The `uint8_t` type in C++ is an unsigned integer data type that occupies 8 bits (1 byte) of memory, which can store values ranging from 0 to 255.
Here’s a simple code snippet demonstrating its use:
#include <cstdint>
#include <iostream>
int main() {
uint8_t myNumber = 200; // Initialize a uint8_t variable
std::cout << "The value of myNumber is: " << static_cast<int>(myNumber) << std::endl; // Cast to int for proper display
return 0;
}
Understanding Fixed-Width Integer Types
What are Fixed-Width Integers?
Fixed-width integers are types of integer data that have a defined size across all platforms. In contrast to standard integer types like `int` and `long`, which can vary in size depending on the architecture, fixed-width integers guarantee that the number of bits used to represent the integer is constant.
For instance, a `uint8_t` will always use 8 bits. This uniformity is essential in system-level programming and scenarios where precise control over memory usage is required.
Benefits of Using Fixed-Width Integer Types
Utilizing fixed-width integer types such as `uint8_t` comes with numerous benefits:
-
Portability: Programs that use fixed-width types are less likely to break when compiled on different platforms because the size remains consistent. This ensures that data handling behaves the same way regardless of where the code is executed.
-
Data Integrity: Fixed-width types can prevent bugs related to integer overflow or sign errors that often occur when using standard integer types. By explicitly defining the integer width, you can handle data more predictably.
Where Used: Common Scenarios for uint8_t
The `uint8_t` type often finds itself in various domains:
-
Embedded Programming: In environments where memory is scarce, using a `uint8_t` allows developers to optimize their applications by storing data in exactly the amount of space needed.
-
Network Protocols: Many communication protocols specify the size of data packets, and `uint8_t` serves as an ideal way to define byte-oriented data structures.
-
Graphics Programming: In situations where color values (e.g., RGB) are represented in bytes (0–255), leveraging `uint8_t` simplifies representation and manipulation.
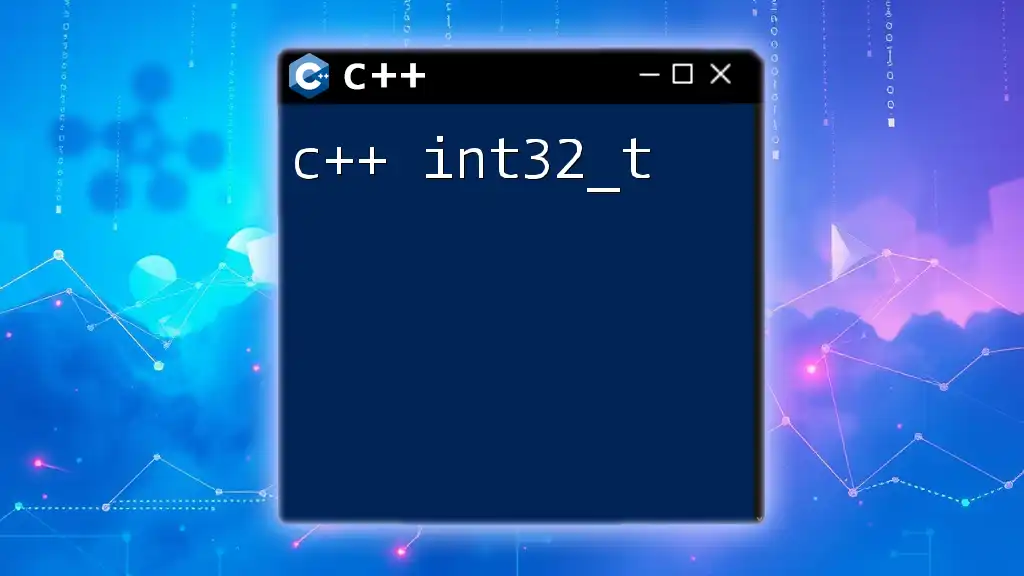
Defining uint8_t
Declaration of uint8_t
To begin using `uint8_t` in your C++ programs, you must include the relevant header file. This can be achieved by adding the following line to your code:
#include <cstdint>
Once included, you can declare a `uint8_t` variable straightforwardly.
uint8_t myVariable = 255; // a valid initialization
Characteristics of uint8_t
The `uint8_t` type is characterized by its size—8 bits or 1 byte—and it supports values in the range from 0 to 255. Understanding the implications of this range is vital, as it means that exceeding this limit can result in unexpected behaviors, such as overflow.
When performing operations that can push the value beyond the maximum, it's essential to be cautious:
uint8_t overflowExample = 250;
overflowExample += 10; // This line causes overflow since it exceeds 255.
This overflow can lead to unintended consequences and bugs if unchecked.
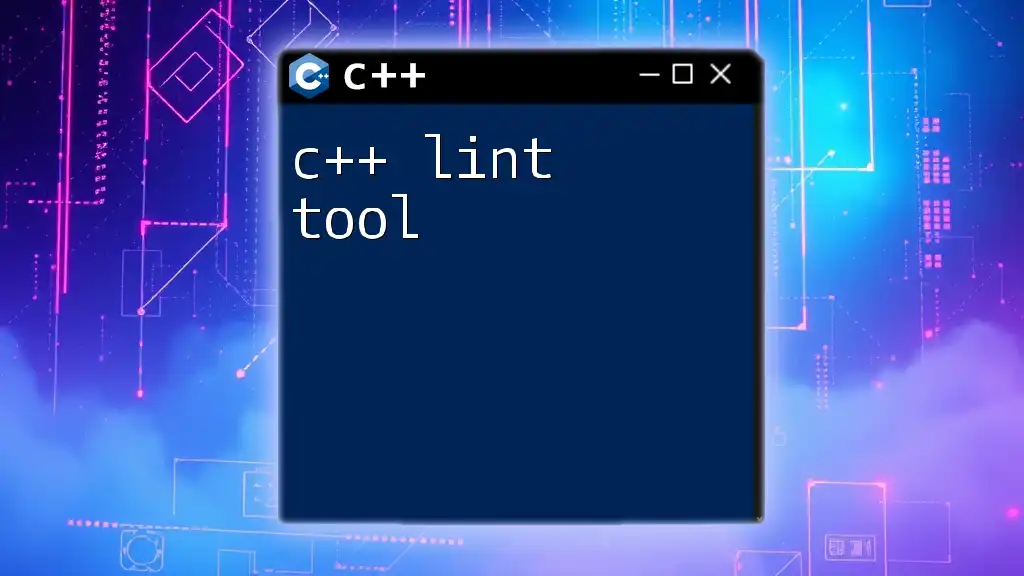
Using uint8_t in C++
Assignment and Initialization
Assigning values to `uint8_t` is straightforward, but care is needed to avoid exceeding its value range. Here’s a simple example:
uint8_t validAssignment = 100; // Within the range
uint8_t invalidAssignment = 300; // This will lead to overflow!
Remember that assigning a value greater than 255 may result in overflow that wraps around, making the value appear as unexpected.
Performing Arithmetic Operations
Arithmetic operations on `uint8_t` are similar to those on other integer types, but keep in mind type promotion and implicit conversions that may occur. Here’s how to perform basic arithmetic with `uint8_t`:
uint8_t num1 = 100;
uint8_t num2 = 50;
uint8_t sum = num1 + num2; // Result will be 150
However, if you add two maximum possible values:
uint8_t num3 = 128;
uint8_t num4 = 128;
uint8_t result = num3 + num4; // This may result in an overflow
In this case, the value would wrap around, causing issues if you expect the result to be within `uint8_t` limits.
Handling Overflow and Underflow
Overflow occurs when an operation exceeds the maximum value a type can hold, while underflow occurs when a type's minimum value is surpassed. Understanding these concepts is crucial when working with `uint8_t`.
For instance:
uint8_t underflowExample = 0;
underflowExample -= 1; // Underflow happens here, wrapping to 255
It’s best practice to implement checks when performing arithmetic to avoid such situations, especially in applications that demand high reliability.
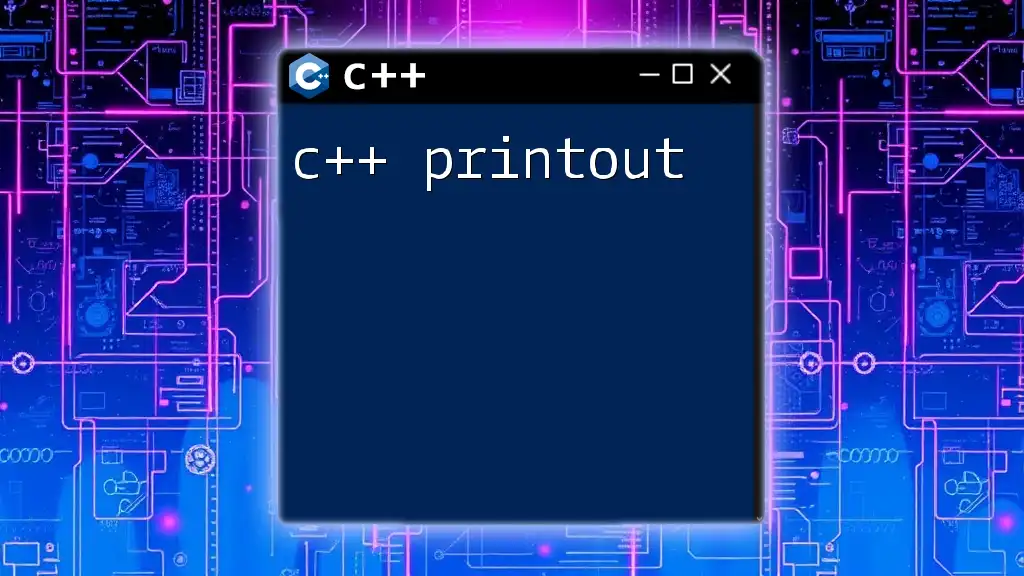
Best Practices for Using uint8_t
Choosing the Right Type for Your Needs
`uint8_t` is an ideal choice when you are sure your values will remain in the range of 0 to 255. For example, it's a great fit for color values or small integer counters. However, when you expect values beyond this range, consider larger types like `uint16_t` or `uint32_t`.
Avoiding Common Mistakes
One common mistake developers often face is failing to account for overflow and underflow. Always ensure your operations are within the safe limits of `uint8_t` and use conditional statements to verify your values when necessary.
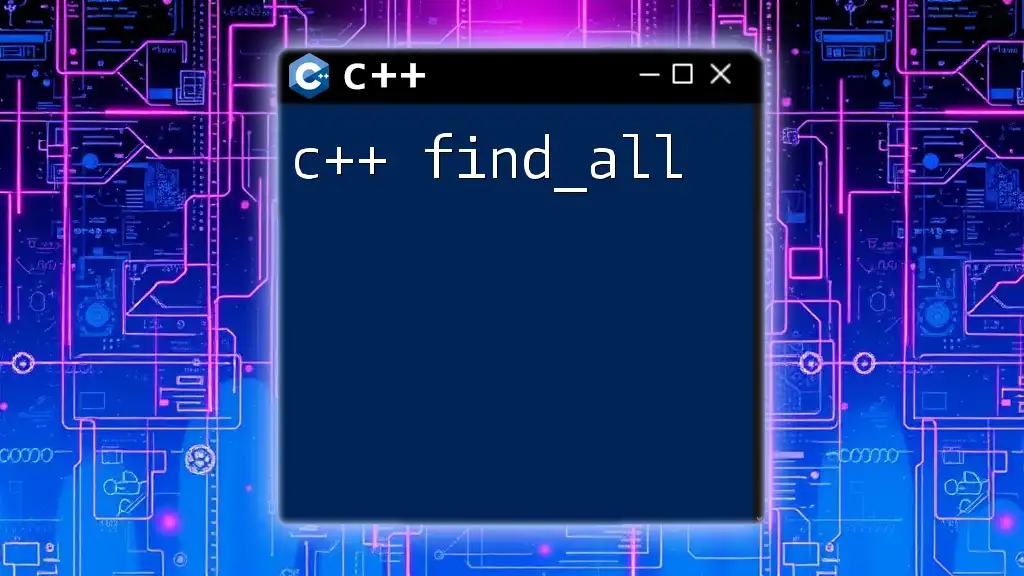
Performance Considerations
Memory Usage and Efficiency
When memory efficiency is a concern, especially in embedded systems, `uint8_t` helps maximize available space. Each `uint8_t` variable occupies precisely one byte, unlike larger integer types that consume more memory.
Compiler Optimizations
Modern compilers are adept at optimizing fixed-width types for performance, often resulting in highly efficient code. Optional compiler flags may also further enhance this performance, particularly in tight loops or computation-heavy operations.
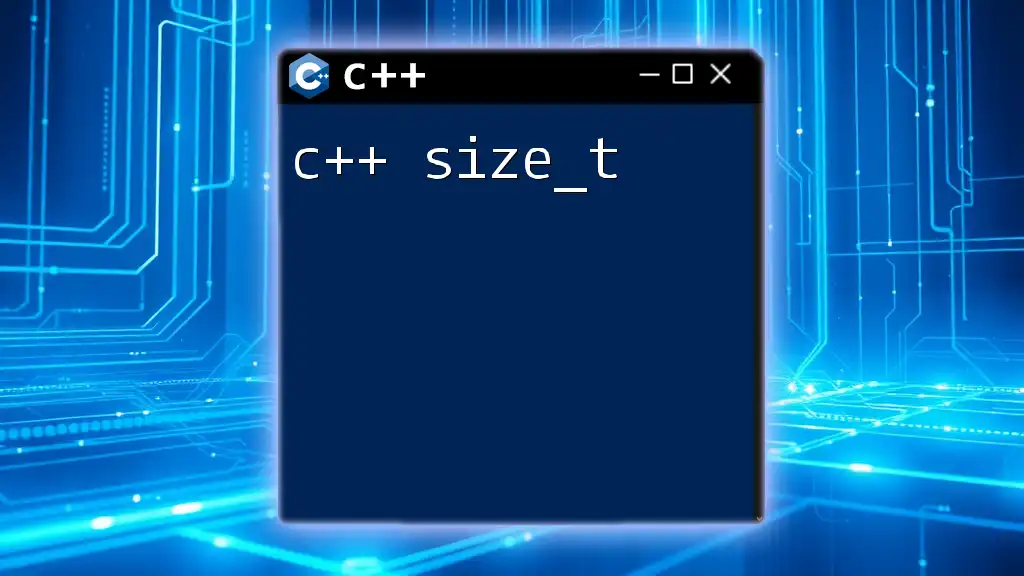
Conclusion
In conclusion, c++ uint8_t is a powerful tool within the C++ programming language, offering developers the ability to manage data precision and memory efficiency effectively. Its characteristics make it ideal for specific applications, supporting precise control over byte-level representations. As you continue your journey in C++, don't hesitate to leverage `uint8_t` when appropriate.
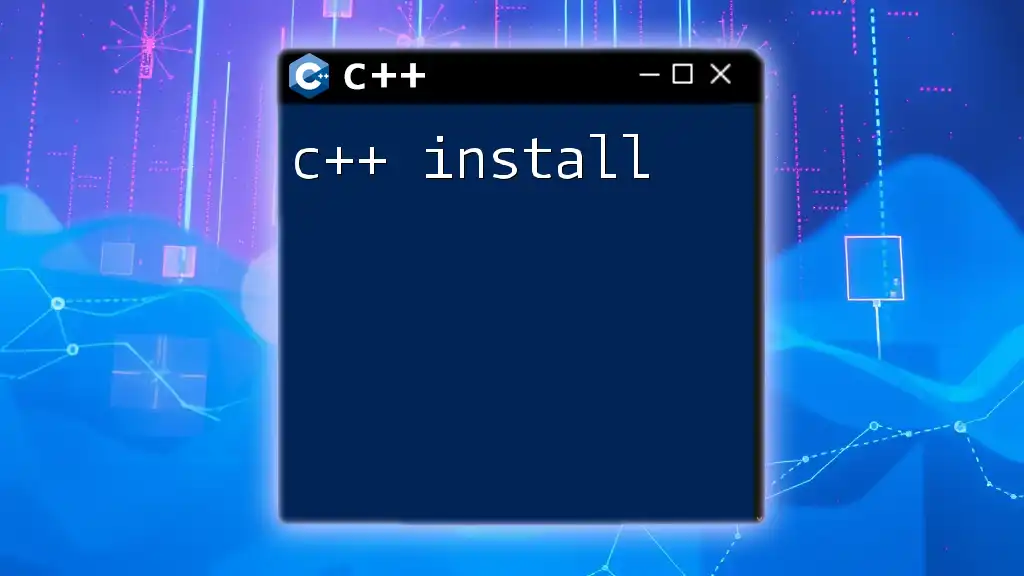
Additional Resources
To further expand your understanding of C++ and fixed-width integer types like `uint8_t`, consider exploring comprehensive books on C++ programming, reputable online courses, and official documentation. These resources can provide deeper insights and real-world examples, enhancing your programming skills.
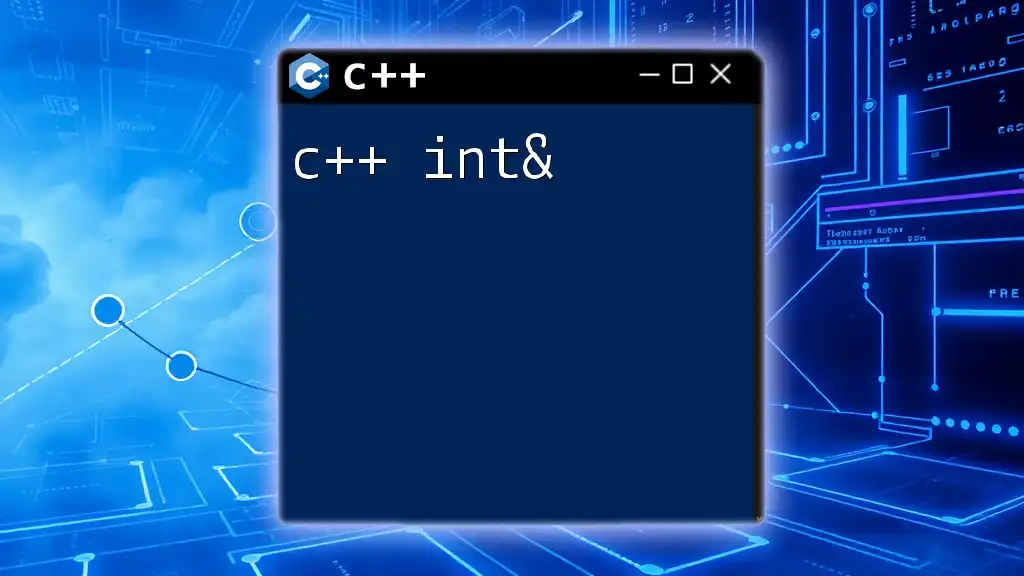
FAQs About uint8_t
Common questions about `uint8_t` often revolve around its limitations and best practices. For instance, developers might inquire about alternatives for larger ranges or how to effectively manage and protect against overflow. Engaging with community forums and experienced developers can also offer valuable perspectives and solutions.
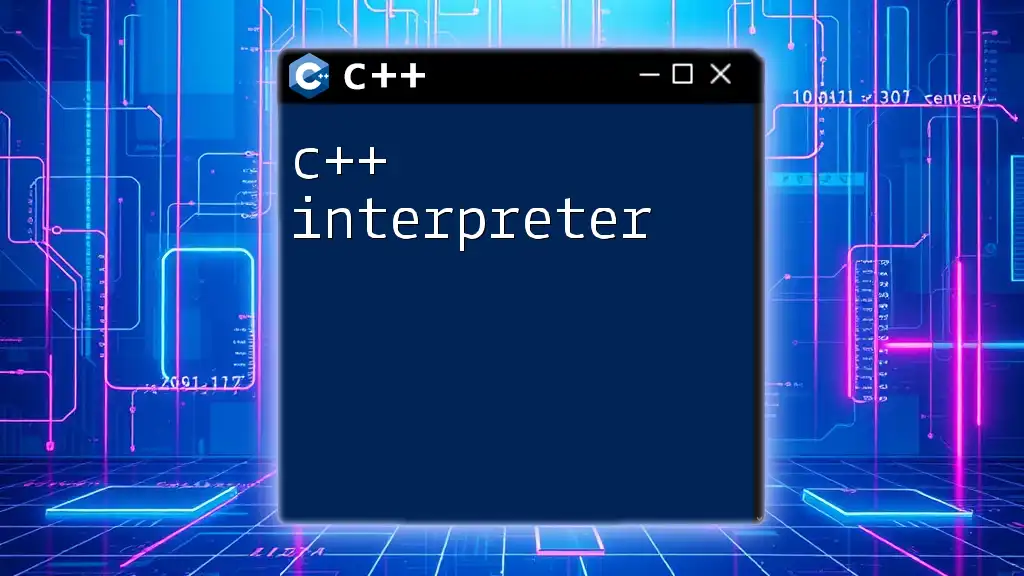
Call to Action
We invite you to share your experiences using `uint8_t` or any questions you may have in the comments section below. Your insights can foster discussions that benefit fellow developers. Don't forget to subscribe for our future posts, where we will delve deeper into more C++ topics!