In C++, the `stat` function is used to retrieve information about a file, such as its size, permissions, and last modification time.
Here’s a simple example of using the `stat` function in C++:
#include <sys/stat.h>
#include <iostream>
int main() {
struct stat fileStat;
if (stat("example.txt", &fileStat) < 0) {
perror("stat");
return 1;
}
std::cout << "File Size: " << fileStat.st_size << " bytes\n";
return 0;
}
Understanding the `stat` Structure
What is the `stat` Structure?
The `stat` structure is a cornerstone of file system interaction in C++. It is defined in the `sys/stat.h` header and is used to obtain information about a file or a directory. This includes various metadata that can be crucial for applications that require file system manipulation or checking.
Fields in the `stat` Structure
The `stat` structure contains several fields, each serving a specific purpose:
- `st_mode`: This field indicates the file's type and its access permissions. It helps determine whether the file is a regular file, directory, symbolic link, etc.
- `st_ino`: The inode number, a unique identifier for files within a filesystem. It can be used to manage files efficiently, particularly in operations that concern file links.
- `st_dev`: This field contains the device ID. It helps identify the device on which the file resides.
- `st_nlink`: Indicates the number of hard links pointing to the file. This is essential for understanding file usage and link management.
- `st_uid`: The user ID of the file's owner. Important for determining access rights and ownership.
- `st_gid`: The group ID of the file's owner, which is similar to `st_uid` in terms of its relevance to permissions.
- `st_size`: Represents the total size of the file in bytes, crucial for size-related calculations or file sorting.
- `st_atime`: The last access time of the file. It is useful for processes that track file use.
- `st_mtime`: The last modification time of the file, which reflects when the content of the file was last changed.
- `st_ctime`: The last status change time, which updates whenever the file's metadata is changed (e.g., permissions).
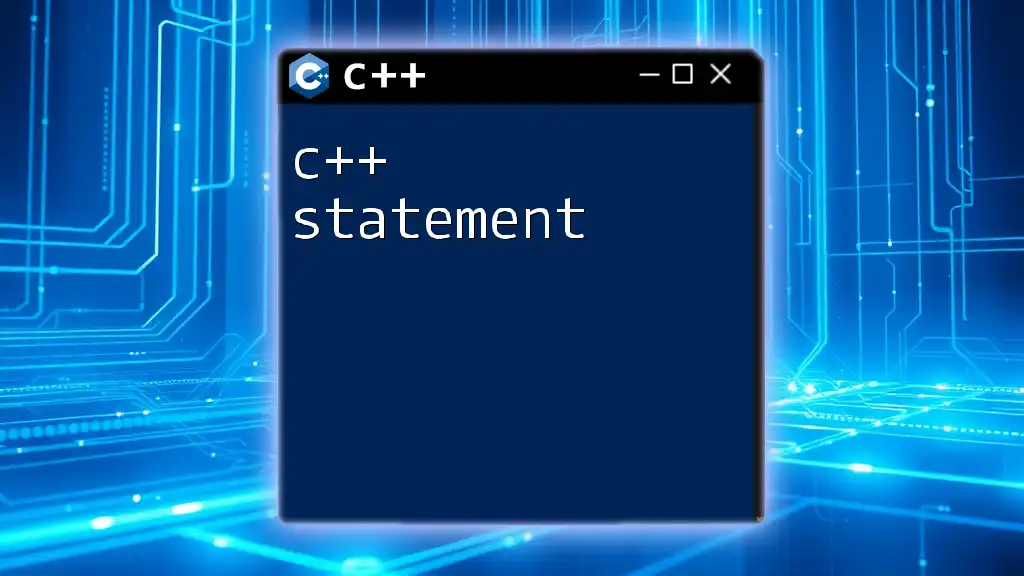
How to Use `stat` in C++
Including the Necessary Headers
Before leveraging the `stat` function, it is paramount to include the necessary headers in your C++ source code. You will typically need:
#include <sys/stat.h>
#include <unistd.h>
#include <iostream>
These headers provide the declarations necessary for using the `stat` function and for handling standard input and output functionalities.
Basic Syntax of `stat` Function
The syntax for using the `stat` function is straightforward:
int stat(const char *path, struct stat *buf);
Here, `path` is a string specifying the file or directory you want to check, and `buf` is a pointer to a `stat` structure where the information will be stored. The function returns `0` on success and `-1` on failure.
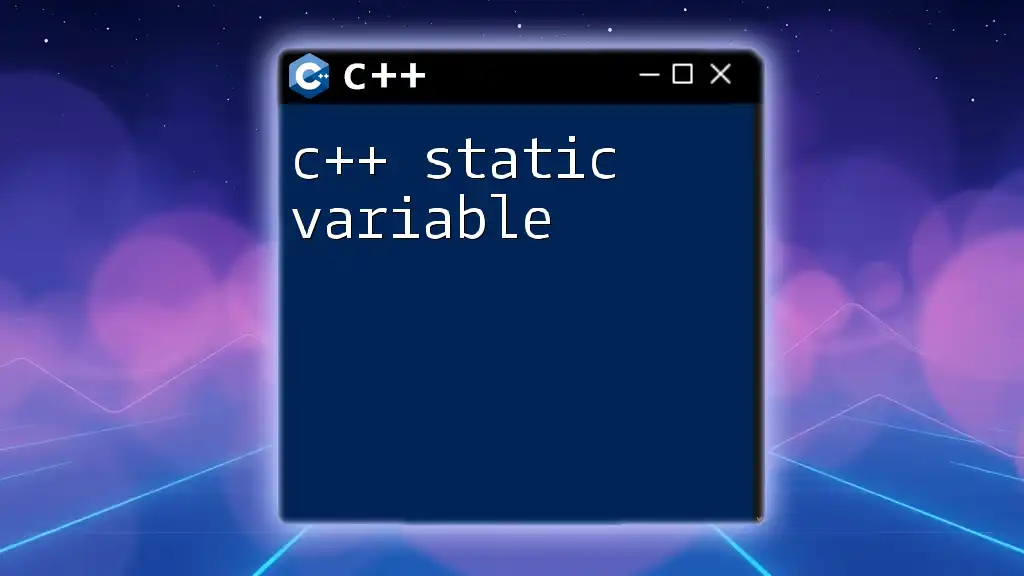
Example: Retrieving File Information
Setting Up the Example
To demonstrate how to utilize the `stat` function effectively, we will create a simple program that retrieves and prints the size and modification times of a file named `example.txt`.
Complete Code Snippet
#include <iostream>
#include <sys/stat.h>
#include <unistd.h>
int main() {
struct stat fileStat;
if (stat("example.txt", &fileStat) < 0) {
perror("stat");
return 1;
}
std::cout << "File Size: " << fileStat.st_size << " bytes\n";
std::cout << "Access Time: " << ctime(&fileStat.st_atime);
std::cout << "Modification Time: " << ctime(&fileStat.st_mtime);
return 0;
}
Explanation of Code
In this program, we first define a variable `fileStat` of the `stat` structure to hold the file information. The `stat` function is called with the path of the file and the reference to `fileStat`. If the function call is successful, we use `std::cout` to display the file size and access/modification times. Notably, we utilize `perror()` to capture and print any potential errors during the operation, ensuring robustness.
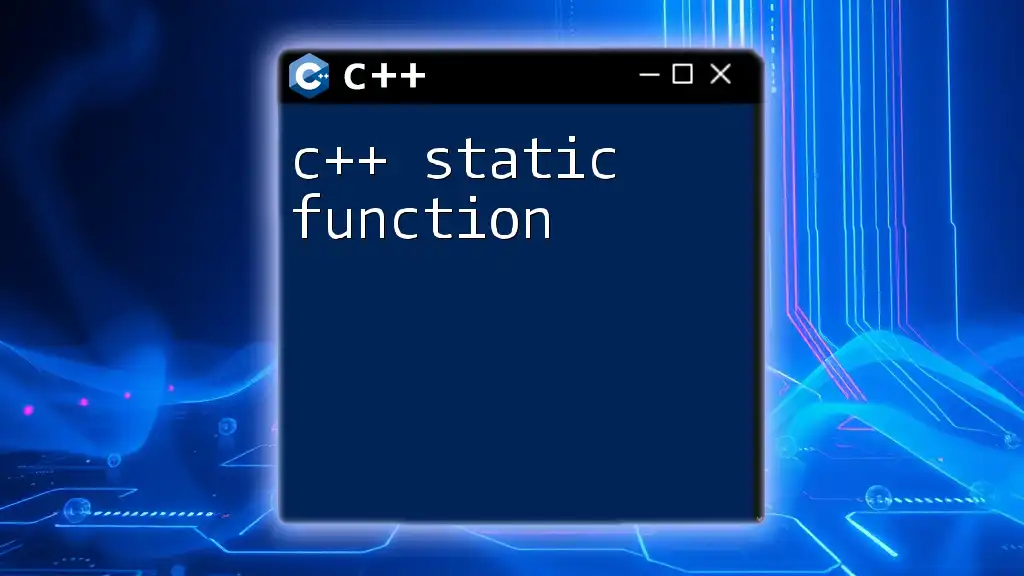
Checking File Types and Permissions
File Type Determination
To determine file types, you will examine the `st_mode` field of the `stat` structure. The file type can be evaluated using macros defined in `sys/stat.h`. For example, you can check if a file is a regular file, directory, or symbolic link.
if (fileStat.st_mode & S_IFREG) {
std::cout << "Regular file\n";
} else if (fileStat.st_mode & S_IFDIR) {
std::cout << "Directory\n";
} else if (fileStat.st_mode & S_IFLNK) {
std::cout << "Symbolic link\n";
} else {
std::cout << "Other type\n";
}
Understanding File Permissions
Access permissions can also be derived from `st_mode`. You can determine whether a file is readable, writable, or executable by checking specific bits within `st_mode`. For example:
if (fileStat.st_mode & S_IRUSR) {
std::cout << "User has read permission\n";
}
if (fileStat.st_mode & S_IWUSR) {
std::cout << "User has write permission\n";
}
if (fileStat.st_mode & S_IXUSR) {
std::cout << "User has execute permission\n";
}
By utilizing these checks, you can enforce security measures in your application based on file permissions.
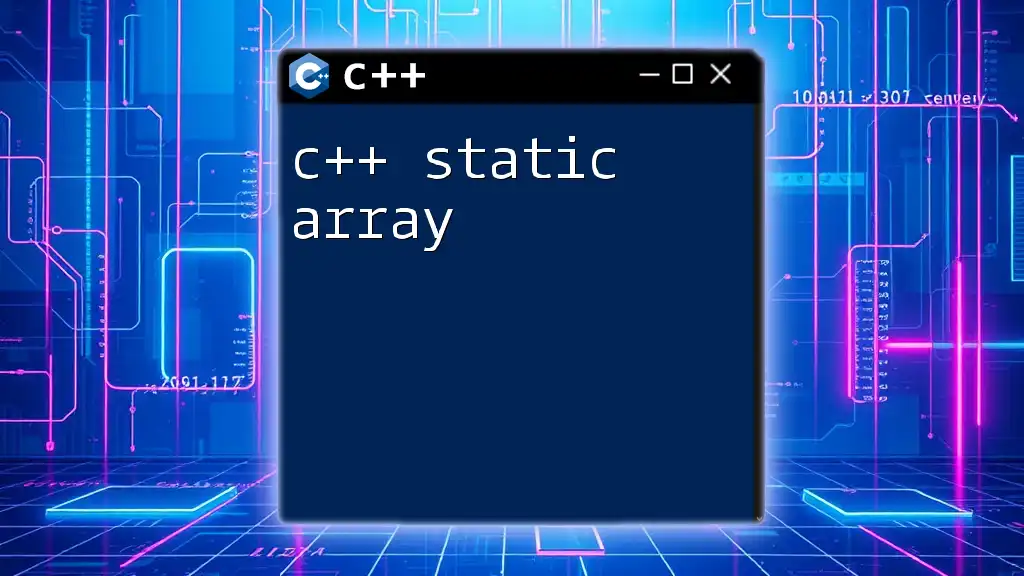
Common Use Cases for `stat`
File System Analysis
The `c++ stat` function is invaluable for file system analysis. It allows programmers to monitor the attributes of files dynamically, facilitating tasks such as file size comparison, type checking, and modification tracking.
Logging and Monitoring Applications
In applications that require continuous monitoring of file states, using `stat` enables developers to efficiently log file changes and access patterns.
Backup Systems
Backup systems can benefit from file statistics to identify changes in files since the last backup. Using `stat`, applications can quickly ascertain which files need to be backed up based on their `st_mtime`.
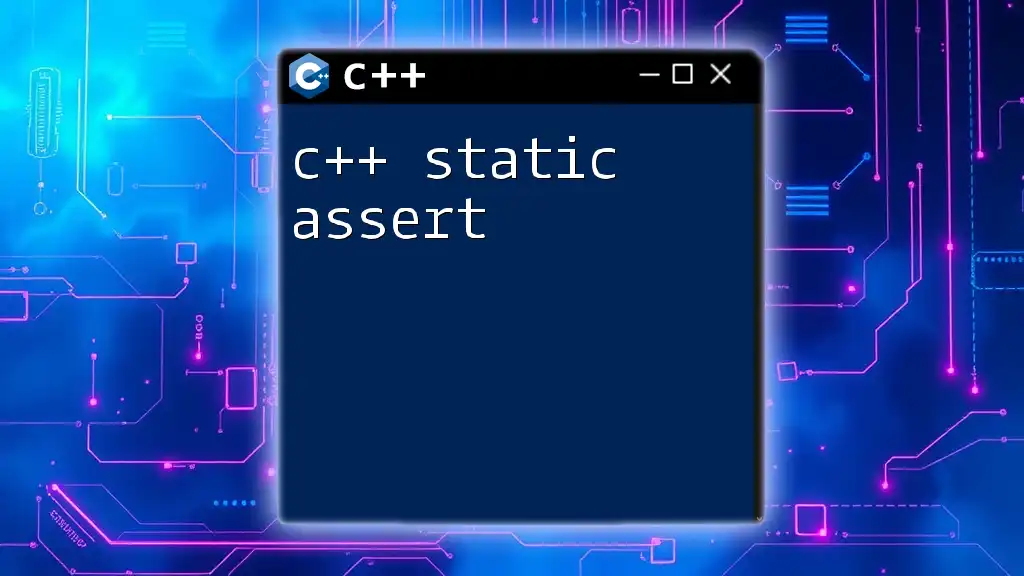
Best Practices when Using `stat`
Error Handling and Validations
When using `stat`, always check for potential errors. It is essential to handle cases where the file may not exist or permissions may not allow access. This can help avoid undefined behavior in your application.
Performance Considerations
If you operate on a large number of files, consider the performance implications of calling `stat` frequently. Batch processing or caching file metadata may yield significant performance improvements.
Handling Symbolic Links
In cases where your application needs to handle symbolic links, use `lstat` instead of `stat`. The `lstat` function behaves similarly but does not dereference symbolic links, thus returning information about the link itself, not the file it points to.
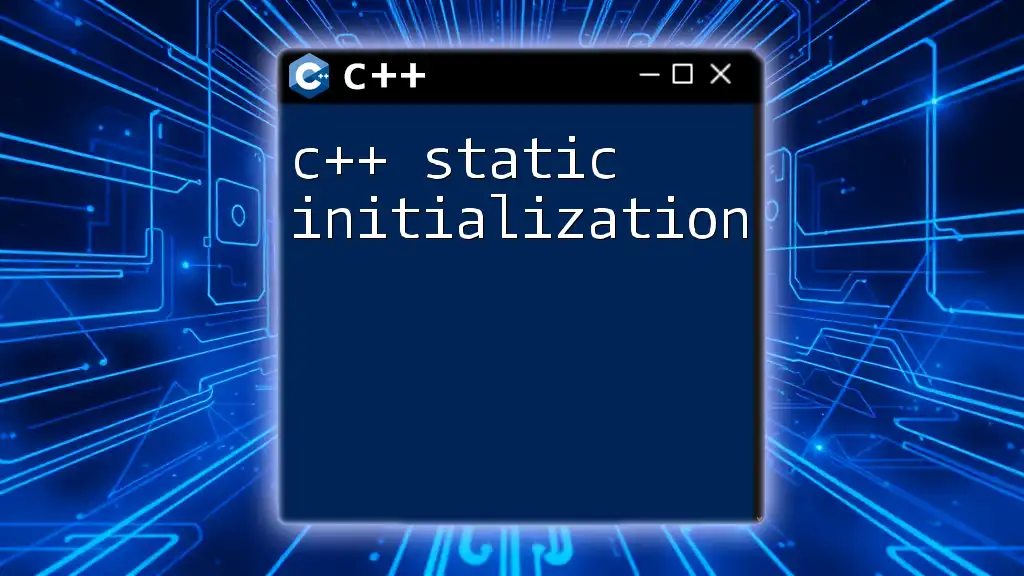
Conclusion
The `c++ stat` function is a powerful tool for dealing with file metadata. From understanding file types to managing permissions, `stat` provides comprehensive insights that can elevate your file handling capabilities. As you delve deeper into C++ programming, exploring the nuances of `stat` opens avenues for advanced file manipulation and system monitoring. Embracing this functionality can significantly enhance the robustness and effectiveness of your applications.