The `std` namespace in C++ allows you to access standard library features, such as input/output and containers, simplifying code and avoiding potential naming conflicts.
Here’s a simple example demonstrating how to use `std` for input and output:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is the Standard Namespace in C++?
In C++, a namespace serves as a declarative region that groups together logical entities, such as classes, functions, and variables, to prevent name conflicts. The std namespace is the standard namespace and contains all the classes, functions, and objects that are part of the C++ Standard Library.
Using the std namespace allows you to access essential components such as input/output streams (`cout`, `cin`), standard containers (`vector`, `list`), algorithms, and more. By utilizing the standard namespace, programmers take advantage of the features and functionalities provided by the C++ standard library to write efficient and portable code.
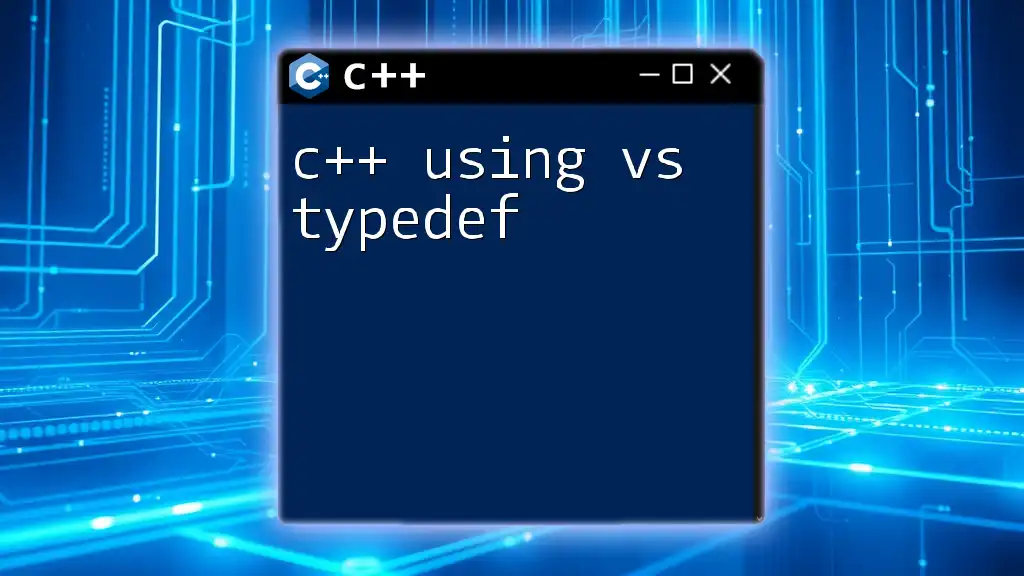
Why Use the std Namespace?
Using the std namespace provides several advantages:
-
Reduced Typing Effort: By declaring `using namespace std;`, you eliminate the need to prefix standard library functions and objects with `std::` every time, which simplifies and shortens your code significantly.
-
Improved Readability: Cleaner code enhances readability and understanding. When you use `cout` instead of `std::cout`, it becomes clear that the focus is on the command as opposed to the namespace.
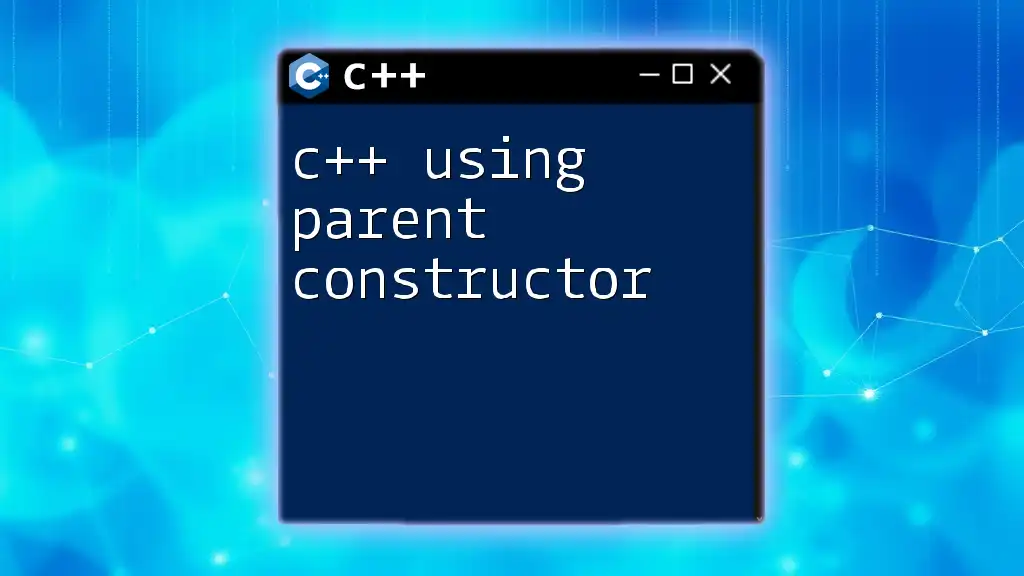
Using Namespace std in C++
Understanding `using namespace std`
When you include the line `using namespace std;` at the beginning of your program, you inform the compiler that you want to use the identifiers from the std namespace directly. This means you can write code without the `std::` prefix for standard library components.
Here is a simple code example illustrating the use of `using namespace std;`:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, thanks to `using namespace std;`, you can use `cout` and `endl` without needing to write `std::cout` and `std::endl`.
When to Use `using namespace std`
The use of `using namespace std;` can be particularly beneficial in specific scenarios:
-
Small Programs: For short, simple programs or during learning exercises, the convenience of omitting `std::` can keep your code unobtrusive and easy to read.
-
Educational Purposes: In instructional materials where clarity is essential, using `using namespace std;` can streamline examples.
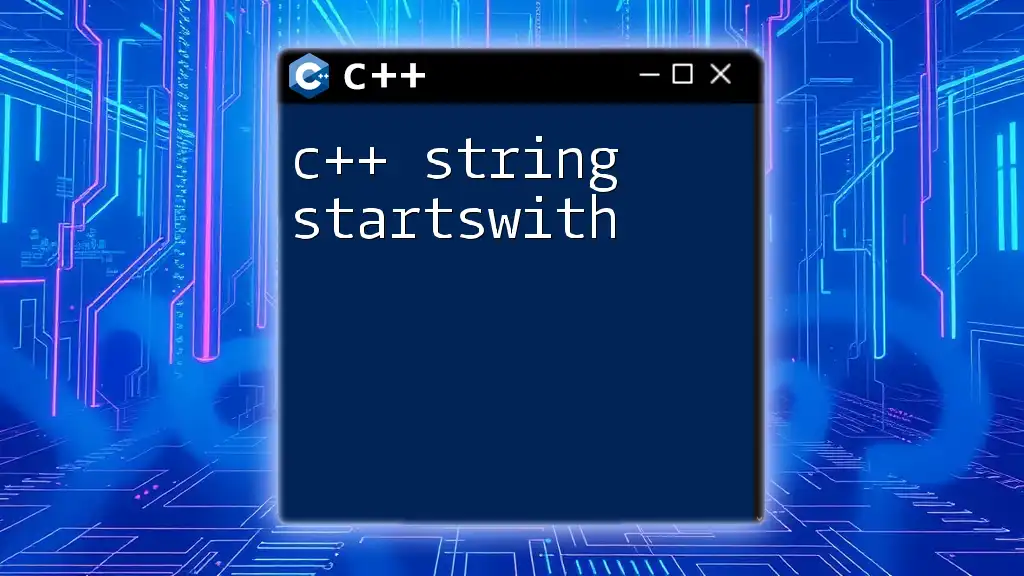
Risks of Using `using namespace std`
Potential Name Conflicts
One significant downside of using `using namespace std;` is the potential for name conflicts. When you introduce a namespace into your code, it can clash with the identifiers defined in your code or in other libraries.
For example, consider the following code snippet:
#include <iostream>
using namespace std;
void print() {
cout << "Standard print" << endl;
}
void print(int value) {
std::cout << "Value: " << value << endl;
}
// This causes ambiguity if not qualified
In this situation, the function `print()` can lead to confusion about which `print` is being referenced, especially if you include additional libraries or definitions with similar names.
Alternatives to `using namespace std`
To avoid conflicts, especially in larger projects, developers often prefer alternatives to using `using namespace std;`. Here are a few strategies:
-
Selective Usage: Instead of importing the entire namespace, you can choose specific components to use, such as:
using std::cout; using std::cin;
This helps you minimize the risk of encountering naming conflicts.
-
Qualified Names: Another approach is to use fully qualified names, such as:
std::cout << "This is a test." << std::endl;
This explicitly indicates where the function or object comes from, thereby avoiding any ambiguity.
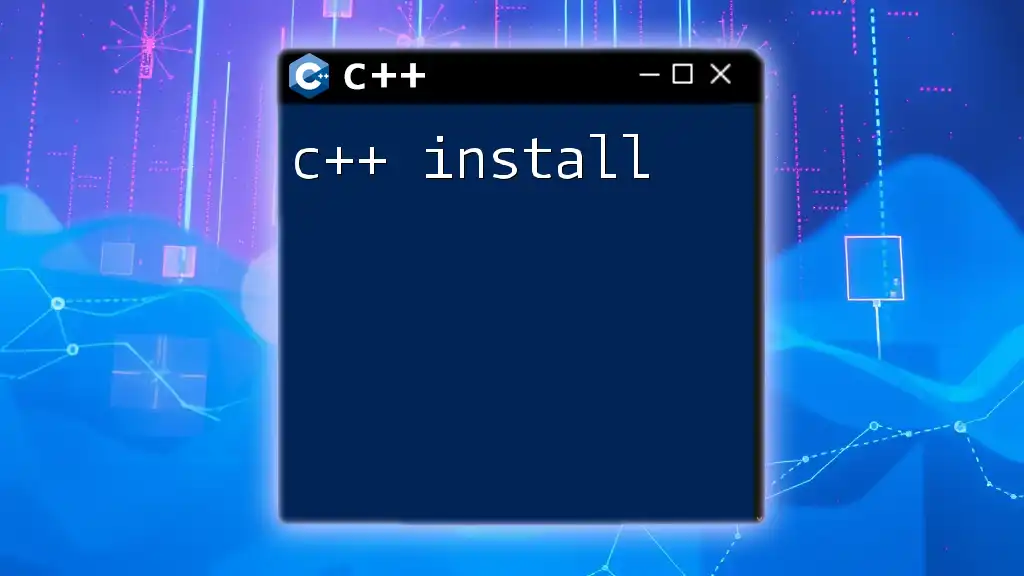
Summary of C++ Namespace std Usage Patterns
Best Practices for Beginners
For those new to programming in C++, it's recommended to use `using namespace std;` sparingly. When you are just starting, it might make your learning experience smoother and simpler. However, as you gain experience, becoming familiar with the namespace concept and its implications will help you write more robust code.
Intermediate to Advanced Use
For more seasoned developers, understanding the balance between convenience and potential pitfalls is critical. Often, in larger codebases or in professional environments, the best approach is to be precise in your declarations. This reduces clutter and minimizes the chances of running into naming conflicts, thereby fostering better coding practices.
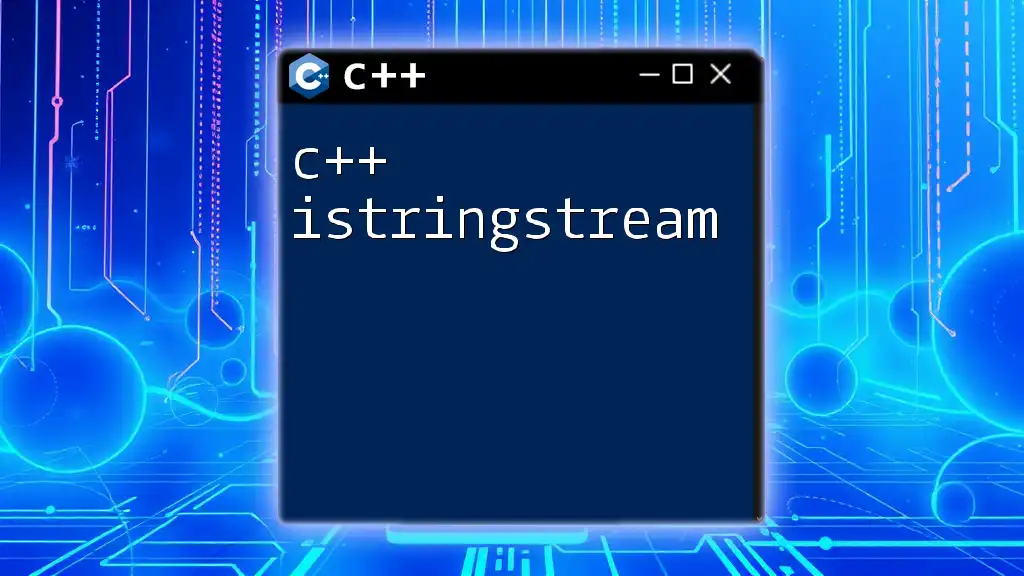
Conclusion
The std namespace is a fundamental aspect of C++ that the programming community actively leverages to take advantage of the powerful functionalities offered within the Standard Library. Knowing how to effectively utilize `using namespace std;` while being aware of its implications will improve your coding experience and help you maintain cleaner, more maintainable code. As you continue your journey with C++, understanding namespaces will enhance your programming skills and set you apart as a developer.
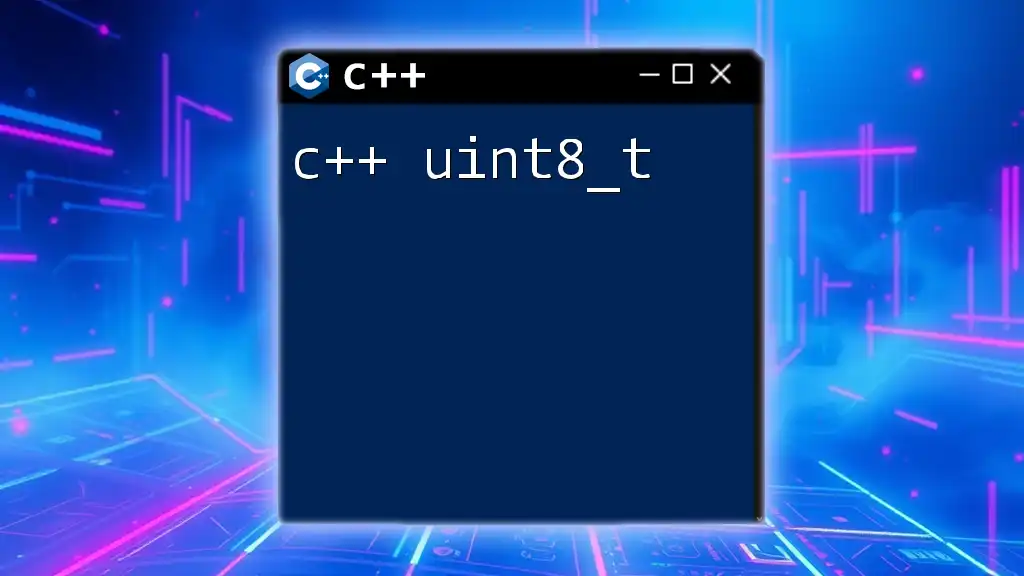
Resources for Further Learning
For further insights, consider exploring C++ references, reputable coding platforms, and comprehensive guides that cater to users at varying skill levels. Engaging with community forums or enrolling in programming courses can expand your knowledge on this crucial topic further.
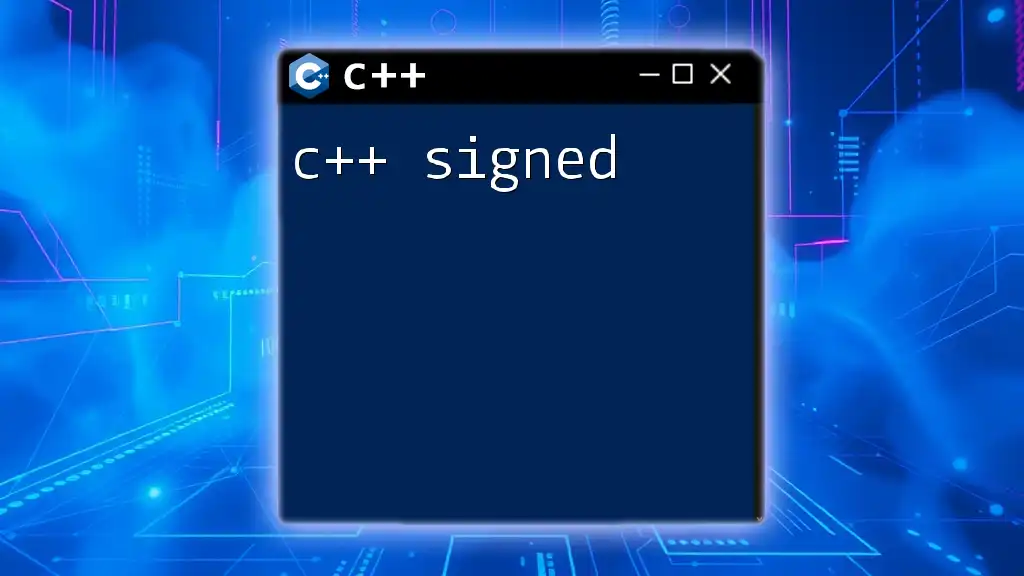
Call to Action
Now, it’s time to practice your C++ skills using std. Experiment with different components of the standard library, and don’t hesitate to share your discoveries and questions in forums. If you are eager for more structured guidance, consider signing up for our courses to deepen your understanding of C++ commands and best practices!