The `_bstr_t` class in C++ is a smart pointer that automatically manages the lifetime of BSTR (Binary String) memory, facilitating the handling of strings in COM programming.
Here’s a simple example demonstrating the use of `_bstr_t`:
#include <comdef.h> // Include the comdef.h header for _bstr_t
int main() {
_bstr_t bstrExample = SysAllocString(L"Hello, World!"); // Allocate and assign a BSTR
wprintf(L"%s\n", (wchar_t*)bstrExample); // Use the _bstr_t to print the string
return 0;
}
What is BSTR?
A BSTR (Binary String) is a string data type used in Windows programming, particularly in COM (Component Object Model) applications. It primarily serves the purpose of interfacing with COM components and managing strings that contain Unicode characters. One of the defining characteristics of a BSTR is that it includes a length prefix, which allows for easy and safe manipulation.
Differences from Standard C++ Strings
Unlike standard C++ strings (`std::string`), BSTRs are allocated in the heap and must be freed using `SysFreeString()`. Additionally, BSTRs are encoded in UTF-16 format, which makes them suitable for internationalization but can introduce complexities when interacting with ASCII or other encodings.

What is _bstr_t?
The _bstr_t class in C++ simplifies the use of BSTRs by providing an object-oriented approach to string manipulation. This class is defined in the comdef.h header and streamlines memory management, ensuring that BSTRs are automatically freed when they go out of scope.
Why Use _bstr_t?
Using _bstr_t provides several advantages over raw BSTRs:
- Automatic Memory Management: _bstr_t automatically handles allocations and deallocations, reducing the chance of memory leaks.
- Easier String Operations: With operator overloading, performing operations like concatenation and assignment becomes intuitive and straightforward.
- Type Safety and Error Handling: The class encapsulates error-checking mechanisms, which makes it easier to work with strings safely.
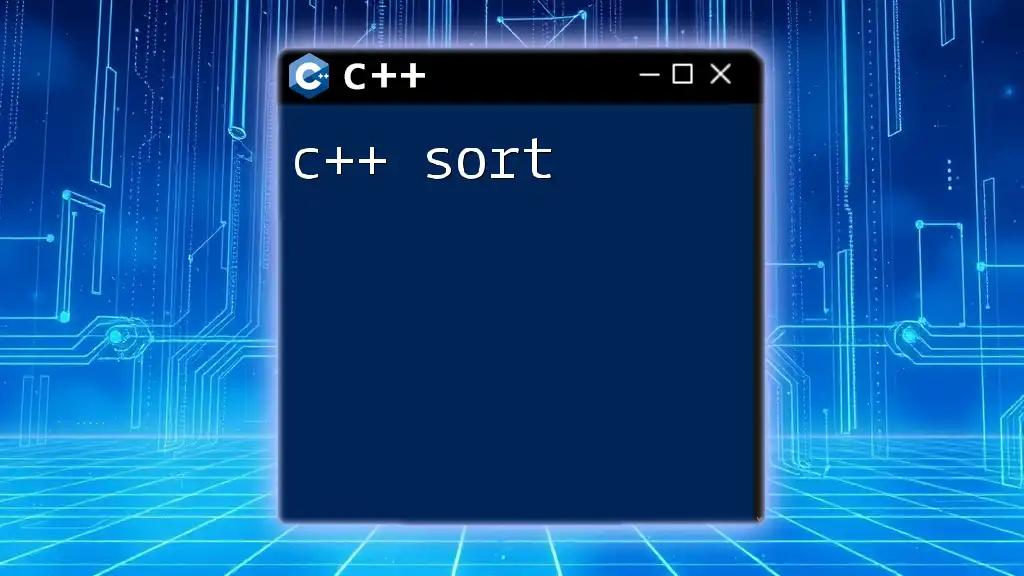
Key Features of _bstr_t
Automatic Memory Management
One of the most significant benefits of using _bstr_t is its automatic memory management capabilities. When an _bstr_t object is destroyed, the memory associated with the BSTR is automatically released, eliminating the need to call `SysFreeString()` manually.
Example Code: Here’s how you can utilize _bstr_t without worrying about memory leaks:
#include <comdef.h>
void ExampleAutoMemoryManagement() {
_bstr_t bstr("Hello, World!");
// Memory is automatically managed; no need to call SysFreeString
}
Conversion to and from Other String Formats
_bstr_t can easily convert between various string formats, making it versatile in different scenarios.**
-
Conversion to CString: You can convert _bstr_t to a `CString` object seamlessly.
-
Conversion to std::string: Similarly, converting _bstr_t to `std::string` is straightforward.
Code Snippet: Here’s how you can perform the conversions:
_bstr_t bstrExample("Sample String");
// Conversion to CString
CString cstrExample(bstrExample);
// Conversion to std::string
std::string stdStrExample((LPCTSTR)bstrExample);
Character Encoding
_bstr_t supports both UTF-16 and ANSI character formats**, making it easy to work with a wide range of text.
When handling string conversions, it's essential to keep track of the encoding. _bstr_t manages this internally, allowing for seamless use of both string types while ensuring that characters are stored correctly.
Code Snippet: Here's an example of encoding conversion:
_bstr_t bstr = L"Some UTF-16 text";
std::string converted((const char *)bstr);

Common Use Cases for _bstr_t
Working with COM Interfaces
bstr_t is particularly effective when interacting with COM objects. For instance, when invoking methods or accessing properties of a COM object that expects a BSTR argument, using _bstr_t makes the task easier and less error-prone.
Example Code:
void UseComInterface(IUnknown* pUnknown) {
_bstr_t bstrPropertyName("MyProperty");
VARIANT result;
VariantInit(&result);
pUnknown->GetProperty(bstrPropertyName, &result);
// Process the result...
}
String Manipulation
_bstr_t supports various string operations, including concatenation, comparison, and substring extraction, making it versatile for any text processing task in C++.
Basic String Operations:
_bstr_t str1("Hello");
_bstr_t str2("World");
_bstr_t result = str1 + " " + str2; // result holds "Hello World"
You can also compare two _bstr_t objects easily:
if (str1 == "Hello") {
// Do something
}
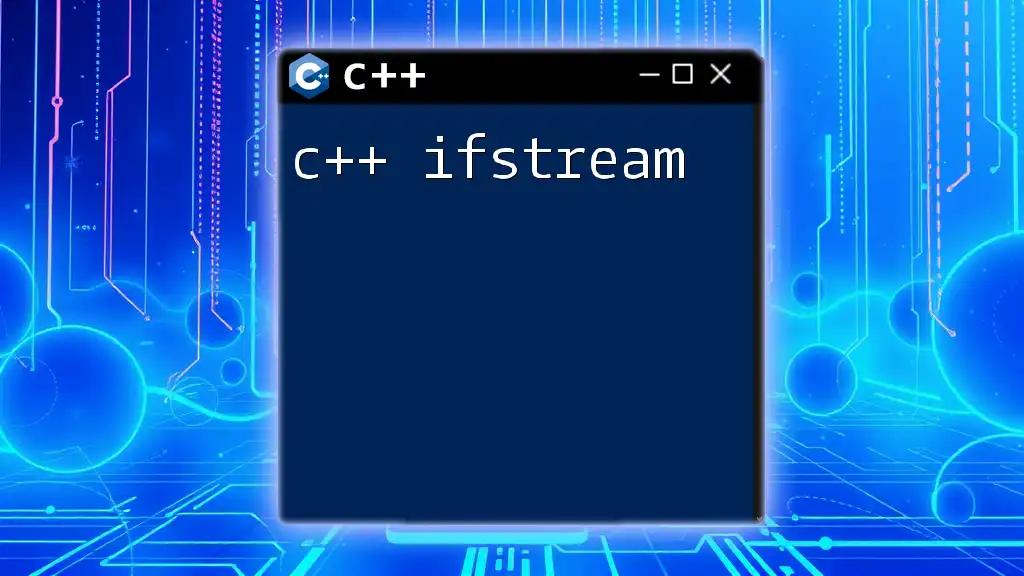
Best Practices When Using _bstr_t
Avoiding Memory Leaks
To ensure efficient memory usage while using _bstr_t, always prefer initializing your _bstr_t instances with string literals or other pre-allocated BSTRs. Be cautious when converting between types to prevent any unintended memory allocation and leaks.
Performance Considerations
While _bstr_t is highly convenient, it's essential to evaluate when to use it effectively. In scenarios where you only need lightweight string manipulation, a standard string may suffice. However, when interfacing with COM components or handling data in a BSTR format, _bstr_t provides significant ease of use and safety.
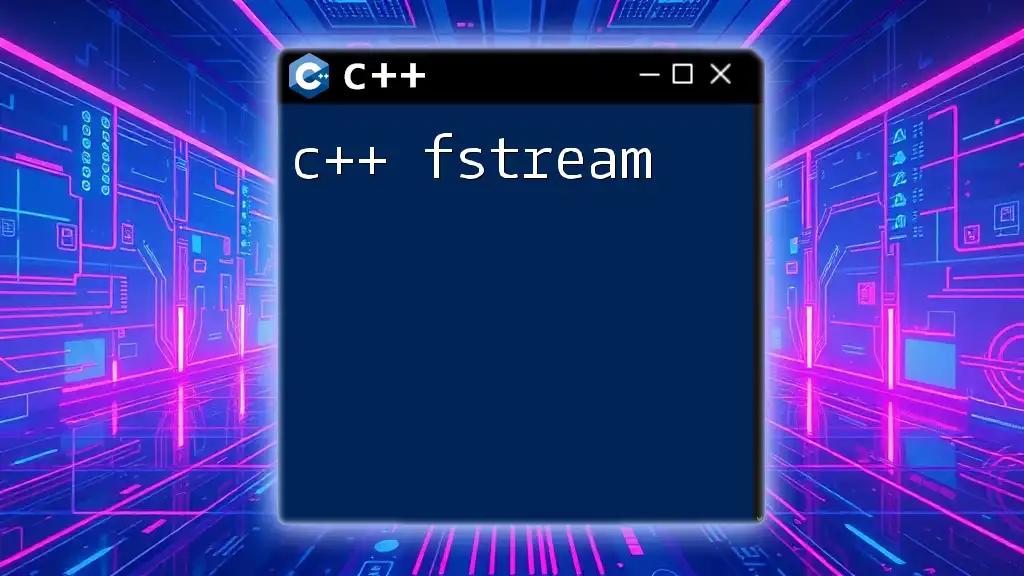
Debugging Tips and Troubleshooting
Common Issues
When using _bstr_t, developers may encounter issues like inadvertent memory management errors, particularly during string conversions or when using pointers. Improper handling of these cases can lead to subtle bugs.
Tools for Debugging
Using debugging tools such as Visual Studio’s built-in capabilities, developers can quickly troubleshoot memory-related issues. Tools like Valgrind or Visual Leak Detector can also help monitor memory usage, allowing developers to identify potential leaks associated with BSTR and _bstr_t usage.
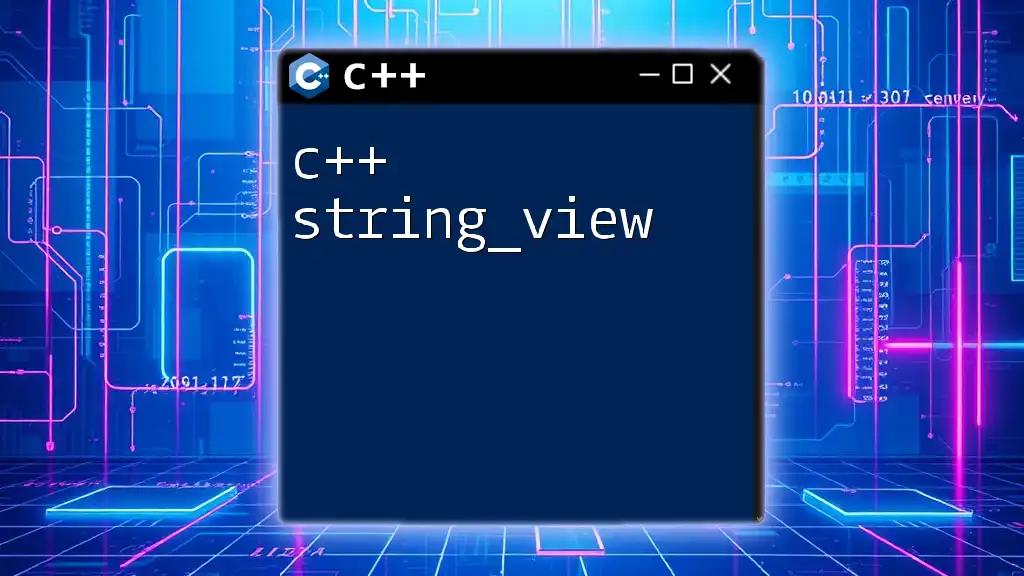
Conclusion
In summary, _bstr_t is a robust and user-friendly wrapper around the BSTR type in C++. It provides automatic memory management and seamless conversion between different string formats, making it invaluable for those working in COM environments. By following best practices and being aware of potential issues, developers can effectively harness the power of _bstr_t in their applications.
By exploring and implementing _bstr_t in your C++ projects, you will find it enhances both your productivity and the reliability of your code.