In C++, "deducing this" allows member functions to infer the type of `this` pointer in generic lambdas or templates, improving code flexibility and type safety.
#include <iostream>
template <typename T>
void printThis(T&& obj) {
std::cout << "The type of this is: " << typeid(obj).name() << std::endl;
}
struct MyClass {
void display() {
printThis(*this); // Deduces the type of this
}
};
int main() {
MyClass myObj;
myObj.display(); // Output will show the deduced type
return 0;
}
Understanding `this` Pointer
What is `this`?
In C++, the `this` pointer is a special pointer that is automatically passed to all non-static member functions of a class. It serves to provide a reference to the calling object itself. Every non-static member function has an implicit parameter of type `ClassName*` (where `ClassName` is the name of the class), which allows you to differentiate member variables from parameters when they have the same names.
How `this` Works in C++
When you call a member function on an object, `this` is automatically set to point to that object. This means that within any non-static member function, you can access the object's members and methods using `this` without explicitly passing it. To illustrate:
class Example {
public:
int value;
void setValue(int value) {
this->value = value; // 'this->value' refers to the member variable
}
};
In the above snippet, `this->value` clearly indicates that we are referring to the member variable `value`, while the parameter `value` is used to pass the value into the function.
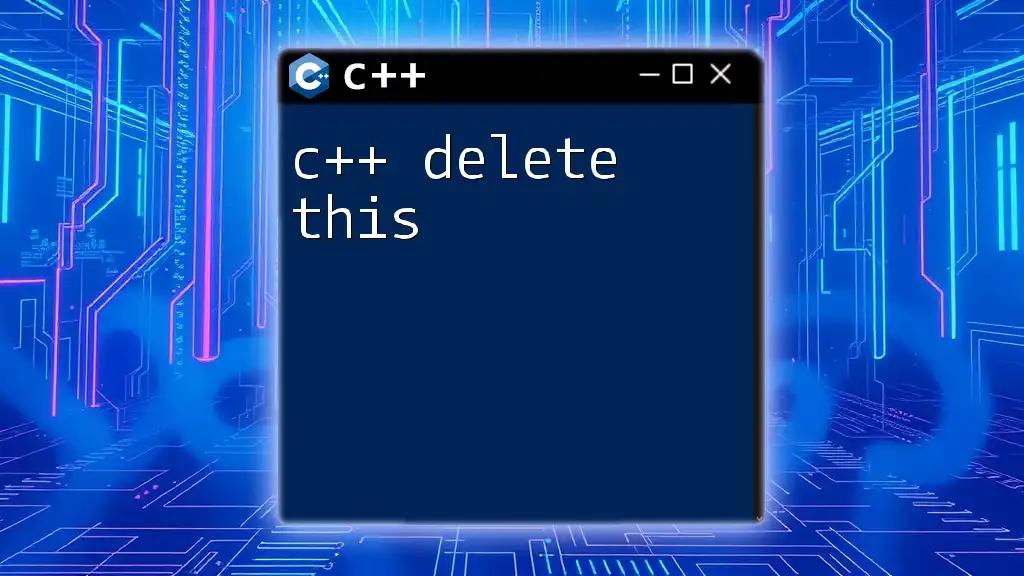
The Concept of Deducing `this`
What Does Deducing `this` Mean?
Deducing `this` refers to the ability of C++ to determine the type of `this` automatically based on the context of the member function. This becomes particularly useful in stages of template programming where methods may apply to different types of objects, allowing for a more generic approach without losing type specificity.
How Deducing `this` Enhances Code Clarity
Utilizing a deduced `this` can simplify member function implementations. Instead of explicitly writing `this->`, you can access the member variables directly if there are no naming conflicts. For example:
class Demo {
public:
int value;
void setValue(int v) {
value = v; // Deducing 'this', avoids 'this->value'
}
};
In this case, the compiler automatically infers that `value` references the member variable when there is no local variable named `value`. Keeping your code concise makes it easier to read and maintain.
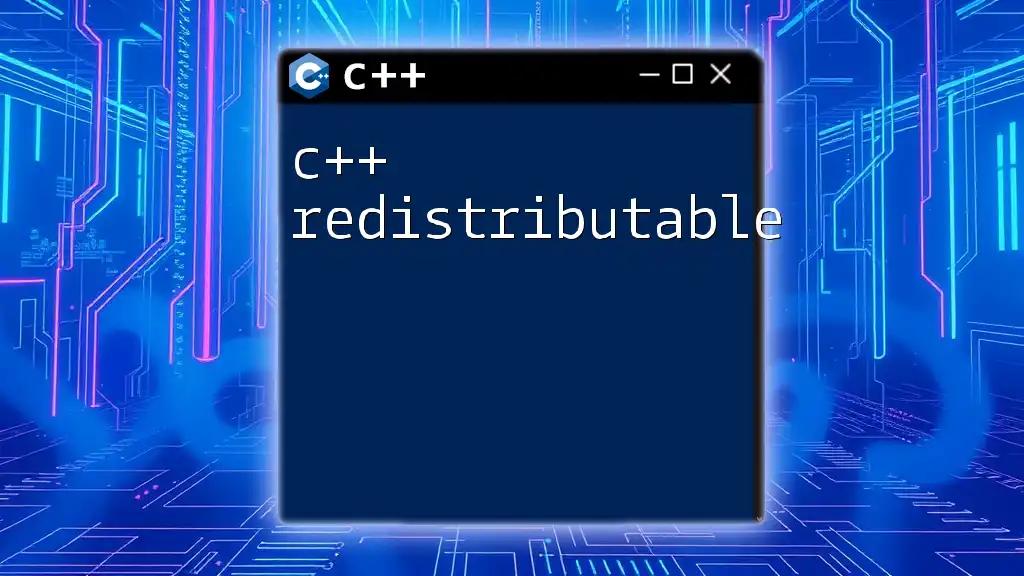
Deducing `this` in Member Functions
Syntax Overview
The use of `this` in member functions follows a straightforward syntax. Consider the code snippet:
class Sample {
public:
void show() {
std::cout << this << std::endl; // Using this to print the memory address
}
};
This example demonstrates how to utilize `this` to access properties of the object. In this case, we print the memory address of the object calling `show()`.
Importance of `const` and `&` with `this`
When dealing with `const` member functions, the deduced `this` is of type `const ClassName*`. This prohibits any modification of the object's state. For instance:
class Sample {
public:
void show() const {
std::cout << this << std::endl; // This is automatically treated as 'const Sample*'
}
};
Understanding this concept is crucial when designing APIs that require certain functions to guarantee immutability.
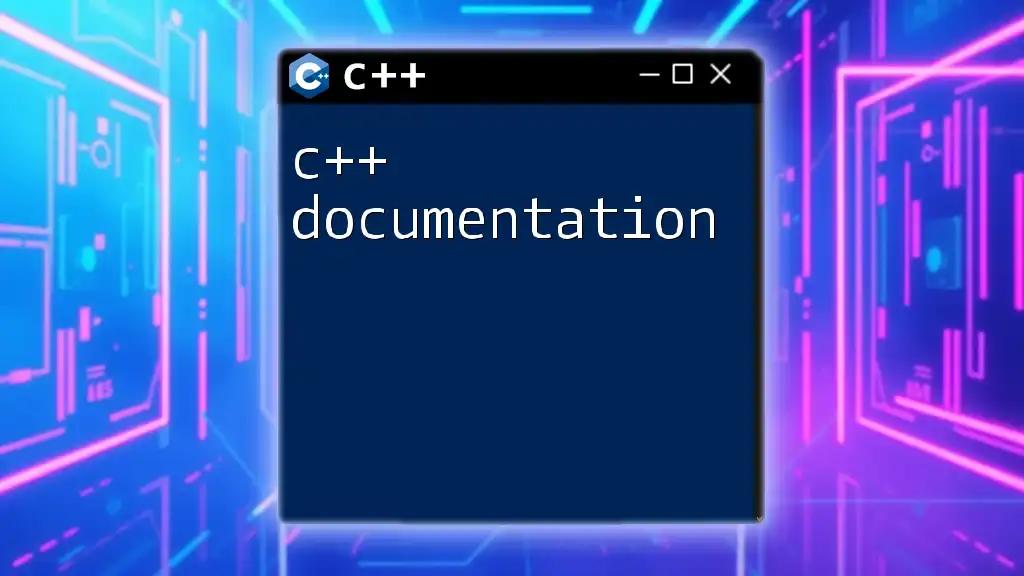
Deducing `this` in Templates
Utilizing `this` in Template Classes
In templates, C++ allows you to deduce `this` for any instantiated class. Example:
template<typename T>
class Wrapper {
public:
void display() {
std::cout << this << std::endl; // Deducing 'this' in a template class
}
};
This approach allows you to define behavior that can work with a variety of types while still benefiting from the clarity provided by deducing `this`.
Special Cases with Template Type Deduction
Type deduction rules can lead to confusion in certain situations. For example, if you reference `this` in a templated member function with complex types, ensure that the type deduced aligns with your expectations to avoid compilation errors.
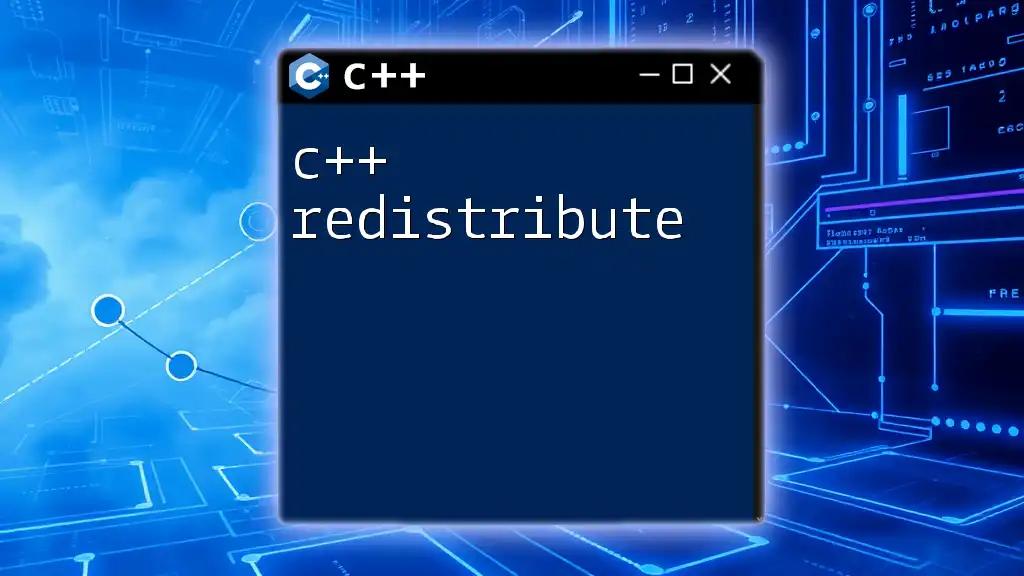
Special Member Functions and `this`
Constructors and `this`
During object construction, the `this` pointer is initialized to point to the newly created object. This allows the constructor to reference members directly:
class Sample {
public:
int value;
Sample(int value) {
this->value = value; // Differentiates constructor’s parameter
}
};
Here, using `this` is essential to resolving ambiguity between the constructor parameter and the member variable.
Operator Overloading and `this`
When overloading operators, the `this` pointer is critical for managing the context of what is being operated on. For instance:
class Sample {
public:
int value;
Sample operator+(const Sample& other) {
Sample result;
result.value = this->value + other.value; // Using this for the member variable
return result;
}
};
In operator overloads, `this` serves to clearly express which object’s members are being utilized, leading to fewer bugs and increased readability.
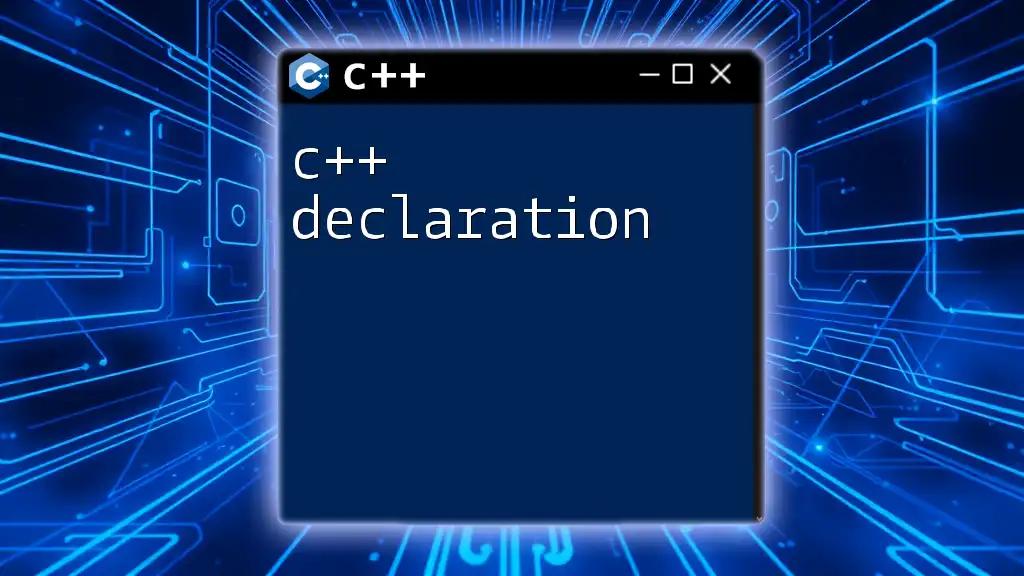
Practical Applications of Deducing `this`
Method Chaining
One practical application of deducing `this` is in method chaining, where returning `*this` allows for multiple method calls in a single expression. Here's an example:
class Chainable {
public:
Chainable& setValue(int value) {
this->value = value;
return *this; // Returning current object for chaining
}
int getValue() const {
return value;
}
private:
int value;
};
With method chaining, you can elegantly streamline operations on objects, greatly enhancing the usability of your APIs.
Fluent Interfaces Using `this`
Fluent interfaces thrive on the concept of `this`, allowing for calls to be made in a clear and concise way. This approach can lead to more readable code. For example:
class Fluent {
public:
Fluent& add(int num) {
this->value += num;
return *this;
}
Fluent& subtract(int num) {
this->value -= num;
return *this;
}
int getValue() const {
return value;
}
private:
int value = 0;
};
Such designs facilitate an effective coding style, providing a natural language-like quality to the interaction with objects.
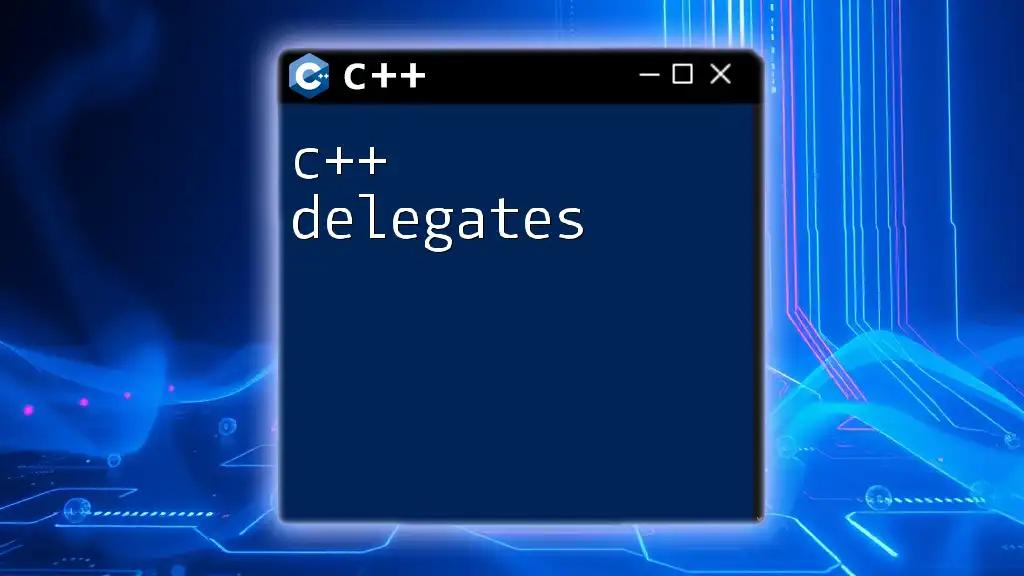
Common Mistakes When Using `this`
Forgetting About Static Members
A common pitfall is misunderstanding that `this` is not available within static member functions. Static functions do not operate on instances of the class, so they cannot use `this`.
class Sample {
public:
static void someStaticFunction() {
// Error: 'this' cannot be used here
}
};
This distinction is crucial for proper class design and avoiding logical errors.
Misusing `this` with Implicit Conversions
Implicit conversions can cause unexpected behavior if `this` is used in scenarios where type conversion occurs. Pay careful attention to how `this` may infer types, as this could lead to subtle bugs in your codebase.
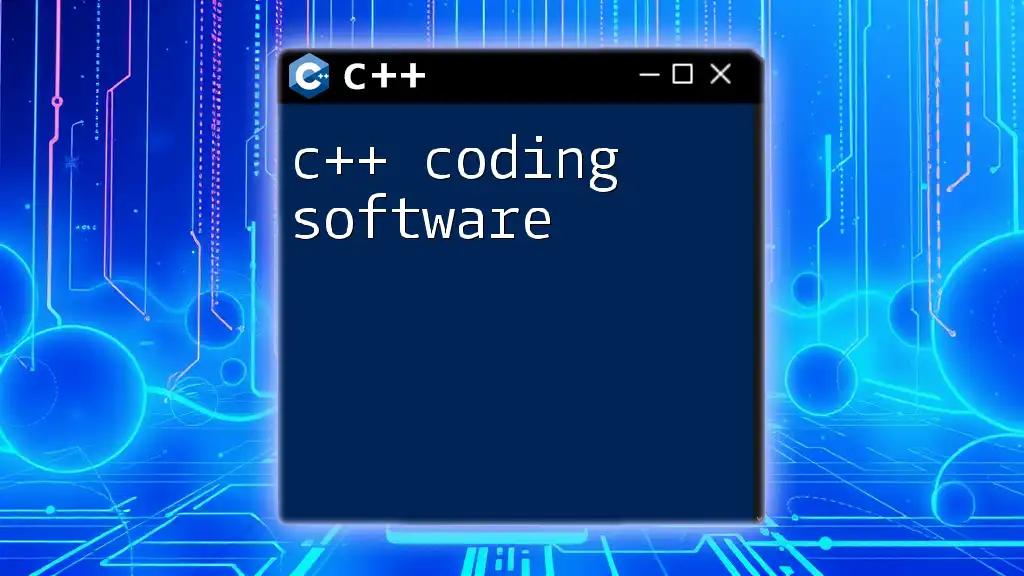
Conclusion
The concept of c++ deducing this is pivotal in managing object-oriented programming in C++. By understanding how `this` operates and embracing its deduced nature in various scenarios, developers can write clearer, more maintainable code. As you continue to practice and produce C++ code, remember the significance of using `this` effectively, and leverage its potential for cleaner design.
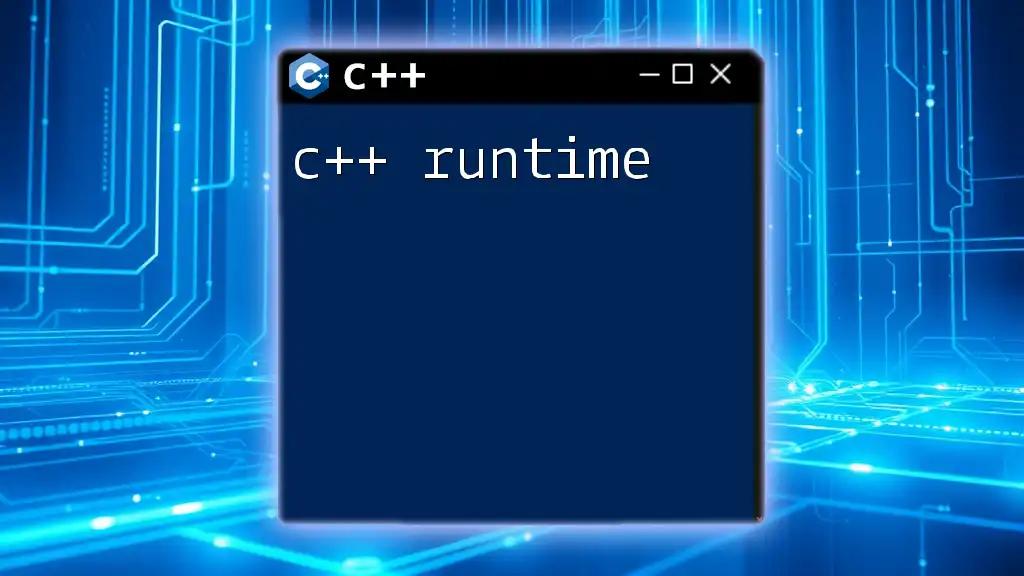
Additional Resources
- For further reading, refer to the official C++ documentation and advanced resources available online.
- Explore recommended books and online courses that provide in-depth coverage of C++ concepts, including object-oriented programming and template metaprogramming.

FAQs
What Happens If You Don’t Use `this`?
If you neglect to use `this`, you may encounter ambiguity in variable scoping, particularly when class member variables share names with function parameters. As a result, the compiler may use the parameter instead of the intended member variable.
When Should You Use Explicit `this`?
Using explicit `this` is particularly beneficial when dealing with naming conflicts or when you want to enhance code clarity, making your intentions explicit to readers and maintainers of your code.