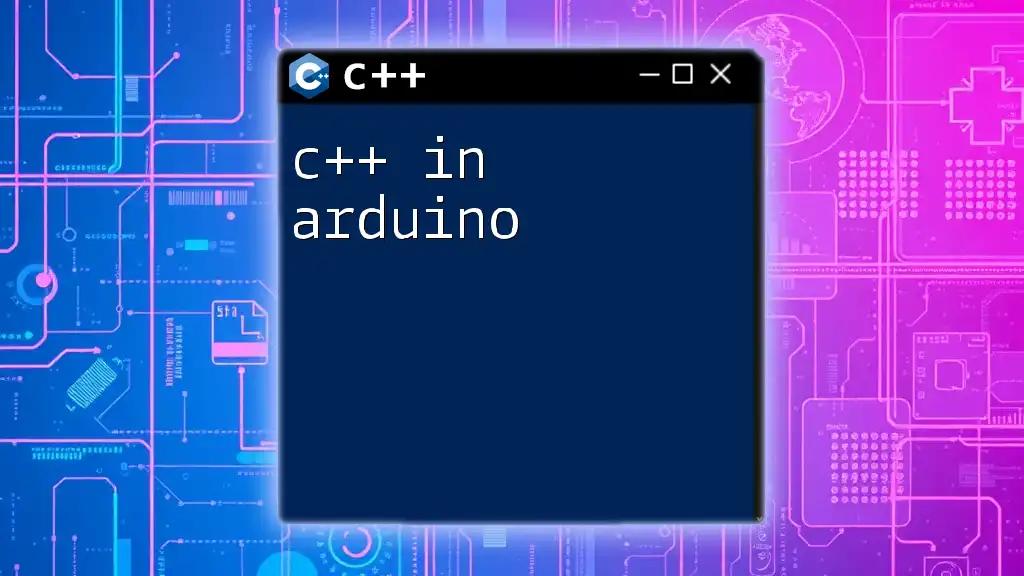
Advanced C++ Signal Handling
Using `sigaction` vs `signal`
While `signal()` is simple to use, it has limitations and undefined behavior in certain contexts. Consequently, the `sigaction()` function is often preferred for more robust signal handling.
The advantages of using `sigaction()` include:
- Fine-Grained Control: You can set flags for how the signal should behave.
- Portability: `sigaction()` is more consistent across platforms and avoids pitfalls associated with `signal()`.
Here's how to use `sigaction()` for signal handling:
#include <cstring>
#include <csignal>
void newSignalHandler(int signum) {
std::cerr << "Signal " << signum << " received.\n";
}
struct sigaction action;
action.sa_handler = newSignalHandler;
sigemptyset(&action.sa_mask);
action.sa_flags = 0;
sigaction(SIGINT, &action, nullptr); // Using sigaction for SIGINT
In this example, the `newSignalHandler` function will process SIGINT signals. By using `sigaction()`, you ensure your application handles the signal consistently.
Signal Masking
Signal masking allows you to block specific signals in a process. This is useful when you need to protect critical sections of your code from being interrupted.
Here’s how to set up signal masking:
sigset_t set;
sigemptyset(&set); // Clear the set
sigaddset(&set, SIGINT); // Add SIGINT to the set
sigprocmask(SIG_BLOCK, &set, nullptr); // Block SIGINT
With this code, SIGINT will be blocked until it is explicitly allowed again. Always be cautious when using signal masking, as it can lead to missed signals if not managed correctly.
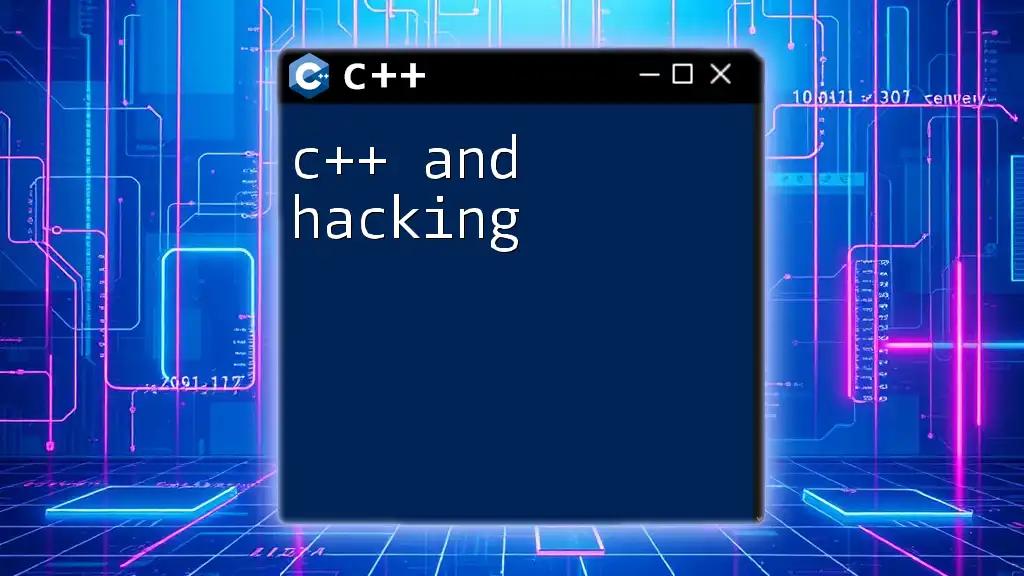
Best Practices for C++ Signal Handling
When implementing C++ signal handling, consider the following best practices:
- Keep Handlers Simple: Signal handlers should complete their operations quickly to prevent deadlocks and performance issues.
- Avoid Complex Logic: Complex operations, like memory allocations or I/O operations, can lead to undefined behavior if called from a signal handler.
- Use `sigaction` for Robustness: Prefer `sigaction()` over `signal()` for better control and reliability.
- Clean Up Resources: If your signal handler modifies shared resources, ensure proper cleanup to avoid resource leaks or corruption.
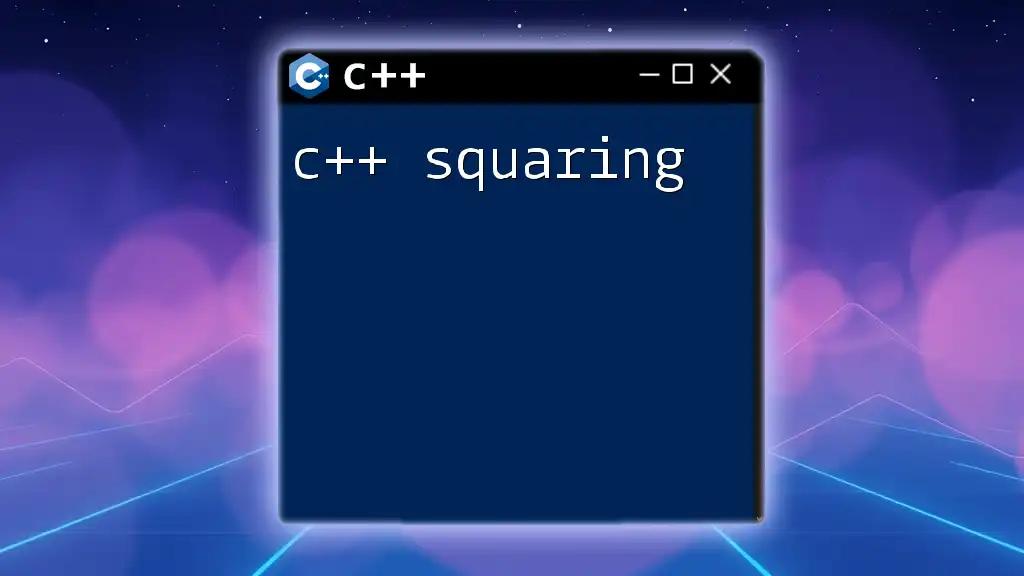
Debugging Signal Handlers
Common Issues and Errors
Debugging signals can be tricky. Here are common issues you might encounter:
- Reentrancy: If a signal interrupts your program in the middle of a critical operation, the signal handler can cause unexpected behavior.
- Lost Signals: If signals are masked or too many signals are sent in quick succession, some may be lost.
Tools for Debugging
To effectively debug your signal handling code, you can leverage tools like gdb (GNU Debugger). Here’s how you can trace signals during execution:
- Compile your C++ code with debugging symbols (use the `-g` flag).
- Run your application in gdb. You can set breakpoints on signal handlers using:
(gdb) break signalHandler
- Monitor signals with:
This command will let you see when signals are received without interfering with execution.(gdb) handle SIGINT noprint

Conclusion
Mastering C++ signal handling is essential for developing robust applications that can manage unexpected events. Understanding the mechanisms behind signals, installing signal handlers efficiently, adhering to best practices, and using debugging tools effectively will empower you to create resilient programs. With hands-on practice and exploration of advanced techniques, you can harness the full potential of C++ signal handling in your projects.
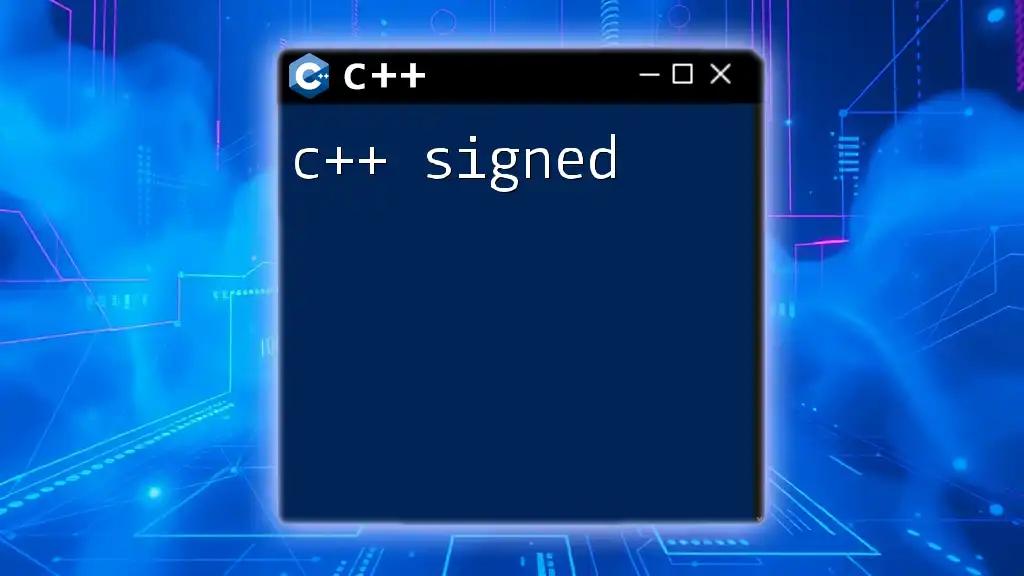
Additional Resources
For further learning, visit the following references:
- C++ Standard Library Documentation
- Advanced C++ Tutorials
- Books on Operating Systems and Signal Processing
These resources will provide deeper insights into advanced topics and signal handling best practices. Happy coding!