The `strtok` function in C++ is used to tokenize a string into smaller substrings (tokens) based on specified delimiters.
Here's an example:
#include <iostream>
#include <cstring>
int main() {
char str[] = "Hello, how are you?";
char* token = strtok(str, " ,?"); // Delimiters are space, comma, and question mark
while (token != nullptr) {
std::cout << token << std::endl;
token = strtok(nullptr, " ,?");
}
return 0;
}
Understanding the Basics of strtok
What is strtok?
strtok is a standard C library function that stands for "string token." It is used for splitting a string into smaller, manageable parts called "tokens." This function is essential, especially when you want to parse strings for specific delimiters, such as spaces, commas, or other characters that separate data elements.
How strtok Works
The operation of strtok is based on the concept of delimiters. When you call strtok, it scans the given string and identifies the delimiters specified. It then replaces the first occurrence of a delimiter with a null character (`'\0'`), effectively splitting the string at that point. In subsequent calls to strtok, you can pass a `NULL` pointer to continue parsing the same string. This allows you to retrieve each subsequent token one at a time.
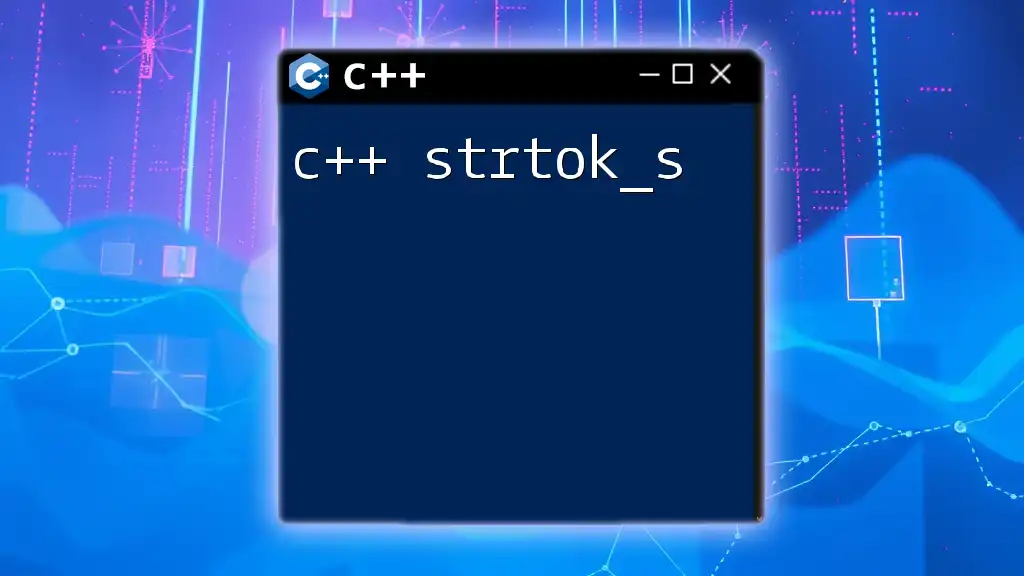
The Syntax of strtok in C++
The syntax structure of strtok is as follows:
char* strtok(char* str, const char* delimiters);
- str: The initial string to tokenize. On subsequent calls, it should be `NULL`.
- delimiters: A string containing all characters that will be treated as delimiters.
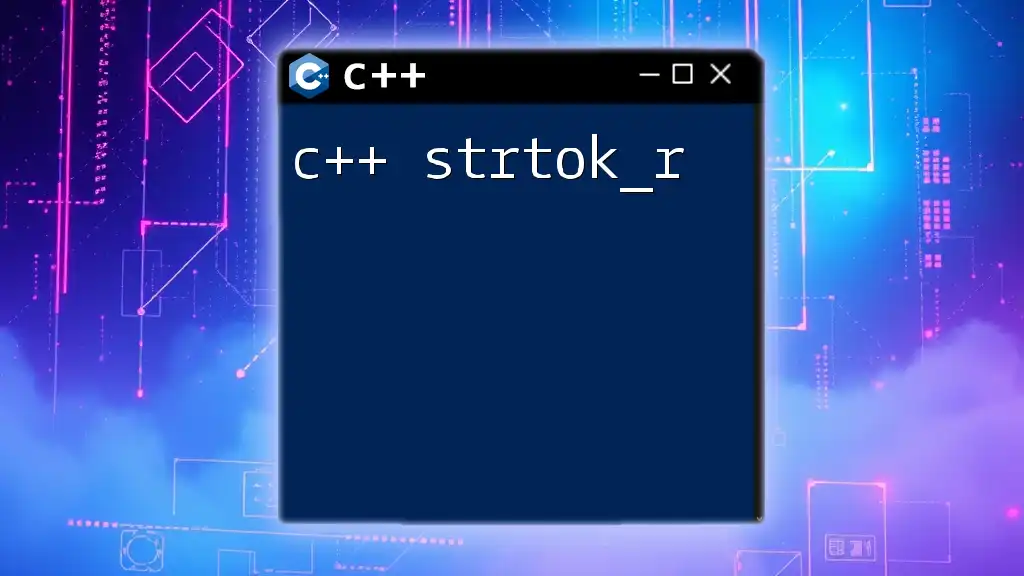
Using strtok: Step-by-Step Guide
Initial Tokenization
To start the tokenization process, you need to make an initial call to strtok with your target string and the delimiters. For example:
char str[] = "Hello, World! Welcome to C++.";
char* token = strtok(str, " ,.!"); // Delimiters: space, comma, period, exclamation
In this example, `str` is the string we want to tokenize, and `" ,.!“` specifies the characters that separate the tokens.
Continuing Tokenization
Once you have the first token from strtok, you can use a loop to retrieve all remaining tokens until no more are available. Here’s how it can be done:
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, " ,.!");
}
In this code snippet, each token is printed until strtok returns `NULL`, indicating there are no more tokens left in the string.
Handling Multiple Strings
One of the powerful features of strtok is that you can tokenize multiple strings in a single program execution. For example:
char str1[] = "apple,orange,banana";
char str2[] = "dog;cat;mouse";
char* token;
token = strtok(str1, ",");
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, ",");
}
token = strtok(str2, ";");
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, ";");
}
In this example, two different strings, `str1` and `str2`, are tokenized with different delimiters.
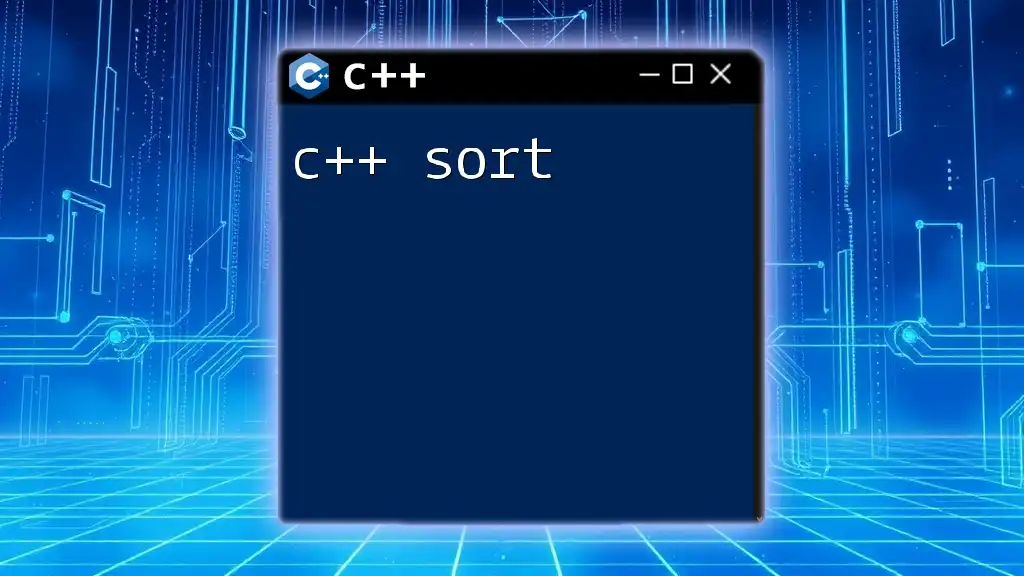
Practical Examples of strtok in Action
Example 1: Tokenizing a CSV Line
Tokenizing a CSV formatted string can be achieved easily with strtok. Here's how you can break down a simple CSV line:
char csvLine[] = "name,age,city";
char* token = strtok(csvLine, ",");
// Process each token sequentially
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, ",");
}
In this example, the output will be three separate tokens: name, age, and city. This illustrates how easy it is to handle CSV data in C++.
Example 2: Parsing Command Line Arguments
Another practical use of strtok is parsing command line input. Here's an example:
char command[] = "run -f file.txt -v verbose";
char* token = strtok(command, " ");
// Demonstrating how to retrieve flags and values
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, " ");
}
This snippet will output each segment of the input command, allowing you to easily access flags and their corresponding values.
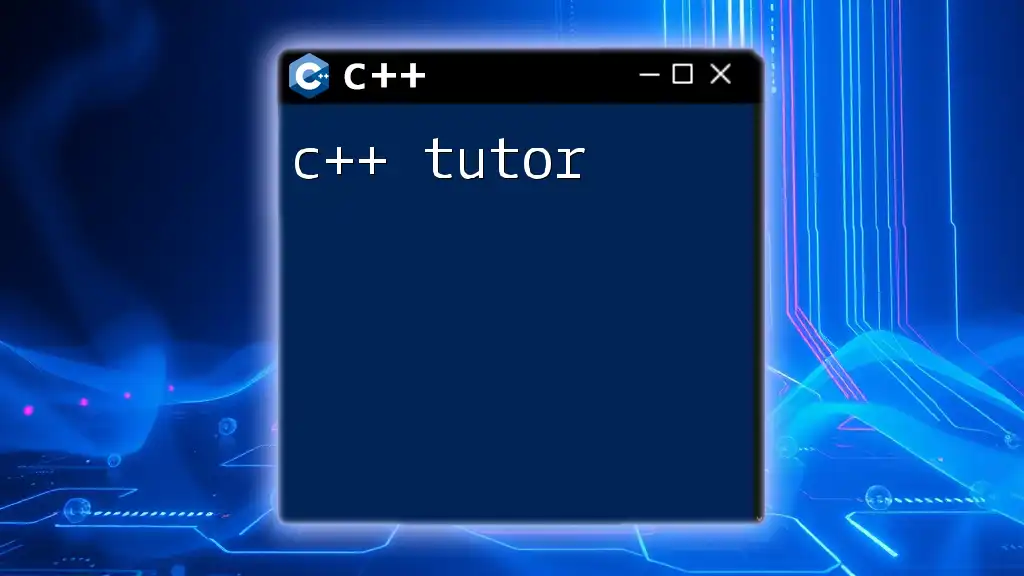
Common Mistakes to Avoid with strtok
Modifying Original String
A crucial aspect to remember is that strtok modifies the original input string by replacing delimiters with null characters. If you need to maintain the original string, make sure to work on a copy instead.
Returning NULL
It's essential to understand when strtok returns `NULL`. This happens when there are no more tokens left to extract from the string. Always check for `NULL` to prevent errors in your loop when processing tokens.
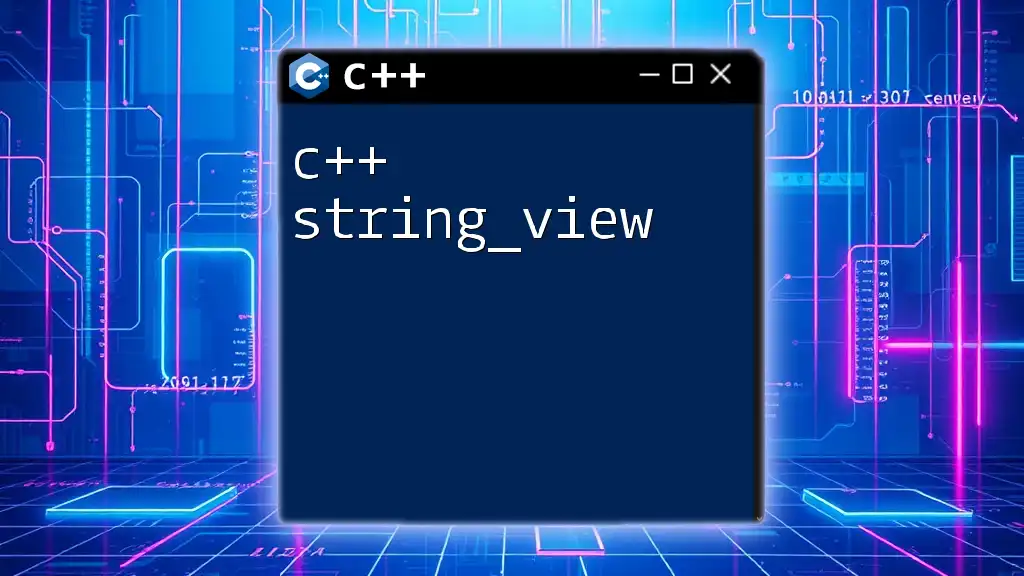
Advanced Usage of strtok
Implementing Custom Delimiters
You can define your own delimiters according to your specific needs. For instance:
const char* customDelimiters = ":-;";
By using this string as delimiters, you can tokenize based on your customized criteria.
Using strtok Safely
While strtok is convenient, it is not thread-safe, which could lead to issues in multi-threaded programs. Consider using alternatives like `std::string` and `std::stringstream` for more complex string handling tasks in C++.
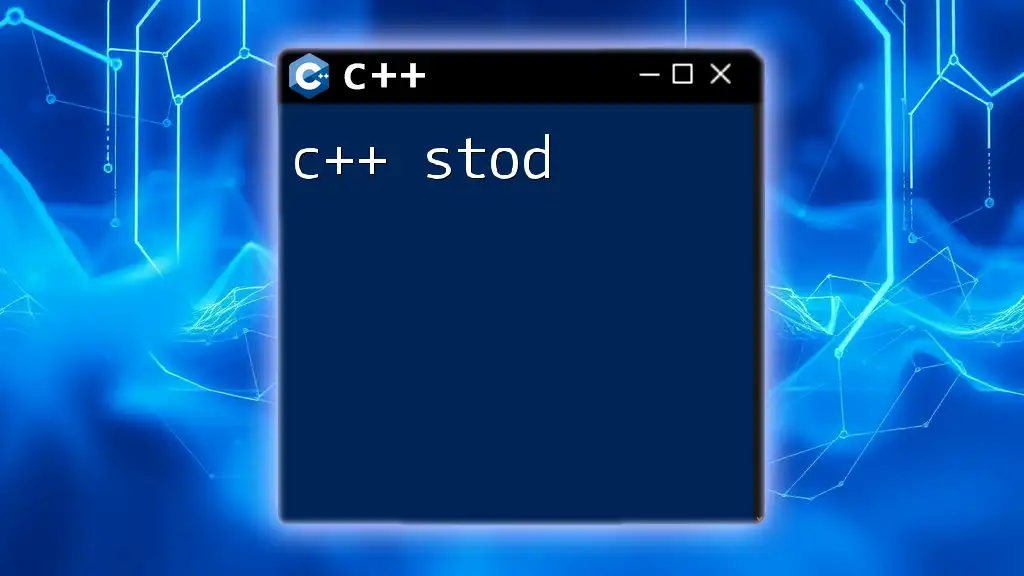
Conclusion
In summary, c++ strtok is a valuable tool for string manipulation, particularly for breaking a string into tokens based on specified delimiters. Understanding how it operates, along with its potential pitfalls, enables developers to harness its power effectively. As you explore and experiment with tokenization in your projects, you'll gain more confidence in using this and similar commands.
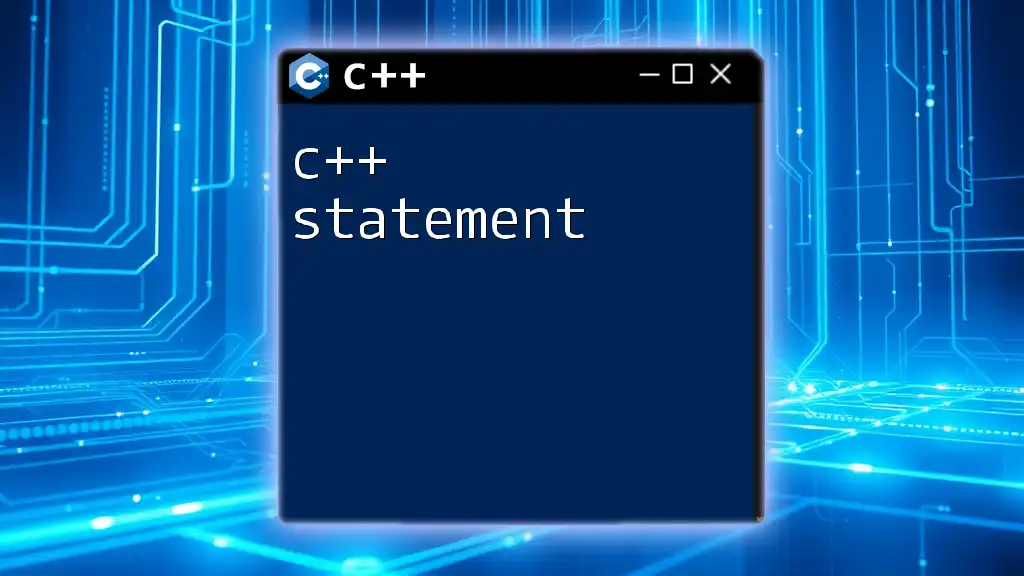
FAQ
What are the limitations of strtok?
While strtok is useful, it has several limitations, including handling strings in a single-threaded context and lack of reentrancy. Each call modifies the input string, which can lead to unintended side effects if you're not careful.
Can strtok be used in a multi-threaded environment?
Using strtok in a multi-threaded environment is risky due to its non-thread-safe nature. It is advisable to use thread-safe alternatives for parsing strings in concurrent applications.
What are alternatives to strtok in C++?
There are numerous alternatives to strtok, such as using `std::string::find` and `std::string::substr` for safer string tokenization. These methods allow for more flexibility and avoid issues related to modifying the original string.
By practicing with strtok, you'll find it an essential addition to your C++ toolkit for effective string management.